Lock/unlock
API
Unlock cars from your app
The Smartcar platform lets your mobility app issue, manage, and share digital car keys for your customers.
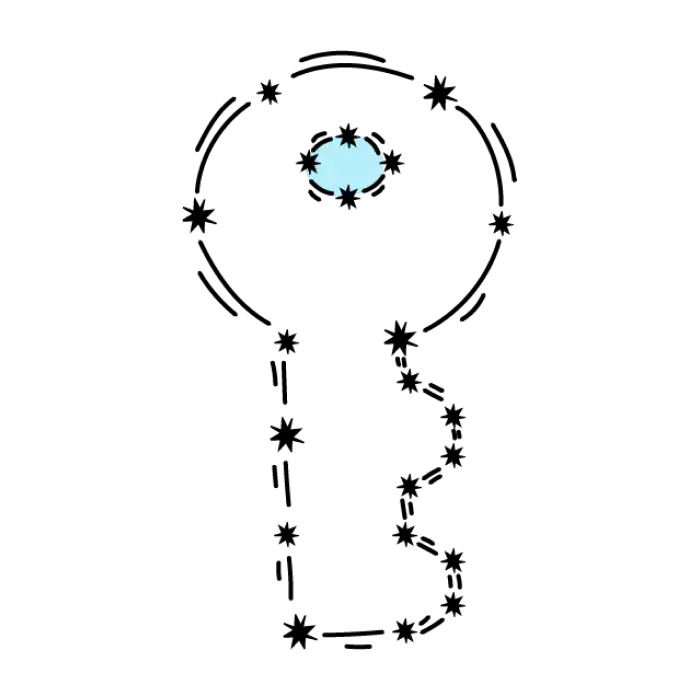
Lock or unlock
Lock or unlock a vehicle’s doors.
Product features
Compatible with 39 car brands
Friendly user consent flow
Works on 2015 and newer vehicles
Trusted & secure
Trigger live actions
SDKs for Go, Java, Node.js, Python, and Ruby
Related industries
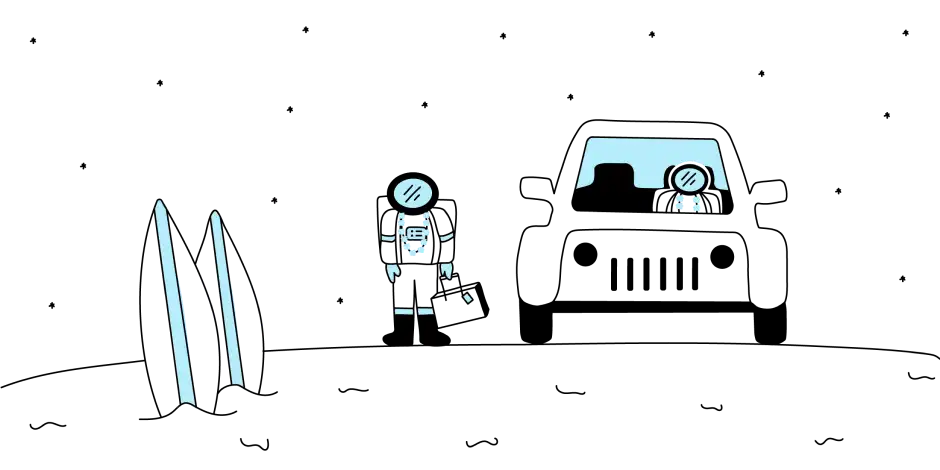
Peer-to-peer car sharing
Offer contactless rentals featuring digital keys directly in your car sharing app.
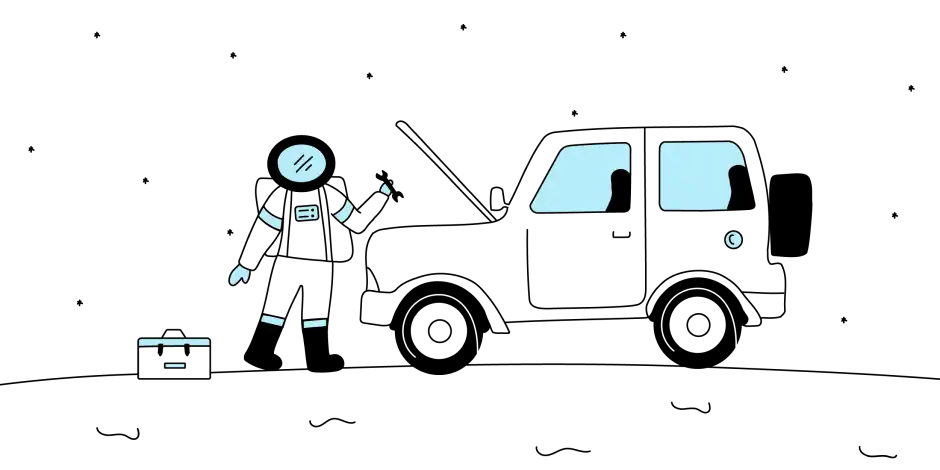
On-demand services
Offer contactless services by letting customers share a digital car key with your technicians.
What we’re building
Latency and frequency of data availability may vary between makes and models.