As you might have heard, we recently redesigned the Smartcar dashboard. Our goal at Smartcar is to make building apps that communicate with cars fast and simple — and the goal of the redesign was to make the process of getting started with Smartcar much easier.
One of our redesign’s many improvements was to reduce the number of steps you have to complete between logging in and viewing your API credentials. In our old dashboard, you would have to select an application on every login. Our redesign has eliminated this unnecessary friction point, reducing the amount of time and the number of steps it takes to get started with the Smartcar API.
The task of removing an application selection page — a page where we list all of your apps and let you pick the configuration you want to see — forced us to think through the following questions:
- What should we do, if a developer just signed up and hasn’t yet registered an application?
- If a developer has more than one application, which one should we load on login?
- If a developer navigates to an invalid application (i.e. an application that they do not have access to), what should we do?
In this blog post, we’ll explain how we tackled each of these questions using React-Router, Redux, and Redux-Sagas. In other words, what happens behind the scenes during your first few seconds on the Smartcar dashboard?
The old flow

On signup
If you signed up on our old dashboard, we would force you to “Register an App” on login. As a developer, you would:
- Sign up for an account at smartcar.com.
- Register an app by entering an app name.
- View your client ID and secret that you can use to make requests to the Smartcar API.
This flow presented the following friction points:
- You may not know what “registering an app” means, particularly if you aren’t fully familiar with our API.
- Even if you know what a registered app is, you may not know what to name it. (Even our team has piled up hundreds of apps with some variation of the name “Test App.”)
On login
If you already created an account and registered an app, and then logged into our old dashboard, you would:
- View a list of your apps.
- Select the application you want to see (or register a new one).
- View your client ID and secret that you can use to make requests to the Smartcar API.
Similarly to our signup flow, this process wasn’t perfect:
- A user who is just getting started with Smartcar will only have one app. Still, they’ll have to go through the app selection screen on every login — just to get to the same app.

Invalid app
Finally, if you tried to navigate to an application that you do not have access to (e.g. if you’ve bookmarked an app from another account), we would render an “Application Does Not Exist” component that lets you choose an existing application or register a new one.
Today’s flow
In our redesigned dashboard, we simplified the login flow as follows:
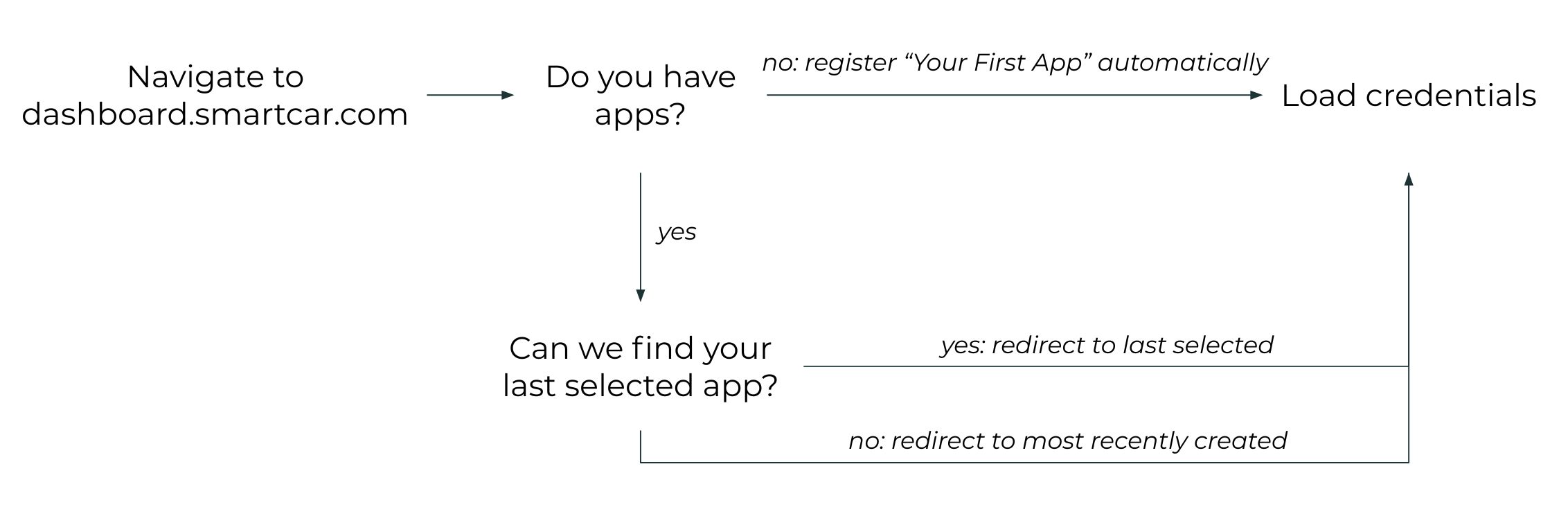
On signup
- Sign up for an account at smartcar.com.
- We automatically register “Your First App” and load your application credentials.
On login
- Log in at smartcar.com.
- Fetch your applications.
- If we can access your most recently viewed app from a previous session, we load the credentials for your most recently viewed app.
- Otherwise, we load your most recently created app.
For an invalid app route
We load an error page with links to your existing applications, and a button to register a new app.
How we do it
Here’s how we implement this flow in our React app:
Step 1: Initialize applications
When you first log in to the dashboard, we render an InitializeApplications component. This component renders a spinner and dispatches an “INITIALIZE_APPLICATIONS” action to the redux store on mount.
Step 2: Trigger initialize applications saga
Our Saga middleware intercepts the Redux action, and executes the following:
Step 2.1: Fetch applications
Step 2.2: Check size of the applications array
If the user doesn’t have any registered applications, automatically register an application.
Step 2.3: If a selected application exists (in local storage), load the selected app
If the user is logging back in on the same device, we can extract their most recently selected application. This is because we use Redux-Persist to persist elements of the user’s redux store in local storage.
Step 2.4: Otherwise, load the user’s most recently registered app
The saga
Here’s what our initialize applications saga looks like (edited for clarity):
Step 3: Render the selected app’s credentials
Now, we render the application! If an application doesn’t exist in the user’s set of applications, we render an “Application Not Found” page.
Here’s what the credentials view looks like to the user:

Now you know how we decide what app to show you when you log in! Give it a try for yourself by creating an account at smartcar.com, and let us know what you think!
Smartcar is an API that allows mobile and web apps to communicate with connected cars (think “check odometer” or “unlock doors”) — across vehicle brands, no hardware needed.
Want to take our API for a spin? Check out our docs and get started with our demo app! Have any questions or feedback? Shoot us an email! 🚀