EV battery
API
Click, consent, retrieve SoC.
Read an electric vehicle’s battery level and battery capacity with simple API requests.

Battery level
Retrieve the state of charge (SoC) and the remaining range from a battery electric vehicle (BEV) or a plug-in hybrid vehicle (PHEV).
Battery capacity
Know the capacity of an electric vehicle’s battery.
Product features
Compatible with 39 car brands
Friendly user consent flow
Works on 2015 and newer vehicles
Trusted & secure
Access to live car data
SDKs for Go, Java, Node.js, Python, and Ruby
Related industries
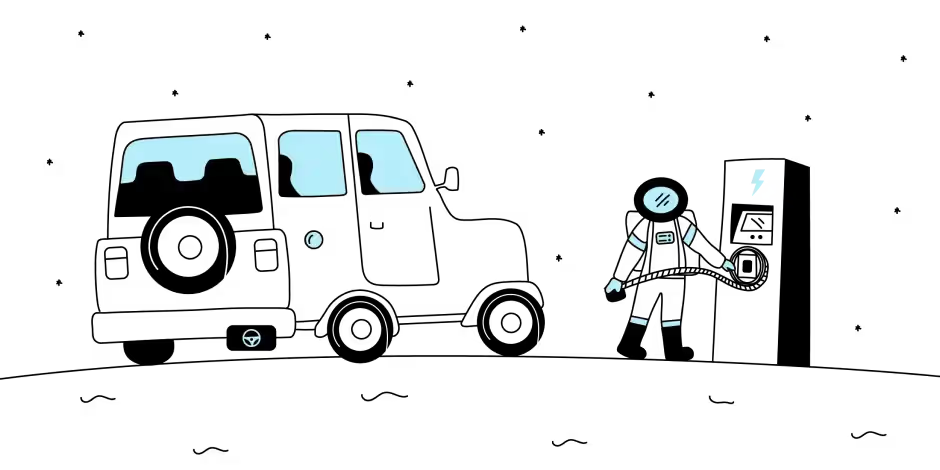
EV charging networks
Provide estimated charging times, automatic charging schedules, and EV trip planning in your app.

Energy & utility providers
Manage your customers’ residential EV charging to best balance electric grid load.
What we’re building
Latency and frequency of data availability may vary between makes and models.