Tire pressure
API
Monitor tire pressure remotely
Inspect a car’s tire pressure with simple API requests.
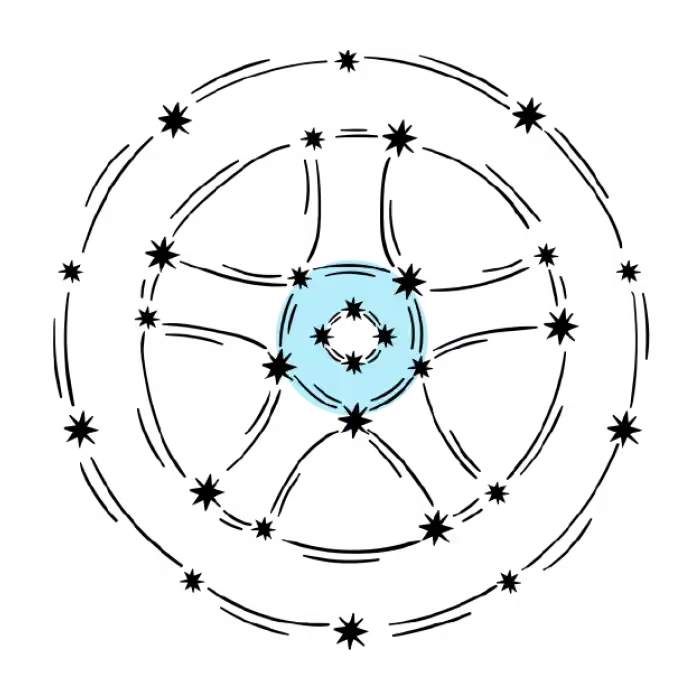
Tire pressure
Retrieve the air pressure for each tire from a vehicle’s TPMS.
Product features
Compatible with 39 car brands
Friendly user consent flow
Works on 2015 and newer vehicles
Trusted & secure
Access to live car data
SDKs for Go, Java, Node.js, Python, and Ruby
Related industries

Fleet management
Allow fleet managers to monitor fuel tank levels and driver efficiency across all vehicles in their fleet.

On-demand services
Encourage customers to schedule an on-demand maintenance appointment whenever their vehicle’s tire pressure is low.
What we’re building
Latency and frequency of data availability may vary between makes and models.