A developer friendly API for RAM vehicles
Mobility apps and services use Smartcar’s API to verify mileage, manage EV charging, track fleets, and more. Our API platform allows vehicle owners to log in with their Uconnect account and connect their car to your app in just a few clicks.

What is Smartcar?
Smartcar enables developers to integrate their apps and services with RAM vehicles. Easily retrieve data and trigger actions with our simple and secure API for RAM vehicles. Our RAM API endpoints enable you to manage charging, track location, verify mileage, and much more.
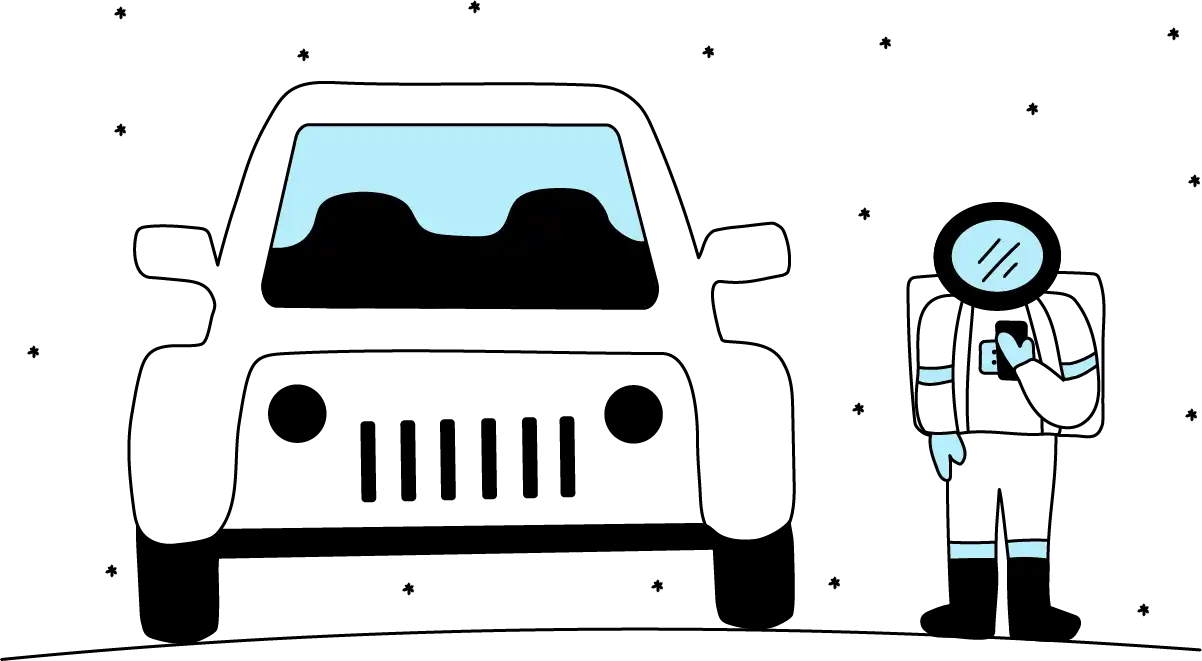
The Smartcar difference
Instead of building your own RAM integration, Smartcar's integration offers mobility businesses a range of benefits:
- A powerful developer experience
Customizable, collaborative, and user-friendly features for faster development. Our developer tools include robust docs, 8 SDKs, consent management, test simulators, dashboard insights, and more.
- Extensive vehicle support and growth
Integrate once and maintain a single integration across 39 car brands in North America and Europe.
- A commitment to consumer data privacy
A granular permissions system that enhances data protection and transparency for vehicle owners.
Learn more about Smartcar's RAM integration
The best way to build apps for RAM vehicles
Our RESTful API for RAM is fast, reliable, and easy to use with every tech stack. No matter which framework your mobile or web app is built in, our documentation and SDKs support every major language.
const smartcar = require('smartcar');
// Get all vehicles associated with this access token
const {vehicles} = await smartcar.getVehicles("<access-token>");
// Construct a new vehicle instance using the first vehicle's id
const vehicle = new smartcar.Vehicle(vehicles[0], "<access-token>");
// Fetch the vehicle's location
const location = await vehicle.location();
// Example http response from Smartcar
{
"latitude": 37.4292,
"longitude": 122.1381
}
import smartcar
# Get all vehicles associated with this access token
response = smartcar.get_vehicles("<access-token>")
# Construct a new vehicle instance using the first vehicle's id
vehicle = smartcar.Vehicle(response.vehicles[0], "<access-token>")
# Fetch the vehicle's location
location = vehicle.location()
// Example http response from Smartcar
{
"latitude": 37.4292,
"longitude": 122.1381
}
import com.smartcar.sdk.*;
// Get all vehicles associated with this access token
VehicleIds response = Smartcar.getVehicles("<access-token>");
String[] vehicleIds = response.getVehicleIds();
// Construct a new vehicle instance using the first vehicle's id
Vehicle vehicle = new Vehicle(vehicleIds[0], "<access-token>");
// Fetch the vehicle's location
VehicleLocation location = vehicle.location();
// Example http response from Smartcar
{
"latitude": 37.4292,
"longitude": 122.1381
}
import (
"context"
smartcar "github.com/smartcar/go-sdk"
);
// Create a smartcar client
var smartcarClient = smartcar.NewClient();
// Get all vehicles associated with this access token
var vehicleIDs, resErr = smartcarClient.GetVehicleIDs(
context.TODO(),
&smartcar.VehicleIDsParams{Access: "<access-token>"},
);
// Construct a new vehicle instance using the first vehicle's id
var vehicle = smartcarClient.NewVehicle(&smartcar.VehicleParams{
ID: vehicleIDs.VehicleIDs[0],
AccessToken: "<access-token>"},
);
// Fetch the vehicle's location
var fuel, resErr = vehicle.GetLocation(context.TODO());
// Example http response from Smartcar
{
"latitude": 37.4292,
"longitude": 122.1381
}
require 'smartcar'
# Get all vehicles associated with this access token
all_vehicles = Smartcar.get_vehicles(token: token)
# Construct a new vehicle instance using the first vehicle's id
vehicle = Smartcar::Vehicle.new(
token: "<access-token>",
id: all_vehicles.vehicles.first
)
# Fetch the vehicle's location
location = vehicle.location()
// Example http response from Smartcar
{
"latitude": 37.4292,
"longitude": 122.1381
}
const smartcar = require('smartcar');
// Get all vehicles associated with this access token
const {vehicles} = await smartcar.getVehicles("<access-token>");
// Construct a new vehicle instance using the first vehicle's id
const vehicle = new smartcar.Vehicle(vehicles[0], "<access-token>");
// Fetch the vehicle's odometer
const odometer = await vehicle.odometer();
// Example http response from Smartcar
{
"distance": 104.32
}
import smartcar
# Get all vehicles associated with this access token
response = smartcar.get_vehicles("<access-token>")
# Construct a new vehicle instance using the first vehicle's id
vehicle = smartcar.Vehicle(response.vehicles[0], "<access-token>")
# Fetch the vehicle's odometer
odometer = vehicle.odometer()
// Example http response from Smartcar
{
"distance": 104.32
}
import com.smartcar.sdk.*;
// Get all vehicles associated with this access token
VehicleIds response = Smartcar.getVehicles("<access-token>");
String[] vehicleIds = response.getVehicleIds();
// Construct a new vehicle instance using the first vehicle's id
Vehicle vehicle = new Vehicle(vehicleIds[0], "<access-token>");
// Fetch the vehicle's odometer
VehicleOdometer odometer = vehicle.odometer();
// Example http response from Smartcar
{
"distance": 104.32
}
import (
"context"
smartcar "github.com/smartcar/go-sdk"
);
// Create a smartcar client
var smartcarClient = smartcar.NewClient();
// Get all vehicles associated with this access token
var vehicleIDs, resErr = smartcarClient.GetVehicleIDs(
context.TODO(),
&smartcar.VehicleIDsParams{Access: "<access-token>"},
);
// Construct a new vehicle instance using the first vehicle's id
var vehicle = smartcarClient.NewVehicle(&smartcar.VehicleParams{
ID: vehicleIDs.VehicleIDs[0],
AccessToken: "<access-token>"},
);
// Fetch the vehicle's odometer
var odometer, resErr = vehicle.GetOdometer(context.TODO());
// Example http response from Smartcar
{
"distance": 104.32
}
require 'smartcar'
# Get all vehicles associated with this access token
all_vehicles = Smartcar.get_vehicles(token: token)
# Construct a new vehicle instance using the first vehicle's id
vehicle = Smartcar::Vehicle.new(
token: "<access-token>",
id: all_vehicles.vehicles.first
)
# Fetch the vehicle's odometer
odometer = vehicle.odometer()
// Example http response from Smartcar
{
"distance": 104.32
}
const smartcar = require('smartcar');
// Get all vehicles associated with this access token
const {vehicles} = await smartcar.getVehicles("<access-token>");
// Construct a new vehicle instance using the first vehicle's id
const vehicle = new smartcar.Vehicle(vehicles[0], "<access-token>");
// Lock the vehicle
await vehicle.lock();
// Unlock the vehicle
await vehicle.unlock();
// Example http response from Smartcar
{
"status": "success"
}
import smartcar
# Get all vehicles associated with this access token
response = smartcar.get_vehicles("<access-token>")
# Construct a new vehicle instance using the first vehicle's id
vehicle = smartcar.Vehicle(response.vehicles[0], "<access-token>")
# Lock the vehicle
vehicle.lock()
# Unlock the vehicle
vehicle.unlock()
// Example http response from Smartcar
{
"status": "success"
}
import com.smartcar.sdk.*;
// Get all vehicles associated with this access token
VehicleIds response = Smartcar.getVehicles("<access-token>");
String[] vehicleIds = response.getVehicleIds();
// Construct a new vehicle instance using the first vehicle's id
Vehicle vehicle = new Vehicle(vehicleIds[0], "<access-token>");
// Lock the vehicle
vehicle.lock();
// Unlock the vehicle
vehicle.unlock();
// Example http response from Smartcar
{
"status": "success"
}
import (
"context"
smartcar "github.com/smartcar/go-sdk"
);
// Create a smartcar client
var smartcarClient = smartcar.NewClient();
// Get all vehicles associated with this access token
var vehicleIDs, resErr = smartcarClient.GetVehicleIDs(
context.TODO(),
&smartcar.VehicleIDsParams{Access: "<access-token>"},
);
// Construct a new vehicle instance using the first vehicle's id
var vehicle = smartcarClient.NewVehicle(&smartcar.VehicleParams{
ID: vehicleIDs.VehicleIDs[0],
AccessToken: "<access-token>"},
);
// Lock the vehicle
var lock, resErr = vehicle.Lock(context.TODO());
// Unlock the vehicle
var unlock, resErr = vehicle.Unlock(context.TODO());
// Example http response from Smartcar
{
"status": "success"
}
require 'smartcar'
# Get all vehicles associated with this access token
all_vehicles = Smartcar.get_vehicles(token: token)
# Construct a new vehicle instance using the first vehicle's id
vehicle = Smartcar::Vehicle.new(
token: "<access-token>",
id: all_vehicles.vehicles.first
)
# Lock the vehicle
vehicle.lock!
# Unlock the vehicle
vehicle.unlock!
// Example http response from Smartcar
{
"status": "success"
}
const smartcar = require('smartcar');
// Get all vehicles associated with this access token
const {vehicles} = await smartcar.getVehicles("<access-token>");
// Construct a new vehicle instance using the first vehicle's id
const vehicle = new smartcar.Vehicle(vehicles[0], "<access-token>");
// Fetch the vehicle's battery level
const battery = await vehicle.battery();
// Fetch the vehicle's battery capacity
const batteryCapacity = await vehicle.batteryCapacity();
// Example http response from Smartcar (battery level)
{
"percentRemaining": 0.3,
"range": 40.5,
}
// Example http response from Smartcar (battery capacity)
{
"capacity": 28.7,
}
import smartcar
# Get all vehicles associated with this access token
response = smartcar.get_vehicles("<access-token>")
# Construct a new vehicle instance using the first vehicle's id
vehicle = smartcar.Vehicle(response.vehicles[0], "<access-token>")
# Fetch the vehicle's battery level
battery = vehicle.battery()
# Fetch the vehicle's battery capacity
battery_capacity = vehicle.battery_capacity()
// Example http response from Smartcar (battery level)
{
"percentRemaining": 0.3,
"range": 40.5,
}
// Example http response from Smartcar (battery capacity)
{
"capacity": 28.7,
}
import com.smartcar.sdk.*;
// Get all vehicles associated with this access token
VehicleIds response = Smartcar.getVehicles("<access-token>");
String[] vehicleIds = response.getVehicleIds();
// Construct a new vehicle instance using the first vehicle's id
Vehicle vehicle = new Vehicle(vehicleIds[0], "<access-token>");
// Fetch the vehicle's battery level
VehicleBattery battery = vehicle.battery();
// Fetch the vehicle's battery capacity
VehicleBatteryCapacity batteryCapacity = vehicle.batteryCapacity();
// Example http response from Smartcar (battery level)
{
"percentRemaining": 0.3,
"range": 40.5,
}
// Example http response from Smartcar (battery capacity)
{
"capacity": 28.7,
}
import (
"context"
smartcar "github.com/smartcar/go-sdk"
);
// Create a smartcar client
var smartcarClient = smartcar.NewClient();
// Get all vehicles associated with this access token
var vehicleIDs, resErr = smartcarClient.GetVehicleIDs(
context.TODO(),
&smartcar.VehicleIDsParams{Access: "<access-token>"},
);
// Construct a new vehicle instance using the first vehicle's id
var vehicle = smartcarClient.NewVehicle(&smartcar.VehicleParams{
ID: vehicleIDs.VehicleIDs[0],
AccessToken: "<access-token>"},
);
// Fetch the vehicle's battery level
var battery, resErr = vehicle.GetBattery(context.TODO());
// Fetch the vehicle's battery capacity
var batteryCapacity, resErr = vehicle.GetBatteryCapacity(context.TODO());
// Example http response from Smartcar (battery level)
{
"percentRemaining": 0.3,
"range": 40.5,
}
// Example http response from Smartcar (battery capacity)
{
"capacity": 28.7,
}
require 'smartcar'
# Get all vehicles associated with this access token
all_vehicles = Smartcar.get_vehicles(token: token)
# Construct a new vehicle instance using the first vehicle's id
vehicle = Smartcar::Vehicle.new(
token: "<access-token>",
id: all_vehicles.vehicles.first
)
# Fetch the vehicle's battery level
battery = vehicle.battery()
# Fetch the vehicle's battery capacity
battery_capacity = vehicle.battery_capacity()
// Example http response from Smartcar (battery level)
{
"percentRemaining": 0.3,
"range": 40.5,
}
// Example http response from Smartcar (battery capacity)
{
"capacity": 28.7,
}
const smartcar = require('smartcar');
// Get all vehicles associated with this access token
const {vehicles} = await smartcar.getVehicles("<access-token>");
// Construct a new vehicle instance using the first vehicle's id
const vehicle = new smartcar.Vehicle(vehicles[0], "<access-token>");
// Fetch the vehicle's charging status
const charge = await vehicle.charge();
// Start the vehicle's charging session
await vehicle.startCharge();
// Set the vehicle's charge limit
await vehicle.setChargeLimit(0.8);
// Example http response from Smartcar (charging status)
{
"isPluggedIn": true,
"state":"FULLY_CHARGED"
}
// Example http response from Smartcar (start charge)
{
"status": "success"
}
// Example http response from Smartcar (set charge limit)
{
"status": "success"
}
import smartcar
# Get all vehicles associated with this access token
response = smartcar.get_vehicles("<access-token>")
# Construct a new vehicle instance using the first vehicle's id
vehicle = smartcar.Vehicle(response.vehicles[0], "<access-token>")
# Fetch the vehicle's charging status
charge = vehicle.charge()
# Start the vehicle's charging session
vehicle.start_charge()
# Set the vehicle's charge limit
vehicle.set_charge_limit(0.8)
// Example http response from Smartcar (charging status)
{
"isPluggedIn": true,
"state":"FULLY_CHARGED"
}
// Example http response from Smartcar (start charge)
{
"status": "success"
}
// Example http response from Smartcar (set charge limit)
{
"status": "success"
}
import com.smartcar.sdk.*;
// Get all vehicles associated with this access token
VehicleIds response = Smartcar.getVehicles("<access-token>");
String[] vehicleIds = response.getVehicleIds();
// Construct a new vehicle instance using the first vehicle's id
Vehicle vehicle = new Vehicle(vehicleIds[0], "<access-token>");
// Fetch the vehicle's charging status
VehicleCharge charge = vehicle.charge();
// Start the vehicle's charging session
vehicle.startCharge();
// Set the vehicle's charge limit
vehicle.setChargeLimit(0.8)
// Example http response from Smartcar (charging status)
{
"isPluggedIn": true,
"state":"FULLY_CHARGED"
}
// Example http response from Smartcar (start charge)
{
"status": "success"
}
// Example http response from Smartcar (set charge limit)
{
"status": "success"
}
import (
"context"
smartcar "github.com/smartcar/go-sdk"
);
// Create a smartcar client
var smartcarClient = smartcar.NewClient();
// Get all vehicles associated with this access token
var vehicleIDs, resErr = smartcarClient.GetVehicleIDs(
context.TODO(),
&smartcar.VehicleIDsParams{Access: "<access-token>"},
);
// Construct a new vehicle instance using the first vehicle's id
var vehicle = smartcarClient.NewVehicle(&smartcar.VehicleParams{
ID: vehicleIDs.VehicleIDs[0],
AccessToken: "<access-token>"},
);
// Fetch the vehicle's charging status
var charge, resErr = vehicle.GetCharge(context.TODO());
// Start the vehicle's charging session
var startCharge, resErr = vehicle.StartCharge(context.TODO());
// Example http response from Smartcar (charging status)
{
"isPluggedIn": true,
"state":"FULLY_CHARGED"
}
// Example http response from Smartcar (start charge)
{
"status": "success"
}
// Example http response from Smartcar (set charge limit)
{
"status": "success"
}
require 'smartcar'
# Get all vehicles associated with this access token
all_vehicles = Smartcar.get_vehicles(token: token)
# Construct a new vehicle instance using the first vehicle's id
vehicle = Smartcar::Vehicle.new(
token: "<access-token>",
id: all_vehicles.vehicles.first
)
# Fetch the vehicle's charging status
charge = vehicle.charge()
# Start the vehicle's charging session
vehicle.startCharge!
# Set the vehicle's charge limit
vehicle.set_charge_limit!(0.8)
// Example http response from Smartcar (charging status)
{
"isPluggedIn": true,
"state":"FULLY_CHARGED"
}
// Example http response from Smartcar (start charge)
{
"status": "success"
}
// Example http response from Smartcar (set charge limit)
{
"status": "success"
}
const smartcar = require('smartcar');
// Get all vehicles associated with this access token
const {vehicles} = await smartcar.getVehicles("<access-token>");
// Construct a new vehicle instance using the first vehicle's id
const vehicle = new smartcar.Vehicle(vehicles[0], "<access-token>");
// Fetch the vehicle's fuel tank level
const fuel = await vehicle.fuel();
// Example http response from Smartcar
{
"amountRemaining": 53.2,
"percentRemaining": 0.3,
"range": 40.5
}
import smartcar
# Get all vehicles associated with this access token
response = smartcar.get_vehicles("<access-token>")
# Construct a new vehicle instance using the first vehicle's id
vehicle = smartcar.Vehicle(response.vehicles[0], "<access-token>")
# Fetch the vehicle's fuel tank level
fuel = vehicle.fuel()
// Example http response from Smartcar
{
"amountRemaining": 53.2,
"percentRemaining": 0.3,
"range": 40.5
}
import com.smartcar.sdk.*;
// Get all vehicles associated with this access token
VehicleIds response = Smartcar.getVehicles("<access-token>");
String[] vehicleIds = response.getVehicleIds();
// Construct a new vehicle instance using the first vehicle's id
Vehicle vehicle = new Vehicle(vehicleIds[0], "<access-token>");
// Fetch the vehicle's fuel tank level
VehicleFuel fuel = vehicle.fuel();
// Example http response from Smartcar
{
"amountRemaining": 53.2,
"percentRemaining": 0.3,
"range": 40.5
}
import (
"context"
smartcar "github.com/smartcar/go-sdk"
);
// Create a smartcar client
var smartcarClient = smartcar.NewClient();
// Get all vehicles associated with this access token
var vehicleIDs, resErr = smartcarClient.GetVehicleIDs(
context.TODO(),
&smartcar.VehicleIDsParams{Access: "<access-token>"},
);
// Construct a new vehicle instance using the first vehicle's id
var vehicle = smartcarClient.NewVehicle(&smartcar.VehicleParams{
ID: vehicleIDs.VehicleIDs[0],
AccessToken: "<access-token>"},
);
// Fetch the vehicle's fuel tank level
var fuel, resErr = vehicle.GetFuel(context.TODO());
// Example http response from Smartcar
{
"amountRemaining": 53.2,
"percentRemaining": 0.3,
"range": 40.5
}
require 'smartcar'
# Get all vehicles associated with this access token
all_vehicles = Smartcar.get_vehicles(token: token)
# Construct a new vehicle instance using the first vehicle's id
vehicle = Smartcar::Vehicle.new(
token: "<access-token>",
id: all_vehicles.vehicles.first
)
# Fetch the vehicle's fuel tank level
fuel = vehicle.fuel()
// Example http response from Smartcar
{
"amountRemaining": 53.2,
"percentRemaining": 0.3,
"range": 40.5
}
const smartcar = require('smartcar');
// Get all vehicles associated with this access token
const {vehicles} = await smartcar.getVehicles("<access-token>");
// Construct a new vehicle instance using the first vehicle's id
const vehicle = new smartcar.Vehicle(vehicles[0], "<access-token>");
// Fetch the vehicle's tire pressure
const tirePressure = await vehicle.tirePressure();
// Example http response from Smartcar
{
"backLeft": 219.3,
"backRight": 219.3,
"frontLeft": 219.3,
"frontRight": 219.3,
}
import smartcar
# Get all vehicles associated with this access token
response = smartcar.get_vehicles("<access-token>")
# Construct a new vehicle instance using the first vehicle's id
vehicle = smartcar.Vehicle(response.vehicles[0], "<access-token>")
# Fetch the vehicle's tire pressure
tire_pressure = vehicle.tirePressure()
// Example http response from Smartcar
{
"backLeft": 219.3,
"backRight": 219.3,
"frontLeft": 219.3,
"frontRight": 219.3,
}
import com.smartcar.sdk.*;
// Get all vehicles associated with this access token
VehicleIds response = Smartcar.getVehicles("<access-token>");
String[] vehicleIds = response.getVehicleIds();
// Construct a new vehicle instance using the first vehicle's id
Vehicle vehicle = new Vehicle(vehicleIds[0], "<access-token>");
// Fetch the vehicle's attributes
VehicleTirePressure tirePressure = vehicle.tirePressure();
// Example http response from Smartcar
{
"backLeft": 219.3,
"backRight": 219.3,
"frontLeft": 219.3,
"frontRight": 219.3,
}
import (
"context"
smartcar "github.com/smartcar/go-sdk"
);
// Create a smartcar client
var smartcarClient = smartcar.NewClient();
// Get all vehicles associated with this access token
var vehicleIDs, resErr = smartcarClient.GetVehicleIDs(
context.TODO(),
&smartcar.VehicleIDsParams{Access: "<access-token>"},
);
// Construct a new vehicle instance using the first vehicle's id
var vehicle = smartcarClient.NewVehicle(&smartcar.VehicleParams{
ID: vehicleIDs.VehicleIDs[0],
AccessToken: "<access-token>"}
);
// Fetch the vehicle's tire pressure
var tirePressure, resErr = vehicle.GetTirePressure(context.TODO());
// Example http response from Smartcar
{
"backLeft": 219.3,
"backRight": 219.3,
"frontLeft": 219.3,
"frontRight": 219.3,
}
require 'smartcar'
# Get all vehicles associated with this access token
all_vehicles = Smartcar.get_vehicles(token: token)
# Construct a new vehicle instance using the first vehicle's id
vehicle = Smartcar::Vehicle.new(
token: "<access-token>",
id: all_vehicles.vehicles.first
)
# Fetch the vehicle's tire pressure
tire_pressure = vehicle.tire_pressure()
// Example http response from Smartcar
{
"backLeft": 219.3,
"backRight": 219.3,
"frontLeft": 219.3,
"frontRight": 219.3,
}
const smartcar = require('smartcar');
// Get all vehicles associated with this access token
const {vehicles} = await smartcar.getVehicles("<access-token>");
// Construct a new vehicle instance using the first vehicle's id
const vehicle = new smartcar.Vehicle(vehicles[0], "<access-token>");
// Fetch the vehicle's engine oil life
const oil = await vehicle.oil();
// Example http response from Smartcar
{
"lifeRemaining": 0.35
}
import smartcar
# Get all vehicles associated with this access token
response = smartcar.get_vehicles("<access-token>")
# Construct a new vehicle instance using the first vehicle's id
vehicle = smartcar.Vehicle(response.vehicles[0], "<access-token>")
# Fetch the vehicle's engine oil life
oil = vehicle.oil()
// Example http response from Smartcar
{
"lifeRemaining": 0.35
}
import com.smartcar.sdk.*;
// Get all vehicles associated with this access token
VehicleIds response = Smartcar.getVehicles("<access-token>");
String[] vehicleIds = response.getVehicleIds();
// Construct a new vehicle instance using the first vehicle's id
Vehicle vehicle = new Vehicle(vehicleIds[0], "<access-token>");
// Fetch the vehicle's engine oil life
VehicleEngineOil oil = vehicle.oil();
// Example http response from Smartcar
{
"lifeRemaining": 0.35
}
import (
"context"
smartcar "github.com/smartcar/go-sdk"
);
// Create a smartcar client
var smartcarClient = smartcar.NewClient();
// Get all vehicles associated with this access token
var vehicleIDs, resErr = smartcarClient.GetVehicleIDs(
context.TODO(),
&smartcar.VehicleIDsParams{Access: "<access-token>"},
);
// Construct a new vehicle instance using the first vehicle's id
var vehicle = smartcarClient.NewVehicle(&smartcar.VehicleParams{
ID: vehicleIDs.VehicleIDs[0],
AccessToken: "<access-token>"},
);
// Fetch the vehicle's engine oil life
var oil, resErr = vehicle.GetOil(context.TODO());
// Example http response from Smartcar
{
"lifeRemaining": 0.35
}
require 'smartcar'
# Get all vehicles associated with this access token
all_vehicles = Smartcar.get_vehicles(token: token)
# Construct a new vehicle instance using the first vehicle's id
vehicle = Smartcar::Vehicle.new(
token: "<access-token>",
id: all_vehicles.vehicles.first
)
# Fetch the vehicle's engine oil life
engine_oil = vehicle.engine_oil()
// Example http response from Smartcar
{
"lifeRemaining": 0.35
}
const smartcar = require('smartcar');
// Get all vehicles associated with this access token
const {vehicles} = await smartcar.getVehicles("<access-token>");
// Construct a new vehicle instance using the first vehicle's id
const vehicle = new smartcar.Vehicle(vehicles[0], "<access-token>");
// Fetch the vehicle's attributes
const vehicleAttributes = await vehicle.attributes();
// Example http response from Smartcar
{
"id": "36ab27d0-fd9d-4455-823a-ce30af709ffc",
"make": "TESLA",
"model": "Model S",
"year": 2014
}
import smartcar
# Get all vehicles associated with this access token
response = smartcar.get_vehicles("<access-token>")
# Construct a new vehicle instance using the first vehicle's id
vehicle = smartcar.Vehicle(response.vehicles[0], "<access-token>")
# Fetch the vehicle's attributes
vehicle_attributes = vehicle.attributes()
// Example http response from Smartcar
{
"id": "36ab27d0-fd9d-4455-823a-ce30af709ffc",
"make": "TESLA",
"model": "Model S",
"year": 2014
}
import com.smartcar.sdk.*;
// Get all vehicles associated with this access token
VehicleIds response = Smartcar.getVehicles("<access-token>");
String[] vehicleIds = response.getVehicleIds();
// Construct a new vehicle instance using the first vehicle's id
Vehicle vehicle = new Vehicle(vehicleIds[0], "<access-token>");
// Fetch the vehicle's attributes
VehicleAttributes vehicleAttributes = vehicle.attributes();
// Example http response from Smartcar
{
"id": "36ab27d0-fd9d-4455-823a-ce30af709ffc",
"make": "TESLA",
"model": "Model S",
"year": 2014
}
import (
"context"
smartcar "github.com/smartcar/go-sdk"
);
// Create a smartcar client
var smartcarClient = smartcar.NewClient();
// Get all vehicles associated with this access token
var vehicleIDs, resErr = smartcarClient.GetVehicleIDs(
context.TODO(),
&smartcar.VehicleIDsParams{Access: "<access-token>"},
);
// Construct a new vehicle instance using the first vehicle's id
var vehicle = smartcarClient.NewVehicle(&smartcar.VehicleParams{
ID: vehicleIDs.VehicleIDs[0],
AccessToken: "<access-token>"},
);
// Fetch the vehicle's attributes
var info, resErr = vehicle.GetInfo(context.TODO());
// Example http response from Smartcar
{
"id": "36ab27d0-fd9d-4455-823a-ce30af709ffc",
"make": "TESLA",
"model": "Model S",
"year": 2014
}
require 'smartcar'
# Get all vehicles associated with this access token
all_vehicles = Smartcar.get_vehicles(token: token)
# Construct a new vehicle instance using the first vehicle's id
vehicle = Smartcar::Vehicle.new(
token: "<access-token>",
id: all_vehicles.vehicles.first
)
# Fetch the vehicle's attributes
vehicle_attributes = vehicle.attributes()
// Example http response from Smartcar
{
"id": "36ab27d0-fd9d-4455-823a-ce30af709ffc",
"make": "TESLA",
"model": "Model S",
"year": 2014
}
const smartcar = require('smartcar');
// Get all vehicles associated with this access token
const {vehicles} = await smartcar.getVehicles("<access-token>");
// Construct a new vehicle instance using the first vehicle's id
const vehicle = new smartcar.Vehicle(vehicles[0], "<access-token>");
// Fetch the vehicle's VIN
const vin = await vehicle.vin();
// Example http response from Smartcar
{
"vin": "1234A67Q90F2T4567"
}
import smartcar
# Get all vehicles associated with this access token
response = smartcar.get_vehicles("<access-token>")
# Construct a new vehicle instance using the first vehicle's id
vehicle = smartcar.Vehicle(response.vehicles[0], "<access-token>")
# Fetch the vehicle's VIN
vin = vehicle.vin()
// Example http response from Smartcar
{
"vin": "1234A67Q90F2T4567"
}
import com.smartcar.sdk.*;
// Get all vehicles associated with this access token
VehicleIds response = Smartcar.getVehicles("<access-token>");
String[] vehicleIds = response.getVehicleIds();
// Construct a new vehicle instance using the first vehicle's id
Vehicle vehicle = new Vehicle(vehicleIds[0], "<access-token>");
// Fetch the vehicle's VIN
VehicleVin vin = vehicle.vin();
// Example http response from Smartcar
{
"vin": "1234A67Q90F2T4567"
}
import (
"context"
smartcar "github.com/smartcar/go-sdk"
);
// Create a smartcar client
var smartcarClient = smartcar.NewClient();
// Get all vehicles associated with this access token
var vehicleIDs, resErr = smartcarClient.GetVehicleIDs(
context.TODO(),
&smartcar.VehicleIDsParams{Access: "<access-token>"},
);
// Construct a new vehicle instance using the first vehicle's id
var vehicle = smartcarClient.NewVehicle(&smartcar.VehicleParams{
ID: vehicleIDs.VehicleIDs[0],
AccessToken: "<access-token>"},
);
// Fetch the vehicle's VIN
var VIN, resErr = vehicle.GetVIN(context.TODO());
// Example http response from Smartcar
{
"vin": "1234A67Q90F2T4567"
}
require 'smartcar'
# Get all vehicles associated with this access token
all_vehicles = Smartcar.get_vehicles(token: token)
# Construct a new vehicle instance using the first vehicle's id
vehicle = Smartcar::Vehicle.new(
token: "<access-token>",
id: all_vehicles.vehicles.first
)
# Fetch the vehicle's VIN
vin = vehicle.vin()
// Example http response from Smartcar
{
"vin": "1234A67Q90F2T4567"
}
Product features
- Compatible withRAMtelematics
Extensive documentation and SDKs
Friendly user consent flow
Trusted & secure
Access to live car data
No need for aftermarket hardware
Compatible with your RAM
Our API platform is compatible with 40 vehicle brands including RAM. Whether you have the RAM 1500, the 2500, or the 3500, your app can connect to all RAM models that support the Uconnect app.
RAM, the RAM logo, and its respective model names are a trademark of Stellantis and does not indicate endorsement of or affiliation with Smartcar.