EV charging
API
Remotely manage EV charging
Check the charging status and charge electric vehicles with simple API requests.
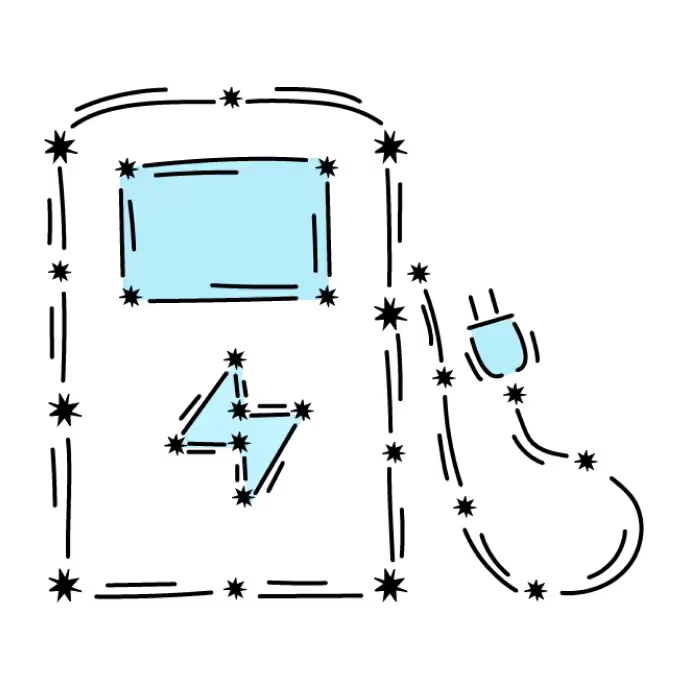
Charging status
Check whether an EV’s charging cable is plugged in and charging.
Start or stop charge
Remotely start or stop charging a battery electric (BEV) or plug-in hybrid vehicle (PHEV).
Get & set charge limit
Preserve battery health by managing charge limits for electric vehicles.
Product features
Compatible with 39 car brands
Friendly user consent flow
Works on 2015 and newer vehicles
Trusted & secure
Retrieve live data and trigger live actions
SDKs for Go, Java, Node.js, Python, and Ruby
Related industries
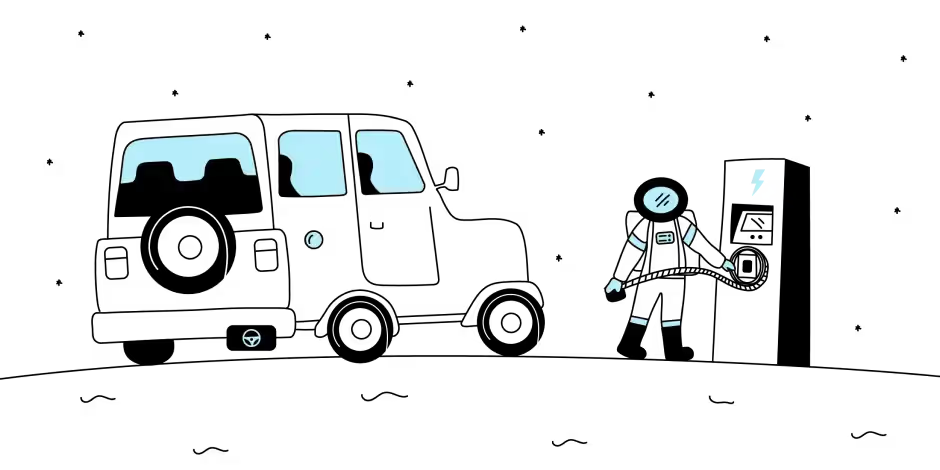
EV charging networks
Provide estimated charging times, automatic charging schedules, and EV trip planning in your app.

Energy & utility providers
Manage your customers’ residential EV charging to best balance electric grid load.
What we’re building
Latency and frequency of data availability may vary between makes and models.