# Make Specific Endpoints
Source: https://smartcar.com/docs/api-reference/about-brand-specific
In cases where there are many differences in how each OEM provides the same data, Smartcar will provide you with a make-specific endpoint before making it widely available to all supported makes. This allows our customers to start taking advantage of these endpoints and share feedback with Smartcar earlier.
These endpoints will maintain consistent behavior and will not "break" with future releases. In time, a make-specific endpoint might be standardized across various makes, eliminating the need to indicate the make in the URL. You won't need to modify your code but you might benefit from a newly standardized version.
When using make specific endpoints, please ensure to specify the make in lower case format. Using upper case letters will result in a PERMISSION error.
# All Vehicles
Source: https://smartcar.com/docs/api-reference/all-vehicles
GET /vehicles
Returns a paged list of all vehicles connected to the application for the current authorized `user`.
## Request
**Query**
The number of vehicles to return per page. `Max: 50`
The index to start the `vehicles` list at.
## Response
Metadata about the current list of elements.
The total number of elements for the entire query (not just the given page).
The current start index of the returned list of elements.
An array of vehicle IDs.
```json Example Response
{
"paging": {
"count": 25,
"offset": 10
},
"vehicles": [
"36ab27d0-fd9d-4455-823a-ce30af709ffc"
]
}
```
# API Errors
Source: https://smartcar.com/docs/api-reference/api-errors
Smartcar uses HTTP status codes to indicate success or failure of API requests. This includes:
* `2XX`: indicates success
* `4XX`: indicates an invalid request (e.g. a required parameter is missing from the request body)
* `5XX`: indicates Smartcar-related issues (e.g. a vehicle is not capable of fulfilling a request).
## Error Response
All Smartcar errors contain the following fields:
| Name | Type | Description |
| ----------- | ------ | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------ |
| type | string | A unique identifier that groups codes into broad categories of errors |
| code | string | A short, descriptive identifier for the error that occurred |
| description | string | A short description of the code that provides additional information about the error. The description is always provided in English. |
| docURL | string | A link to Smartcar’s doc center guide for the given type and code |
| statusCode | number | The HTTP status code |
| requestId | string | Smartcar’s request ID |
| resolution | object | An object with at least one enumerated property named as "type" that specifies which action can be taken to resolve this error. There are three possible values for the property "type": `RETRY_LATER` - Retry the request at a later time; `REAUTHENTICATE` - Prompt the user to re-authenticate in Smartcar Connect; and `CONTACT_SUPPORT` - Contact Smartcar’s support team. This object could contain other properties depending on the "type" of error. |
| detail | array | An array of objects that provide further detail regarding the error. Only included with validation errors. |
## Error Codes
| Code | Type | Status |
| ----------------------------- | ---------------------------- | ------ |
| `ACCOUNT_ISSUE` | `CONNECTED_SERVICES_ACCOUNT` | 400 |
| `AUTHENTICATION_FAILED` | `CONNECTED_SERVICES_ACCOUNT` | 400 |
| `NO_VEHICLES` | `CONNECTED_SERVICES_ACCOUNT` | 400 |
| `PERMISSION` | `CONNECTED_SERVICES_ACCOUNT` | 400 |
| `SUBSCRIPTION` | `CONNECTED_SERVICES_ACCOUNT` | 400 |
| `VEHICLE_MISSING` | `CONNECTED_SERVICES_ACCOUNT` | 400 |
| `VIRTUAL_KEY_REQUIRED` | `CONNECTED_SERVICES_ACCOUNT` | 400 |
| `null` | `VALIDATION` | 400 |
| `PARAMETER` | `VALIDATION` | 400 |
| `null` | `AUTHENTICATION` | 401 |
| `null` | `PERMISSION` | 403 |
| `PATH` | `RESOURCE_NOT_FOUND` | 404 |
| `VERSION` | `RESOURCE_NOT_FOUND` | 404 |
| `ASLEEP` | `VEHICLE_STATE` | 409 |
| `CHARGING_IN_PROGRESS` | `VEHICLE_STATE` | 409 |
| `CHARGE_FAULT` | `VEHICLE_STATE` | 409 |
| `NOT_CHARGING` | `VEHICLE_STATE` | 409 |
| `CHARGING_PLUG_NOT_CONNECTED` | `VEHICLE_STATE` | 409 |
| `CHARGING_PLUG_CONNECTED` | `VEHICLE_STATE` | 409 |
| `DOOR_OPEN` | `VEHICLE_STATE` | 409 |
| `FULLY_CHARGED` | `VEHICLE_STATE` | 409 |
| `HOOD_OPEN` | `VEHICLE_STATE` | 409 |
| `IGNITION_ON` | `VEHICLE_STATE` | 409 |
| `IN_MOTION` | `VEHICLE_STATE` | 409 |
| `LOW_BATTERY` | `VEHICLE_STATE` | 409 |
| `REMOTE_ACCESS_DISABLED` | `VEHICLE_STATE` | 409 |
| `TRUNK_OPEN` | `VEHICLE_STATE` | 409 |
| `UNKNOWN` | `VEHICLE_STATE` | 409 |
| `UNREACHABLE` | `VEHICLE_STATE` | 409 |
| `VEHICLE_OFFLINE_FOR_SERVICE` | `VEHICLE_STATE` | 409 |
| `VEHICLE` | `RATE_LIMIT` | 429 |
| `SMARTCAR_API` | `RATE_LIMIT` | 429 |
| `INVALID_PLAN` | `BILLING` | 430 |
| `VEHICLE_LIMIT` | `BILLING` | 430 |
| `VEHICLE_REQUEST_LIMIT` | `BILLING` | 430 |
| `ACCOUNT_SUSPENDED` | `BILLING` | 430 |
| `INTERNAL` | `SERVER` | 500 |
| `INTERNAL` | `MULTIPLE_RECORDS_FOUND` | 500 |
| `INTERNAL` | `RECORD_NOT_FOUND` | 500 |
| `MAKE_NOT_COMPATIBLE` | `COMPATIBILITY` | 501 |
| `SMARTCAR_NOT_CAPABLE` | `COMPATIBILITY` | 501 |
| `VEHICLE_NOT_CAPABLE` | `COMPATIBILITY` | 501 |
| `PLATFORM_NOT_CAPABLE` | `COMPATIBILITY` | 501 |
| `INVALID_DATA` | `UPSTREAM` | 502 |
| `KNOWN_ISSUE` | `UPSTREAM` | 502 |
| `NO_RESPONSE` | `UPSTREAM` | 502 |
| `RATE_LIMIT` | `UPSTREAM` | 502 |
| `UNKNOWN_ISSUE` | `UPSTREAM` | 502 |
# SDKs
Source: https://smartcar.com/docs/api-reference/api-sdks
Our backend SDKs simplify the process of making calls to our API.
While we provide a number of SDKs for popular languages, you do not need to use an SDK to integrate with our API.
Our APIs are just standard HTTP endpoints that can be reached with any HTTP library of your choice.
# Permissions
Source: https://smartcar.com/docs/api-reference/application-permissions
GET /vehicles/{id}/permissions
Returns a list of the permissions that have been granted to your application in relation to this vehicle.
## Request
The vehicle id.
The number of vehicles to return per page. `Max: 50`
The index to start vehicle list at.
## Response
An array of [permissions](/api-reference/permissions).
Metadata about the current list of elements.
The total number of elements for the entire query (not just the given page).
The current start index of the returned list of elements.
```json Example Response
{
"paging": {
"count": 25,
"offset": 10
},
"permissions": [
"read_vehicle_info"
]
}
```
# Audi: Charge Status
Source: https://smartcar.com/docs/api-reference/audi/get-charge
GET /vehicles/{id}/{make}/charge
Returns all charging related data for an Audi vehicle.
## Permission
`read_charge`
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/charge" \
-H "Authorization: Bearer {token}" \
-X "GET"
```
```python Python
charge = vehicle.request(
"GET",
"{make}/charge"
)
```
```js Node
const charge = await vehicle.request(
"GET",
"{make}/charge"
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("GET")
.path("{make}/charge")
.build();
VehicleResponse charge = vehicle.request(request);
```
```ruby Ruby
charge = vehicle.request(
"GET",
"{make}/charge"
)
```
## Request
**Path**
## Response
Indicates the charging status of the vehicle
Indicates if the vehicle is plugged in
The instant power measured by the vehicle (in kilowatts).
The rate of range added in the charging session (in kilometers added / hour).
Indicates the type of charger.
The indicator light color of the connector.
Indicates if the charge port latch status.
An ISO8601 formatted datetime (YYYY-MM-DDTHH:mm:ss.SSSZ) for the time at which the vehicle expects to complete this charging session.
Indicates if the vehicle is set to charge on a timer. One of `manual` or `timer`.
Indicates the level at which the vehicle will stop charging and be considered fully charged as a percentage.
When plugged in indicates if the charging station is able to provide power to the vehicle.
```json Example Response
{
"chargingStatus": "CHARGING",
"isPluggedIn": null,
"chargeRate": 21,
"chargeType": "ac",
"chargePortColor": "green",
"chargePortLatch": "locked",
"completionTime": "2022-01-13T22:52:55.358Z",
"chargeMode": "manual",
"socLimit": 0.8,
"externalPowerStatus": "active",
"wattage" : 1.5
}
```
# Auth Code Exchange
Source: https://smartcar.com/docs/api-reference/authorization/auth-code-exchange
POST https://auth.smartcar.com/oauth/token
To interact with the Smartcar API, you will need to exchange your authorization code from the [Connect redirect](/docs/connect/handle-the-response) for an access token. See [Token Management](https://smartcar.com/docs/connect/token-management/overview) for an overview.
# Token Refresh
Source: https://smartcar.com/docs/api-reference/authorization/token-refresh
POST https://auth.smartcar.com/oauth/token
Your access token will expire 2 hours after it is issued. When this happens, your application can retrieve a new one by using the `refresh_token` returned in the [auth code exchange](/docs/api-reference/authorization/auth-code-exchange) or prior token refresh. See [Token Refresh](https://smartcar.com/docs/connect/token-management/refreshing-access-token) for additional guidance.
# Batch
Source: https://smartcar.com/docs/api-reference/batch
POST /vehicles/{id}/batch
Returns a list of responses from multiple Smartcar `GET` endpoints, all combined into a single request.
Each endpoint in the batch counts against your [request limit](/errors/api-errors/billing-errors) for a vehicle.
## Request
**Path**
**Body**
An array of requests to make.
The Smartcar endpoint to request data from.
## Response
The responses from Smartcar.
The headers for this response.
The unit system to use for the request.
The timestamp (ISO-8601 format) of when the returned data was recorded by the vehicle.
The HTTP response status code.
The Smartcar endpoint to request data from.
The response body of this request. The structure of this object will vary by endpoint. See the according endpoint specification.
```json Example Response
{
"responses": [
{
"path": "/odometer",
"body": {
"distance": 37829
},
"code": 200,
"headers": {
"sc-data-age": "2019-10-24T00:43:46.000Z",
"sc-unit-system": "metric"
}
},
{
"path": "/location",
"body": {
"latitude": 37.4292,
"longitude": 122.1381
},
"code": 200,
"headers": {
"sc-data-age": "2019-10-24T00:43:46.000Z"
}
}
]
}
```
# Charge Records
Source: https://smartcar.com/docs/api-reference/bmw/get-charge-records
GET /vehicles/{id}/{make}/charge/records
Returns data associated with completed charging sessions for a vehicle. Limited to the last 30 days or when the owner first granted your application access, which ever is shorter.
This endpoint is currently available for `bmw` and `mini`
## Permission
`read_charge_records`
## Request
**Path**
**Query**
Date of the first record to return in YYYY-MM-DD format.
Defaults to 30 days prior or when the owner first granted your application access, whichever is shorter.
Date of the final record to return in YYYY-MM-DD format. Defaults to the date of the request.
## Response
An array of charge records for the vehicle.
The start date of the charging record, formatted in ISO 8601 standard
The end date of the charging record, formatted in ISO 8601 standard
Location of the charge session.
The amount of energy consumed during the charging session.
Indicates whether the charging location is public or not.
The remaining battery soc at the start of the charging session.
The remaining battery soc at the end of the charging session.
The type of charger used for the session.
```json Example Response
{
"records": [
{
"chargeStart" : "2023-03-08T12:54:44Z",
"chargeEnd" : "2023-03-08T14:14:44Z",
"location": "Cockfosters Road, Barnet, EN4 0",
"energy" : 34.22998046875,
"isPublic" : true,
"startPercentRemaining" : 0.57,
"endPercentRemaining" : 1,
"chargingType" : "DC"
}
]
}
```
# By Region and Make
Source: https://smartcar.com/docs/api-reference/compatibility/by-region-and-make
GET /compatibility/matrix
Compatibility will vary by model, year, and trim. This API is for reference purposes only and it showcases vehicle makes and models that may be compatible with Smartcar and it does not guarantee that a specific vehicle will be compatible.
The Compatibility API is an Enterprise feature. Please [contact us](https://smartcar.com/pricing/) to upgrade your plan.
Compatibility by region and make allows developers to query the latest version of our Compatibility Matrix based on region, engine type, make, and permission.
## Request
**Headers**
**Query**
One of the following regions: `US`, `CA` or `EUROPE`
Queries for all engine types if none are specified.
A space-separated list of makes. Valid makes for a given region can be found in the makes section. This field is optional. If no make is specified, all makes will be returned.
A space-separated list of [permissions](/api-reference/permissions).
Queries for all permissions if none are specified.
## Response
An array of models supported for the given `make`.
`model` for the given `make`.
The earliest model year supported by Smartcar.
The latest model year supported by Smartcar.
Engine type for the given `model`.
An array of endpoints supported for the given `model`.
An array of `permissions` supported for the given `model`.
```json Example Response
{
"NISSAN": [
{
"model": "LEAF",
"startYear": 2018,
"endYear": 2022,
"type": "BEV",
"endpoints": [
"EV battery",
"EV charging status",
"Location",
"Lock & unlock",
"Odometer"
],
"permissions": [
"read_battery",
"read_charge",
"read_location",
"control_security",
"read_odometer"
]
}
],
"TESLA": [
{
"model": "3",
"startYear": 2017,
"endYear": 2023,
"type": "BEV",
"endpoints": [
"EV battery",
"EV charging status",
"EV start & stop charge",
"Location",
"Lock & unlock",
"Odometer",
"Tire pressure"
],
"permissions": [
"read_battery",
"read_charge",
"control_charge",
"read_location",
"control_security",
"read_odometer",
"read_tires"
]
}
]
}
```
# By VIN
Source: https://smartcar.com/docs/api-reference/compatibility/by-vin
GET /compatibility
Compatibility will vary by model, year, and trim. This API is for reference purposes only and it showcases vehicle makes and models that may be compatible with Smartcar and it does not guarantee that a specific vehicle will be compatible.
The Compatibility API is an Enterprise feature. Please [contact us](https://smartcar.com/pricing/) to upgrade your plan.
Compatibility by VIN allows developers to determine if a specific vehicle could be compatible with Smartcar.
A vehicle is capable of a given feature if:
1. The vehicle supports the feature (e.g., a Ford Escape supports /fuel but a Mustang Mach-e does not)
2. Smartcar supports the feature for the vehicle's make
This endpoint only supports checking capabilities for vehicles sold in the United States. It **does not** support checking `capabilities` for VINs in Canada and Europe.
## Request
**Headers**
**Parameters**
The VIN (Vehicle Identification Number) of the vehicle.
A space-separated list of permissions.
An optional country code string according to ISO 3166-1 alpha-2.
## Response
`true` if the vehicle is likely compatible, `false` otherwise.
One of the reasons described below if compatible is `false`, `null` otherwise
The vehicle does not have the hardware required for internet connectivity
Smartcar is not yet compatible with the vehicle's make in the specified country
An array containing capability objects for the set of endpoints that the provided scope value can provide authorization for.
This array will be empty if `compatible` is `false`.
One of the permissions provided in the scope parameter
One of the endpoints that the permission authorizes access to
`true` if the vehicle is likely capable of this feature, `false` otherwise
One of the reasons described below if capable is `false`, `null` otherwise
The vehicle does not support this feature
Smartcar is not capable of supporting the given feature on the vehicle's make
```json Example Response
{
"compatible": true,
"reason": null,
"capabilities": [
{
"capable": false,
"endpoint": "/engine/oil",
"permission": "read_engine_oil",
"reason": "SMARTCAR_NOT_CAPABLE"
},
{
"capable": true,
"endpoint": "/battery",
"permission": "read_battery",
"reason": null
},
{
"capable": true,
"endpoint": "/battery/capacity",
"permission": "read_battery",
"reason": null
},
{
"capable": true,
"endpoint": "/vin",
"permission": "read_vin",
"reason": null
}
]
}
```
A vehicle's compatibility depends on many factors such as its make, model, model year, trim, etc. The API optimizes returning false positives.
# Lock & Unlock
Source: https://smartcar.com/docs/api-reference/control-lock-unlock
POST /vehicles/{id}/security
Lock or unlock the vehicle.
## Permission
`control_security`
## Request
**Path**
**Body**
`LOCK` or `UNLOCK` the vehicle’s doors.
## Response
If the request is successful, Smartcar will return “success” (HTTP 200 status).
If the request is successful, Smartcar will return a message (HTTP 200 status).
```json Example Response
{
"message": "Successfully sent request to vehicle",
"status": "success"
}
```
# Start & Stop Charge
Source: https://smartcar.com/docs/api-reference/evs/control-charge
POST /vehicles/{id}/charge
Start or stop the vehicle charging.
## Permission
`control_charge`
## Request
**Path**
**Body**
`START` or `STOP` the vehicle charging.
## Response
If the request is successful, Smartcar will return “success”.
If the request is successful, Smartcar will return a message.
```json Example Response
{
"message": "Successfully sent request to vehicle",
"status": "success"
}
```
## Notes
**BMW and MINI**
Vehicle needs to be on OS Version 8+
**Ford and Lincoln**
Issuing a start command while the vehicle has a schedule in place for its current charging location will result in the vehicle charging to 100%.
Please see [charge schedule by location](/api-reference/ford/set-charge-schedule-by-location) for details on setting a charge limit with preferred charging times
or clearing schedules.
**Nissan**
Currently only START charge commands are supported in the US. See [Set Charge Schedule](/api-reference/nissan/set-charge-schedule) for details on setting a charge schedule for Nissan vehicles.
**Chevrolet, GMC, Buick and Cadillac**
These vehicles require a minimum charge of 50% in order to be able to start or stop charging via the API.
# Battery Level
Source: https://smartcar.com/docs/api-reference/evs/get-battery-level
GET /vehicles/{id}/battery
Returns the state of charge and the remaining range of an electric vehicle's high voltage battery.
## Permission
`read_battery`
## Request
**Path**
## Response
The EV's state of charge as a percentage.
The estimated remaining distance the vehicle can travel powered by its high voltage battery.
```json Example Response
{
"percentRemaining": 0.3,
"range": 40.5
}
```
# Charge Limit
Source: https://smartcar.com/docs/api-reference/evs/get-charge-limit
GET /vehicles/{id}/charge/limit
Returns the charge limit configuration for the vehicle.
## Permission
`read_charge`
## Request
**Path**
## Response
The level at which the vehicle will stop charging and be considered fully charged as a percentage.
```json Example Response
{
"limit": 0.8,
}
```
## Notes
This endpoint will return a [`CHARGING_PLUG_NOT_CONNECTED`](/errors/api-errors/vehicle-state-errors#charging-plug-not-connected)
error if the OEM is unable to provide a charge limit unless the vehicle is plugged in.
**Ford and Lincoln**
If a vehicle starts charing as a result of a [start charge](/api-reference/evs/control-charge) request, this endpoint will always return `1` if the charging location has a schedule in place.
For the vehicle to respect its charge limit, please set one along with preferred charge times or clear the schedule through through the [charge schedule by location](/api-reference/ford/set-charge-schedule-by-location) endpoint.
**BMW and MINI**
Vehicle needs to be on OS Version 8+
# Charge Status
Source: https://smartcar.com/docs/api-reference/evs/get-charge-status
GET /vehicles/{id}/charge
Returns the charge status for the vehicle.
## Permission
`read_charge`
## Request
**Path**
## Response
Indicates whether a charging cable is currently plugged into the vehicle’s charge port.
Returns the current charge status of a vehicle. A vehicle can be `FULLY_CHARGED` at less than 100% SoC if its [Charge Limit](/api-reference/evs/get-charge-limit) is less than `1`.
```json Example Response
{
"isPluggedIn": true,
"state": "FULLY_CHARGED"
}
```
# Charge Limit
Source: https://smartcar.com/docs/api-reference/evs/set-charge-limit
POST /vehicles/{id}/charge/limit
Set the charge limit of an electric vehicle.
## Permission
`control_charge`
## Request
**Path**
**Body**
The level at which the vehicle should stop charging and be considered fully charged.
Cannot be less than `0.5`, or greater than `1`.
## Response
If the request is successful, Smartcar will return “success” (HTTP 200 status).
If the request is successful, Smartcar will return a message (HTTP 200 status).
## Notes
This endpoint will return a [`CHARGING_PLUG_NOT_CONNECTED`](/errors/api-errors/vehicle-state-errors#charging-plug-not-connected) error if
the OEM is unable to set a charge limit while the vehicle is unpluged.
**Ford and Lincoln**
If a vehicle starts charing as a result of a [start charge](/api-reference/evs/control-charge) request it will always charge to 100%
if the charging location has a schedule in place.
For the vehicle to respect its charge limit, please set one along with preferred charge
times or clear the schedule through the [charge schedule by location](/api-reference/ford/set-charge-schedule-by-location) endpoint.
**BMW and MINI**
Vehicle needs to be on OS Version 8+
```json Example Response
{
"message": "Successfully sent request to vehicle",
"status": "success"
}
```
# Charge Schedule by Location
Source: https://smartcar.com/docs/api-reference/ford/get-charge-schedule-by-location
GET /vehicles/{id}/{make}/charge/schedule_by_location
Returns all saved charging locations for a vehicle and their associated charging limits, schedules and configurations.
## Permission
`read_charge_locations`
## Request
**Path**
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/charge/schedule_by_location" \
-H "Authorization: Bearer {token}" \
-X "GET"
```
```python Python
chargeScheduleByLocation = vehicle.request(
"GET",
"{make}/charge/schedule_by_location"
)
```
```js Node
const chargeScheduleByLocation = await vehicle.request(
"GET",
"{make}/charge/schedule_by_location"
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("GET")
.path("{make}/charge/schedule_by_location")
.build();
VehicleResponse getChargeScheduleByLocation = vehicle.request(request)
```
```ruby Ruby
chargeScheduleByLocation = vehicle.request(
"GET",
"{make}/charge/schedule_by_location"
)
```
## Response
An array of charging locations. Empty if none are currently set on the vehicle.
The maximum charge limit for the vehicle at the location as a percent.
The latitude and longitude of the charging location.
The longitude of the charging location.
The latitude of the charging location.
The weekday and weekend charging schedules for the vehicle at the location.
The charging schedule for the vehicle on weekdays (Monday - Friday).
The exact hour a vehicle should start charging in HH:00 e.g. 17:00.
The exact hour a vehicle should stop charging in HH:00 e.g. 21:00.
The charging schedule for the vehicle on weekends (Saturday - Sunday).
The exact hour a vehicle should start charging in HH:00 e.g. 17:00.
The exact hour a vehicle should stop charging in HH:00 e.g. 21:00.
```json Example Response
{
"chargingLocations": [
{
"chargeLimit": 0.8,
"chargingSchedules": {
"weekday": [
{
"end": "17:00",
"start": "09:00"
}
],
"weekend": [
{
"end": "17:00",
"start": "09:00"
}
]
},
"location": {
"latitude": 48.8566,
"longitude": 2.3522
}
}
]
}
```
## Example Schedule States
```json
{
"chargingLocations": [
{
"chargeLimit": 0.8,
"chargingSchedules": {
"weekday": [],
"weekend": []
},
"location": {
"latitude": 48.8566,
"longitude": 2.3522
}
}
]
}
```
```json
{
"chargingLocations": [
{
"chargeLimit": 0.8,
"chargingSchedules": {
"weekday": [
{
"end": "17:00",
"start": "09:00"
}
],
"weekend": []
},
"location": {
"latitude": 48.8566,
"longitude": 2.3522
}
}
]
}
```
```json
{
"chargingLocation" : {
"chargeLimit": 0.9,
"chargingSchedules": {
"weekday": [],
"weekend": [
{
"start": "16:00",
"end": "07:00"
}
]
}
}
}
```
# Charge Schedule by Location
Source: https://smartcar.com/docs/api-reference/ford/set-charge-schedule-by-location
PUT /vehicles/{id}/{make}/charge/schedule_by_location
Set all schedules for the specified charging location.
## Permission
`control_charge`
## Request
In order to set a schedule, you must use a location from the [`GET Charge Schedule by Location`](/api-reference/ford/get-charge-schedule-by-location) endpoint.
If the location you want to manage is not in the response, the vehicle owner has not charged at that location within the last 90 days and it has been
removed from their app's saved charging location list.
**Path**
**Query**
The longitude of a charging location from [`GET Charge Schedule by Location`](/api-reference/ford/get-charge-schedule-by-location)
The latitude of a charging location from [`GET Charge Schedule by Location`](/api-reference/ford/get-charge-schedule-by-location)
**Body**
The maximum charge limit for the vehicle at the location as a percent between `0.5` and `1`.
The weekday and weekend charging schedules for the vehicle at the location.
The charging schedule for the vehicle on weekdays (Monday - Friday).
The exact hour a vehicle should start charging in HH:00 e.g. 17:00.
The exact hour a vehicle should stop charging in HH:00 e.g. 21:00.
The charging schedule for the vehicle on weekends (Saturday - Sunday).
The exact hour a vehicle should start charging in HH:00 e.g. 17:00.
The exact hour a vehicle should stop charging in HH:00 e.g. 21:00.
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/charge/schedule_by_location?longitude=2.3522&latitude=48.8566" \
-H "Authorization: Bearer {token}" \
-X "PUT" \
-H "Content-Type: application/json" \
-d '{chargingLocation:{"chargeLimit": 0.9, "chargingWindows": {"weekday": [{"start": "09:00", "end": "17:00"}], "weekend": [{"start": "09:00", "end": "17:00"}]}}}'
```
```python Python
setChargeScheduleByLocation = vehicle.request(
"PUT",
"{make}/charge/schedule_by_location?longitude=2.3522&latitude=48.8566",
{
"chargingLocation" : {
"chargeLimit": 0.9,
"chargingWindows": {
"weekday": [
{
"start": "09:00",
"end": "10:00"
}
],
"weekend": [
{
"start": "09:00",
"end": "10:00"
}
]
}
}
}
)
```
```js Node
const setChargeScheduleByLocation = vehicle.request(
"PUT",
"{make}/charge/schedule_by_location?longitude=2.3522&latitude=48.8566",
{
"chargingLocation" : {
"chargeLimit": 0.9,
"chargingWindows": {
"weekday": [
{
"start": "09:00",
"end": "10:00"
}
],
"weekend": [
{
"start": "09:00",
"end": "10:00"
}
]
}
}
}
);
```
```java Java
JsonArrayBuilder weekday = Json.createArrayBuilder().add(
Json.createObjectBuilder().add("start", "05:00").add("end", "07:00"));
JsonArrayBuilder weekend = Json.createArrayBuilder().add(
Json.createObjectBuilder().add("start", "06:00").add("end", "07:00"));
JsonObject chargingWindows = Json.createObjectBuilder()
.add("weekday", weekday)
.add("weekend", weekend)
.build();
JsonObject chargingLocation = Json.createObjectBuilder()
.add("chargeLimit", 1.0)
.add("chargingWindows", chargingWindows)
.build();
SmartcarVehicleRequest request =
new SmartcarVehicleRequest.Builder()
.method("PUT")
.path("ford/charge/schedule_by_location")
.addQueryParameter("latitude", "37.749008")
.addQueryParameter("longitude", "-122.46981")
.addBodyParameter("chargingLocation", chargingLocation)
.build();
VehicleResponse chargeSched = vehicle.request(request);
```
```ruby Ruby
setChargeScheduleByLocation = vehicle.request(
"PUT",
"{make}/charge/schedule_by_location?longitude=2.3522&latitude=48.8566",
{
"chargingLocation" : {
"chargeLimit": 0.9,
"chargingWindows": {
"weekday": [
{
"start": "09:00",
"end": "10:00"
}
],
"weekend": [
{
"start": "09:00",
"end": "10:00"
}
]
}
}
}
)
```
## Response
If the request is successful, Smartcar will return a message.
If the request is successful, Smartcar will return `success`.
```json Example Response
{
"message": "Successfully sent request to vehicle",
"status": "success"
}
```
## Example Request Bodies
```json
{
"chargingLocation" : {
"chargeLimit": 0.9,
"chargingWindows": {
"weekday": [
{
"start": "17:00",
"end": "06:00"
}
],
"weekend": [
{
"start": "16:00",
"end": "07:00"
}
]
}
}
}
```
```json
{
"chargingLocation" : {
"chargeLimit": 0.9,
"chargingWindows": {
"weekday": [
{
"start": "17:00",
"end": "06:00"
}
],
"weekend": []
}
}
}
```
```json
{
"chargingLocation" : {
"chargeLimit": 0.9,
"chargingWindows": {
"weekday": [],
"weekend": [
{
"start": "16:00",
"end": "07:00"
}
]
}
}
}
```
```json
{
"chargingLocation" : {
"chargeLimit": 0.9,
"chargingWindows": {
"weekday": [],
"weekend": []
}
}
}
```
# Diagnostic Trouble Codes
Source: https://smartcar.com/docs/api-reference/get-dtcs
GET /vehicles/{id}/diagnostics/dtcs
Provides a list of active Diagnostic Trouble Codes (DTCs) reported by the vehicle. Currently supporting GM brands including Chevrolet and GMC.
## Permission
`read_diagnostics`
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/diagnostics/dtcs" \\
-H "Authorization: Bearer {token}" \\
-X "GET"
```
```python Python
diagnostic_trouble_codes = vehicle.diagnostic_trouble_codes()
```
```js Node
const diagnosticTroubleCodes = await vehicle.diagnosticTroubleCodes();
```
```java Java
VehicleDiagnosticTroubleCodes diagnosticTroubleCodes = vehicle.diagnosticTroubleCodes();
```
```ruby Ruby
diagnostic_trouble_codes = vehicle.diagnostic_trouble_codes
```
## Request
**Path**
## Response
The Diagnostic Trouble Code reported by the vehicle.
The date and time the troublecode last became active.
```json Example Response
{
"activeCodes": [
{
"code": "P302D",
"timestamp": "2024-09-05T14:48:00.000Z"
},
{
"code": "xxxxx",
"timestamp": null
},
...
]
}
```
# Oil Life
Source: https://smartcar.com/docs/api-reference/get-engine-oil-life
GET /vehicles/{id}/engine/oil
Returns the remaining life span of a vehicle’s engine oil.
## Permission
`read_engine_oil`
## Request
**Path**
## Response
The engine oil’s remaining life span based on the current quality of the oil as a percentage.
`1` indicates the oil was changed recently and `0` indicates the oil should be changed immediately.
It is not a representation of how much oil is left in the vehicle.
```json Example Response
{
"lifeRemaining": 0.35
}
```
# Fuel Tank
Source: https://smartcar.com/docs/api-reference/get-fuel-tank
GET /vehicles/{id}/fuel
Returns the status of the fuel remaining in the vehicle’s fuel tank.
## Permission
`read_fuel`
This endpoint may return `null` values for vehicles sold in Europe. Please see the [Notes](/api-reference/get-fuel-tank#notes) section for details.
## Request
**Path**
## Response
The remaining level of fuel in the tank as a percentage.
The amount of fuel in the tank.
The estimated remaining distance the car can travel.
```json Example Response
{
"amountRemaining": 53.2,
"percentRemaining": 0.3,
"range": 40.5
}
```
## Notes
The table below indicates values Smartcar attempts to retrieve from vehicles sold in Europe.
| | `range` | `percentRemaining` | `amountRemaining` |
| ------------------------------------ | ------- | ------------------ | ----------------- |
| Audi | ✅ | ✅ | |
| BMW, MINI | ✅ | ✅ | ✅ |
| Citroen, DS, Opel, Peugeot, Vauxhall | ✅ | | ✅ |
| Ford | ✅ | ✅ | |
| Hyundai | ✅ | | ✅ |
| Jaguar, Land Rover | ✅ | ✅ | |
| Kia | ✅ | ✅ | |
| Mazda | ✅ | ✅ | |
| Mercedes | ✅ | ✅ | |
| Renault | ✅ | | ✅ |
| Skoda, Volkswagen | ✅ | ✅ | |
| Volvo | ✅ | ✅ | ✅ |
# Location
Source: https://smartcar.com/docs/api-reference/get-location
GET /vehicles/{id}/location
Returns the vehicle's last known location.
## Permission
`read_location`
## Request
**Path**
## Response
The latitude in degrees.
The longitude in degrees.
```json Example Response
{
"latitude": 37.4292,
"longitude": 122.1381
}
```
# Lock Status
Source: https://smartcar.com/docs/api-reference/get-lock-status
GET /vehicles/{id}/security
Returns the lock status for a vehicle and the open status of its doors, windows, storage units, sunroof and charging port where available.
## Permission
`read_security`
## Request
**Path**
## Response
Indicates the current lock status of the vehicle as reported by the OEM.
An array of the open status of the vehicle's doors.
The location of the door.
Indicates the current state of the vehicle's door.
`UNKNOWN` indicates the vehicle supports this status, but did not provide a valid status for the request
An array of the open status of the vehicle's windows.
The location of the window.
Indicates the current state of the vehicle's window.
`UNKNOWN` indicates the vehicle supports this status, but did not provide a valid status for the request
An array of the open status of the vehicle's sunroofs.
The location of the sunroof.
Indicates the current state of the vehicle's sunroof.
`UNKNOWN` indicates the vehicle supports this status, but did not provide a valid status for the request
An array of the open status of the vehicle's storages.
For internal combustion and plug-in hybrid vehicles front refers to the engine hood.
For battery vehicles, this will be the front trunk.
The location of the sunroof.
Indicates the current state of the vehicle's storage.
`UNKNOWN` indicates the vehicle supports this status, but did not provide a valid status for the request
An array of the open status of the vehicle's charging port.
The location of the charging port.
Indicates the current state of the vehicle's charging port.
`UNKNOWN` indicates the vehicle supports this status, but did not provide a valid status for the request
```json Example Response
{
"isLocked" : false,
"doors" : [
{"type": "frontLeft" , "status" : "CLOSED"},
{"type": "frontRight" , "status" : "OPEN"},
{"type": "backRight" , "status" : "CLOSED"},
{"type": "backLeft" , "status" : "CLOSED"}
],
"windows" : [
{"type": "frontLeft" , "status" : "CLOSED"},
{"type": "frontRight" , "status" : "CLOSED"},
{"type": "backRight" , "status" : "UNKNOWN"},
{"type": "backLeft" , "status" : "CLOSED"}
],
"sunroof" : [
{"type": "sunroof" , "status" : "CLOSED"}
],
"storage" : [
{"type": "rear" , "status" : "UNKNOWN"},
{"type": "front" , "status" : "CLOSED"}
],
"chargingPort" : [
{"type" : "chargingPort", "status" : "CLOSED" }
]
}
```
# Notes
* The open status array(s) will be empty if a vehicle has partial support.
* The request will error if `lockStatus` can not be retrieved from the vehicle or the brand is not supported.
# Battery Capacity
Source: https://smartcar.com/docs/api-reference/get-nominal-capacity
GET /vehicles/{id}/battery/nominal_capacity
Returns a list of nominal rated battery capacities for a vehicle.
This endpoint is only available for US and European based vehicles. Please refer to [this page](/api-reference/evs/get-battery-capacity) if you need battery capacity for Canadian based vehicles for the time being.
## Permission
`read_battery`
## Request
**Path**
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/battery/nominal_capacity" \
-H "Authorization: Bearer {token}" \
-X "GET"
```
## Response
An array of capacites Objects.
The rated nominal capacity for the vehicle's battery.
A a description of the uniquness for the nominal capacity and engine
type of the vehicle in the form `{ENGINE_TYPE}:{TRIM}`, for example `"BEV:Extended Range"`.
Engine type can be one of `BEV` or `PHEV`.
The rated nominal capacity for the vehicle's battery.
Indicates if this capacity was determined by a user or Smartcar.
Options are `USER_SELECTED` or `SMARTCAR`.
A URL that will launch the flow for a vehicle owner to specify the correct
battery capacity for a vehicle. **Please to ensure you append a
redirect URI** for us to send a response to once the user exits the flow.
Please see [this article](/connect/user-selected-batcap) on how to handle the URL callback.
```json Response Example
{
"availableCapacities": [
{
"capacity" : 70.9,
"description" : null
},
{
"capacity" : 80.9,
"description" : null
},
{
"capacity" : 90.9,
"description" : "BEV:Extended Range"
}
],
"capacity": {
"nominal" : 80.9,
"source": "USER_SELECTED"
},
"url" : "https://connect.smartcar.com/battery-capacity?vehicle_id=36ab27d0-fd9d-4455-823a-ce30af709ffc&client_id=8229df9f-91a0-4ff0-a1ae-a1f38ee24d07&token=90abecb6-e7ab-4b85-864a-e1c8bf67f2ad&response_type=vehicle_id&redirect_uri="
}
```
### Case 1: Smartcar has determined the battery capacity
```json Single capacity
{
"availableCapacities": [
{
"capacity" : 70.9,
"description" : null
}
],
"capacity": {
"nominal" : 70.9,
"source": "SMARTCAR"
},
"url" : null
}
```
### Case 2: Unable to determine the battery capacity
```json Multiple capacites
{
"availableCapacities": [
{
"capacity" : 70.9,
"description" : null
},
{
"capacity" : 80.9,
"description" : null
},
{
"capacity" : 90.9,
"description" : "BEV:Extended Range"
}
],
"capacity": null,
"url" : "https://connect.smartcar.com/battery-capacity?vehicle_id=36ab27d0-fd9d-4455-823a-ce30af709ffc&client_id=8229df9f-91a0-4ff0-a1ae-a1f38ee24d07&token=90abecb6-e7ab-4b85-864a-e1c8bf67f2ad&response_type=vehicle_id&redirect_uri="
}
```
### Case 3: User selected battery capacity
Smartcar will sometimes return an `availableCapacities` object along side a Connect URL you can use to prompt users to select the battery capacity of their vehicle. This can occur when an accurate match could not be found, or vehicle owners purchased extension packs, or software upgrades specific to their vehicle.
When you recirect a vehicle owner to this Smartcar Connect url, they can select the battery capacity of their vehicle for cases where the battery capacity cannot be accurately deteremined.
When a user selects an option, Smartcar will return this value with `USER_SELECTED` as the source.
```json Multiple capacites
{
"availableCapacities": [
{
"capacity" : 70.9,
"description" : null
},
{
"capacity" : 80.9,
"description" : null
},
{
"capacity" : 90.9,
"description" : "BEV:Extended Range"
}
],
"capacity": {
"nominal" : 80.9,
"source": "USER_SELECTED"
},
"url" : "https://connect.smartcar.com/battery-capacity?vehicle_id=36ab27d0-fd9d-4455-823a-ce30af709ffc&client_id=8229df9f-91a0-4ff0-a1ae-a1f38ee24d07&token=90abecb6-e7ab-4b85-864a-e1c8bf67f2ad&response_type=vehicle_id&redirect_uri="
}
```
# Odometer
Source: https://smartcar.com/docs/api-reference/get-odometer
GET /vehicles/{id}/odometer
Returns the vehicle’s last known odometer reading.
## Permission
`read_odometer`
## Request
**Path**
## Response
The current odometer of the vehicle.
```json Example Response
{
"distance": 104.32
}
```
# Service History
Source: https://smartcar.com/docs/api-reference/get-service-records
GET /vehicles/{id}/service/history
Retrieve service records tracked by the vehicle's dealer or manually added by the vehicle owner. Currently supporting Ford, Lincoln, Toyota, Lexus, Mazda and Volkswagen (US)
## Permission
`read_service_history`
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/service/history \
-H "Authorization: Bearer {token}" \
-X "GET"
```
```python Python
service = vehicle.request(
"GET",
"service/history"
)
```
```js Node
const service = await vehicle.request(
"GET",
"service/history"
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("GET")
.path("service/history")
.build();
VehicleResponse service =vehicle.request(request);
```
```ruby Ruby
Service = vehicle.request(
"GET",
"service/history"
)
```
## Request
**Path**
## Response
A unique identifier of the service record.
The date and time the vehicle was serviced
the odometer of the vehicle at time of service in kilometers.
The unique identifier of the tasks completed as part of the service event.
Tasks completed as part of the service event. Note that not all makes provide service tasks.
Additional service details.
Indicates if the service event was completed by a dealership or manually entered by the vehicle owner.
An overview of service costs.
The total cost of the service event. Note that not all makes provide service cost.
Identifies the currency used for service cost.
```json Example Response
{
"serviceId": null,
"odometerDistance": 46047.22,
"serviceDate": "2023-06-28T22:21:41.583Z",
"serviceTasks": [
{
"taskId": "3262",
"taskDescription": "Service Task 0"
},
{
"taskId": "3041",
"taskDescription": null
},
{
"taskId": null,
"taskDescription": null
}
],
"serviceDetails": [
{
"type": "Service Details Type 0",
"value": "Service Details Value 0"
},
{
"type": "Service Details Type 1",
"value": null
}
],
"serviceCost": {
"totalCost": null,
"currency": null
}
}
```
# System Status
Source: https://smartcar.com/docs/api-reference/get-system-status
GET /vehicles/{id}/diagnostics/system_status
Provides a list of vehicle systems and their current health status. Currently supporting FCA and GM brands including RAM, Jeep, Chrysler, Dodge, Fiat, Alpha Romeo, Buick, Cadillac, Chevrolet and GMC. See [Diagnostic Systems](/docs/help/diagnostic-systems) for a complete list of Smartcar System IDs.
## Permission
`read_diagnostics`
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/diagnostics/system_status" \\
-H "Authorization: Bearer {token}" \\
-X "GET"
```
```python Python
diagnostic_system_status = vehicle.diagnostic_system_status()
```
```js Node
const diagnosticSystemStatus = await vehicle.diagnosticSystemStatus();
```
```java Java
VehicleDiagnosticSystemStatus diagnosticSystemStatus = vehicle.diagnosticSystemStatus();
```
```ruby Ruby
diagnostic_system_status = vehicle.diagnostic_system_status
```
## Request
**Path**
## Response
An overview of systems reported by the vehicle.
The unique identifier of a system reported by the vehicle.
The status of a vehicle, expected as "OK" or "ALERT".
A plain-text description of the status, if available.
```json Example Response
{
"systems": [
{
// System ID from Smartcar unified system definition list
"systemId": "SYSTEM_TPMS",
"status": "ALERT",
"description": "Left rear tire sensor battery low"
},
{
"systemId": "SYSTEM_AIRBAG",
"status": "OK",
"description": null
},
{
"systemId": "SYSTEM_MIL",
"status": "OK",
"description": null
},
...
]
}
```
# Tire Pressure
Source: https://smartcar.com/docs/api-reference/get-tire-pressure
GET /vehicles/{id}/tires/pressure
Returns the air pressure of each of the vehicle’s tires.
## Permission
`read_tires`
## Request
**Path**
## Response
The current air pressure of the front left tire.
The current air pressure of the front right tire.
The current air pressure of the back left tire.
The current air pressure of the back right tire.
```json Example Response
{
"backLeft": 219.3,
"backRight": 219.3,
"frontLeft": 219.3,
"frontRight": 219.3
}
```
# Vehicle Attributes
Source: https://smartcar.com/docs/api-reference/get-vehicle-info
GET /vehicles/{id}
Returns a single vehicle object, containing identifying information.
## Permission
`read_vehicle_info`
## Request
**Path**
## Response
The ID for the vehicle.
The manufacturer of the vehicle.
The model of the vehicle.
The model year.
```json Example Response
{
"id": "36ab27d0-fd9d-4455-823a-ce30af709ffc",
"make": "TESLA",
"model": "Model S",
"year": "2014"
}
```
# VIN
Source: https://smartcar.com/docs/api-reference/get-vin
GET /vehicles/{id}/vin
Returns the vehicle’s manufacturer identifier.
## Permission
`read_vin`
## Request
**Path**
## Response
The manufacturer unique identifier.
```json Example Response
{
"vin": "5YJSA1CN5DFP00101"
}
```
# Charge Completion Time
Source: https://smartcar.com/docs/api-reference/gm/get-charge-completion-time
GET /vehicles/{id}/{make}/charge/completion
When the vehicle is charging, returns the date and time the vehicle expects to "complete" this charging session. When the vehicle is not charging, this endpoint results in a vehicle state error.
This endpoint is currently available for `cadillac` and `chevrolet`.
## Permission
`read_charge`
## Request
`vehicle_id` of the vehicle you are making the request to.
The make to pass in the URL.
## Response
An ISO8601 formatted datetime (`YYYY-MM-DDTHH:mm:ss.SSSZ`) for the time at which the vehicle expects to complete this charging session.
```json Example Response
{
"time": "2022-01-13T22:52:55.358Z"
}
```
# Voltage
Source: https://smartcar.com/docs/api-reference/gm/get-charge-voltmeter
GET /vehicles/{id}/{make}/charge/voltmeter
When the vehicle is plugged in, returns the charging voltage measured by the vehicle. When the vehicle is not plugged in, this endpoint results in a vehicle state error.
This endpoint is currently available for `cadillac` and `chevrolet`.
## Permission
`read_charge`
## Request
## Response
The potential difference measured by the vehicle in volts (V).
```json Example Response
{
"voltage": 240
}
```
# Headers
Source: https://smartcar.com/docs/api-reference/headers
Smartcar uses the following headers for requests and responses.
## Request
Smartcar supports both `metric` and `imperial` unit systems of measurement.
```curl Request Headers
SC-Unit-System: metric
```
## Response
Indicates the timestamp (ISO-8601 format) of when the returned data was recorded by the vehicle.
Indicates the timestamp (ISO-8601 format) of when Smartcar fetched the data from the OEM.
Indicates whether the unit system used in the returned data is `imperial` or `metric`.
Each response from Smartcar’s API has a unique request identifier. If you need to contact us about a specific request, providing the request identifier will ensure the fastest possible resolution.
```curl Response Headers
SC-Data-Age: 2018-06-20T01:33:37.078Z
SC-Fetched-At: 2018-06-20T01:48:37.078Z
SC-Unit-System: metric
SC-Request-Id: 26c14915-0c26-43c5-8e42-9edfc2a66a0f
```
# Smartcar API Reference
Source: https://smartcar.com/docs/api-reference/intro
Welcome to Smartcar! Here you’ll find everything you need to get started with Smartcar.
If you have any questions, please email us at [support@smartcar.com](mailto:support@smartcar.com)!
Smartcar makes it easy to read vehicle data and send commands to vehicles of any brand using HTTP
requests. All requests made to our API require an access token. Our API uses the OAuth2 authorization framework
and provides a granular permissioning system that authorizes API endpoints based on user preferences.
This allows applications to easily authorize and interact with vehicles through
the Smartcar API.
Easily connect a vehicle and make API requests using our starter app.
Get a feel for the API using our Postman collection.
# Makes
Source: https://smartcar.com/docs/api-reference/makes
Valid values for the `make` parameter
#### United States
| | | | | | | |
| ---------- | ----------- | ---------- | --------------- | --------- | ------------ | - |
| `ACURA` | `CHEVROLET` | `HYUNDAI` | `LAND_ROVER` | `MINI` | `SUBARU` | |
| `AUDI` | `CHRYSLER` | `INFINITI` | `LEXUS` | `NISSAN` | `TESLA` | |
| `BMW` | `DODGE` | `JAGUAR` | `LINCOLN` | `PORSCHE` | `TOYOTA` | |
| `BUICK` | `FORD` | `JEEP` | `MAZDA` | `RAM` | `VOLKSWAGEN` | |
| `CADILLAC` | `GMC` | `KIA` | `MERCEDES_BENZ` | `RIVIAN` | `VOLVO` | |
#### Europe
| | | | | |
| ------------ | --------- | --------------- | --------- | ------------ |
| `AUDI` | `DS` | `KIA` | `NISSAN` | `SKODA` |
| `ALFA_ROMEO` | `FIAT` | `LAND_ROVER` | `OPEL` | `TESLA` |
| `BMW` | `FORD` | `MAZDA` | `PEUGEOT` | `VAUXHALL` |
| `CITROEN` | `JAGUAR` | `MERCEDES_BENZ` | `PORSCHE` | `VOLKSWAGEN` |
| `CUPRA` | `HYUNDAI` | `MINI` | `RENAULT` | `VOLVO` |
See [this](https://smartcar.com/global/) page for supported countries.
#### Canada
| | | | | |
| ---------- | ----------- | ------------ | --------- | -------- |
| `ACURA` | `CHEVROLET` | `HYUNDAI` | `LEXUS` | `TOYOTA` |
| `AUDI` | `CHRYSLER` | `JAGUAR` | `LINCOLN` | `VOLVO` |
| `BMW` | `DODGE` | `JEEP` | `MINI` | |
| `BUICK` | `FORD` | `KIA` | `RAM` | |
| `CADILLAC` | `GMC` | `LAND_ROVER` | `TESLA` | |
# Vehicle Connections
Source: https://smartcar.com/docs/api-reference/management/delete-vehicle-connections
DELETE https://management.smartcar.com/v2.0/management/connections
Deletes all vehicle connections associated with a Smartcar user ID or a specific vehicle.
## Request
**Header**
In the format `Basic base64(default:{application_management_token})`. You can find your `application_management_token` under
your Application Configuration in the Smartcar Dashboard.
**Query**
Delete a connection for the given `vehicle_id` or all vehicle connections
for the given `user_id`.
## Response
An array of connections
ID of the user that authorized the deleted connection (UUID v4)
ID of the vehicle disconnected (UUID v4)
# Vehicle Connections
Source: https://smartcar.com/docs/api-reference/management/get-vehicle-connections
GET https://management.smartcar.com/v2.0/management/connections
Returns a paged list of all vehicles that are connected to the application associated with the management API token used, sorted in descending order by connection date.
## Request
**Header**
In the format `Basic base64(default:{application_management_token})`. You can find your `application_management_token` under
your Application Configuration in the Smartcar Dashboard.
**Query**
Number of connections to return per page. `Max: 100`
Used for accessing pages other than the first page. Each page returned has a cursor value
that can be passed here to fetch the “next” page.
Filter for connections created by the provider user ID.
Filter for connections to the provided vehicle ID.
Filter for connections by either `live`, `simulated`, or the deprecated `test` mode.
## Response
An array of connections
ID of the user that authorized this connection (UUID v4).
ID of the vehicle connected (UUID v4).
Time at which the connection was created in UTC.
Vehicle mode: live, simulated, or test (deprecated).
Metadata about the current query
The cursor parameter that should be used to fetch the next page of results.
This field will be null if there are no more pages.
# Charge Schedule
Source: https://smartcar.com/docs/api-reference/nissan/get-charge-schedule
GET /vehicles/{id}/{make}/charge/schedule
Returns the charging schedule of a vehicle. The response contains the start time and departure time of the vehicle's charging schedule.
This endpoint is currently available for `nissan` EVs on the `MyNISSAN` platform.
## Permission
`read_charge`
## Request
**Path**
## Response
An array of charge schedules. Maximum of 3 schedules, empty if no schedules are set.
HH:mm in UTC for a schedule start time.
HH:mm in UTC for a schedule end time.
An array of days the schedule applies to.
```json Example Response
{
"chargeSchedules": [
{
"end": "2020-01-01T02:00:00.000Z",
"start": "2020-01-01T01:00:00.000Z",
"days": [
"MONDAY",
"WEDNESDAY",
"FRIDAY"
]
}
]
}
```
# Charge Schedule
Source: https://smartcar.com/docs/api-reference/nissan/set-charge-schedule
PUT /vehicles/{id}/{make}/charge/schedule
Sets the charging schedule for a vehicle.
This endpoint is currently available for `nissan` EVs on the `MyNISSAN` platform
## Permission
`control_charge`
## Request
**Path**
**Body**
An array of charge schedules. A maximum of 3 schedules can be set.
HH:mm in UTC for a schedule start time.
HH:mm in UTC for a schedule start time.
An array of days for the schedule to be applied.
Options: `MONDAY` `TUESDAY` `WEDNESDAY` `THURSDAY` `FRIDAY` `SATURDAY` `SUNDAY`
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/charge/schedule" \
-H "Authorization: Bearer {token}" \
-X "PUT" \
-H "Content-Type: application/json" \
-d '{
"chargeSchedules": [
{
"start": "08:00",
"end": "12:00",
"days": ["MONDAY", "WEDNESDAY", "FRIDAY"]
},
{
"start": "14:00",
"end": "18:00",
"days": ["TUESDAY", "THURSDAY", "SATURDAY"]
}
]
}'
```
```python Python
charge_schedule = vehicle.request("PUT", "{make}/charge/schedule", {
"chargeSchedules": [
{
"start": "08:00",
"end": "12:00",
"days": ["MONDAY", "WEDNESDAY", "FRIDAY"]
},
{
"start": "14:00",
"end": "18:00",
"days": ["TUESDAY", "THURSDAY", "SATURDAY"]
}
]
})
```
```js Node
const chargeSchedule = vehicle.request("PUT", "{make}/charge/schedule", {
"chargeSchedules": [
{
"start": "08:00",
"end": "12:00",
"days": ["MONDAY", "WEDNESDAY", "FRIDAY"]
},
{
"start": "14:00",
"end": "18:00",
"days": ["TUESDAY", "THURSDAY", "SATURDAY"]
}
]
});
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("PUT")
.path("{make}/charge/schedule")
.addBodyParameter("chargeSchedules", [
{
"start": "08:00",
"end": "12:00",
"days": ["MONDAY", "WEDNESDAY", "FRIDAY"]
},
{
"start": "14:00",
"end": "18:00",
"days": ["TUESDAY", "THURSDAY", "SATURDAY"]
}
])
.build();
ChargeSchedule chargeSchedule = vehicle.request(request);
```
```ruby Ruby
charge_schedule = vehicle.request("PUT", "{make}/charge/schedule", {
"chargeSchedules": [
{
"start": "08:00",
"end": "12:00",
"days": ["MONDAY", "WEDNESDAY", "FRIDAY"]
},
{
"start": "14:00",
"end": "18:00",
"days": ["TUESDAY", "THURSDAY", "SATURDAY"]
}
]
})
```
## Response
If the request is successful, Smartcar will return a message.
If the request is successful, Smartcar will return `success`.
```json Example Response
{
"message": "Successfully sent request to vehicle",
"status": "success"
}
```
# Permissions
Source: https://smartcar.com/docs/api-reference/permissions
In order to use an endpoint or webhook, you'll need to request the associated permissions from your user in [Connect](/docs/connect/what-is-connect).
A permission is *optional* by default. This means that if you were to pass `read_fuel` in your Connect URL and the user tried to connect a Tesla, the permission would not show up as Tesla does not support fuel.
It can be made required by adding the `required:` prefix to the permission name, e.g. `required:read_fuel`. In this case, if a user were to try and connect a Tesla they'd see a `No Compatible Vehicles` Connect error as your
application is requiring vehicles to have the `read_fuel` permission.
In Europe, permissions can be conditional meaning that even if the `required:` prefix is provided, a vehicle can make it through Connect as we cannot be sure of a vehicle's capability until you attempt to make an API request.
In this case you'll receive a [VEHICLE\_NOT\_CAPABLE](/errors/api-errors/compatibility-errors#vehicle-not-capable) API error. To read more about capabilities please see [Compatibility by VIN](/api-reference/compatibility/by-vin).
## Read
Permissions prefixed with `read_` allow your application to get data from a vehicle as part of `GET` requests.
| | |
| ---------------------------- | --------------------------------------------------------------------------------------------------------------------- |
| `read_alerts` | Read alerts from the vehicle |
| `read_battery` | Read an EV's high voltage battery data |
| `read_charge` | Read charging data |
| `read_charge_locations` | Access previous charging locations and their associated charging configurations |
| `read_charge_records` | Read charge records and associated billing information |
| `read_charge_events` | Receive notifications for events associate with charging |
| `read_climate` | Read the status and settings of the vehicle's climate control system |
| `read_compass` | Read the compass direction the vehicle is facing |
| `read_diagnostics` | Read a vehicle's system status and/or Diagnostic Trouble Codes |
| `read_engine_oil` | Read vehicle engine oil health |
| `read_extended_vehicle_info` | Read vehicle configuration information from a vehicle |
| `read_fuel` | Read fuel tank level |
| `read_location` | Access the vehicles location |
| `read_odometer` | Retrieve total distance traveled |
| `read_security` | Read the lock status of doors, windows, charging port, etc. |
| `read_service_history` | Read a vehicle's dealer service history |
| `read_speedometer` | Read a vehicle's speed |
| `read_thermometer` | Read temperatures from inside and outside the vehicle |
| `read_tires` | Read a vehicle's tire status |
| `read_user_profile` | Read the information associated with a users connected services account profile such as their email and phone number. |
| `read_vehicle_info` | Know make, model, and year |
| `read_vin` | Read VIN |
## Control
Permissions prefixed with `control_` allow your application to issue commands or apply settings to a vehicle as part of `POST` or `PUT` requests.
| | |
| -------------------- | ------------------------------------------------------------------- |
| `control_charge` | Control a vehicle's charge state |
| `control_climate` | Set the status and settings of the vehicle's climate control system |
| `control_navigation` | Send commands to the vehicle's navigation system |
| `control_security` | Lock or unlock the vehicle |
| `control_pin` | Modify a PIN and enable the PIN to Drive feature for the vehicle. |
| `control_trunk` | Open a vehicle's trunk or frunk |
# Send Destination
Source: https://smartcar.com/docs/api-reference/send-destination-to-vehicle
POST /vehicles/{id}/navigation/destination
Send destination coordinates to the vehicle's navigation system.
## Permission
`control_navigation`
## Request
**Path**
**Body**
The latitude of the location you wish to set the vehicle's navigation to.
The longitude of the location you wish to set the vehicle's navigation to.
## Response
If the request is successful, Smartcar will return “success” (HTTP 200 status).
If the request is successful, Smartcar will return a message (HTTP 200 status).
```json Example Response
{
"message": "Successfully sent request to vehicle",
"status": "success"
}
```
# Clear PIN to Drive
Source: https://smartcar.com/docs/api-reference/tesla/clear-pin-to-drive
DELETE /vehicles/{id}/{make}/pin
Disables this feature on the vehicle and resets the PIN.
## Permission
`control_pin`
## Request
**Path**
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/pin" \
-H "Authorization: Bearer {token}" \
-X "DELETE" \
-H "Content-Type: application/json" \
```
```python Python
pin = vehicle.request(
"DELETE",
"{make}/pin"
)
```
```js Node
const pin = vehicle.request(
"DELETE",
"{make}/pin"
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("DELETE")
.path("{make}/pin")
.build();
VehicleResponse pin = vehicle.request(request);
```
```ruby Ruby
pin = vehicle.request(
"DELETE",
"{make}/pin"
)
```
## Response
If the request is successful, Smartcar will return “success”.
If the request is successful, Smartcar will return a message.
```json Example Response
{
"message": "Successfully sent request to vehicle",
"status": "success"
}
```
## Notes
* Call `POST` [PIN to Drive](/api-reference/tesla/set-pin-to-drive) in order to enable this feature and set the PIN
* Currently both owner and driver account types can clear a PIN for the vehicle and disable the feature via the API.
# Charge Port
Source: https://smartcar.com/docs/api-reference/tesla/control-charge-port
POST /vehicles/{id}/{make}/charge/charge_port_door
Open or close the vehicle's charge port door.
## Permission
`control_charge`
## Request
**Path**
**Body**
Indicate whether to open or close the charge port door.
Options: `OPEN` or `CLOSE`
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/charge/charge_port_door" \
-H "Authorization: Bearer {token}" \
-X "POST" \
-H "Content-Type: application/json" \
-d '{"action" : "OPEN"}'
```
```python Python
charge_port = vehicle.request(
"POST",
"{make}/charge/charge_port_door",
{"action" : "OPEN"}
)
```
```js Node
const chargePort = vehicle.request(
"POST",
"{make}/charge/charge_port_door",
{"action" : "OPEN"}
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("POST")
.path("{make}/charge/charge_port_door")
.addBodyParameter("action" : "OPEN")
.build();
VehicleResponse chargePort = vehicle.request(request);
```
```ruby Ruby
charge_port = vehicle.request(
"POST",
"{make}/charge/charge_port_door",
{"action" : "OPEN"}
)
```
## Response
If the request is successful, Smartcar will return “success”.
If the request is successful, Smartcar will return a message.
```json Example Response
{
"message": "Successfully sent request to vehicle",
"status": "success"
}
```
# Frunk
Source: https://smartcar.com/docs/api-reference/tesla/control-frunk
POST /vehicles/{id}/{make}/security/frunk
Open or close the frunk (front trunk) of the Tesla vehicle.
## Permission
`control_trunk`
## Request
**Path**
**Body**
Indicate whether to open or close the charge port door.
Options: `OPEN` or `CLOSE`
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/security/frunk" \
-H "Authorization: Bearer {token}" \
-X "POST" \
-H "Content-Type: application/json" \
-d '{"action" : "OPEN"}'
```
```python Python
frunk = vehicle.request(
"POST",
"{make}/security/frunk",
{"action" : "OPEN"}
)
```
```js Node
const frunk = vehicle.request(
"POST",
"{make}/security/frunk",
{"action" : "OPEN"}
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("POST")
.path("{make}/security/frunk")
.addBodyParameter("action" : "OPEN")
.build();
VehicleResponse frunk = vehicle.request(request);
```
```ruby Ruby
frunk = vehicle.request(
"POST",
"{make}/security/frunk",
{"action" : "OPEN"}
)
```
## Response
If the request is successful, Smartcar will return “success”.
If the request is successful, Smartcar will return a message.
```json Example Response
{
"message": "Successfully sent request to vehicle",
"status": "success"
}
```
# Trunk
Source: https://smartcar.com/docs/api-reference/tesla/control-trunk
POST /vehicles/{id}/{make}/security/trunk
Open or close the trunk of the Tesla vehicle.
## Permission
`control_trunk`
## Request
**Path**
**Body**
Indicate whether to open or close the charge port door.
Options: `OPEN` or `CLOSE`
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/security/trunk" \
-H "Authorization: Bearer {token}" \
-X "POST" \
-H "Content-Type: application/json" \
-d '{"action" : "OPEN"}'
```
```python Python
trunk = vehicle.request(
"POST",
"{make}/security/trunk",
{"action" : "OPEN"}
)
```
```js Node
const trunk = vehicle.request(
"POST",
"{make}/security/trunk",
{"action" : "OPEN"}
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("POST")
.path("{make}/security/trunk")
.addBodyParameter("action" : "OPEN")
.build();
VehicleResponse trunk = vehicle.request(request);
```
```ruby Ruby
trunk = vehicle.request(
"POST",
"{make}/security/trunk",
{"action" : "OPEN"}
)
```
## Response
If the request is successful, Smartcar will return “success”.
If the request is successful, Smartcar will return a message.
```json Example Response
{
"message": "Successfully sent request to vehicle",
"status": "success"
}
```
# Alerts
Source: https://smartcar.com/docs/api-reference/tesla/get-alerts
GET /vehicles/{id}/{make}/alerts
Returns recent alerts from the vehicle.
## Permission
`read_alerts`
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/alerts" \
-H "Authorization: Bearer {token}" \
-X "GET"
```
```python Python
alerts = vehicle.request(
"GET",
"{make}/alerts"
)
```
```js Node
const alerts = await vehicle.request(
"GET",
"{make}/alerts"
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("GET")
.path("{make}/alerts")
.build();
VehicleResponse alerts =vehicle.request(request);
```
```ruby Ruby
alerts = vehicle.request(
"GET",
"{make}/alerts"
)
```
## Request
**Path**
## Response
The name of the alert.
Date and time of the alert.
Indicates recipients of the alert.
Additional context related to the alert.
```json Example Response
{
"alerts" : [
{
"name": "Name_Of_The_Alert",
"dateTime": "2022-07-10T16:20:00.000Z",
"audience": [
"service-fix",
"customer"
],
"userText": "additional description text"
}
]
}
```
# Tesla: Battery Status
Source: https://smartcar.com/docs/api-reference/tesla/get-battery
GET /vehicles/{id}/{make}/battery
Returns all battery related data for a Tesla vehicle.
The following fields are not supported for streaming vehicles:
* TeslaBatteryPercentRemainingUsable
* TeslaBatteryMaxRangeChargeCounter
## Permission
`read_battery`
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/battery" \
-H "Authorization: Bearer {token}" \
-X "GET"
```
```python Python
battery = vehicle.request(
"GET",
"{make}/battery"
)
```
```js Node
const battery = await vehicle.request(
"GET",
"{make}/battery"
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("GET")
.path("{make}/battery")
.build();
VehicleResponse battery = vehicle.request(request);
```
```ruby Ruby
battery = vehicle.request(
"GET",
"{make}/battery"
)
```
## Request
**Path**
## Response
The EV’s state of charge as a percentage.
The distance the vehicle can travel powered by it’s high voltage battery.
Indicates if the battery heater is on.
The estimated remaining distance the vehicle can travel powered by it’s high voltage battery as determined by Tesla.
The ideal remaining distance the vehicle can travel powered by it’s high voltage battery as determined by Tesla.
The EV’s useable state of charge as a percentage as reported by Tesla.
The number of times the vehicle has been charged to 100% as reported by Tesla.
Indicates if there is enough power to heat the battery.
```json Example Response
{
"heaterOn": null,
"maxRangeChargeCounter" : null,
"notEnoughPowerToHeat" : true,
"percentRemaining": 0.3,
"percentRemainingUsable" : 0.29,
"range": 40.5,
"rangeEstimated": 39.01,
"rangeIdeal" : 40.5
}
```
# Cabin Climate
Source: https://smartcar.com/docs/api-reference/tesla/get-cabin
GET /vehicles/{id}/{make}/climate/cabin
Returns the current state and target temperature setting of a vehicle's cabin climate system.
## Permission
`read_climate`
## Request
**Path**
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/climate/cabin" \
-H "Authorization: Bearer {token}" \
-X "GET"
```
```python Python
cabin = vehicle.request(
"GET",
"{make}/climate/cabin"
)
```
```js Node
const chargeCompletion = await vehicle.request(
"GET",
"{make}/climate/cabin"
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("GET")
.path("{make}/climate/cabin")
.build();
VehicleResponse chargeCompletion = vehicle.request(request);
```
```ruby Ruby
chargeCompletion = vehicle.request(
"GET",
"{make}/climate/cabin"
)
```
## Response
The current state of the climate cabin system.
The target temperature setting of the vehicle when the climate system is on (in Celsius by default or in Fahrenheit using the sc-unit-system).
```json Example Response
{
"status": "ON",
"temperature": 20
}
```
# Tesla: Charge Status
Source: https://smartcar.com/docs/api-reference/tesla/get-charge
GET /vehicles/{id}/{make}/charge
Returns all charging related data for a Tesla vehicle.
The following fields are not supported for streaming vehicles:
* TeslaChargeRangeAddedRated
* TeslaChargeRangeAddedIdeal
* TeslaChargeChargeRate
* TeslaChargeAmperageMaxCharger
* TeslaChargeChargePortColor
## Permission
`read_charge`
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/charge" \
-H "Authorization: Bearer {token}" \
-X "GET"
```
```python Python
charge = vehicle.request(
"GET",
"{make}/charge"
)
```
```js Node
const charge = await vehicle.request(
"GET",
"{make}/charge"
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("GET")
.path("{make}/charge")
.build();
VehicleResponse charge = vehicle.request(request);
```
```ruby Ruby
charge = vehicle.request(
"GET",
"{make}/charge"
)
```
## Request
**Path**
## Response
The rate that the vehicle is charging at (in amperes).
An ISO8601 formatted datetime (YYYY-MM-DDTHH:mm:ss.SSSZ) for the time at which the vehicle expects to complete this charging session.
The instant power measured by the vehicle (in kilowatts).
Indicates if the vehicle is plugged in
Indicates the charging status of the vehicle
Indicates the level at which the vehicle will stop charging and be considered fully charged as a percentage.
Indicates the max amperage the vehicle can request from the charger.
Indicates the max SoC limit that can be set.
Indicates the min SoC limit that can be set.
Indicates the default SoC limit of the vehicle.
Indicates the amperage requested from the charger by the vehicle.
Energy added in the current charging session or most recent session if the vehicle is not charging (in kilowatts).
The rated range as determined by Tesla added in the current charging session or most recent session if the vehicle is not charging (in kilometers added).
The ideal range as determined by Tesla added in the current charging session or most recent session if the vehicle is not charging (in kilometers added).
The rate of range added in the charging session (in kilometers added / hour).
Standard of the connector e.g. SAE
When charging, indicates if the vehicle connected to a fast charger.
Indicates the type of fast charger.
Indicates the brand of fast charger.
Indicates the max amperage supported by the charger.
The indicator light color of the connector.
Indicates if th charge port door is open.
Indicates if the charge port latch status.
Indicates the charging phase.
```json Example Response
{
"amperage": 0,
"completionTime": null,
"wattage": 0,
"voltage": 1,
"isPluggedIn": false,
"state": "NOT_CHARGING",
"socLimit": 0.8,
"amperageMaxVehicle": 48,
"socLimitMax": 1,
"socLimitMin": 0.5,
"socLimitDefault": 0.8,
"amperageRequested": 48,
"energyAdded": 11.52,
"rangeAddedRated": 70.811,
"rangeAddedIdeal": 70.811,
"chargeRate": 0,
"connector": "",
"fastChargerPresent": false,
"fastChargerType": "",
"fastChargerBrand": "",
"amperageMaxCharger": 48,
"chargePortColor": "",
"chargePortOpen": false,
"chargePortLatch": "Engaged",
"chargerPhases": null
}
```
# Charge Completion Time
Source: https://smartcar.com/docs/api-reference/tesla/get-charge-completion-time
GET /vehicles/{id}/{make}/charge/completion
When the vehicle is charging, returns the date and time when the vehicle is expected to reach its charge limit. When the vehicle is not charging, this endpoint results in a vehicle state error.
This endpoint is currently available for `tesla`.
## Permission
`read_charge`
## Request
`vehicle_id` of the vehicle you are making the request to.
The make to pass in the URL.
## Response
An ISO8601 formatted datetime (`YYYY-MM-DDTHH:mm:ss.SSSZ`) for the time at which the vehicle expects to complete this charging session.
```json Example Response
{
"time": "2022-01-13T22:52:55.358Z"
}
```
# Charge Billing Records
Source: https://smartcar.com/docs/api-reference/tesla/get-charge-records-billing
GET /vehicles/{id}/{make}/charge/records/billing
Returns information about charging sessions for Tesla vehicles at public Tesla chargers including cost and charging site.
## Permission
`read_charge_records`
## Request
**Path**
**Query**
Date of the first record to return in YYYY-MM-DD format.
Defaults to 30 days prior or when the owner first granted your application access, whichever is shorter.
Date of the final record to return in YYYY-MM-DD format. Defaults to the date of the request.
The page number to fetch from Tesla where page 1 contains the most recent records.
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/charge/records/billing?page=12&startDate=2023-08-24" \
-H "Authorization: Bearer {token}" \
-X "GET" \
-H "Content-Type: application/json" \
```
```python Python
billing = vehicle.request(
"GET",
"{make}/charge/records/billing?startDate=2023-08-24&page=12"
)
```
```js Node
const billing = vehicle.request(
"GET",
"{make}/charge/records/billing?startDate=2023-08-24&page=12"
);
```
```java Java
SmartcarVehicleRequest request =
new SmartcarVehicleRequest.Builder()
.method("GET")
.path("ford/charge/schedule_by_location")
.addQueryParameter("startDate", "2023-08-24")
.addQueryParameter("page", "12")
.build();
VehicleResponse billing = vehicle.request(request);
```
```ruby Ruby
billing = vehicle.request(
"GET",
"{make}/charge/records/billing?startDate=2023-08-24&page=12"
)
```
## Response
An array of billing records for the vehicle associated with charging at public Tesla charging stations.
Can be empty if the specified page does not contain any records for the vehicle.
This **does not** mean that subsequent pages will also contain no records. Please check the `hasMoreData` field for confirmation instead.
The date and time of charging session start, formatted in ISO 8601 standard.
The date and time of charging session end, formatted in ISO 8601 standard.
Energy consumed in the charging session.
A cost breakout of the charging session.
The currency code for the fees.
Fess associated with charging the vehicle.
Fees associated with the session other than charging the vehicle e.g. parking.
The name of the charging site.
Tesla’s id for this charging session.
Indicates if there are any more records to fetch from Tesla for this vehicle. **Does not** guarantee a non-empty list for the next page when `true`.
```json Example Response
{
"records": [
{
"chargeEnd": "2022-07-10T16:20:00.000Z",
"chargeStart": "2022-07-10T15:40:00.000Z",
"energyConsumed": 44.10293884
"cost": {
"currency": "USD",
"energy": "41",
"other": "41"
},
"location": "Los Gatos, CA",
"recordId": "GF220075000028-3-1682903685"
}
],
"hasMoreData" : false
}
```
# Charge Schedule
Source: https://smartcar.com/docs/api-reference/tesla/get-charge-schedule
GET /vehicles/{id}/{make}/charge/schedule
Returns the charging schedule of a vehicle. The response contains the start time and departure time of the vehicle's charging schedule.
## Permission
`read_charge`
## Request
**Path**
## Response
The departure time configuration for the charging schedule.
Indicates whether this schedule type is enabled or disabled.
Only one of `departureTime` or `departureTime` can be enabled at a time.
When plugged in, the vehicle **may** delay starting a charging session as long as it can reach its
[charge limit](/api-reference/evs/get-charge-limit) by this time in HH:mm.
The start time configuration for the charging schedule.
Indicates whether this schedule type is enabled or disabled.
Only one of `departureTime` or `departureTime` can be enabled at a time.
When plugged in, the vehicle will delay starting a charging session until this time in HH:mm.
```json Example Response
{
"departureTime": {
"enabled": false,
"time": null
},
"startTime": {
"enabled": true,
"time": "18:30"
}
}
```
# Voltage
Source: https://smartcar.com/docs/api-reference/tesla/get-charge-voltmeter
GET /vehicles/{id}/{make}/charge/voltmeter
When the vehicle is plugged in, returns the charging voltage measured by the vehicle. When the vehicle is not plugged in, this endpoint results in a vehicle state error.
This endpoint is currently available for `tesla`.
## Permission
`read_charge`
## Request
## Response
The potential difference measured by the vehicle in volts (V).
```json Example Response
{
"voltage": 240
}
```
# Wattage
Source: https://smartcar.com/docs/api-reference/tesla/get-charge-wattmeter
GET /vehicles/{id}/{make}/charge/wattmeter
When the vehicle is charging, returns the instant charging wattage as measured by the vehicle. When the vehicle is not charging, this endpoint results in a vehicle state error.
## Permission
`read_charge`
## Request
**Path**
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/charge/wattmeter" \
-H "Authorization: Bearer {token}" \
-X "GET"
```
```python Python
wattmeter = vehicle.request(
"GET",
"{make}/charge/wattmeter"
)
```
```js Node
const wattmeter = await vehicle.request(
"GET",
"{make}/charge/wattmeter"
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("GET")
.path("{make}/charge/wattmeter")
.build();
VehicleResponse wattmeter = vehicle.request(request);
```
```ruby Ruby
wattmeter = vehicle.request(
"GET",
"{make}/charge/wattmeter"
)
```
## Response
The instant power measured by the vehicle (in kilowatts).
```json Example Response
{
"wattage": 3.5
}
```
# Compass
Source: https://smartcar.com/docs/api-reference/tesla/get-compass-heading
GET /vehicles/{id}/{make}/compass
Returns the current compass heading and direction of the vehicle.
## Permission
`read_compass`
## Request
**Path**
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/compass" \
-H "Authorization: Bearer {token}" \
-X "GET"
```
```python Python
compass = vehicle.request(
"GET",
"{make}/compass"
)
```
```js Node
const compass = await vehicle.request(
"GET",
"{make}/compass"
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("GET")
.path("{make}/compass")
.build();
VehicleResponse compass = vehicle.request(request);
```
```ruby Ruby
compass = vehicle.request(
"GET",
"{make}/compass"
)
```
## Response
The current direction of the vehicle.
The current compass heading of the vehicle (in degrees).
```json Example Response
{
"direction": "SW",
"heading": 185
}
```
# Defroster
Source: https://smartcar.com/docs/api-reference/tesla/get-defroster
GET /vehicles/{is}/{make}/climate/defroster
Returns the current state of a vehicle's front and rear defroster.
## Permission
`read_climate`
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/climate/defroster" \
-H "Authorization: Bearer {token}" \
-X "GET"
```
```python Python
defroster = vehicle.request(
"GET",
"{make}/climate/defroster"
)
```
```js Node
const defroster = await vehicle.request(
"GET",
"{make}/climate/defroster"
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("GET")
.path("{make}/climate/defroster")
.build();
VehicleResponse defroster = vehicle.request(request);
```
```ruby Ruby
defroster = vehicle.request(
"GET",
"{make}/climate/defroster"
)
```
## Request
**Path**
## Response
The current state of the front defroster.
The current state of the rear defroster.
```json Example Response
{
"frontStatus": "ON",
"rearStatus": "OFF"
}
```
# Extended Vehicle Info
Source: https://smartcar.com/docs/api-reference/tesla/get-ext-vehicle-info
GET /vehicles/{id}/{make}/attributes
Returns detailed configuration information for a vehicle.
## Permission
`read_extended_vehicle_info`
## Request
**Path**
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/attributes" \
-H "Authorization: Bearer {token}" \
-X "GET"
```
```python Python
attributes = vehicle.request(
"GET",
"{make}/attributes"
)
```
```js Node
const attributes = await vehicle.request(
"GET",
"{make}/attributes"
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("GET")
.path("{make}/attributes")
.build();
VehicleResponse attributes = vehicle.request(request);
```
```ruby Ruby
attributes = vehicle.request(
"GET",
"{make}/attributes"
)
```
## Response
A vehicle ID (UUID v4).
The manufacturer of the vehicle.
The model of the vehicle.
The model year.
Vehicle's current firmware.
Indicates the Tesla's trim.
Efficiency package.
Performance package.
Vehicle's nickname.
Driver Assist version.
Indicates `true` if the vehicle is currently at the home address set in the vehicle. `False` indicates the vehicle is not at home or home location is not set. This value is `null` if `atHome` status could not be determined.
This field comes back as `null` if the vehicle is not capable of supporting Tesla's Telemetry API (streaming).
Sentry Mode status and availability.
Does the vehicle support Sentry Mode.
Is Sentry Mode currently enabled for a vehicle.
Descriptors of the vehicle's interior and exterior.
Exterior color.
Exterior trim.
Interior trim.
Descriptors of the vehicle's wheel.
Style of the wheel.
Diameter of the wheel.
Details on the vehicle's drive unit(s).
Rear drive unit.
Front drive unit.
```json Example Response
{
"driveUnit": {
"front": "PM216MOSFET",
"rear": "NoneOrSmall"
},
"driverAssistVersion": "TeslaAP3",
"efficiencyPackage": "MY2021",
"firmwareVersion": "2022.8.10.12 0ce482dac45d",
"id": "36ab27d0-fd9d-4455-823a-ce30af709ffc",
"make": "TESLA",
"model": "Model S",
"nickname": "Tommy",
"performancePackage": "Base",
"sentryMode": {
"available": false,
"enabled": true
},
"style": {
"exteriorColor": "SolidBlack",
"exteriorTrim": "Black",
"interiorTrim": "Black2"
},
"trimBadging": "74d",
"wheel": {
"diameter": 482.6,
"style": "Apollo"
},
"year": 2022,
"atHome": true
}
```
# Exterior Temperature
Source: https://smartcar.com/docs/api-reference/tesla/get-exterior-temperature
GET /vehicles/{id}/{make}/thermometer/exterior
Returns the vehicle’s last known exterior thermometer reading. See our [climate setting](/docs/api-reference/tesla/get-cabin) endpoints for managing a cabin temperature.
## Permission
`read_thermometer`
## Request
**Path**
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/thermometer/exterior" \
-H "Authorization: Bearer {token}" \
-X "GET"
```
```python Python
temperature = vehicle.request(
"GET",
"{make}/thermometer/exterior"
)
```
```js Node
const temperature = await vehicle.request(
"GET",
"{make}/thermometer/exterior"
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("GET")
.path("{make}/thermometer/exterior")
.build();
VehicleResponse temperature = vehicle.request(request);
```
```ruby Ruby
temperature = vehicle.request(
"GET",
"{make}/thermometer/exterior"
)
```
## Response
The current exterior temperature of the vehicle.
```json Example Response
{
"temperature": 33.42
}
```
# Interior Temperature
Source: https://smartcar.com/docs/api-reference/tesla/get-interior-temperature
GET /vehicles/{id}/{make}/thermometer/interior
Returns the vehicle’s last known interior thermometer reading. See our [climate setting](/docs/api-reference/tesla/get-cabin) endpoints for managing a cabin temperature.
## Permission
`read_thermometer`
## Request
**Path**
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/thermometer/interior" \
-H "Authorization: Bearer {token}" \
-X "GET"
```
```python Python
temperature = vehicle.request(
"GET",
"{make}/thermometer/interior"
)
```
```js Node
const temperature = await vehicle.request(
"GET",
"{make}/thermometer/interior"
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("GET")
.path("{make}/thermometer/interior")
.build();
VehicleResponse temperature = vehicle.request(request);
```
```ruby Ruby
temperature = vehicle.request(
"GET",
"{make}/thermometer/interior"
)
```
## Response
The current interior temperature of the vehicle.
```json Example Response
{
"temperature": 25.64
}
```
# Migration Status
Source: https://smartcar.com/docs/api-reference/tesla/get-migration-status
GET /vehicles/{id}/{make}/migration
Indicates if the vehicle needs to migrate to Tesla's new API. See [Tesla - What's New](https://smartcar.com/docs/help/oem-integrations/tesla/whats-new) for more details.
## Permission
`read_vehicle_info`
## Request
**Path**
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/migration" \
-H "Authorization: Bearer {token}" \
-X "GET"
```
```python Python
status = vehicle.request(
"GET",
"{make}/migration"
)
```
```js Node
const status = await vehicle.request(
"GET",
"{make}/migration"
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("GET")
.path("{make}/migration")
.build();
VehicleResponse status = vehicle.request(request);
```
```ruby Ruby
status = vehicle.request(
"GET",
"{make}/migration"
)
```
## Response
Set to `false` if the vehicle is connected to Smartcar via Tesla's new integration. See [Tesla - What's New](/help/oem-integrations/tesla/whats-new) for more details.
```json Example Response
{
"requiresMigration": false
}
```
# Speed
Source: https://smartcar.com/docs/api-reference/tesla/get-speedometer
GET /vehicles/{id}/{make}/speedometer
Returns the current speed of the vehicle.
## Permission
`read_speedometer`
## Request
**Path**
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/speedometer" \
-H "Authorization: Bearer {token}" \
-X "GET"
```
```python Python
speed = vehicle.request(
"GET",
"{make}/speedometer"
)
```
```js Node
const speed = await vehicle.request(
"GET",
"{make}/speedometer"
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("GET")
.path("{make}/speedometer")
.build();
VehicleResponse speed = vehicle.request(request);
```
```ruby Ruby
speed = vehicle.request(
"GET",
"{make}/speedometer"
)
```
## Response
The current speed of the vehicle.
```json Example Response
{
"speed": 84.32
}
```
# Steering Heater
Source: https://smartcar.com/docs/api-reference/tesla/get-steering-heater
GET /vehicles/{id}/{make}/climate/steering_wheel
Returns the current state of a vehicle's steering wheel heater system.
## Permission
`read_climate`
## Request
**Path**
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/climate/steering_wheel" \
-H "Authorization: Bearer {token}" \
-X "GET"
```
```python Python
steering_wheel = vehicle.request(
"GET",
"{make}/climate/steering_wheel"
)
```
```js Node
const steeringWheel = await vehicle.request(
"GET",
"{make}/climate/steering_wheel"
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("GET")
.path("{make}/climate/steering_wheel")
.build();
VehicleResponse steeringWheel = vehicle.request(request);
```
```ruby Ruby
steering_wheel = vehicle.request(
"GET",
"{make}/climate/steering_wheel"
)
```
## Response
The current state of the steering wheel heater system. `UNAVAILABLE` indicates the vehicle is not equipped with a steering wheel heater.
```json Example Response
{
"status": "ON",
}
```
# User Access
Source: https://smartcar.com/docs/api-reference/tesla/get-user-access
GET /vehicles/{id}/{make}/user/access
Returns the account type and permissions for the connected Tesla account.
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/user/access" \
-H "Authorization: Bearer {token}" \
-X "GET"
```
```python Python
access = vehicle.request(
"GET",
"{make}/user/access"
)
```
```js Node
const access = await vehicle.request(
"GET",
"{make}/user/access"
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("GET")
.path("{make}/user/access")
.build();
VehicleResponse access =vehicle.request(request);
```
```ruby Ruby
access = vehicle.request(
"GET",
"{make}/user/access"
)
```
## Request
**Path**
## Response
Returns the type of Tesla account connected. Can be either `OWNER` or `DRIVER`. Please see our [Tesla FAQs](/help/oem-integrations/tesla/faqs) for details on the differences between account types.
Returns a list of permissions granted by the user with their Tesla account. Please see [this page](/help/oem-integrations/tesla/developers#permission-mappings) on the mapping from Smartcar permissions to Tesla's.
```json Example Response
{
"accessType": "OWNER",
"permissions" : ["vehicle_cmds", "vehicle_device_data"]
}
```
## Notes
* If you're receiving a [SERVER:INTERNAL](/errors/api-errors/server-errors#internal) error please have the user reconnect their vehicle.
# User Info
Source: https://smartcar.com/docs/api-reference/tesla/get-user-info
GET /vehicles/{id}/{make}/user/info
Returns the email associated with the connected Tesla account.
## Permission
`read_user_profile`
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/user/info" \
-H "Authorization: Bearer {token}" \
-X "GET"
```
```python Python
info = vehicle.request(
"GET",
"{make}/user/info"
)
```
```js Node
const info = await vehicle.request(
"GET",
"{make}/user/info"
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("GET")
.path("{make}/user/info")
.build();
VehicleResponse info =vehicle.request(request);
```
```ruby Ruby
info = vehicle.request(
"GET",
"{make}/user/info"
)
```
## Request
**Path**
## Response
The email associated with the connected Tesla account.
```json Example Response
{
"email" : "api@smartcar.com"
}
```
# Vehicle Status
Source: https://smartcar.com/docs/api-reference/tesla/get-vehicle-status
GET /vehicles/{id}/{make}/status
Returns the status for the vehicle.
## Permission
`read_extended_vehicle_info`
## Request
**Path**
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/status" \
-H "Authorization: Bearer {token}" \
-X "GET"
```
```python Python
status = vehicle.request(
"GET",
"{make}/status"
)
```
```js Node
const status = await vehicle.request(
"GET",
"{make}/status"
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("GET")
.path("{make}/status")
.build();
VehicleResponse status = vehicle.request(request);
```
```ruby Ruby
status = vehicle.request(
"GET",
"{make}/status"
)
```
## Response
The current status of the vehicle. If the vehicle is asleep, this request will not wake the vehicle.
Indicates if the vehicle is in service mode.
Indicates the current gear shift position.
```json Example Response
{
"status": "ASLEEP",
"inService": true,
"gear": "DRIVE"
}
```
# Virtual Key Status
Source: https://smartcar.com/docs/api-reference/tesla/get-virtual-key-status
GET /vehicles/{id}/{make}/virtual_key
Indicates if a vehicle has the appropriate virtual key installed. See [Tesla - What's New](https://smartcar.com/docs/help/oem-integrations/tesla/whats-new#if-your-application-issues-commands) for more details on Tesla's virtual key requirements.
## Permission
`read_vehicle_info`
## Request
**Path**
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/virtual_key" \
-H "Authorization: Bearer {token}" \
-X "GET"
```
```python Python
virtual_key = vehicle.request(
"GET",
"{make}/virtual_key"
)
```
```js Node
const virtualKey = await vehicle.request(
"GET",
"{make}/virtual_key"
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("GET")
.path("{make}/virtual_key")
.build();
VehicleResponse virtualKey = vehicle.request(request);
```
```ruby Ruby
virtual_key = vehicle.request(
"GET",
"{make}/virtual_key"
)
```
## Response
Returns `true` if the vehicle has the appropriate Virtual Key installed. See [Tesla - What's New](/help/oem-integrations/tesla/whats-new#if-your-application-issues-commands) for more details on Tesla's virtual key requirements.
```json Example Response
{
"isPaired": true
}
```
## Notes
* This endpoint will throw a [COMPATIBILITY:PLATFORM\_NOT\_CAPABLE](/errors/api-errors/compatibility-errors#platform-not-capable) error if a vehicle is connected to your application via Tesla's legacy integration.
* This endpoint will throw a [CONNECTED\_SERVICES\_ACCOUNT:VEHICLE\_MISSING](/errors/api-errors/compatibility-errors#vehicle-missing) error if the Tesla account connected to Smartcar is **not** the Owner of the vehicle. See our [FAQs](/help/oem-integrations/tesla/faqs#can-owner-and-driver-accounts-authorize-access-with-smartcar-through-connect) for more information on Owner and Driver account types.
# Cabin Climate
Source: https://smartcar.com/docs/api-reference/tesla/set-cabin
POST /vehicles/{id}/{make}/climate/cabin
Set the temperature and control the cabin climate system for a vehicle.
## Permission
`control_climate`
## Request
**Path**
**Body**
Indicate whether to start or stop the cabin climate control system, or set the temperature.
If starting or stopping the system, `temperature` is optional and will use the vehicle's current setting by default.
Use `SET` to set the `temperature` without changing the climate systems status.
Indicate what temperature to set (in Celsius by default or in Fahrenheit using the sc-unit-system).
If the provided temperature is out of the bounds allowed by the vehicle's climate control system,
the request will fail with the upper and lower limits in the error response message.
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/climate/cabin" \
-H "Authorization: Bearer {token}" \
-X "POST" \
-H "Content-Type: application/json" \
-d '{"action": "SET", "temperature": 20}'
```
```python Python
set_climate_cabin = vehicle.request(
"POST",
"{make}/climate/cabin",
{
"action": "SET",
"temperature": 18
}
)
```
```js Node
const vehicleStopCabin = vehicle.request(
"POST",
"{make}/climate/cabin",
{"action": "STOP"}
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("POST")
.path("{make}/climate/cabin")
.addBodyParameter("action", "START")
.build();
VehicleResponse startClimateCabin = vehicle.request(request);
```
```ruby Ruby
setChargeScheduleByLocation = vehicle.request(
"POST",
"{make}/climate/cabin",
{"action" : "START"}
)
```
## Response
If the request is successful, Smartcar will return `"success"` (HTTP 200 status) containing the current state of the climate cabin system.
The target temperature setting of the vehicle when the climate system is on (in Celsius by default or in Fahrenheit using the sc-unit-system).
```json Example Response
{
"status": "ON",
"temperature": 24
}
```
# Amperage
Source: https://smartcar.com/docs/api-reference/tesla/set-charge-ammeter
POST /vehicles/{id}/{make}/charge/ammeter
Set the amperage drawn by the vehicle from the EVSE for the current charging session. If the vehicle is not plugged in, this endpoint results in a vehicle state error.
## Permission
`control_charge`
## Request
**Path**
**Body**
The target amperage to be drawn by the vehicle from the charging point (in amperes). If the value passed is greater than what is supported by the charger, it will be set to the maximum.
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/charge/ammeter" \
-H "Authorization: Bearer {token}" \
-X "POST" \
-H "Content-Type: application/json" \
-d '{"amperage": 48}'
```
```python Python
ammeter = vehicle.request(
"POST",
"{make}/charge/ammeter",
{"amperage": 48}
)
```
```js Node
const ammeter = vehicle.request(
"POST",
"{make}/charge/ammeter",
{"amperage": 48}
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("POST")
.path("{make}/charge/ammeter")
.addBodyParameter("amperage": 48)
.build();
VehicleResponse ammeter = vehicle.request(request);
```
```ruby Ruby
ammeter = vehicle.request(
"POST",
"{make}/charge/ammeter",
{"amperage": 48}
)
```
## Response
If the request is successful, Smartcar will return “success” (HTTP 200 status). If the amperage passed was greater than what is supported by the charger, it will be set to the maximum which will be shown here.
If the request is successful, Smartcar will return a message (HTTP 200 status) containing the amperage set to be drawn from the vehicle.
```json Example Response
{
"message": "Successfully sent the following amperage: 48",
"status": "success"
}
```
# Charge Schedule
Source: https://smartcar.com/docs/api-reference/tesla/set-charge-schedule
POST /vehicles/{id}/{make}/charge/schedule
Sets the charging schedule for a vehicle.
## Permission
`control_charge`
## Request
**Path**
**Body**
The type of schedule you want to set.
When plugged in, the vehicle will delay starting a charging session until this time in `HH:mm`.
When plugged in, the vehicle may delay starting a charging session as long as it can reach its
[charge limit](/api-reference/evs/get-charge-limit) by this time in `HH:mm`.
Enables or disables the specified charging schedule.
The time for the provided schedule type in HH:mm.
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/charge/schedule" \
-H "Authorization: Bearer {token}" \
-X "POST" \
-H "Content-Type: application/json" \
-d '{"type": "START_TIME", "enable": true, "time": "23:30"}'
```
```python Python
chargeSchedule = vehicle.request(
"POST",
"{make}/charge/schedule",
{"type": "START_TIME", "enable": true, "time": "23:30"}
)
```
```js Node
const chargeSchedule = vehicle.request(
"POST",
"{make}/charge/schedule",
{"type": "START_TIME", "enable": true, "time": "23:30"}
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("POST")
.path("{make}/charge/schedule")
.addBodyParameter("type", "START_TIME")
.addBodyParameter("enable", "true")
.addBodyParameter("time", "23:30")
.build();
ChargeSchedule chargeSchedule = vehicle.request(request);
```
```ruby Ruby
chargeSchedule = vehicle.request(
"POST",
"{make}/charge/schedule",
{"type": "START_TIME", "enable": true, "time": "23:30"}
)
```
## Response
The departure time configuration for the charging schedule.
Indicates whether this schedule type is enabled or disabled.
Only one of `departureTime` or `departureTime` can be enabled at a time.
When plugged in, the vehicle **may** delay starting a charging session as long as it can reach its
[charge limit](/api-reference/evs/get-charge-limit) by this time in HH:mm.
The start time configuration for the charging schedule.
Indicates whether this schedule type is enabled or disabled.
Only one of `departureTime` or `departureTime` can be enabled at a time.
When plugged in, the vehicle will delay starting a charging session until this time in HH:mm.
```json Example Response
{
"departureTime": {
"enabled": false,
"time": null
},
"startTime": {
"enabled": true,
"time": "18:30"
}
}
```
# Defroster
Source: https://smartcar.com/docs/api-reference/tesla/set-defroster
POST /vehicles/{id}/{make}/climate/defroster
Start or stop the front and rear defroster for a vehicle.
## Permission
`control_climate`
## Request
**Path**
**Body**
Indicate whether to start or stop defrosting the vehicle.
Options: `START` or `STOP`
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/climate/defroster" \
-H "Authorization: Bearer {token}" \
-X "POST" \
-H "Content-Type: application/json" \
-d '{"action" : "STOP"}'
```
```python Python
defroster = vehicle.request(
"POST",
"{make}/climate/defroster",
{"action" : "STOP"}
)
```
```js Node
const defroster = vehicle.request(
"POST",
"{make}/climate/defroster",
{"action" : "STOP"}
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("POST")
.path("{make}/climate/defroster")
.addBodyParameter("action" : "STOP")
.build();
VehicleResponse defroster = vehicle.request(request);
```
```ruby Ruby
defroster = vehicle.request(
"POST",
"{make}/climate/defroster",
{"action" : "STOP"}
)
```
## Response
If the request is successful, Smartcar will return `status` containing the action sent to the
climate defroster system of the vehicle.
```json Example Response
{
"status": "START"
}
```
# Set PIN to Drive
Source: https://smartcar.com/docs/api-reference/tesla/set-pin-to-drive
POST /vehicles/{id}/{make}/pin
Enables this feature on the vehicle and sets the PIN needed in order to drive it.
## Permission
`control_pin`
## Request
**Path**
**Body**
A four digit numeric PIN
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/pin" \
-H "Authorization: Bearer {token}" \
-X "POST" \
-H "Content-Type: application/json" \
-d '{"pin" : "1234"}'
```
```python Python
pin = vehicle.request(
"POST",
"{make}/pin",
{"pin" : "1234"}
)
```
```js Node
const pin = vehicle.request(
"POST",
"{make}/pin",
{"pin" : "1234"}
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("POST")
.path("{make}/pin")
.addBodyParameter("pin" : "1234")
.build();
VehicleResponse pin = vehicle.request(request);
```
```ruby Ruby
pin = vehicle.request(
"POST",
"{make}/pin",
{"pin" : "1234"}
)
```
## Response
If the request is successful, Smartcar will return “success”.
If the request is successful, Smartcar will return a message.
```json Example Response
{
"message": "Successfully sent request to vehicle",
"status": "success"
}
```
## Notes
* Calling this endpoint will override an existing PIN on the vehicle.
* Call `DELETE` [PIN to Drive](/api-reference/tesla/clear-pin-to-drive) in order to enable this feature and set the PIN
* Currently both owner and driver account types can set a PIN for the vehicle and enable the feature via the API.
* Only account owners can disable this feature from the Tesla app.
# Steering Heater
Source: https://smartcar.com/docs/api-reference/tesla/set-steering-heater
POST /vehicles/{id}/{make}/climate/steering_wheel
Start or stop heating a vehicle's steering wheel.
## Permission
`control_climate`
## Request
**Path**
**Body**
Indicate whether to start or stop heating the vehicle's steering wheel.
Options: `START` or `STOP`
```curl cURL
curl "https://api.smartcar.com/v2.0/vehicles/{id}/{make}/climate/steering_wheel" \
-H "Authorization: Bearer {token}" \
-X "POST" \
-H "Content-Type: application/json" \
-d '{"action" : "STOP"}'
```
```python Python
steering_wheel = vehicle.request(
"POST",
"{make}/climate/steering_wheel",
{"action" : "STOP"}
)
```
```js Node
const steeringWheel = vehicle.request(
"POST",
"{make}/climate/steering_wheel",
{"action" : "STOP"}
);
```
```java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("POST")
.path("{make}/climate/steering_wheel")
.addBodyParameter("action" : "STOP")
.build();
VehicleResponse steeringWheel = vehicle.request(request);
```
```ruby Ruby
steering_wheel = vehicle.request(
"POST",
"{make}/climate/steering_wheel",
{"action" : "STOP"}
)
```
## Response
If the request is successful, Smartcar will return status containing the action sent to the vehicle.
`UNAVAILABLE` indicates the vehicle is not equipped with a steering wheel heater.
```json Example Response
{
"status": "START",
}
```
# User
Source: https://smartcar.com/docs/api-reference/user
GET /user
Returns the ID of the vehicle owner who granted access to your application.
This should be used as the static unique identifier for storing the access token and refresh token pair in your database.
Note: A single user can own multiple vehicles, and multiple users can own the same vehicle.
When using Single Select for Connect, a single user with multiple vehicles
will have a 1:1 (`access_token`:`vehicle_id`) mapping.
## Response
A user ID (UUID v4).
```json Example Response
{
"id": "e0514ef4-5226-11e8-8c13-8f6e8f02e27e"
}
```
# Callback URI Verification
Source: https://smartcar.com/docs/api-reference/webhooks/callback-verification
Verify your newly-created webhook.
This page covers the verification step **when first setting up a webhook** on Dashboard. Please see our [payload verification](/api-reference/webhooks/payload-verification) section for information on how to verify webhook payloads from vehicles.
When you first set up a webhook in the Smartcar Dashboard, Smartcar will post a challenge request to ensure we're sending payloads to the correct place. This is a **one time** event and will be in the following format:
```json verificationRequest.body
{
"version": "2.0",
"webhookId": "",
"eventName": "verify",
"payload": { "challenge": "" }
}
```
Upon receiving the request, your server will need respond to the challenge by hashing `payload.challenge` with your `application_management_token` to create a `SHA-256` based `HMAC`.
Our [backend SDKs](/api-reference/api-sdks) have helper methods to generate the `HMAC`.
```python Python
hmac = smartcar.hash_challenge(
application_management_token,
payload.challenge
)
```
```js Node
let hmac = smartcar.hashChallenge(
application_management_token,
payload.challenge
);
```
```java Java
String hmac = Smartcar.hashChallenge(
application_management_token,
payload.challenge
);
```
```ruby Ruby
hmac = Smartcar.hash_challenge(
application_management_token,
payload.challenge
)
```
Return the hex-encoded hash as the value for `challenge` in your response body with a `200` status code, and the `Content-Type` header set to `application/json`.
```json verificationResponse.body
{
"challenge" : "{HMAC}"
}
```
# Payload Verification
Source: https://smartcar.com/docs/api-reference/webhooks/payload-verification
This page covers verification of payloads from vehicles.
Please see our [callback verification](/api-reference/webhooks/callback-verification) section for
information on how to initially verify your callback uri.
Verify webhook payloads against the `SC-Signature` header and a `SHA-256` based `HMAC` generated by hashing
your `application_management_token` and the body.
Our [backend SDKs](/api-reference/api-sdks) have helper methods that return `true` if the payload is good.
```python Python
Smartcar.verify_payload(
{application_management_token},
{SC-Signature},
{webhook_body}
)
```
```js Node
smartcar.verify_payload(
{application_management_token},
{SC-Signature},
{webhook_body}
);
```
```java Java
Smartcar.verifyPayload(
{application_management_token},
{SC-Signature},
{webhook_body}
);
```
```ruby Ruby
Smartcar.verify_payload(
{application_management_token},
{SC-Signature},
{webhook_body}
)
```
# Subscribe
Source: https://smartcar.com/docs/api-reference/webhooks/subscribe-webhook
POST /vehicles/{id}/webhooks/{webhook_id}
Subscribe a vehicle to a webhook.
## Request
**Path**
The webhook id you are subscribing the vehicle to. This can be found in Dashboard under Webhooks.
## Response
The [vehicle id](/api-reference/all-vehicles) of the vehicle you are making a request to.
The webhook id you are subscribing the vehicle to.
```json ResponseExample
{
"vehicleId": "dc6ea99e-57d1-4e41-b129-27e7eb58713e",
"webhookId": "9b6ae692-60cc-4b3e-89d8-71e7549cf805"
}
```
# Unsubscribe
Source: https://smartcar.com/docs/api-reference/webhooks/unsubscribe-webhook
DELETE /vehicles/{id}/webhooks/{webhook_id}
Unsubscribe a vehicle from a webhook.
## Request
**Header**
In the format `Bearer {application_management_token}`. You can find your `application_management_token` under
your Application Configuration in Dashboard.
**Path**
The [webhook id](api-reference/management/all-vehicles) you are unsubscribing the vehicle from.
## Response
Status of the request.
```json Example Response
{
"status": "success"
}
```
# 2022 Releases
Source: https://smartcar.com/docs/changelog/2022
## January 5, 2022
Smartcar is now compatible with Mercedes-Benz vehicles in the United States.
## February 23, 2022
The EV Battery and EV Charging status and control endpoints are now available for Lexus and Toyota vehicles in Canada and the United States.
## February 21, 2022
The Tire Pressure endpoint is now available for Lexus and Toyota vehicles in Canada and the United States.
## February 14, 2022
Smartcar is now compatible with Chrysler, Dodge, Jeep, and RAM vehicles in Canada.
## February 2, 2022
Brand-specific endpoints are now available for Cadillac, Chevrolet, and Tesla. A full list of the available endpoints is available in the API Reference.
```Node Node
const amperage = vehicle.request('GET', 'tesla/charge/ammeter');
```
```Python Python
amperage = vehicle.request("GET", "tesla/charge/ammeter")
```
```Java Java
SmartcarVehicleRequest request = new SmartcarVehicleRequest.Builder()
.method("GET")
.path("tesla/charge/ammeter")
.build();
VehicleResponse amperage = vehicle.request(request);
```
```Ruby Ruby
amperage = vehicle.request("GET", "tesla/charge/ammeter")
```
## March 7, 2022
Smartcar is now compatible with MINI vehicles in Canada, the United States, and our supported European countries.
## April 13, 2022
Smartcar's webhooks now have beta support for sending events in response to events that are generated by the vehicle itself. The initial release supports the following events on Ford, Tesla, and Toyota vehicles in Canada, the United States, and our supported European countries:
* `CHARGING_STARTED`
* `CHARGING_STOPPED`
* `CHARGING_COMPLETED`
Webhook events sent in response to vehicle events can be distinguished from schedule events based on the `eventName` property of the POST body. For example, the POST body for event based webhooks will have the following structure:
```json
{
"version": "2.0",
"webhookId": "uuid",
"eventName": "eventBased",
"mode": "test|live",
"payload": {
"eventId": "uuid",
"vehicleId": "uuid",
"eventType": "CHARGING_STARTED|CHARGING_STOPPED|CHARGING_COMPLETED",
"eventTime": "ISO8601 Datetime"
}
}
```
## May 9, 2022
Smartcar is now compatible with Kia vehicles in our supported European countries.
## May 5, 2022
The API now returns more detailed errors for the following:
`UPSTREAM:RATE_LIMIT` - a request fails due to a vehicle rate limit.
`VEHICLE_STATE:NOT_CHARGING` - a vehicle is not charging (only applies to endpoints that return details about a specific charging session e.g. Charging Completion Time).
## June 29, 2022
Smartcar is now compatible with Peugeot and Opel vehicles in our supported European countries.
## June 22, 2022
The Tire Pressure endpoint is now available for BMW, MINI, and Tesla vehicles in Canada, the United States, and our supported European countries.
## June 15, 2022
The API now returns a more detailed VEHICLE\_STATE error whenever a charge request fails due to charger issues or charging schedules. Click the following link to learn more:
VEHICLE\_STATE:CHARGE\_FAULT
## June 8, 2022
Smartcar is now compatible with Nissan vehicles in our supported European countries.
## July 20, 2022
Smartcar is now compatible with Citroën, DS, and Vauxhall vehicles in our supported European countries.
## July 6, 2022
The following brand-specific endpoints are now available for Tesla vehicles in Canada, the United States, and our supported European countries:
* GET `/tesla/compass`
* GET `/tesla/speedometer`
## August 24, 2022
Smartcar is now compatible with Rivian vehicles in the United States.
## August 3, 2022
Smartcar is now compatible with Škoda vehicles in our supported European countries.
The EV Charging control endpoint is now available for Hyundai vehicles in the United States.
The EV Battery Capacity endpoint is now available in our supported European countries.
## September 22, 2022
The following brand-specific endpoint is now available for Tesla vehicles in Canada, the United States, and our supported European countries:
* POST /tesla/charge/ammeter
## November 30, 2022
Smartcar is now compatible with Kia vehicles in Canada and the United States.
## December 29, 2022
The Location endpoint is now available for Volkswagen ID series vehicles in our supported European countries.
# 2023 Releases
Source: https://smartcar.com/docs/changelog/2023
## January 6, 2023
Smartcar API v1.0 has been sunset and is no longer supported. If you are currently using v1.0 for API requests, webhooks, errors, or compatibility, we recommend switching to v2.0 as soon as possible.
## March 16, 2023
The API now returns a new code for the RATE\_LIMIT error type if your application tries to make a request to a vehicle too frequently. Click the following link to learn more:
[RATE\_LIMIT:VEHICLE](https://smartcar.com/docs/errors/v2.0/rate-limit/#vehicle)
## March 14, 2023
Smartcar has revamped the Connect flow UI and backend to improve error handling and optimize user conversion rates. Our updates fall into 3 categories: improving brand selection, expanding login methods, and establishing new error codes specific to Connect. These include:
1. Allowing a user to search for:
a. Brand aliases in Brand Select such as VW for Volkswagen
b. Unavailable brands that we plan to introduce in the future, which will display a message highlighting future compatibility with the chosen brand.
2. Expanding login method coverage to include phone numbers for certain brands. We now allow users to log in using email or phone number for the Connect flow starting with Kia and Mercedes-Benz. Stay tuned to our changelog for more brand updates.
3. Providing clearer errors for the Connect flow:
a. no\_vehicles for when the user does not have any vehicles tied to their account and they click to go back to the application
b. configuration\_error for when exiting Connect back to your app through an error page (see below).
c. server\_error which is thrown if there is not another Connect error specified.
More documentation on error handling can be found [here](https://smartcar.com/docs/integration-guide/test-your-integration/test-errors/#1-connect-errors).
## March 6, 2023
The disconnect endpoint now supports the use of management API token (MAT) for authorization purposes. Documentation can be found [here](https://smartcar.com/docs/api#delete-disconnect)
## May 10, 2023
Smartcar is now compatible with Infiniti vehicles in the United States.
## June 7, 2023
Smartcar is now compatible with Hyundai vehicles in Canada.
## June 5, 2023
Test mode supports the following email format with any password to generate an account with multiple vehicles:
`-vehicles@smartcar.com`
## July 26, 2023
The following brand-specific endpoints are now available for Tesla vehicles in Canada, the United States, and our supported European countries:
* GET `/tesla/charge/records`
* GET `/tesla/charge/records/billing`
## July 19, 2023
Smartcar is now compatible with Mercedes-Benz vehicles in our supported European countries.
## July 12, 2023
The following brand-specific endpoints are now available for Tesla vehicles in Canada, the United States, and our supported European countries:
* GET `/tesla/charge/schedule`
* POST ` /tesla/charge/schedule`
* GET `/tesla/climate/cabin`
* POST ` /tesla/climate/cabin`
* GET `/tesla/climate/defroster`
* POST ` /tesla/climate/defroster`
* GET `/tesla/climate/steering_wheel`
* POST ` /tesla/climate/steering_wheel`
## August 22, 2023
The following brand-specific endpoints are now available for Nissan vehicles in the United States:
* GET /nissan/charge/schedule
* PUT /nissan/charge/schedule
## August 17, 2023
The following endpoints are now available to manage vehicle connections:
* GET Vehicle Connections
* DELETE Vehicle Connections
## August 9, 2023
The following brand-specific endpoints are now available for Ford and Lincoln vehicles in Canada, the United States, and our supported European countries:
* GET ford/charge/schedule\_by\_location
* PUT ford/charge/schedule\_by\_location
* GET lincoln/charge/schedule\_by\_location
* PUT lincoln/charge/schedule\_by\_location
## September 21, 2023
Introducing Vehicle Management! With this initial release you're able to see and manage
vehicles connected to your applications from within the [Smartcar Dashboard](https://dashboard.smartcar.com/login).
Logos and brand names are for identification purposes only and do not
indicate endorsement of or affiliation with Smartcar.
## September 19, 2023
[Charge Records](/api-reference/bmw/get-charge-records) is now available as a make specific endpoint for BMW and MINI in Canada, the United States, and our supported European countries.
## September 06, 2023
Lock Status is now available as a Core endpoint and supported by the following makes:
* Tesla (Global)
* Ford (Global)
* Kia (Global)
* Jaguar (Global)
* Land Rover (Global)
* BMW (Global)
* MINI (Global)
* Lincoln (US)
* Toyota (US)
* Lexus (US)
In addition to the lock status of the vehicle, Smartcar will also return the open status of doors, sunroof, windows, based on what the vehicle supports.
## October 19, 2023
BMW and MINI EVs are now compatible with the following endpoints globally:
* [Get Charge Limit](/api-reference/evs/get-charge-limit)
* [Set Charge Limit](/api-reference/evs/set-charge-limit)
## October 5, 2023
Smartcar is now compatible with Hyundai in supported European countries.
## October 3, 2023
Smartcar is now compatible with Mazda and Porsche in supported European countries.
## November 15, 2023
Smartcar is now compatible with CUPRA in supported European countries.
# 2024 Releases
Source: https://smartcar.com/docs/changelog/2024
## Fetched At Header
A new [SC-Feched-At](https://smartcar.com/docs/api-reference/headers) header is now available. This new header highlights when Smartcar fetched the returned data from an OEM whereas `sc-data-age` indicates when the returned data was recorded by the vehicle.
## Connect Playground
The [Connect Playground](https://smartcar.com/docs/getting-started/dashboard/playground) is available, making it easier than ever to create your Connect URL and start requesting vehicle consent.
## Diagnostic Webhook
A new [Diagnostic Webhook](https://smartcar.com/docs/getting-started/tutorials/webhooks-diagnostic) is available in beta. This webhook delivers either [Diagnostic Trouble Code](https://smartcar.com/docs/api-reference/get-dtcs) events or [System Status](https://smartcar.com/docs/api-reference/get-system-status) changes.
## Diagnostic Trouble Codes
A new [Diagnostic Trouble Code](https://smartcar.com/docs/api-reference/get-dtcs) endpoint is available in beta. This endpoint returns a list of active Diagnostic Trouble Codes (DTCs) and the timestamp they last became active.
## Ford authentication update
Ford's authentication process has been updated to require the use of SDKs. Our [Connect SDKs](https://smartcar.com/docs/connect/connect-sdks) have been updated to redirect to Ford's site to handle the authentication process when the user logs in.
## System Status endpoint now in Beta
A new [System Status](https://smartcar.com/docs/api-reference/get-system-status) endpoint is available in beta. This endpoint returns a list of vehicle components and their health state for FCA and GM makes.
## Webhooks subscription status
The Smartcar Dashboard now indicates which webhooks a vehicle is subscribed to. From the Vehicles table, click into the three dot menu to view vehicle details. From here, navigate to the new Webhooks tab!
## Dynamic Webhooks now in Beta
[Dynamic Webhooks](/getting-started/tutorials/webhooks-dynamic) are now in beta for Enterprise customers! Please request access from the Webhooks tab on the Smartcar Dashboard!
## Multi-Factor Authentication in Dashboard
[Multi-Factor Authentication](/getting-started/dashboard-mfa) is available to offer an additional layer of security for the Smartcar Dashboard.
## Support for MG in Europe
Support for the following endpoints are now available for the MG brand in Europe:
* [VIN](/api-reference/get-vin)
* [Odometer](/api-reference/get-odometer)
* [Lock Status](/api-reference/get-lock-status)
* [Lock/Unlock](/api-reference/control-lock-unlock)
* [Tire Pressure](/api-reference/get-tire-pressure)
* [GET Charge Limit](/api-reference/get-charge-limit)
* [POST Charge Limit](/api-reference/set-charge-limit)
* [Battery Level](/api-reference/get-battery-level)
* [Battery Capacity](/api-reference/get-battery-capacity)
## Support for Honda, Subaru, Acura, and more!
Smartcar is now compatible with the **Honda Prologue**, **Subaru Solterra** and the **Acura ZDX** in supported regions.
### Additional Releases
The following brands now support [Control Charge](/api-reference/control-charge), [Battery Level](/api-reference/get-battery-level), [Battery Capacity](/api-reference/evs/get-battery-capacity) and [Charge Status](/api-reference/get-charge-status):
* Jeep, Fiat and Alfa Romeo (US, Canada and Europe)
* Chevrolet, Cadillac and GMC (US and Canada)
* RAM and Dodge (US and Canada)
* Porsche (US and Europe)
* Mazda (US and Europe)
* Renault (Europe)
* Nissan (Europe)
## Smartcar is now compatible with Dacia in Europe.
Support for the following endpoints are now available for the MG brand in Europe:
* [Vehicle Info](/api-reference/get-vehicle-info)
* [Location](/api-reference/get-location)
* [Control Charge](/api-reference/control-charge)
* [State of Charge](/api-reference/get-battery-level)
* [Charging Status](/api-reference/get-charge-status)
## Connect Insights is now available!
* Each Connect flow launched for the previous two weeks is available in the Smartcar Dashboard with a variety of ways to search for specific Connect launches.
* Enterprise customers have access to funnel analysis so they can understand their conversion rates in the Connect flow.
## Test & Simulated modes are now one
Test and Simulated mode have been combined into a singular mode; Simulated.
If a simulated vehicle is enabled, we will return data following the selected trip. If the simulated vehicle doesn't support the requested endpoint, randomized data will be returned.
If no simulated vehicle is enabled for, we will return data as if a simulated vehicle with "Day Commute" was selected. If the simuday commute doesn't support the requested endpoint, randomized data will be returned.
## Support for Google authentication in Dashboard
The Smartcar Dashboard now supports Google authentication.
## User information for Tesla
The following endpoints are now available for Tesla:
* [Get User Info](/api-reference/tesla/get-user-info)
* [Get User access](/api-reference/tesla/get-user-access)
## Customize brands in Connect
You can now customize which bands show up in Connect from the [Dashboard](https://dashboard.smartcar.com/). Easily manage which brands your users can connect to based on engine type or endpoint.
## Get charge endpoint for Audi
The following Brand Specific Endpoint is now available for Audi:
* [Get Charge Status](/api-reference/audi/get-charge)
## Additional endpoints for Audi
The following endpoints are now available for Audi:
* [Get Charge Limit](/api-reference/evs/get-charge-limit)
* [Set Charge Limit](/api-reference/evs/set-charge-limit)
* [Control Charge](/api-reference/evs/control-charge)
* [Lock Status](/api-reference/get-lock-status)
## Service history
The following endpoint is now available for Ford, Lincoln, Toyota, Lexus, Mazda and Volkswagen (US) vehicles:
* [GET /service/history](/api-reference/get-service-records)
## Tesla alerts
The following make specific endpoint is now available for Tesla across all supported regions:
* [GET /tesla/alerts](/api-reference/tesla/get-alerts)
## Tesla charge battery and suggested user messages
The following make specific endpoints are now available for Tesla across all supported regions:
* [GET /tesla/charge](/api-reference/tesla/get-charge)
* [GET /tesla/battery](/api-reference/tesla/get-battery)
### Additional Releases
Suggested user messages are now included as part of API error responses for you to easily surface resolution steps to vehicle owners. See our [error documentation](/errors/overview) for more details.
## Country management for Smartcar Connect
Country Management is now available for Connect! Easily manage what countries are enabled for your application on Dashboard.
### Additional Releases
The `user` parameter is now available when [building the Connect URL](/connect/redirect-to-connect) to pass a unique identifier for a vehicle owner to track and aggregate analytics across their Connect sessions for your application.
## Organization access for teams
Organization access for Teams is now available on Dashboard. Check out our docs page on [Teams](/help/teams) for details.
### Additional Releases
The following endpoint is now available for Tesla vehicles globally:
* [Virtual Key Status](/api-reference/tesla/get-virtual-key-status)
## Subaru US
Smartcar is now compatible with Subaru in the US.
### Additional Releases
The following endpoints are now available for Tesla vehicles globally:
* [Control Charge Port](/api-reference/tesla/control-charge-port)
* [Control Frunk](/api-reference/tesla/control-frunk)
* [Control Trunk](/api-reference/tesla/control-trunk)
## Fuel endpoint for European brands
The [GET /fuel](/api-reference/get-fuel-tank) endpoint is now available for the following brands in supported European countries:
| | | | |
| ----------------------------- | ----------------------------- | ----------------------------- | ----------------------------- |
| Audi | Hyundai | Mercedes-Benz | Skoda |
| BMW | Jaguar | MINI | Vauxhall |
| Citroen | Kia | Opel | Volkswagen |
| DS | Land Rover | Peugeot | Volvo |
| Ford | Mazda | Renault | |
# Latest Releases
Source: https://smartcar.com/docs/changelog/latest
Learn about Smartcar's latest product updates and improvements
## Rivian Commands Now Available!
You can now use the Smartcar API to send commands—such as lock, unlock, and start/stop charge—to Rivian vehicles!
When requesting command permissions (like `control_security` or `control_charge`), Rivian owners will be prompted to pair their phone with their vehicle. After successful pairing, your application will be able to issue commands to the connected Rivian.
For step-by-step instructions, see our [Rivian Bluetooth Pairing guide](/connect/other-actions/rivian-bluetooth-pairing).
> **Note:** Data permissions (such as `read_location` or `read_charge`) do not require Bluetooth pairing.
## Hyundai Lock and Unlock now available!
You can now lock and unlock Hyundai vehicles using the same standard Smartcar API. This new feature enables remote control of vehicle doors for supported Hyundai models, making it easier to build secure and convenient experiences for your users.
For more details on how to use this capability, see our [API reference](/api-reference/control-lock-unlock).
## Webhook Logs Now Available in Dashboard
You can now view logs for your webhooks directly in the Smartcar Dashboard, alongside your API request logs. This update gives you greater visibility into webhook deliveries, making it easier to monitor, debug, and ensure successful integrations.
To see your webhook logs in action, head over to the [Smartcar Dashboard](https://dashboard.smartcar.com/logs?tab=webhooks).
## New Dashboard Overview Page
Our new Dashboard overview page offers a comprehensive snapshot of your application's performance at a glance. Now, you can quickly visualize:
* Total vehicle connections
* New connections in the last 7 days
* Request trends over time
* Conversion rates
* Top five vehicle brands connected to your application
This update is designed to help you monitor key metrics effortlessly.
## Virtual Keys installation Are Now Supported in Connect
Smartcar now provides detailed steps for adding and managing Virtual Keys during the connection flow. Virtual Keys are required for third-party applications to issue commands to Tesla vehicles and are the preferred method for accessing data.
For more details, visit the [Virtual Key documentation](/help/oem-integrations/tesla/virtual-key-tesla).
## atHome signal is now available!
Smartcar now supports a new vehicle signal `atHome` that returns true or false if a vehicle is at the configured home location. This signal is currently available for Tesla vehicles capable of streaming via [this endpoint](/api-reference/tesla/get-ext-vehicle-info).
## Our New Support Features Are Live!
We’re excited to share that our new support experience is now available. These updates are designed to make it easier and faster to get the help you need through self-service tools, AI-powered assistance, and improved visibility into your support history.
**Customer Support Portal** *(Available to paid customers via the Smartcar Dashboard)*
Your new central hub for all support-related needs:
* Browse the **Knowledge Base** for helpful articles, guides, and troubleshooting tips
* Submit new support requests through a user-friendly form
* View and update **open tickets**, track past communications, and receive timely updates
* Get help fast with our **AI-powered chat agent**, available 24/7
**Enhanced Slack Support** *(For eligible plans)*
Our upgraded Slack support includes:
* AI-powered answers to common questions directly in your Slack channel
* Ability to create and track support tickets without leaving Slack
* One-click access to the Customer Support Portal
* Real-time updates and faster response workflows
For more information about what’s included in your current plan, please reach out to your account manager or contact us at **[support@smartcar.com](mailto:support@smartcar.com)**.
For step-by-step instructions on how to use the new features, check out our documentation [here](https://smartcar.com/docs/help/accessing-support-center).
## Support for Single Sign-on (SSO)
Single Sign-On (SSO) is now available for teams on an Enterprise plan. To get
started, reach out to your account manager to have it enabled. For
implementation details and setup instructions, check out our [SSO
documentation](/getting-started/dashboard/single-sign-on).
## User Selected Battery Capacity
Developers can now redirect vehicle owners to a Smartcar Connect url where they can select the [battery capacity](/api-reference/get-nominal-capacity) of their vehicle for cases where the battery capacity cannot be accurately deteremined. This can occur when vehicle owners purchase extension packs, or software upgrades specific to their vehicle. When a user selects an option, Smartcar will return this value with `USER_SELECTED` as the source.
## Diagnostics Webhooks, DTSs, and System Status
Smartcar now provides Diagnostic Webhooks, DTCs, and System Status vehicle data for an [initial set of brands](https://smartcar.com/product/compatible-vehicles) with more brands added in the future.
## Support for Simplified Chinese
Vehicle owners can now select Simplified Chinese as they go through the Smartcar Connect flow to connect their cars.
## Tesla Telemetry API
Smartcar natively supports Tesla's Telemetry API as the default mechanism for retrieving data from Tesla vehicles out of the box and without any configuration from developers. Tesla's Telemtry API is the most efficient and effective way of gathering data from Tesla vehicles. It allows vehicles to stream data directly to Smartcar, eliminating the need to poll Tesla servers. This prevents unnecessary vehicle wakes and battery drain.
## Smartcar MFA
When a vehicle owner attempts to login to their OEM Connected Services Account and they do not have MFA enabled, Smartcar customers can enable this feature to enforce MFA verification for extra security. If you don't see this option in the Smartcar dashboard, contact [support@smartcar.com](mailto:support@smartcar.com) to get access to this feature.
## New Billing page in Dashboard
This new page provides more visibility and the ability to update your billing information, view past invoices, and view your plan features and available upgrade options.
# Country Selection
Source: https://smartcar.com/docs/connect/advanced-config/country-flag
By default Connect will launch based on the devices location impacting the brands that are available to the user e.g. Renault is only available in Europe.
You can override this behaviour by passing a country feature flag in your Connect URL in the form `country:{country_code}`.
Your feature flag should contain the [two-character ISO country code](https://en.wikipedia.org/wiki/ISO_3166-1_alpha-2)
of the country that your user is located in.
Our SDKs provide ways for you to add this as part of the the various `authUrlBuilder` or `getAuthUrl` methods.
```plaintext Connect URL w/ country feature flag
https://connect.smartcar.com/oauth/authorize?
response_type=code
&client_id=8229df9f-91a0-4ff0-a1ae-a1f38ee24d07
&scope=read_odometer read_vehicle_info
&redirect_uri=https://example.com/home
&flags=country:GB
```
Alternatively, your users can manually change their country and language on Connect's preamble screen:
# Connect Flows
Source: https://smartcar.com/docs/connect/advanced-config/flows
Connect can be launched with three different workflows. Depending on your use case and what information you have about the vehicle ahead of a launching Connect, you may be able to leverage one of these flows for a more streamlined Connect experience.
When you launch Connect, users will be able to select the brand of their vehicle from a list before they enter their credentials and grant access to your application.
In some cases you may only want to connect to a single vehicle, even if there are more than one on your user's connected services account. Smartcar provides two ways for you to do this:
* Single Select
* Single Select with VIN
### Single Select
Limits the user's selection on the permissions screen to a single car if there are multiple vehicles on their connected services account. Notice that check-boxes turn into radio buttons, and the call to action wording changes slightly.
To enable Single Select, you can pass `single_select=true` as URL parameter when launching Smartcar Connect.
### Single Select with VIN
In addition, if you know the user’s VIN ahead of time you can pass this over to us in the connect URL. In doing so:
1. Smartcar decodes the VIN to get the brand and send the user to the appropriate login form directly
2. If the owner has more than one vehicle on their connected services account, we’ll only show the VIN that was passed to us on the permission grant screen.
To enable Single Select with VIN, you can pass `single_select=true` and `single_select_vin=:vin` as URL parameters when launching Smartcar Connect.
### Brand Select
Instead of having users go through the brand selector screen, you can pass us a brand in the Connect URL and send the user to the appropriate login form directly .
To enable Brand Select, you can pass `make` as URL parameter when launching Connect. For example, `make=TESLA`
# Modes
Source: https://smartcar.com/docs/connect/advanced-config/modes
If no mode is specified, Connect will launch in `live` mode by default.
| Mode | Description |
| --------- | -------------------------------------------------------------------------------------------------------------------- |
| live | Launches Connect in live mode allowing a user to login in with a real connected services account. |
| simulated | Launches Connect in simulated mode allowing developer to connect to vehicles created on Dashboard via the Simulator. |
# Auth Code Exchange
Source: https://smartcar.com/docs/connect/auth-code-exchange
To interact with the Smartcar API, you will need to exchange your authorization code for an access token. The authorization code represents a user’s consent, but cannot be used to make requests to a vehicle. Instead, it must be exchanged for an access token. An example request is provided to the right.
# SDKs for Connect
Source: https://smartcar.com/docs/connect/connect-sdks
Our SDKs make integrating Smartcar fast and easy in different languages and frameworks.
For mobile or single page web apps, you can use one of our frontend SDKs. Please note that our frontend SDKs only handle
integrating Connect into your application. You will still need to use a backend SDK to manage tokens and make API requests to vehicles.
For server-side rendered applications, you can use one of our backend SDKs:
Don't see an SDK for your language? No problem!
As long as you can build a URL and handle http requests, you're good to go.
# Dashboard Configuration
Source: https://smartcar.com/docs/connect/dashboard-config
## Registration
To get started, register your application with Smartcar by navigating to our [dashboard](https://dashboard.smartcar.com/login).
After registration, your application will be assigned a `CLIENT_ID` and a `CLIENT_SECRET`. The `CLIENT_SECRET` must be kept safe and used only in exchanges between your application’s server and Smartcar’s authorization server.
## Redirect URIs
To authorize with Smartcar, you’ll need to provide one or more redirect URIs. The user will be redirected to the specified URI upon authorization. On redirect, the URI will contain an authorization `code` query parameter that must be exchanged with Smartcar’s authorization server for an `ACCESS_TOKEN`.
The redirect URIs must match one of the following formats:
| Protocol | Format | Example |
| -------------- | ---------------------------------------------------------------------------------------- | ------------------------------------------------------------------------------ |
| HTTP | a localhost URI with protocol `http://` | `http://localhost:8000` |
| HTTPS | a URI with protocol `https://` | `https://myapplication.com` |
| JavaScript SDK | `https://javascript-sdk.smartcar.com/v2/redirect?app_origin={localhost-or-HTTPS-origin}` | `https://javascript-sdk.smartcar.com/v2/redirect?app_origin=https://myapp.com` |
| Custom-scheme | `sc{clientId}://{hostname-with-optional-path-component-or-TLD}` | `sc4a1b01e5-0497-417c-a30e-6df6ba33ba46://callback` |
HTTP is allowed only for testing purposes on localhost
### Javascript SDK
The JavaScript SDK redirect is used along with the JavaScript SDK library. For more details and examples on the redirect usage, see the [SDK documentation](https://github.com/smartcar/javascript-sdk).
### Custom-scheme
Custom-scheme URIs are used for mobile applications. They must begin with `sc{clientId}` and can have an optional path or TLD. See the OAuth reference on redirect URIs.
# Handle the Response
Source: https://smartcar.com/docs/connect/handle-the-response
Upon successfully accepting the permissions, Smartcar will redirect the user back to your application using the specified `REDIRECT_URI`, along with an authorization code as a query parameter. In the case of on error, we'll provide an error and description as parameters instead.
## Success
An authorization code used to obtain your initial `ACCESS_TOKEN`. The auth `code` expires after **10 minutes**.
```http Success
HTTP/1.1 302 Found
Location: https://example.com/home?
code=90abecb6-e7ab-4b85-864a-e1c8bf67f2ad
&state=0facda3319
```
## Error
For a detailed description of these errors, please see our [errors page](/errors/overview).
The type of error
A detailed description of what caused the error
```http Error
HTTP/1.1 302 Found
Location: https://example.com/home?
error=access_denied
&error_description=User+denied+access+to+application.
&state=0facda3319
```
In addition to the error code and description, Smartcar will return the following parameters when a
user tries to authorize an incompatible vehicle in Connect.
Can be returned for errors where the vehicle is incompatible.
The manufacturer of the vehicle.
The model of the vehicle.
The year of production of the vehicle.
# Rivian Bluetooth Pairing
Source: https://smartcar.com/docs/connect/other-actions/rivian-bluetooth-pairing
To support vehicle commands such as lock/unlock or start/stop charge, Rivian requires a mobile device to be paired via Bluetooth with the vehicle.
If permissions for commands are requested in your Connect URL (i.e. `control_security`, `control_charge`), Rivian owners will be prompted to pair their phone to the vehicle to complete the connection. After successful login, users will see a list of vehicles within their Rivian account to pair to. It is important the the mobile phone has Bluetooth enabled at this time.
After selecting the vehicle, the user will be prompted to tap “Set up now” on the Rivian vehicle’s display and follow any on-screen prompts to connect.
Once setup is complete, the application will be able to issue commands to the Rivian vehicle.
Should Bluetooth be disabled or the Rivian display prompts not followed, the Bluetooth connection will not be established and the vehicle will not be connected to your application.
## Notes
* Rivian vehicles must be paired via Bluetooth to support commands. Data permissions (i.e. `read_location`, `read_charge`) do not require Bluetooth pairing.
* The following commanads are supported for Rivian vehicles: lock/unlock, start/stop charge, and set charge limit.
* If a user has multiple Rivian vehicles, they will be prompted to pair each vehicle that they wish to connect to your application.
* At least one vehicle must be paired via Bluetooth to complete the pairing process before the user can continue with the Connect flow and connect their Rivian to your application.
* If there are multiple drivers associated with a Rivian account and both drivers want to connect to your application, each driver must pair their mobile device to the vehicle via Bluetooth.
* There is a limit of four devices that can be paired to a Rivian vehicle at one time. If a user has already paired four devices to their Rivian, they will need to unpair one of the existing devices from the vehicle before they can pair their mobile device and complete the connection to your application.
# Tesla Virtual Keys
Source: https://smartcar.com/docs/connect/other-actions/tesla-virtual-key
To support vehicle commands such as lock/unlock or start/stop charge, and benefit from faster data frequencies, Tesla requires a Virtual Key to be added to the vehicle.
For more information about Virtual Keys, please see visit the [Virtual Key page](/help/oem-integrations/tesla/virtual-key-tesla).
### Vehicle Owners adding a Virtual Key
Smartcar Connect will present Tesla vehicle owners a prompt to install the Tesla Virtual Key after granting access and prior to redirecting them back to your application.
When users open the virtual key link, depending on their device, they will be redirected to the Tesla app or prompted to scan a QR code.
On mobile devices, they will be redirected to the Tesla app and prompted to add the Virtual Key
On Desktop, they will be prompted to scan the QR code which in turn opens up the Tesla app and prompts them to add a Virtual Key
Virtual Key status can be checked at any time from the Tesla infotainment screen under Settings, Locks.
Smartcar will automatically determine which Tesla vehicles require a Virtual Key and prompt users to add it only for those vehicles. If a user has multiple Tesla vehicles, they will be prompted to add a Virtual Key for each vehicle that requires it. At least one vehicle complete the pairing process before the user can continue with the Connect flow and connect their Tesla to your application.
# Handle the Response
Source: https://smartcar.com/docs/connect/re-auth/handle-response
If the re-auth is successful, the redirect to your application will contain a vehicle ID. In the case of an error, we'll provide an error and description as parameters instead.
## Success
Unique identifier for a vehicle on Smartcar’s platform.
```http Success
HTTP/1.1 302 Found
Location: https://example.com/home?
vehicle_id=sc4a1b01e5-0497-417c-a30e-6df6ba33ba46
&state=0facda3319
```
## Error
For a detailed description of these errors, please see our [errors page](/errors/overview).
The type of error
A detailed description of what caused the error
```http Error
HTTP/1.1 302 Found
Location: https://example.com/home?
error=access_denied
&error_description=User+denied+access+to+application.
&state=0facda3319
```
# Redirect to Connect
Source: https://smartcar.com/docs/connect/re-auth/redirect-to-connect
There will be times when a user updates credentials to their connected services account. When this happens, the user will need to go through Connect
again to update your authorization through Smartcar.
You can do this with a streamlined Connect flow using a special re-auth URL with the parameters below.
The [`AUTHENTICATION_FAILED`]() API error contains a partially constructed re-authentication URL in the `resolution.url` field.
The application’s unique identifier.
The URI a user will be redirected to after authorization. This value **must** match one of the redirect URIs set in the credentials tab of the dashboard.
This value must be set to `vehicle_id`.
The id of the vehicle you are reauthenticating.
An optional value included as a query parameter in the redirect\_uri back to your application. This value is often used to identify a user and/or prevent cross-site request forgery
Specify a unique identifier for the vehicle owner to track and aggregate analytics across Connect sessions for each vehicle owner on Dashboard.
Note: Use the `state` parameter in order to identify the user at your callback URI when receiving an authorization or error code after the user exits the Connect flow.
```http Connect URL Example
HTTP/1.1 302 Found
Location: https://connect.smartcar.com/oauth/reauthenticate?
response_type=vehicle_id
&client_id=8229df9f-91a0-4ff0-a1ae-a1f38ee24d07
&vehicle_id=sc4a1b01e5-0497-417c-a30e-6df6ba33ba46
&redirect_uri=https://example.com/home
&state=0facda3319
```
# Build the Connect URL
Source: https://smartcar.com/docs/connect/redirect-to-connect
Construct the Smartcar Connect redirect with the parameters below. Launching this link will send users through the Smartcar Connect flow.
You can build the Connect URL directly from the [Smartcar Dashboard](https://dashboard.smartcar.com/connect/playground)!
[](https://dashboard.smartcar.com/connect/playground)
For mobile or single-page web applications you can use one of our [frontend SDKs](/connect/connect-sdks), or for server-side rendered applications you can use one of
our [backend SDKs](/connect/connect-sdks).
Alternatively, you can build the Connect URL manually by passing the following parameters:
{/*
You can use the `required:` prefix for `permissions` in the `scope` parameter to help filter out vehicles
on your users connected services account. You can read more [here](/api-reference/permissions#required-permissions).
*/}
The application’s unique identifier. This is available on the credentials tab of the dashboard
The URI a user will be redirected to after authorization. This value must match one of the redirect URIs set in the credentials tab of the dashboard
This value must be set to `code`. OAuth2 outlines multiple authorization types. Smartcar Connect utilizes the “Authorization Code” flow.
A space-separated list of permissions that your application is requesting access to. The valid permission names can be found in the permissions section. A permission is optional by default. It can be made required by adding the required: prefix to the permission name, e.g. required:read\_odometer. Please refer to the integration guide in our docs for more information.
An optional value included as a query parameter in the `REDIRECT_URI` back to your application. This value is often used to identify a user and/or prevent cross-site request forgery
An optional value that sets the behavior of the approval dialog displayed to the user.
Defaults to `auto` and will only display the approval dialog if the user has not previously approved the scope.
Set this to `force` to ensure the approval dialog is always shown to the user even if they have previously approved the same scope.
Simulated mode lets you make requests to a vehicle created using the Vehicle Simulator to receive realistic responses from specific makes, models, years, and various states of the vehicle.
Should the selected simulator not support the desired endpoint randomized data will be returned instead.
Live mode should be used when connecting to a real vehicle.
Allows users to bypass the Brand Selector screen. Valid `makes` can be found in the [makes](/api-reference/makes) section on API reference.
The `single_select_vin` parameter takes precedence over this parameter.
Sets the vehicle selection behavior of the grant dialog. If set to true, then the user is only allowed to select a single vehicle. Please refer to the Single Select section for more information.
Sets the behavior of the permissions screen in Smartcar Connect.
When using `single_select_vin`, you need to pass in the VIN (Vehicle Identification Number) of a specific vehicle into the `single_select_vin` parameter.
Additionally, you need to set the `single_select` parameter to `true`.
Smartcar Connect will then let the user authorize **only the vehicle with that specific VIN**.
The `single_select_vin` parameter takes precedence over the `make` parameter.
Please refer to the Single Select section for more information.
A space separated list of feature flags in the form `{flag}:{value}`.
The two-character ISO country code of the country that your user is located in. You can set the default country for Connect on Dashboard
If no flag is passed or set as default on Dashboard, Smartcar will use the devices IP to set an appropriate country.
This flag determines what brands are listed on the Brand Selector screen in Connect e.g. Renault is available in Europe, but not the US.
Disable remembering a user's authorization on subsequent login sessions. This feature is enabled by default and can only be disabled (by setting the parameter to false) in simulated mode.
Specify a unique identifier for the vehicle owner to track and aggregate analytics across Connect sessions for each vehicle owner on Dashboard.
Note: Use the `state` parameter in order to identify the user at your callback URI when receiving an authorization or error code after the user exits the Connect flow.
```http Example Connect URL
HTTP/1.1 302 Found
Location: https://connect.smartcar.com/oauth/authorize?
response_type=code
&client_id=8229df9f-91a0-4ff0-a1ae-a1f38ee24d07
&scope=read_odometer read_vehicle_info read_location
&redirect_uri=https://example.com/home
&state=0facda3319
```
# Overview
Source: https://smartcar.com/docs/connect/token-management/overview
After fetching your first token pair, you'll need to manage them in order to maintain access to the vehicle.
## Storing access tokens
### Default and Brand Select Connect Flow
By default tokens are scoped to the user's connected services account.
This means that if there are multiple vehicles
on the account - and they are selected at the time of authorization, the access token is valid for all those vehicle Ids.
To manage this, we recommend using the [Smartcar User Id](/api-reference/management/user) to link tokens to your corresponding user id. The diagram below
will also allow multiple users to connect to vehicles on the same account.
### Single Select Connect Flow
When using the Single Select flow, tokens are strictly scoped to the vehicle that was authorized for that Connect session.
This means that if a user connects multiple vehicles under the same connected services account, each vehicle id will be tied
to its own set of tokens.
## Token expiry
Access tokens are valid for 2 hours, while refresh tokens are valid for 60 days. You can use the corresponding refresh token to fetch a new token pair
once an access token has expired.
In order to maintain access to a vehicle without having a user go through Connect again, you'll want to make sure the refresh token never expires.
Whenever you fetch a new token pair, we will return a **new access and refresh token**.
Prior to expiry, access tokens will remain valid until their expiry when fetching a new token pair. Refresh tokens on the other hand are invalidated
1 minute after they're used.
To avoid common 401 Authentication errors, please ensure you are **persisting both the access and refresh token** we return whenever you fetch a new pair.
In addition to any logic that checks access token expiry when making an API request, we strongly recommend having another job that periodically
checks for refresh tokens that are close to expiry and refreshes them.
# Refreshing Refresh Tokens
Source: https://smartcar.com/docs/connect/token-management/refresh-refresh-token
Refresh tokens expire after **60 days ordinarily** or **after 1 minute when used to fetch a new token pair**.
Depending on your application logic, there may be cases where you don't end up fetching a fresh access token for more than 60 days.
If this is the case, you should fetch a new token pair before the 60 days are up so the user doesn’t need to go through Connect again.
This will give you a newly minted access token **and** refresh token.
# Refreshing Access Tokens
Source: https://smartcar.com/docs/connect/token-management/refreshing-access-token
Your access token will expire **2 hours** after it is issued. When this happens, your application can retrieve a new one by using the corresponding `REFRESH_TOKEN`.
# What is Connect?
Source: https://smartcar.com/docs/connect/what-is-connect
Smartcar Connect is the fastest and most transparent way to collect user consent. Before you can make API requests to a car, your customer needs to link the vehicle to your app. It’s a simple process for your users to enroll their vehicles:
1. Select the car brand
2. Sign in
3. Give consent
From your app, you'll [redirect your users to Connect](/connect/redirect-to-connect), and [handle the response](/connect/handle-the-response) once the user completes or exits the Connect flow. You'll receive a `code` that you'll use to [exchange](/connect/auth-code-exchange) for an access token to start making requests to their vehicle(s).
We have designed Connect in compliance with the OAuth2 authorization protocol, safely handling all user information.
Connect comes with robust configurations to fit your needs and frontend [SDKs](/connect/connect-sdks) for faster integration.
# Authentication Errors
Source: https://smartcar.com/docs/errors/api-errors/authentication-errors
Thrown when there is an issue with your authorization header.
# `NULL`
The authorization header is missing or malformed, or it contains invalid or expired authentication credentials (e.g. access token, client ID, client secret). Please check for missing parameters, spelling and casing mistakes, and other syntax issues.
```json
{
"type": "AUTHENTICATION",
"code": null,
"description": "The authorization header is missing or malformed, or it contains invalid or expired authentication credentials. Please check for missing parameters, spelling and casing mistakes, and other syntax issues.",
"docURL": "https://smartcar.com/docs/errors/api-errors/authentication-errors",
"statusCode": 401,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": null }
}
```
### Suggested Resolution
You can resolve this error by referring to our API reference and ensuring that you pass all the parameters as specified. If you are certain that your request is well-formed, please try refreshing your access token.
### Troubleshooting Steps
Refer to our API reference and ensure that you use the correct authentication mechanism for your request
* Check constants like Bearer and Basic for spelling mistakes.
* If you make a request to a vehicle endpoint, verify that your access token grants you access to the correct vehicle. You can do so by making a request to the /vehicles endpoint and ensuring that the correct vehicle ID is included in the returned response.
* If you have refreshed your access token, make sure that it persists and that you use your new token to make your request.
# Billing Errors
Source: https://smartcar.com/docs/errors/api-errors/billing-errors
Thrown when limits have been reached based on your plan, or if the feature is not avaible.
# `VEHICLE_LIMIT`
You’ve reached the limit of vehicles in your plan. Please upgrade to unlock access to more vehicles.
```json VEHICLE_LIMIT
{
"type": "BILLING",
"code": "VEHICLE_LIMIT",
"description": "You’ve reached the limit of vehicles in your plan. Please upgrade to unlock access to more vehicles.",
"docURL": "https://smartcar.com/docs/errors/api-errors/billing-errors#vehicle-limit",
"statusCode": 430,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": "CONTACT_SUPPORT" },
}
```
### Troubleshooting Steps
To resolve this error, please [contact us](mailto:support@smartcar.com) to upgrade your plan and increase your vehicle limit.
Please note that you won’t be able to make requests to additional vehicles until the end of the current billing period or until you have upgraded your plan.
# `INVALID_PLAN`
The feature you are trying to use is not included in your current pricing plan. Please visit our pricing page to learn more about our plans and features.
```json INVALID_PLAN
{
"type": "BILLING",
"code": "INVALID_PLAN",
"description": "The feature you are trying to use is not included in your current pricing plan. Please visit our pricing page to learn more about our plans and features.",
"docURL": "https://smartcar.com/docs/errors/api-errors/billing-errors#invalid-plan",
"statusCode": 430,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": "CONTACT_SUPPORT" }
}
```
### Troubleshooting Steps
If you have checked our pricing page and believe that the feature you are trying to use is included in your plan,
please [contact us](mailto:support@smartcar.com) and we’ll be happy to assist you.
# `VEHICLE_REQUEST_LIMIT`
You have exceeded the number of API requests that are allowed for **this** vehicle in the current billing period.
Please log in to the [Smartcar dashboard](https://dashboard.smartcar.com/login) and visit the Billing tab to learn more.
```json VEHICLE_REQUEST_LIMIT
{
"type": "BILLING",
"code": "VEHICLE_REQUEST_LIMIT",
"description": "You have exceeded the number of API requests that are allowed for this vehicle in the current billing period. Please log into the Smartcar dashboard and visit the Billing tab to learn more.",
"docURL": "https://smartcar.com/docs/errors/api-errors/billing-errors#vehicle-request-limit",
"statusCode": 430,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": "CONTACT_SUPPORT" }
}
```
### Troubleshooting Steps
To resolve this error, please [contact us](mailto:support@smartcar.com) to upgrade your plan and increase your vehicle limit.
Please note that you won’t be able to make additional requests to this vehicle until the end of the current billing period or until you have upgraded your plan.
# `ACCOUNT_SUSPENDED`
Your Smartcar account is past due and has been suspended.
Please reach out to our finance team to resolve this issue.
```json ACCOUNT_SUSPENDED
{
"type": "BILLING",
"code": "ACCOUNT_SUSPENDED",
"description": "Your Smartcar account is past due and has been suspended. Please reach out to our finance team to resolve this issue.",
"docURL": "https://smartcar.com/docs/errors/api-errors/billing-errors#account-suspended",
"statusCode": 430,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20882a",
"resolution": { "type": "FINANCE" }
}
```
### Troubleshooting Steps
To resolve this error, please [contact us](mailto:finance@smartcar.com) to pay past due invoices.
Please note that you won’t be able to make any requests until you have resolved this issue.
# Compatibility Errors
Source: https://smartcar.com/docs/errors/api-errors/compatibility-errors
Thrown when Smartcar does not support a make, or feature for a vehicle.
# `MAKE_NOT_COMPATIBLE`
Smartcar is not yet compatible with this vehicle’s make in the country you specified.
```json
{
"type": "COMPATIBILITY",
"code": "MAKE_NOT_COMPATIBLE",
"description": "Smartcar is not yet compatible with this vehicle’s make in the country you specified.",
"docURL": "https://smartcar.com/docs/errors/api-errors/compatibility-errors#make-not-compatible",
"statusCode": 501,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": null }
}
```
**Suggested User Messaging**
`` is not yet able to connect to your car brand.
# `PLATFORM_NOT_CAPABLE`
You're connected to the vehicle using an OEM integration that does not support this endpoint. This can be a result of the OEM migrating to a newer API or requiring vehicle owners to migrate to a new application they have released.
```json
{
"type": "COMPATIBILITY",
"code": "PLATFORM_NOT_CAPABLE",
"description": "You're connected to the vehicle using an OEM integration that does not support this endpoint.",
"docURL": "https://smartcar.com/docs/errors/api-errors/compatibility-errors#platform-not-capable",
"statusCode": 501,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": "REAUTHENTICATE" }
}
```
**Troubleshooting Steps**
For Tesla vehicles please have the owner re-authenticate using the latest Tesla authorization flow.
# `SMARTCAR_NOT_CAPABLE`
Smartcar does not yet support this feature for this vehicle.
```json
{
"type": "COMPATIBILITY",
"code": "SMARTCAR_NOT_CAPABLE",
"description": "Smartcar does not yet support this feature for this vehicle.",
"docURL": "https://smartcar.com/docs/errors/api-errors/compatibility-errors#smartcar-not-capable",
"statusCode": 501,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": null }
}
```
**Troubleshooting Steps**
Please check that the vehicles make and engine type support the feature you are trying to use.
Please contact us to learn when this feature will become available.
If you have a vehicle that supports this feature and would like to help out, check out Smartcar’s Research Fleet.
# `VEHICLE_NOT_CAPABLE`
This error occurs when a physical limitation makes the vehicle incapable of performing your request
(e.g. battery electric vehicles are incapable of responding to read fuel requests).
```json
{
"type": "COMPATIBILITY",
"code": "VEHICLE_NOT_CAPABLE",
"description": "The vehicle is incapable of performing your request.",
"docURL": "https://smartcar.com/docs/errors/api-errors/compatibility-errors#vehicle-not-capable",
"statusCode": 501,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": null }
}
```
**Suggested User Messaging**
Your car is unable to perform this request.
# Connected Services Account Errors
Source: https://smartcar.com/docs/errors/api-errors/connected-services-account-errors
Thrown when there are issues with the user's connected service account.
# `ACCOUNT_ISSUE`
Action needed in the user’s connected services account. The user needs to log in and complete an outstanding task in their connected services account.
Examples:
* The user needs to accept the connected services app’s Terms of Service.
* The user has created but not yet fully activated their connected services account.
* If you've received this error while testing with Vehicle Simulator, it likely means a simulation has not been started for the vehicle. Visit the Simulator tab in the Smartcar Dashboard to start a simulation for the vehicle.
```json
{
"type": "CONNECTED_SERVICES_ACCOUNT",
"code": "ACCOUNT_ISSUE",
"description": "Action needed in the user’s connected services account. Please prompt the user to log into their connected services account and complete any outstanding tasks.",
"docURL": "https://smartcar.com/docs/errors/api-errors/connected-service-errors#account-issue",
"statusCode": 400,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": null },
"suggestedUserMessage": "Action required in your connected services account. Please log into your connected services app or web portal and complete any outstanding tasks (e.g. finish activating your account or accept the Terms of Service)."
}
```
### Suggested resolution
The user can resolve this error by logging into their connected services account and completing any outstanding tasks.
### Troubleshooting steps
* Prompt the user to log into their connected services account using the app or web portal.
* Prompt the user to try triggering an action on their car, e.g. locking the doors or flashing the lights.
* At this point, the app should prompt the user to complete their outstanding task.
### Suggested user message
> Action required in your connected services account. Please log into your connected services app or web portal and complete any outstanding tasks (e.g. finish activating your account or accept the Terms of Service).
***
# `AUTHENTICATION_FAILED`
Smartcar was unable to authenticate with the user’s connected services account. This error usually occurs when the user has updated their connected
services account credentials and not yet re-authenticated in Smartcar Connect. The user should re-authenticate using Smartcar Connect.
```json
{
"type": "CONNECTED_SERVICES_ACCOUNT",
"code": "AUTHENTICATION_FAILED",
"description": "Smartcar was unable to authenticate with the user’s connected services account. Please prompt the user to re-authenticate using Smartcar Connect.",
"docURL": "https://smartcar.com/docs/errors/api-errors/connected-service-errors#authentication-failed",
"statusCode": 400,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": {
"type": "REAUTHENTICATE",
"url": "https://connect.smartcar.com/oauth/reauthenticate?response_type=vehicle_id&client_id=8229df9f-91a0-4ff0-a1ae-a1f38ee24d07&vehicle_id=sc4a1b01e5-0497-417c-a30e-6df6ba33ba46",
}
"suggestedUserMessage": "Your car got disconnected from . Please use this link to re-connect your car: ."
}
```
### Suggested resolution
Please provide the user with a link to Smartcar Connect Re-authentication and prompt them to re-connect their vehicle.
The resolution field contains a partially constructed URL for Smartcar Connect Re-authentication, please see the API reference for more detail.
### Suggested user message
> Your car got disconnected from \. Please use this link to re-connect your car: \.
***
# `NO_VEHICLES`
No vehicles found in the user’s connected services account. The user might not yet have added their vehicle to their account, or they might not yet have activated connected services for the vehicle.
```json
{
"type": "CONNECTED_SERVICES_ACCOUNT",
"code": "NO_VEHICLES",
"description": "No vehicles found in the user’s connected services account. Please prompt the user to add their vehicle to their connected services account and re-authenticate using Smartcar Connect.",
"docURL": "https://smartcar.com/docs/errors/api-errors/connected-service-errors#no-vehicles",
"statusCode": 400,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": null }
"suggestedUserMessage": "Your car got disconnected from . Please use this link to re-connect your car: ."
}
```
### Suggested resolution
Please provide the user with a link to Smartcar Connect Re-authentication and prompt them to re-connect their vehicle.
The resolution field contains a partially constructed URL for Smartcar Connect Re-authentication, please see the API reference for more detail.
### Troubleshooting Steps
* Prompt the user to log into their connected services account using the app or web portal.
* Prompt the user to check whether there are any vehicles listed in their account.
* If there are no vehicles listed, prompt the user to add their vehicle.
* If there are one or more vehicles listed, this means that none of the vehicles have had their connected services activated. Prompt the user to activate connected services for at least one vehicle.
* Provide the user with a link to Smartcar Connect and prompt them to re-connect their vehicle.
### Suggested user message
> Your car got disconnected from \. Please use this link to re-connect your car: \.
***
# `PERMISSION`
This error occurs when a vehicle owner had initially granted Smartcar access to their OEM account, but has since revoked it via the OEM's management portal.
```json
{
"type": "CONNECTED_SERVICES_ACCOUNT",
"code": "PERMISSION",
"description": "The vehicle owner has revoked Smartcar’s access to their OEM account.",
"docURL": "https://smartcar.com/docs/errors/api-errors/connected-service-errors#permission",
"statusCode": 400,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": {
"type" : "REAUTHENTICATE"
}
}
```
### Suggested resolution
For Tesla Owners:
* Prompt the vehicle owner to go through the Connect flow again or use our [reauthentication flow](/help/oem-integrations/tesla/developers#stand-alone-flow) to streamline the process.
***
# `SUBSCRIPTION`
The vehicle’s connected services subscription is inactive or does not support the requested API endpoint.
```json
{
"type": "CONNECTED_SERVICES_ACCOUNT",
"code": "SUBSCRIPTION",
"description": "The vehicle’s connected services subscription is inactive or does not support the requested API endpoint.",
"docURL": "https://smartcar.com/docs/errors/api-errors/connected-service-errors#subscription",
"statusCode": 400,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": null },
"suggestedUserMessage": "Your car’s connected services subscription has either expired or it doesn’t support the necessary features to connect to . Please activate your subscription or contact us to upgrade your subscription."
}
```
### Suggested resolution
The user can resolve this error by logging into their connected services account and either (re-)activating their subscription or purchasing the required subscription package.
### Troubleshooting Steps
If you don’t know which subscription package the user needs to purchase, please contact our team for help.
### Suggested user message
> Your car’s connected services subscription has either expired or it doesn’t support the necessary features to connect to \. Please activate your subscription or contact us to upgrade your subscription.
***
# `VEHICLE_MISSING`
This vehicle is no longer associated with the user’s connected services account. The user might have removed it from their account.
The user needs to log into their connected services account and either re-add the vehicle or re-activate its subscription.
If you've received this error while testing with Vehicle Simulator, it likely means the simulated vehicle has not been found in your application. Visit the Simulator tab in the Smartcar Dashboard to create a simulated vehicle to test with.
```json
{
"type": "CONNECTED_SERVICES_ACCOUNT",
"code": "VEHICLE_MISSING",
"description": "This vehicle is no longer associated with the user’s connected services account. Please prompt the user to re-add the vehicle to their account.",
"docURL": "https://smartcar.com/docs/errors/api-errors/connected-service-errors#vehicle-missing",
"statusCode": 400,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": null },
"suggestedUserMessage": "Your car’s connected services subscription has either expired or it doesn’t support the necessary features to connect to . Please activate your subscription or contact us to upgrade your subscription."
}
```
### Suggested resolution
The user can resolve this issue by logging into their connected services account and either re-adding the vehicle or re-activating its subscription.
If the user wishes to disconnect their vehicle from your app, please make a request to our Disconnect endpoint and refrain from making API requests to this vehicle in the future.
### Troubleshooting Steps
* Prompt the user to log into their connected services account using the app or web portal.
* Prompt the user to check whether the vehicle is listed under their account.
* If the vehicle is not listed, prompt the user to add the vehicle to their account.
* If the vehicle is listed, prompt the user to activate the vehicle’s connected services subscription.
### Suggested user message
> This car is no longer associated with your connected services account. Please log into your account and re-add this car.
***
# `VIRTUAL_KEY_REQUIRED`
The vehicle owner has granted your application access to the vehicle through Smartcar. However, additional steps are needed on the owners part in order to perform this specific request.
```json
{
"type": "CONNECTED_SERVICES_ACCOUNT",
"code": "VIRTUAL_KEY_REQUIRED",
"description": "The vehicle owner needs to grant additional access to Smartcar in order to perform this request.",
"docURL": "https://smartcar.com/docs/errors/api-errors/connected-service-errors#virtual-key-required",
"statusCode": 400,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": {
"type" : "ACTION_REQUIRED",
"url" : "https://www.tesla.com/_ak/smartcar.com"
}
"suggestedUserMessage": "In order to perform this request you’ll need to add a Third-Party Virtual key to your vehicle. Please open this link on the phone with your Tesla App to complete this step."
}
```
### Suggested resolution
For Tesla Owners:
* The vehicle owner needs to add Smartcar’s Third-Party Virtual Key. As of late 2023, Tesla is starting to require virtual keys for Third-Party applications to perform commands.
* In order to issue commands, please prompt the vehicle owner to add Smartcar’s Third-Party virtual key: [https://www.tesla.com/\_ak/smartcar.com](https://www.tesla.com/_ak/smartcar.com)
### Troubleshooting steps
* Please direct the vehicle owner to add Smartcar’s [Third-Party Virtual Key (tesla.com)](https://www.tesla.com/_ak/smartcar.com) in order to allow your application to issue commands to the vehicle.
### Suggested user message
> In order to perform this request you’ll need to add a Third-Party Virtual key to your vehicle. Please open [this](https://www.tesla.com/_ak/smartcar.com) link on the phone with your Tesla App to complete this step.
# Permission Errors
Source: https://smartcar.com/docs/errors/api-errors/permission-errors
Thrown when Smartcar does not support a make or feature for a vehicle.
# `NULL`
Your application has insufficient permissions to access the requested resource. Please prompt the user to re-authenticate using Smartcar Connect.
If you receive this error while testing with Vehicle Simulator, it is likely because the simulated vehicle has not yet been connected to your application, or your application doesn't have access to the requested permission.
Visit the Simulator documentation to learn how to connect the vehicle to your application.
```json
{
"type": "PERMISSION",
"code": null,
"description": "Your application has insufficient permissions to access the requested resource. Please prompt the user to re-authenticate using Smartcar Connect.",
"docURL": "https://smartcar.com/docs/errors/api-errors/permission-errors#null",
"statusCode": 403,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": "REAUTHENTICATE" }
}
```
### Suggested Resolution
You can resolve this error by ensuring that the scope parameter contains all the permissions that your application requires and prompting the user to re-authenticate using Smartcar Connect.
### Troubleshooting Steps
* Ensure that the scope parameter contains all the permissions that your application requires.
* Ensure that you spell all permission names in the scope parameter correctly.
* Prompt the user to re-authenticate using Smartcar Connect.
# Rate Limit Errors
Source: https://smartcar.com/docs/errors/api-errors/rate-limit-errors
Thrown when there is an issue with the frequency of your requests.
# `SMARTCAR_API`
Your application has exceeded its rate limit. Please retry your request in a few minutes.
```json
{
"type": "RATE_LIMIT",
"code": "SMARTCAR_API",
"description": "Your application has exceeded its rate limit. Please retry your request in a few minutes.",
"docURL": "https://smartcar.com/docs/errors/api-errors/rate-limit-errors#smartcar-api",
"statusCode": 429,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": "RETRY_LATER" },
"suggestedUserMessage": "Your vehicle is temporarily unable to connect to . Please be patient while we’re working to resolve this issue."
}
```
### Suggested resolution
You can resolve this error by refraining from making API requests for a certain period of time.
If your application automatically retries requests for certain errors, please disable automatic retries or implement a backoff period to retry certain requests less frequently.
### Troubleshooting steps
If you believe that you received this error by mistake and your application didn’t actually exceed its rate limit, please contact us and we’ll be happy to assist you.
### Suggested user message
> Your car is temporarily unable to connect to \. Please be patient while we’re working to resolve this issue.
***
# `VEHICLE`
You have reached the throttling rate limit for this vehicle. Please see the `retry-after` header (seconds) for when to retry the request.
This limit is in place to prevent excessive vehicle requests that could:
* Lead to 12v battery drain.
* Trigger UPSTREAM:RATE\_LIMIT errors that prevent you from making requests for a longer period of time.
```json
{
"type": "RATE_LIMIT",
"code": "VEHICLE",
"description": "You have reached the throttling rate limit for this vehicle. Please see the retry-after header for when to retry the request.",
"docURL": "https://smartcar.com/docs/errors/api-errors/rate-limit-errors#vehicle",
"statusCode": 429,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": "RETRY_LATER" },
"suggestedUserMessage": "Your vehicle is temporarily unable to connect to . Please be patient while we’re working to resolve this issue."
}
```
### Suggested resolution
You can resolve this error by refraining from making API requests. Please see the retry-after header for when to try again. If your application automatically retries requests for certain errors, please disable them for this one.
### Suggested user message
> Your car is temporarily unable to connect to \. Please be patient while we’re working to resolve this issue.
***
# Resource Not Found
Source: https://smartcar.com/docs/errors/api-errors/resource-not-found-errors
Thrown when the incorrect API version is used or when the endpoint URL is incorrect.
# `PATH`
The requested resource does not exist. Please check the URL and try again.
```json
{
"type": "RESOURCE_NOT_FOUND",
"code": "PATH",
"description": "The requested resource does not exist. Please check the URL and try again.",
"docURL": "https://smartcar.com/docs/errors/api-errors/resource-errors#pathh",
"statusCode": 404,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": null },
"suggestedUserMessage": "Your vehicle is temporarily unable to connect to . Please be patient while we’re working to resolve this issue."
}
```
### Suggested Resolution
You can resolve this error by referring to our API reference and ensuring that you use the correct URL for your request.
### Troubleshooting Steps
* Ensure that you spell all static parts of the URL correctly.
* Ensure that you use the correct URL path parameters (e.g. vehicle ID).
* Ensure that you use the correct HTTP method.
***
# `VERSION`
The requested resource does not exist. Your request either does not specify a version number or it specifies a version number that is not supported by this resource.
```json
{
"type": "RESOURCE_NOT_FOUND",
"code": "VERSION",
"description": "The requested resource does not exist. Please check your specified version number and try again.",
"docURL": "https://smartcar.com/docs/errors/api-errors/resource-errors#version",
"statusCode": 404,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": null },
"suggestedUserMessage": "Your vehicle is temporarily unable to connect to . Please be patient while we’re working to resolve this issue."
}
```
### Suggested Resolution
You can resolve this error by referring to our API reference and ensuring that you specify v2.0 in the URL path (e.g. [https://api.smartcar.com/v2.0/vehicles](https://api.smartcar.com/v2.0/vehicles)). Version 1 has been sunset and is no longer supported.
# Server Errors
Source: https://smartcar.com/docs/errors/api-errors/server-errors
Thrown when Smartcar runs into an unexpected issue and was unable to process the request.
# `INTERNAL`
An internal Smartcar error has occurred. Our team has been notified and is working to resolve this issue.
```json
{
"type": "SERVER",
"code": "INTERNAL",
"description": "An internal Smartcar error has occurred. Our team has been notified and is working to resolve this issue.",
"docURL": "https://smartcar.com/docs/errors/api-errors/server-errors#internal",
"statusCode": 500,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": "RETRY_LATER" },
"suggestedUserMessage": "Your car is temporarily unable to connect to . Please be patient while we’re working to resolve this issue."
}
```
### Suggested Resolution
Please contact us to learn more about the error and our steps to resolve it.
### Suggested User Message
> Your car is temporarily unable to connect to \. Please be patient while we’re working to resolve this issue.
***
# `RECORD_NOT_FOUND`
Smartcar is unable to locate a battery capacity record for this vehicle. Depending on the make of the vehicle, battery capacity may depend on upstream sources to fulfil a request if it is not directly provided by the OEM.
If you’re seeing this error then the OEM doesn’t provide all the data we aim to provide in our response and we were unable to locate this information from other sources.
```json
{
"type": "SERVER",
"code": "RECORD_NOT_FOUND",
"description": "Smartcar was unable to locate a battery capacity record for this vehicle.",
"docURL": "https://smartcar.com/docs/errors/api-errors/server-errors#record-not-found",
"statusCode": 500,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": "CONTACT_SUPPORT" },
"suggestedUserMessage": "Your car is temporarily unable to connect to . Please be patient while we’re working to resolve this issue."
}
```
### Suggested Resolution
Please reach out to support to see if we are able to update capacity data for the vehicle.
### Suggested User Message
> Your car is temporarily unable to connect to \. Please be patient while we’re working to resolve this issue.
***
# `MULTIPLE_RECORDS_FOUND`
Smartcar is unable to locate a battery capacity record for this vehicle. Depending on the make of the vehicle, battery capacity may depend on upstream sources to fulfil a request if it is not directly provided by the OEM.
If you’re seeing this error then the OEM doesn’t provide all the data we aim to provide in our response and we were unable to find a specific match. Please see the error response `detail` field for possible matches.
```json
{
"statusCode": 500,
"type": "SERVER",
"code": "MULTIPLE_RECORDS_FOUND",
"description": "Smartcar found multiple battery capacity records for this vehicle.",
"docURL": "https://smartcar.com/docs/errors/api-errors/server-errors#multiple-records-found",
"resolution": {
"type": "CONTACT_SUPPORT"
},
"suggestedUserMessage": "Your car is temporarily unable to connect to . Please be patient while we’re working to resolve this issue.",
"detail": [
{
"capacities": [83.9, 83.9, 70.2]
}
],
"requestId": "9c8dd6fa-f9d7-4d18-9fdd-2255f6cd8613"
}
```
### Suggested Resolution
For battery capacity errors please check the detail field of the error response for a list of possible options and reach out support if you need us to update the capacity value for a specific vehicle.
### Suggested User Message
> Your car is temporarily unable to connect to \. Please be patient while we’re working to resolve this issue.
# Upstream Errors
Source: https://smartcar.com/docs/errors/api-errors/upstream-errors
Thrown when the OEM servers or vehicle failed to process the request.
# `INVALID_DATA`
One of Smartcar’s upstream sources provided data that is malformed, invalid, or logically invalid (e.g. 65535 psi for tire pressure).
```json
{
"type": "UPSTREAM",
"code": "INVALID_DATA",
"description": "Smartcar received invalid data from an upstream source. Please retry your request at a later time.",
"docURL": "https://smartcar.com/docs/errors/api-errors/upstream-errors#invalid-data",
"statusCode": 502,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": "RETRY_LATER" },
"suggestedUserMessage": "Your car is temporarily unable to connect to . Please be patient while we’re working to resolve this issue."
}
```
### Suggested resolution
You can resolve this error by retrying your request at a later time.
### Suggested user message
> Your car is temporarily unable to connect to \. Please be patient while we’re working to resolve this issue.
***
# `KNOWN_ISSUE`
Smartcar received an error from an upstream source. This error usually occurs when an upstream source experiences an error that we have previously investigated and categorized as a known issue.
```json
{
"type": "UPSTREAM",
"code": "KNOWN_ISSUE",
"description": "Smartcar received an error from an upstream source. Please retry your request at a later time.",
"docURL": "https://smartcar.com/docs/errors/api-errors/upstream-errors#known-issue",
"statusCode": 502,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": "RETRY_LATER" },
"suggestedUserMessage": "Your car is temporarily unable to connect to . Please be patient while we’re working to resolve this issue."
}
```
### Suggested resolution
You can resolve this error by retrying your request at a later time.
### Suggested user message
> Your car is temporarily unable to connect to \. Please be patient while we’re working to resolve this issue.
***
# `NO_RESPONSE`
One of Smartcar’s upstream sources failed to respond. This error usually occurs when one of Smartcar’s upstream sources is experiencing issues and is currently unavailable.
This error can also occur when a specific vehicle in located in an area with weak cellular reception and fails to respond to your request in a timely manner.
```json
{
"type": "UPSTREAM",
"code": "NO_RESPONSE",
"description": "One of Smartcar’s upstream sources failed to respond. Please retry your request at a later time.",
"docURL": "https://smartcar.com/docs/errors/api-errors/upstream-errors#no-response",
"statusCode": 502,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": "RETRY_LATER" },
"suggestedUserMessage": "Your car is temporarily unable to connect to . Please be patient while we’re working to resolve this issue."
}
```
### Suggested resolution
You can resolve this error by retrying your request at a later time.
### Suggested user message
> Your car is temporarily unable to connect to \. Please be patient while we’re working to resolve this issue.
***
# `RATE_LIMIT`
Your application’s requests have exceeded this vehicle’s rate limit.
```json
{
"type": "UPSTREAM",
"code": "NO_RESPONSE",
"description": "One of Smartcar’s upstream sources failed to respond. Please retry your request at a later time.",
"docURL": "https://smartcar.com/docs/errors/api-errors/upstream-errors#rate-limit",
"statusCode": 502,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": "RETRY_LATER" },
"suggestedUserMessage": "Your car is temporarily unable to connect to . Please be patient while we’re working to resolve this issue."
}
```
### Suggested resolution
You can resolve this error by refraining from making API requests to this specific vehicle for a period of time.
If your application automatically retries requests, please disable automatic retries or implement a backoff period to retry less frequently.
### Suggested user message
> Your car is temporarily unable to connect to \. Please be patient while we’re working to resolve this issue.
***
# `UNKNOWN_ISSUE`
Smartcar received an unknown error from an upstream source. This error usually occurs when one of Smartcar’s upstream sources experiences an error that we have not yet investigated or mitigated.
There are several reasons why we might not have investigated this error yet:
* The feature or vehicle make is still in beta.
* This error does not occur very frequently.
* This is the first time we have received this error.
```json
{
"type": "UPSTREAM",
"code": "UNKNOWN_ISSUE",
"description": "Smartcar received an unknown error from an upstream source. Please retry your request at a later time and contact us if the issue persists.",
"docURL": "https://smartcar.com/docs/errors/api-errors/upstream-errors#unknown-issue",
"statusCode": 502,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": "RETRY_LATER" },
"suggestedUserMessage": "Your car is temporarily unable to connect to . Please be patient while we’re working to resolve this issue."
}
```
### Suggested resolution
Given the broad range of situations that can cause this error, you might or might not be able to resolve this error by retrying your request at a later time.
If you retry your request and the issue persists, please contact us to help you mitigate this error.
### Suggested user message
> Your car is temporarily unable to connect to \. Please be patient while we’re working to resolve this issue.
***
# Validation Errors
Source: https://smartcar.com/docs/errors/api-errors/validation-errors
Thrown if there is an issue with the format of the request or body.
# `NULL`
Request invalid or malformed. Please check for missing parameters, spelling and casing mistakes, and other syntax issues.
```json
{
"type": "VALIDATION",
"code": null,
"description": "Request invalid or malformed. Please check for missing parameters, spelling and casing mistakes, and other syntax issues.",
"docURL": "https://smartcar.com/docs/errors/api-errors/validation-errors#null",
"statusCode": 400,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": null },
"suggestedUserMessage": "Your car is temporarily unable to connect to . Please be patient while we’re working to resolve this issue."
}
```
### Suggested resolution
You can resolve this error by referring to our API reference and ensuring that you pass all the parameters as specified.
### Troubleshooting Steps
* Ensure that you spell and case all parameters correctly.
* Ensure that your request has the correct content-type (i.e. application/json or application/x-www-form-urlencoded).
* Ensure that your request has the correct URL and HTTP method.
### Suggested user message
> Your car is temporarily unable to connect to \. Please be patient while we’re working to resolve this issue.
***
# `PARAMETER`
Your request is formatted correctly, but one or more of the provided parameters is incorrect.
```json
{
"type": "VALIDATION",
"code": null,
"description": "Your request is formatted correctly, but one or more of the provided parameters is incorrect.",
"docURL": "https://smartcar.com/docs/errors/api-errors/validation-errors#parameter",
"statusCode": 400,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": null },
"suggestedUserMessage": "Your car is temporarily unable to connect to . Please be patient while we’re working to resolve this issue."
}
```
### Suggested resolution
You can resolve this error by referring to our API reference and ensuring that you pass all the parameters as specified.
For Ford or Lincoln vehicles:
* Please check the coordinates provided for setting a charge schedule are associated with a saved charging location for this vehicle.
### Suggested user message
> Your car is temporarily unable to connect to \. Please be patient while we’re working to resolve this issue.
# Vehicle State Errors
Source: https://smartcar.com/docs/errors/api-errors/vehicle-state-errors
Thrown when a request fails due to the state of a vehicle or logically cannot be completed e.g. you can't start a vehicle charging if it's not plugged in.
# `ASLEEP`
The vehicle is in a sleep state and temporarily unable to perform your request. Either the vehicle has been inactive for a certain period of time (time periods vary between makes and models) or the user has manually triggered the vehicle’s sleep state.
```json
{
"type": "VEHICLE_STATE",
"code": "ASLEEP",
"description": "The vehicle is in a sleep state and temporarily unable to perform your request.",
"docURL": "https://smartcar.com/docs/errors/api-errors/vehicle-state-errors#asleep",
"statusCode": 409,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": null },
"suggestedUserMessage": "Your vehicle is in a sleep state. This request was not sent to preserve battery life. "
}
```
### Suggested resolution
The vehicle is in a sleep state and temporarily unable to perform your request.
### Troubleshooting Steps
* Prompt the user to start the vehicle’s engine (required) and to drive the vehicle for a short distance (optional).
* Wait for a few minutes and retry your request.
* Ensure that the vehicle’s 12-volt battery is sufficiently charged.
### Suggested user message
> Your car is temporarily unable to connect to \. To re-establish the connection, please start your car and take it for a short drive.
***
# `CHARGING_IN_PROGRESS`
The vehicle is currently charging and unable to perform your request. This error usually occurs when you make a request to the start charge endpoint while the vehicle is already charging.
```json
{
"type": "VEHICLE_STATE",
"code": "CHARGING_IN_PROGRESS",
"description": "The vehicle is currently charging and unable to perform your request.",
"docURL": "https://smartcar.com/docs/errors/api-errors/vehicle-state-errors#charging-in-progress",
"statusCode": 409,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": null },
"suggestedUserMessage": "Your vehicle is already charging."
}
```
### Suggested resolution
You usually don’t need to resolve this error. If you believe that this error should not have occurred, please follow the troubleshooting steps below.
### Troubleshooting Steps
* Prompt the user to stop charging their vehicle and/or unplug the charger.
* Wait for a few minutes and retry your request.
### Suggested user message
> Your car is already charging.
***
# `CHARGING_PLUG_CONNECTED`
The vehicle is connected to an EV charger and unable to perform your request.
```json
{
"type": "VEHICLE_STATE",
"code": "CHARGING_PLUG_CONNECTED",
"description": "The vehicle is connected to an EV charger and unable to perform your request.",
"docURL": "https://smartcar.com/docs/errors/api-errors/vehicle-state-errors#charging-plug-connected",
"statusCode": 409,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": null },
"suggestedUserMessage": "We’re unable to issue the command because the vehicle is currently plugged in."
}
```
### Suggested resolution
The user can resolve this error by unplugging the vehicle.
### Suggested user message
> We're unable to issue the command because the vehicle is currently plugged in. Please ensure that you have unplugged the vehicle from an EV charger.
***
# `CHARGING_PLUG_NOT_CONNECTED`
The vehicle is not connected to an EV charger and unable to perform your request. This error usually occurs when you make a request to the start charge endpoint
but the vehicle’s charge port does not have an EV charger plugged into it.
If testing an electric vehicle with Vehicle Simulator, you will receive this error when attempting a start or stop charge request while the vehicle is in a parked
or driving state (not charging).
```json
{
"type": "VEHICLE_STATE",
"code": "CHARGING_PLUG_NOT_CONNECTED",
"description": "The vehicle is not connected to an EV charger and unable to perform your request.",
"docURL": "https://smartcar.com/docs/errors/api-errors/vehicle-state-errors#charging-plug-not-connected",
"statusCode": 409,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": null },
"suggestedUserMessage": "Your vehicle is not plugged in."
}
```
### Suggested resolution
The user can resolve this error by plugging an EV charger into the vehicle’s charge port.
### Troubleshooting Steps
* Prompt the user to plug an EV charger into the vehicle’s charge port.
* If this doesn’t resolve the issue, prompt the user to ensure that the EV charger is connected to a working power source, that it isn’t faulty, and that it is compatible with the user’s vehicle. For example, prompt the user to try out other EV chargers and to check the vehicle’s owner manual for a list of compatible chargers.
### Suggested user message
> Your car isn’t able to start charging. Please ensure that you have plugged an EV charger into your car’s charge port.
***
# `DOOR_OPEN`
The vehicle could not perform your request because one or more of its doors are open. This error usually occurs when you make a request to the lock endpoint while one or more of the vehicle’s doors stand open.
```json
{
"type": "VEHICLE_STATE",
"code": "DOOR_OPEN",
"description": "The vehicle could not perform your request because one or more of its doors are open.",
"docURL": "https://smartcar.com/docs/errors/api-errors/vehicle-state-errors#door-open",
"statusCode": 409,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": null },
"suggestedUserMessage": "Your vehicle was unable to lock. Please ensure that all doors and the trunk are fully closed."
}
```
### Suggested resolution
The user can resolve this error by fully closing all of the vehicle’s doors and the trunk.
### Troubleshooting Steps
* Prompt the user to ensure that there is nothing preventing the doors from fully closing and/or locking properly.
* Prompt the user to ensure that the vehicle’s trunk is fully closed.
### Suggested user message
> Your car was unable to lock. Please ensure that all doors and the trunk are fully closed.
***
# `FULLY_CHARGED`
The vehicle is unable to perform your request because its EV battery is fully charged. This error usually occurs when you make a request to the start charge endpoint while the vehicle is already fully charged.
```json
{
"type": "VEHICLE_STATE",
"code": "FULLY_CHARGED",
"description": "The vehicle is unable to perform your request because its EV battery is fully charged.",
"docURL": "https://smartcar.com/docs/errors/api-errors/vehicle-state-errors#fully-charged",
"statusCode": 409,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": null },
"suggestedUserMessage": "Your vehicle is already fully charged."
}
```
### Suggested user message
> Your car is already fully charged.
***
# `NOT_CHARGING`
The vehicle is unable to perform your request because it is not currently charging. This error usually occurs when you request information about a vehicle’s charge session while the battery is not charging.
```json
{
"type": "VEHICLE_STATE",
"code": "NOT_CHARGING",
"description": "The vehicle is unable to perform your request because it is not currently charging.",
"docURL": "https://smartcar.com/docs/errors/api-errors/vehicle-state-errors#not-charging",
"statusCode": 409,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": null },
"suggestedUserMessage": "Please ensure that you have plugged an EV charger into your car’s charge port and the vehicle is charging."
}
```
### Suggested resolution
You can resolve this error by having the user start a charge session or by sending a start charge request if the vehicle supports that capability.
### Troubleshooting Steps
* Prompt the user to start charging their vehicle.
* Wait for a few minutes and retry your request.
### Suggested user message
> Please ensure that you have plugged an EV charger into your car’s charge port and the vehicle is charging.
***
# `CHARGE_FAULT`
The vehicle is connected to an EV charger but cannot perform the request because:
* the charger is not providing any current
* there is a conflict with another charging schedule
```json
{
"type": "VEHICLE_STATE",
"code": "CHARGE_FAULT",
"description": "The vehicle is connected to an EV charger but cannot perform the request because 1) the charger is not providing any current, or 2) there is a conflict with another charging schedule.",
"docURL": "https://smartcar.com/docs/errors/api-errors/vehicle-state-errors#charge-fault",
"statusCode": 409,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": null },
"suggestedUserMessage": "Your vehicle is unable to start or stop charging because of a conflict with the vehicle's charging schedule."
}
```
### Suggested resolution
The user can resolve this error by:
* Disabling any schedules that control when the charger can supply current and charge the vehicle.
* Ensuring that the charging cable is securely connected to the vehicle.
* Disabling any charge schedules on the vehicle’s infotainment system or connected service application.
* Disabling any departure timers on the vehicle’s infotainment system or connected service application.
* Removing the vehicle from other charge scheduling services.
### Troubleshooting Steps
* Prompt the user to start charging their vehicle.
* Wait for a few minutes and retry your request.
### Suggested user message
> Your car is unable to start or stop charging because of a conflict with your vehicle’s charging schedule. Please check that:
>
> * You have disabled any schedules that control when your charger is able to supply current to and charge the vehicle.
> * The charging cable is securely connected to your vehicle.
> * You have disabled any charge schedules on your vehicle’s infotainment system or connected service application.
> * You have disabled any departure timers on your vehicle’s infotainment system or connected service application.
> * Your vehicle is not connected to any other charge scheduling services.
***
# `HOOD_OPEN`
The vehicle is unable to perform your request because its hood is open. This error usually occurs when you make a request to the lock endpoint while the vehicle’s hood stands open.
```json
{
"type": "VEHICLE_STATE",
"code": "HOOD_OPEN",
"description": "The vehicle is unable to perform your request because its hood is open.",
"docURL": "https://smartcar.com/docs/errors/api-errors/vehicle-state-errors#hood-open",
"statusCode": 409,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": null },
"suggestedUserMessage": "Your vehicle was unable to lock. Please ensure that the vehicle's hood is fully closed."
}
```
### Suggested resolution
The user can resolve this error by ensuring that the vehicle’s hood is fully closed.
### Suggested user message
> Your car was unable to lock. Please ensure that your car’s hood is fully closed.
***
# `IGNITION_ON`
The vehicle was unable to perform your request because its engine is running. Depending on the vehicle’s make and model, this error can occur for a variety of API endpoints.
```json
{
"type": "VEHICLE_STATE",
"code": "IGNITION_ON",
"description": "The vehicle was unable to perform your request because its engine is running.",
"docURL": "https://smartcar.com/docs/errors/api-errors/vehicle-state-errors#ignition-on",
"statusCode": 409,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": null },
"suggestedUserMessage": "Your vehicle is currently running."
}
```
### Suggested resolution
The user can resolve this error by turning off their vehicle. You can also resolve this error by retrying your request at a later time.
### Suggested user message
> Your car is temporarily unable to connect to \ because its engine is running. To re-establish the connection, please turn off your car’s engine.
***
# `IN_MOTION`
The vehicle is currently in motion and unable to perform your request. Depending on the vehicle’s make and model, this error can occur for a variety of API endpoints. The lock/unlock endpoint is a common example.
If testing with Vehicle Simulator, you will receive this error when attempting a lock/unlock request while the vehicle is in a driving state along the simulation.
```json
{
"type": "VEHICLE_STATE",
"code": "IN_MOTION",
"description": "The vehicle is currently in motion and unable to perform your request.",
"docURL": "https://smartcar.com/docs/errors/api-errors/vehicle-state-errors#in-motion",
"statusCode": 409,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": null },
"suggestedUserMessage": "Your vehicle is unable to perform the request while in motion."
}
```
### Suggested resolution
The user can resolve this error by parking their car and turning off the engine. You can also resolve this error by retrying your request at a later time.
### Suggested user message
> Your car is in motion and temporarily unable to connect to \. To re-establish the connection, please park your car and turn off its engine.
***
# `REMOTE_ACCESS_DISABLED`
The vehicle is unable to perform your request because the user has disabled remote access for connected services.
```json
{
"type": "VEHICLE_STATE",
"code": "REMOTE_ACCESS_DISABLED",
"description": "The vehicle is unable to perform your request because the user has disabled remote access for connected services.",
"docURL": "https://smartcar.com/docs/errors/api-errors/vehicle-state-errors#remote-access-disabled",
"statusCode": 409,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": null },
"suggestedUserMessage": "Your vehicle is unable to perform the request as remote access is disabled or unavailable."
}
```
### Suggested resolution
The user can resolve this error by re-enabling remote access inside their vehicle. If the user is unsure how to enable remote access for connected services, prompt them to refer to their vehicle’s owner manual for more details.
### Suggested user message
> Your car is temporarily unable to connect to \. To re-establish the connection, please make sure that remote access for connected services is enabled inside your car. Please refer to your car’s owner manual for more details.
***
# `TRUNK_OPEN`
The vehicle is unable to perform your request because its trunk is open. This error usually occurs when you make a request to the lock endpoint while the vehicle’s trunk and/or front trunk are open.
```json
{
"type": "VEHICLE_STATE",
"code": "TRUNK_OPEN",
"description": "The vehicle is unable to perform your request because its trunk is open.",
"docURL": "https://smartcar.com/docs/errors/api-errors/vehicle-state-errors#trunk-open",
"statusCode": 409,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": null },
"suggestedUserMessage": "Your vehicle was unable to open the trunk because it is already open."
}
```
### Suggested resolution
The user can resolve this error by closing the vehicle’s trunk and/or front trunk.
### Suggested user message
> Your car was unable to lock. Please ensure that your car’s trunk (and front trunk) is closed.
***
# `UNKNOWN`
The vehicle was unable to perform your request due to an unknown issue. This error occurs when the vehicle is in a state that makes it unable to perform your request, and we are unable to determine which state the vehicle is in.
If testing an electric vehicle with Vehicle Simulator, you will receive this error when attempting a stop charge request while the vehicle is in a charging state.
```json
{
"type": "VEHICLE_STATE",
"code": "UNKNOWN",
"description": "The vehicle was unable to perform your request due to an unknown issue.",
"docURL": "https://smartcar.com/docs/errors/api-errors/vehicle-state-errors#unknown",
"statusCode": 409,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": "RETRY_LATER" },
"suggestedUserMessage": "Your vehicle is temporarily unable to connect due to an unknown issue. Please be patient while we’re working to resolve this issue."
}
```
### Suggested resolution
Please retry your request at a later time.
### Suggested user message
> Your car is temporarily unable to connect to \. Please be patient while we’re working to resolve this issue.
***
# `UNREACHABLE`
The vehicle was unable to perform your request because it is currently unreachable. This error usually occurs when the vehicle is in a location with poor cellular reception (e.g. a parking garage or underground parking).
If testing with Vehicle Simulator, you will receive this error when attempting a lock/unlock or start/stop charge request while the simulation is paused or completed.
```json
{
"type": "VEHICLE_STATE",
"code": "UNREACHABLE",
"description": "The vehicle was unable to perform your request because it is currently unreachable.",
"docURL": "https://smartcar.com/docs/errors/api-errors/vehicle-state-errors#unreachable",
"statusCode": 409,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": null },
"suggestedUserMessage": "Your vehicle is currently unreachable. Ensure the vehicle has adequate mobile coverage."
}
```
### Suggested resolution
The user can resolve this error by moving the vehicle to a location with better cellular reception. You can also resolve this error by retrying your request at a later time.
### Suggested user message
> Your car is temporarily unable to connect to \. To re-establish the connection, please move your car to a location with a better cell phone signal.
***
# `VEHICLE_OFFLINE_FOR_SERVICE`
The vehicle was unable to perform your request because it is currently in service mode. Service mode temporarily limits or disables the vehicle’s remote capabilities.
```json
{
"type": "VEHICLE_STATE",
"code": "VEHICLE_OFFLINE_FOR_SERVICE",
"description": "The vehicle was unable to perform your request because it is currently in service mode.",
"docURL": "https://smartcar.com/docs/errors/api-errors/vehicle-state-errors#vehicle-offline-for-service",
"statusCode": 409,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": null },
"suggestedUserMessage": "Your vehicle is in service mode and temporarily unable to perform this task."
}
```
### Suggested resolution
Please retry your request at a later time.
### Suggested user message
> Your car is in service mode and temporarily unable to connect to \. Please try again later.
***
# `LOW_BATTERY`
The vehicle was unable to perform your request because the 12v or high voltage battery is too low.
```json
{
"type": "VEHICLE_STATE",
"code": "LOW_BATTERY",
"description": "The vehicle was unable to perform your request because the 12v or high voltage battery is too low.",
"docURL": "https://smartcar.com/docs/errors/api-errors/vehicle-state-errors#low-battery",
"statusCode": 409,
"requestId": "5dea93a1-3f79-4246-90c5-89610a20471b",
"resolution": { "type": "RETRY_LATER" },
"suggestedUserMessage": "Your vehicle battery is too low to perform this task."
}
```
### Suggested resolution
Please retry your request at a later time.
* For EVs ensure that the battery is topped up to at least 60% SoC.
* Ensure that the vehicle’s 12-volt battery is sufficiently charged. Taking the vehicle on a short drive can help.
### Suggested user message
> \ was unable to connect to your car because its battery is too low. Please ensure the battery is charged and try again later.
***
# Access Denied
Source: https://smartcar.com/docs/errors/connect-errors/access-denied
This error occurs when a user denies your application access to the requested scope of permissions.
| Parameter | Required | Description |
| ------------------- | -------- | ---------------------------------- |
| `error` | `true` | `access_denied` |
| `error_description` | `true` | User denied access to application. |
```http Example redirect uri
HTTP/1.1 302 Found
Location: https://example.com/home
?error=access_denied
&error_description=User+denied+access+to+application
```
## Testing
To test this error, launch Smartcar Connect in test mode and select “Deny access” on the permissions screen.
We recommend handling this error by re-prompting the user to authorize their vehicle and adding a message like in the example below.
# Configuration
Source: https://smartcar.com/docs/errors/connect-errors/configuration-error
This error occurs when the user has encountered an Error page in Connect and has chosen to return to your application.
| Parameter | Required | Description |
| ------------------- | -------- | ----------------------------------------------------------------- |
| `error` | `true` | `configuration_error` |
| `error_description` | `true` | There has been an error in the configuration of your application. |
| `status_code` | `true` | The status code of the error encountered in Connect |
| `error_message` | `true` | The error message seen by the user |
```http Example redirect uri
HTTP/1.1 302 Found
Location: https://example.com/callback
?error=configuration_error
&error_description=There%20has%20been%20an%20error%20in%20the%20configuration%20of%20your%20application.&status_code=400
&error_message=You%20have%20entered%20a%20test%20mode%20VIN.%20Please%20enter%20a%20VIN%20that%20belongs%20to%20a%20real%20vehicle.
```
On redirect, Connect will return the status code and message of the error that they encountered. This will be triggered if:
* A user tried to directly navigate to a page that is past their current step in Connect (ie. going directly from the Preamble screen to the Permission Grants screen by directly going to connect.smartcar.com/grant).
* A user is trying to use Single Select in live mode with a test mode VIN or with a simulated VIN in a non-simulated mode.
* A user is trying to use Single Select with an invalid mock VIN.
* A validation error occurs when trying to check compatibility by VIN.
These cases will trigger the following error and give the user the ability to “Exit” Connect.
# Invalid Subscription
Source: https://smartcar.com/docs/errors/connect-errors/invalid-subscription
This error occurs when a user’s vehicle is compatible but their connected services subscription is inactive because either it has expired or it has never been activated.
| Parameter | Required | Description | |
| ------------------ | -------- | ------------------------------------------------------------ | - |
| error | true | invalid\_subscription | |
| error\_description | true | User does not have an active connected vehicle subscription. | |
```http Example redirect uri
HTTP/1.1 302 Found
Location: https://example.com/callback
?error=invalid_subscription
&error_description=User%20does%20not%20have%20an%20active%20connected%20vehicle%20subscription
```
Smartcar will direct the user to the connected services website to (re-)activate their subscription. However, a user may choose to return back to your application instead, like in the example below.
## Testing
To test this error, launch Smartcar Connect in test mode and log in with the email [smartcar@invalid-subscription.com](mailto:smartcar@invalid-subscription.com) and any password. If you use Single Select, please see the table below for a simulated VIN.
| Email | VIN |
| ----------------------------------------------------------------------------- | ----------------- |
| [smartcar@invalid-subscription.com](mailto:smartcar@invalid-subscription.com) | 0SCAUDI0155C49A95 |
In the event of an `AUTHENTICATION_FAILED`, the user will need to be prompted to re-connect their vehicle. Smartcar Connect Re-authentication makes the process of re-authenticating a user much more seamless. In addition, the `AUTHENTICATION_FAILED` error provides a partially constructed URL for the re-authentication flow inside the resolution object.
# No Vehicles
Source: https://smartcar.com/docs/errors/connect-errors/no-vehicles
This error occurs when a vehicle owner has a connected services account, but there are no vehicles associated with the account.
| Parameter | Required | Description | |
| ------------------ | -------- | ------------------------------------------------------------ | - |
| error | true | no\_vehicles | |
| error\_description | true | User does not have an active connected vehicle subscription. | |
```http Example redirect uri
HTTP/1.1 302 Found
Location: https://example.com/callback
?error=no_vehicles&error_description=No%20vehicles%20found.%20Please%20add%20vehicles%20to%20your%20account%20and%20try%20again.
```
The error is triggered when a user logs into their connected services account and is shown to have no vehicles listed like in the example below for BMW.
The user has the option to add vehicles or return to your application.
# OEM Login Cancelled
Source: https://smartcar.com/docs/errors/connect-errors/oem-login-cancelled
This error occurs when a user went to authorize directly with the OEM but exited the flow for some reason.
| Parameter | Required | Description |
| ------------------- | -------- | ---------------------------------------------------- |
| `error` | `true` | `oem_cancelled_login` |
| `error_description` | `true` | The user did not complete authorization with the OEM |
```http Example redirect uri
HTTP/1.1 302 Found
Location: https://example.com/callback
?error=oem_cancelled_login
&the%20user%20did%20not%20complete%20authorization%20with%20the%20OEM
```
# User returned to application
Source: https://smartcar.com/docs/errors/connect-errors/returned-to-application
This error occurs when a user leaves the Connect flow after hitting **Back to application** before granting your application access to their vehicle.
| Parameter | Required | Description |
| ------------------- | -------- | --------------------------------------------------------------- |
| `error` | `true` | `user_manually_returned_to_application` |
| `error_description` | `true` | The user exited Connect before granting your application access |
There are cases where a user may wish to exit Connect before granting your application access to their vehicle. In these cases, if the user hits **Back to application** instead of closing out the window, Smartcar will return this error to your callback URI. Below are places in the Connect flow users can return to your application.
# Server
Source: https://smartcar.com/docs/errors/connect-errors/server-error
If there is a server error, the user will return to your application.
| Parameter | Required | Description |
| ------------------ | -------- | ------------------------------------------ |
| error | true | server\_error |
| error\_description | true | Unexpected server error. Please try again. |
```http Example redirect uri
HTTP/1.1 302 Found
Location: https://example.com/callback
?error=configuration_error
&error_description=There%20has%20been%20an%20error%20in%20the%20configuration%20of%20your%20application.&status_code=400
&error_message=You%20have%20entered%20a%20test%20mode%20VIN.%20Please%20enter%20a%20VIN%20that%20belongs%20to%20a%20real%20vehicle.
```
We only show the “Exit” button if a redirect URI is available. If there is a failure before the validation of the redirect URI, we are unable to include the “Exit” button on the Error page. In those cases, we would not be able to report the `status_code` and `error_message` back to the developer, but they will still be available on screen in the Error page.
# Vehicle Incompatible
Source: https://smartcar.com/docs/errors/connect-errors/vehicle-incompatible
This error occurs when a user tries to authorize an incompatible vehicle in Smartcar Connect.
| Parameter | Required | Description |
| ------------------- | -------- | ------------------------------------- |
| `error` | `true` | `vehicle_incompatible` |
| `error_description` | `true` | The user’s vehicle is not compatible. |
| `make` | `false` | The manufacturer of the vehicle. |
| `vin` | `false` | The VIN of the vehicle. |
In order to be compatible, a vehicle must:
* Have the hardware required for internet connectivity
* Belong to the makes and models Smartcar is compatible with
* Be capable of the required permissions that your application is requesting access to
* If the user’s vehicle is incompatible, Smartcar will let the user know and offer them to share their vehicle’s VIN, make, model, and year with your application.
This error is triggered when a user selects an unspported make, or selects "I don't see my brand..." and then hits "Back to application"
We recommend that your application provides a flow for incompatible vehicles like in the example below.
Note: This error will never occur if your application uses the Compatibility API. The Compatibility API verifies the compatibility of a vehicle before the user enters Smartcar Connect.
To test this error, launch Smartcar Connect in test mode and log in with the email [smartcar@vehicle-incompatible.com](mailto:smartcar@vehicle-incompatible.com) and any password.
If you use Single Select, please see the table below for a simulated VIN.
| Email | VIN |
| ----------------------------------------------------------------------------- | ----------------- |
| [smartcar@vehicle-incompatible.com](mailto:smartcar@vehicle-incompatible.com) | 0SCAUDI012FE3B132 |
# Overview
Source: https://smartcar.com/docs/errors/overview
A comprehensive breakout of all Smartcar errors.
# Connect Errors
Connect errors are returned as a parameter in the redirect from Connect.
### Access Denied
| | Code |
| --------------------------------------------------------------------------------------------------- | ------------------------------------------------------ |
| This error occurs when a user denies your application access to the requested scope of permissions. | [access\_denied](/errors/connect-errors/access-denied) |
### Invalid Subscription
| | Code |
| ------------------------------------------------------------------------------------------------------------------------------------------------------------------------- | -------------------------------------------------------------------- |
| This error occurs when a user’s vehicle is compatible but their connected services subscription is inactive because either it has expired or it has never been activated. | [invalid\_subscription](/errors/connect-errors/invalid-subscription) |
### Configuration Error
| | Code |
| ---------------------------------------------------------------------------------------------------------------------- | ------------------------------------------------------------------ |
| This error occurs when the user has encountered an Error page in Connect and has chosen to return to your application. | [configuration\_error](/errors/connect-errors/configuration-error) |
### No Vehicles
| | Code |
| ------------------------------------------------------------------------------------------------------------------------------- | -------------------------------------------------- |
| This error occurs when a vehicle owner has a connected services account, but there are no vehicles associated with the account. | [no\_vehicles](/errors/connect-errors/no-vehicles) |
### Vehicle Incompatible
| | Code |
| --------------------------------------------------------------------------------------------- | -------------------------------------------------------------------- |
| This error occurs when a user tries to authorize an incompatible vehicle in Smartcar Connect. | [vehicle\_incompatible](/errors/connect-errors/vehicle-incompatible) |
### Server Error
| | Code |
| --------------------------------------------------------------------- | ---------------------------------------------------- |
| If there is a server error, the user will return to your application. | [server\_error](/errors/connect-errors/server-error) |
# API Errors
API errors are returned from requests made via Smartcar API.
### Authentication
| | Code |
| -------------------------------------------------------------- | ----------------------------------------------------- |
| Thrown when there is an issue with your authorization headers. | [NULL](/errors/api-errors/authentication-errors#null) |
### Billing
| | Code |
| ------------------------------------------------------------------------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| Thrown when limits have been reached based on your plan or if the feature is not available. | [INVALID\_PLAN](/errors/api-errors/billing-errors#invalid-plan)
[ACCOUNT\_SUSPENDED](/errors/api-errors/billing-errors#account-suspended) |
### Compatibility
| | Code |
| ---------------------------------------------------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| Thrown when Smartcar does not support a make or feature for a vehicle. | [MAKE\_NOT\_COMPATIBLE](/errors/api-errors/compatibility-errors#make-not-compatible)
[VEHICLE\_NOT\_CAPABLE](/errors/api-errors/compatibility-errors#vehicle-not-capable) |
### Connected Services Account
| | Code |
| ----------------------------------------------------------------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| Thrown when there are issues with the user's connected service account. | [ACCOUNT\_ISSUE](/errors/api-errors/connected-service-errors#account_issue)
[VEHICLE\_MISSING](/errors/api-errors/connected-service-errors#vehicle-missing) |
### Permission
| | Code |
| --------------------------------------------------------------------------------------------------------------- | ------------------------------------------------- |
| Thrown when you make a requests to an endpoint associated with permissions not yet granted to your application. | [NULL](/errors/api-errors/permission-errors#null) |
### Rate Limit
| | Code |
| ------------------------------------------------------------------ | ------------------------------------------------------------------------------------------------------------------------------------- |
| Thrown when there is an issue with the frequency of your requests. | [SMARTCAR\_API](/errors/api-errors/rate-limit-errors#smartcar-api)
[VEHICLE](/errors/api-errors/rate-limit-errors#vehicle) |
### Resource Not Found
| | Code |
| -------------------------------------------- | ----------------------------------------------------------------------------------------------------------------------------- |
| Thrown if the requested path does not exist. | [PATH](/errors/api-errors/resource-errors#path)
|
### Server
| | Code |
| ----------------------------------------------------------------------------------------- | ----------------------------------------------------- |
| Thrown when Smartcar runs into an unexpected issue and was unable to process the request. | [INTERNAL](/errors/api-errors/server-errors#internal) |
### Upstream
| | Code |
| ------------------------------------------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| Thrown when the OEM or vehicle failed to process the request. | [INVALID\_DATA](/errors/api-errors/upstream-errors#invalid-data)
[UNKNOWN\_ISSUE](/errors/api-errors/upstream-errors#unknown-issue) |
### Validation
| | Code |
| ------------------------------------------------------------------- | ------------------------------------------------------------------------------------------------------------------------ |
| Thrown if there is an issue with the format of the request or body. | [NULL](/errors/api-errors/validation-errors#null)
[PARAMETER](/errors/api-errors/validation-errors#parameter) |
### Vehicle State
| | Code |
| ---------------------------------------------------------------------------------------------------------------------------------------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| Thrown when a request fails due to the state of a vehicle or logically cannot be completed e.g. you can't start a vehicle charging if it's not plugged in. | [ASLEEP](/errors/api-errors/vehicle-state-errors#asleep)
[VEHICLE\_OFFLINE\_FOR\_SERVICE](/errors/api-errors/vehicle-state-errors#vehicle-offline-for-service) |
# Testing Errors
Source: https://smartcar.com/docs/errors/testing-errors
By launching Connect in [simulated mode](/docs/connect/advanced-config/modes), you're able to test your application against certain errors.
## Connect Errors
You can use the following emails to test a subset of Connect errors:
| Error | Email | VIN |
| ---------------------- | ----------------------------------------------------------------------------- | ----------------- |
| `invalid_subscription` | [smartcar@invalid-subscription.com](mailto:smartcar@invalid-subscription.com) | 0SCAUDI0155C49A95 |
| `vehicle_incompatible` | [smartcar@vehicle-incompatible.com](mailto:smartcar@vehicle-incompatible.com) | 0SCAUDI012FE3B132 |
Visit the [Connect Errors](/errors/overview#connect-errors) page for more details.
## API Errors
You can use use the following emails to test these API error types:
* `COMPATIBILITY`
* `CONNECTED_SERVICES_ACCOUNT`
* `UPSTREAM`
* `VEHICLE_STATE`
| Type | Code | Email | VIN |
| ---------------------------- | ----------------------------- | ---------------------------------------------------------------------------------------------------------------------------------------- | ----------------- |
| `COMPATIBILITY` | `MAKE_NOT_COMPATIBLE` | [COMPATIBILITY.MAKE\_NOT\_COMPATIBLE@smartcar.com](mailto:COMPATIBILITY.MAKE_NOT_COMPATIBLE@smartcar.com) | 0SCLR000270BE6600 |
| `COMPATIBILITY` | `SMARTCAR_NOT_CAPABLE` | [COMPATIBILITY.SMARTCAR\_NOT\_CAPABLE@smartcar.com](mailto:COMPATIBILITY.SMARTCAR_NOT_CAPABLE@smartcar.com) | 0SCLR0002DC18F57F |
| `COMPATIBILITY` | `VEHICLE_NOT_CAPABLE` | [COMPATIBILITY.VEHICLE\_NOT\_CAPABLE@smartcar.com](mailto:COMPATIBILITY.VEHICLE_NOT_CAPABLE@smartcar.com) | 0SCLR00024C7CDF7A |
| `CONNECTED_SERVICES_ACCOUNT` | `ACCOUNT_ISSUE` | [CONNECTED\_SERVICES\_ACCOUNT.ACCOUNT\_ISSUE@smartcar.com](mailto:CONNECTED_SERVICES_ACCOUNT.ACCOUNT_ISSUE@smartcar.com) | 0SCLR00026BAAA136 |
| `CONNECTED_SERVICES_ACCOUNT` | `AUTHENTICATION_FAILED` | [CONNECTED\_SERVICES\_ACCOUNT.AUTHENTICATION\_FAILED@smartcar.com](mailto:CONNECTED_SERVICES_ACCOUNT.AUTHENTICATION_FAILED@smartcar.com) | 0SCLR00021D803C7B |
| `CONNECTED_SERVICES_ACCOUNT` | `NO_VEHICLES` | [CONNECTED\_SERVICES\_ACCOUNT.NO\_VEHICLES@smartcar.com](mailto:CONNECTED_SERVICES_ACCOUNT.NO_VEHICLES@smartcar.com) | 0SCLR00027671837B |
| `CONNECTED_SERVICES_ACCOUNT` | `SUBSCRIPTION` | [CONNECTED\_SERVICES\_ACCOUNT.SUBSCRIPTION@smartcar.com](mailto:CONNECTED_SERVICES_ACCOUNT.SUBSCRIPTION@smartcar.com) | 0SCLR0002B9BAF29F |
| `CONNECTED_SERVICES_ACCOUNT` | `VEHICLE_MISSING` | [CONNECTED\_SERVICES\_ACCOUNT.VEHICLE\_MISSING@smartcar.com](mailto:CONNECTED_SERVICES_ACCOUNT.VEHICLE_MISSING@smartcar.com) | 0SCLR00024E29EEBD |
| `UPSTREAM` | `INVALID_DATA` | [UPSTREAM.INVALID\_DATA@smartcar.com](mailto:UPSTREAM.INVALID_DATA@smartcar.com) | 0SCLR000298A0A649 |
| `UPSTREAM` | `KNOWN_ISSUE` | [UPSTREAM.KNOWN\_ISSUE@smartcar.com](mailto:UPSTREAM.KNOWN_ISSUE@smartcar.com) | 0SCLR0002A8760B72 |
| `UPSTREAM` | `NO_RESPONSE` | [UPSTREAM.NO\_RESPONSE@smartcar.com](mailto:UPSTREAM.NO_RESPONSE@smartcar.com) | 0SCLR0002C23E43AB |
| `UPSTREAM` | `UNKNOWN_ISSUE` | [UPSTREAM.UNKNOWN\_ISSUE@smartcar.com](mailto:UPSTREAM.UNKNOWN_ISSUE@smartcar.com) | 0SCLR0002D4878901 |
| `VEHICLE_STATE` | `ASLEEP` | [VEHICLE\_STATE.ASLEEP@smartcar.com](mailto:VEHICLE_STATE.ASLEEP@smartcar.com) | 0SCLR0002E8896323 |
| `VEHICLE_STATE` | `CHARGING_IN_PROGRESS` | [VEHICLE\_STATE.CHARGING\_IN\_PROGRESS@smartcar.com](mailto:VEHICLE_STATE.CHARGING_IN_PROGRESS@smartcar.com) | 0SCLR000221F1E672 |
| `VEHICLE_STATE` | `CHARGING_PLUG_NOT_CONNECTED` | [VEHICLE\_STATE.CHARGING\_PLUG\_NOT\_CONNECTED@smartcar.com](mailto:VEHICLE_STATE.CHARGING_PLUG_NOT_CONNECTED@smartcar.com) | 0SCLR0002CBFC7C21 |
| `VEHICLE_STATE` | `DOOR_OPEN` | [VEHICLE\_STATE.DOOR\_OPEN@smartcar.com](mailto:VEHICLE_STATE.DOOR_OPEN@smartcar.com) | 0SCLR0002F8BD90B8 |
| `VEHICLE_STATE` | `FULLY_CHARGED` | [VEHICLE\_STATE.FULLY\_CHARGED@smartcar.com](mailto:VEHICLE_STATE.FULLY_CHARGED@smartcar.com) | 0SCLR00029CD9D894 |
| `VEHICLE_STATE` | `HOOD_OPEN` | [VEHICLE\_STATE.HOOD\_OPEN@smartcar.com](mailto:VEHICLE_STATE.HOOD_OPEN@smartcar.com) | 0SCLR000219CD4A2C |
| `VEHICLE_STATE` | `IGNITION_ON` | [VEHICLE\_STATE.IGNITION\_ON@smartcar.com](mailto:VEHICLE_STATE.IGNITION_ON@smartcar.com) | 0SCLR00021D49E58A |
| `VEHICLE_STATE` | `IN_MOTION` | [VEHICLE\_STATE.IN\_MOTION@smartcar.com](mailto:VEHICLE_STATE.IN_MOTION@smartcar.com) | 0SCLR00024F6EE640 |
| `VEHICLE_STATE` | `REMOTE_ACCESS_DISABLED` | [VEHICLE\_STATE.REMOTE\_ACCESS\_DISABLED@smartcar.com](mailto:VEHICLE_STATE.REMOTE_ACCESS_DISABLED@smartcar.com) | 0SCLR0002588A5CC1 |
| `VEHICLE_STATE` | `TRUNK_OPEN` | [VEHICLE\_STATE.TRUNK\_OPEN@smartcar.com](mailto:VEHICLE_STATE.TRUNK_OPEN@smartcar.com) | 0SCLR00023C607FDB |
| `VEHICLE_STATE` | `UNKNOWN` | [VEHICLE\_STATE.UNKNOWN@smartcar.com](mailto:VEHICLE_STATE.UNKNOWN@smartcar.com) | 0SCLR0002E6CC0314 |
| `VEHICLE_STATE` | `UNREACHABLE` | [VEHICLE\_STATE.UNREACHABLE@smartcar.com](mailto:VEHICLE_STATE.UNREACHABLE@smartcar.com) | 0SCLR000247EBF067 |
| `VEHICLE_STATE` | `VEHICLE_OFFLINE_FOR_SERVICE` | [VEHICLE\_STATE.VEHICLE\_OFFLINE\_FOR\_SERVICE@smartcar.com](mailto:VEHICLE_STATE.VEHICLE_OFFLINE_FOR_SERVICE@smartcar.com) | 0SCLR0002AF1D71A1 |
# Dashboard Configurations
Source: https://smartcar.com/docs/getting-started/dashboard/configuration
## Customizing Connect
You can customize the Smartcar Connect flow to match your brand and user experience.
From the dashboard, you can specify which brands you'd like to present to your customers and in which order.
This changes apply only when launching Connect in [default mode](/connect/advanced-config/flows).
### Appearance
You can customize the appearance of the Smartcar Connect flow to match your brand. This includes:
* **Logo**: Upload your logo to be displayed in the Smartcar Connect flow.
* **Brand Name**: Set the name of your brand to be displayed in the Smartcar Connect flow.
* **Brand Icon**: Upload an icon to be displayed in the Smartcar Connect flow.
* **Display Theme**: Select the theme of the Smartcar Connect flow. This includes light, dark, and auto (which will follow the user's system preference).
### Brands
You can customize the brands that are displayed in the Smartcar Connect flow. This includes:
* **Brand Selection**: Select which brands are displayed in the Smartcar Connect flow. All brands supported by Smartcar are enabled by default.
* **Engine type filter**: Select which brands are displayed in the Smartcar Connect flow based on supported engine types (Internal Combustion Engine, Hybrid Electric, Plug-in Hybrid, Battery Electric).
* **Display new brands automatically**: Automatically display new vehicle brands in the Smartcar Connect flow when they become available. This is enabled by default.
### Countries
You can customize the countries that are displayed in the Smartcar Connect flow.
# Connect Insights
Source: https://smartcar.com/docs/getting-started/dashboard/connect-insights
Connect Insights allows you to visualize your Connect conversion rate and identify where individual users are running into issues during onboarding.
## Conversion Rate
For Enterprise customers, the **Conversion Rate** gives you high-level insight into how users are progessing
through the Connect Flow the last two weeks.
## Session Details
Clicking into an event in the **Sessions Feed** will pull up more detailed
information for that specific user's session, allowing you to see where they
dropped off and hilighting issues they may have run into.
If you need to dial into a specific time frame or individual user, you can use
filters such as Date, VIN, User or Session Id to do so.
# Dashboard Multi-Factor Authentication (MFA)
Source: https://smartcar.com/docs/getting-started/dashboard/dashboard-mfa
## Configuring Multi-Factor Authentication
From the settings gear, you can access User Security, which supports Multi-Factor Authentication (MFA). Enable MFA and scan with your preferred authenticator app such as Google Authenticator or 1Password.
Once enabled, each following login will require the use of your authenticator app to provide a unique code for login.
MFA can be disabled for your user at any time, though the authenticator app will be required to disable MFA.
# Dashboard Overview
Source: https://smartcar.com/docs/getting-started/dashboard/overview
All you need to know about the Smartcar Dashboard
You can sign up for a Smartcar account [here](https://dashboard.smartcar.com/signup).
The Smartcar Dashboard allows you to manage your application configurations, connected vehicles and billing settings.
As well as invite team members, create simulated vehicles and set up webhooks.
# Connect Playground
Source: https://smartcar.com/docs/getting-started/dashboard/playground
Creating your Connect URL can be easily done through the Dashboard's new Playground feature.
Your Connect URL gives a path for vehicle owners to provide consent for requested data and/or commands. While this can be done following our [Build the Connect URL](https://smartcar.com/docs/connect/redirect-to-connect) guide, you can also build the URL through the Smartcar Dashboard.
With the Generated Connect URL preview, you can see how your adjustments change the vehicle-owner facing URL.
## 1. Basic Settings
#### Client ID
Your Client ID will automatically populate for the selected Dashboard application.
#### Redirect URI
Select from one of your pre-existing Redirect URIs, or add it now.
#### Mode
Select from Live or [Simulated](https://smartcar.com/docs/getting-started/dashboard/simulator) mode.
## 2. Permissions
In this step, select which permissions your application will request from the vehicle. This may include read access to data like odometer or location, or commands that may lock or unlock the vehicle.
Requested permissions will be presented to the vehicle owner for confirmation before connecting.
## 3. Advanced Settings
Optional advanced settings can be added to your Connect URL.
### State
State is an optional value included as a query parameter in the `REDIRECT_URI` back to your application.
### User
Specify a unique identifier for the vehicle owner to track and aggregate analytics across Connect sessions for each vehicle owner on Dashboard.
Note: Use the `state` parameter in order to identify the user at your callback URI when receiving an authorization or error code after the user exits the Connect flow.
### Make
Allows users to bypass the Brand Selector screen. Valid `makes` can be found in the [makes](/api-reference/makes) section on API reference.
The `single_select_vin` parameter takes precedence over this parameter.
### Country
The two-character ISO country code of the country that your user is located in. You can set the default country for Connect on Dashboard
If no flag is passed or set as default on Dashboard, Smartcar will use the devices IP to set an appropriate country.
This flag determines what brands are listed on the Brand Selector screen in Connect e.g. Renault is available in Europe, but not the US.
### Vehicle Single Select
Sets the vehicle selection behavior of the grant dialog. If enabled, then the user is only allowed to select a single vehicle.
# Vehicle Simulator
Source: https://smartcar.com/docs/getting-started/dashboard/simulator
Before launching your integration, we recommend using Smartcar’s Vehicle Simulator to test your application on a simulated vehicle. With the Simulator, you can choose the vehicle's region, make, model and year as well as the vehicle state to test with. Watch your simulated vehicle travel in real-time from the Smartcar Dashboard as you test API requests from your application with realistic vehicle data responses.
## 1. Create a simulated vehicle
#### Create a simulated vehicle
Begin by logging into the Smartcar Dashboard and navigating to the Simulator tab. Choose your region and select a Smartcar-compatible vehicle using the Make, Model, and Year dropdowns. Or, use the VIN search feature to create a simulated vehicle of the same Make, Model and Year as the VIN entered, as long as the vehicle is compatible with Smartcar. Please note the VIN search is only available for US-based VINs at this time.
#### Review the vehicle capabilities
Confirm the vehicle's supported capabilities includes those you wish to test.
#### Select a vehicle state
Select from one of three available vehicle states for your simulated vehicle. The states vary in duration from 8 to 24 hours and include driving, charging, and parked vehicle states for a wide range of test scenarios.
## 2. Connect your app to the simulated vehicle
Once you've selected a vehicle and state, you are ready to connect the simulated vehicle to your application for testing.
Grab the simulated vehicle credentials
On the simulation screen, you will find a Connect Credentials button that will open a modal containing the simulated vehicle's credentials. You will need these to connect your app and vehicle using Smartcar Connect.
#### Launch Smartcar Connect in simulated mode
When launching Smartcar Connect, pass in the query parameter mode=simulated to enable simulated mode and ensure you can connect to your newly created simulated vehicle. Smartcar's SDKs provide a convenient option to facilitate this:
```js Node
const client = new smartcar.AuthClient({
clientId: CLIENT_ID,
clientSecret: CLIENT_SECRET,
redirectUri: REDIRECT_URI,
mode: "simulated",
});
```
```python Python
client = smartcar.AuthClient(
client_id=CLIENT_ID,
client_secret=CLIENT_SECRET,
redirect_uri=REDIRECT_URI,
mode="simulated"
)
```
```java Java
AuthClient authClient = new AuthClient.Builder()
.clientId(CLIENT_ID)
.clientSecret(CLIENT_SECRET)
.redirectUri(REDIRECT_URI)
.mode("simulated")
.build();
```
```ruby Ruby
client = Smartcar::AuthClient.new({
client_id: CLIENT_ID,
client_secret: CLIENT_SECRET,
redirect_uri: REDIRECT_URI,
mode: "simulated"
})
```
#### Log into a connected services account
After launching Smartcar Connect, select the brand that matches your simulated vehicle and enter the credentials pulled from the Smartcar Dashboard simulation screen on the brand login page. You will then be prompted to grant access to your selected permissions.
Upon completing the Smartcar Connect flow, return to the Smartcar Dashboard. It may take up to a minute to reflect that your vehicle is now connected. Once connected, the vehicle ID will appear next to the vehicle's VIN near the top of the simulation screen.
## 3. Start the simulation and begin testing
Once the vehicle is connected to your application, you are ready to start the simulation and begin making API requests to the simulated vehicle.
### Start the simulation
In the Smartcar Dashboard on the simulation screen for your selected simulated vehicle, start the simulation by pressing the Play button. You have the option to pause, resume or restart the simulation at any time. Additional controls you have over the simulation:
#### Control the simulation speed
You can control the simulation's speed in case you would like to test the vehicle at various stages at a faster or slower pace.
#### Change the simulation stage
Once the simulation has started, you can jump to any stage of the simulation in the event you would like to test the vehicle in a particular state.
#### Set the API response latency
Control the response latency of any API requests you make to the simulated vehicle.
`Ideal` - API responses are returned immediately upon request.
`Realistic` - API responses are delayed a certain amount of time based on average API response latency statistics that Smartcar has gathered for each supported brand.
A breakdown of estimated response latencies can be viewed by clicking on the ‘View latencies’ link directly underneath the Request Latency dropdown in the Dashboard. Please note latency estimates may not be available for all brands. In these cases, the Realistic setting will return immediate responses -- same as Ideal.
#### Change the vehicle state
You can select a different vehicle state for your simulated vehicle at any time. Select the Edit icon in the `Trip type` card on the simulation screen.
### Send an API request to the simulated vehicle
The Smartcar API will return realistic data matching the current state of the simulated vehicle as displayed on the simulation screen. Note that if you've selected Realistic API response latency, the data returned to your application may appear somewhat delayed.
# Single Sign-on (SSO)
Source: https://smartcar.com/docs/getting-started/dashboard/single-sign-on
Smartcar offers SSO through your identity provider (IdP) for Enterprise customers.
## About SSO with Smartcar
Our Dashboard supports SSO integrations with popular identity providers
including:
* Okta
* Microsoft Azure AD
* Google Workspace
* OneLogin
* Ping Identity
* ADFS
* Custom SAML 2.0 providers
To set up SSO for your organization's Dashboard accounts, you'll need to:
1. Be an Owner for an existing Team on an Enterprise plan
2. Verify your domain
3. Configure your identity provider
The following section will guide you through each step of this process.
## Setting up SSO
On Dashboard navigate to **Team settings > Security** and select **Enable SSO**
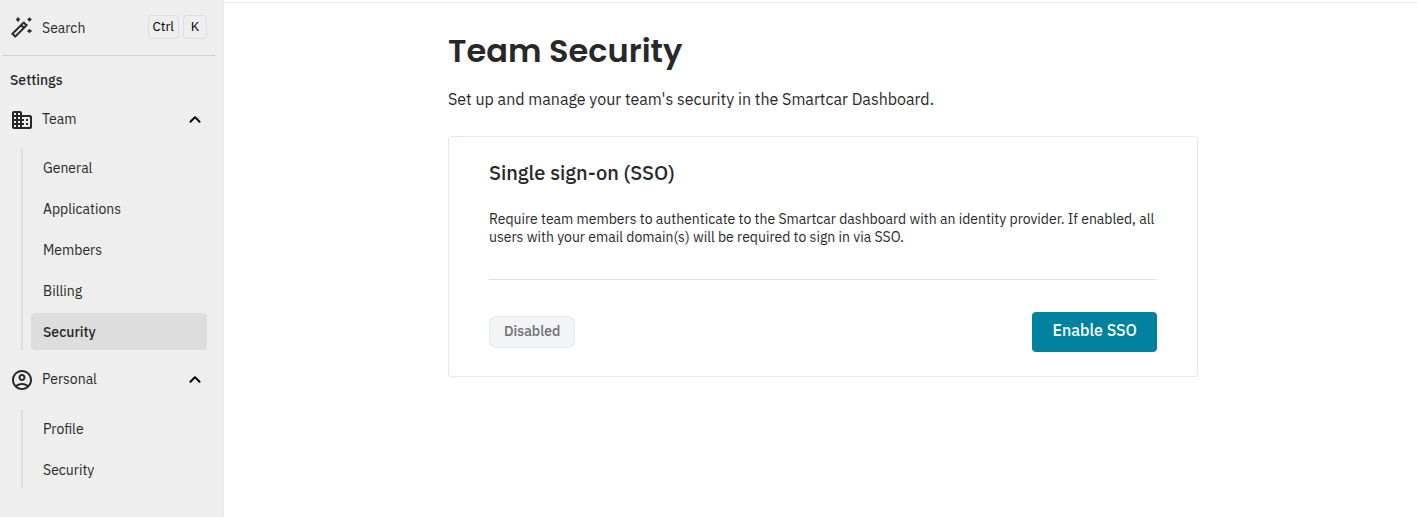
You’ll be redirected to start the SSO configuration process.
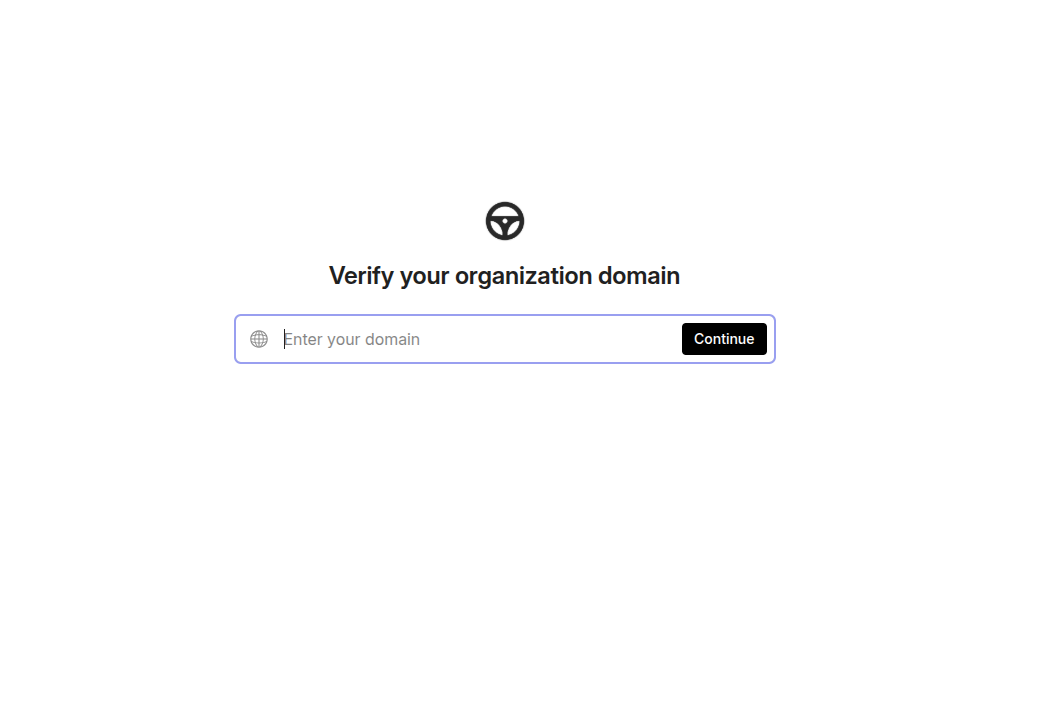
Depending on your DNS provider, you'll receive instructions on how to verify your domain.
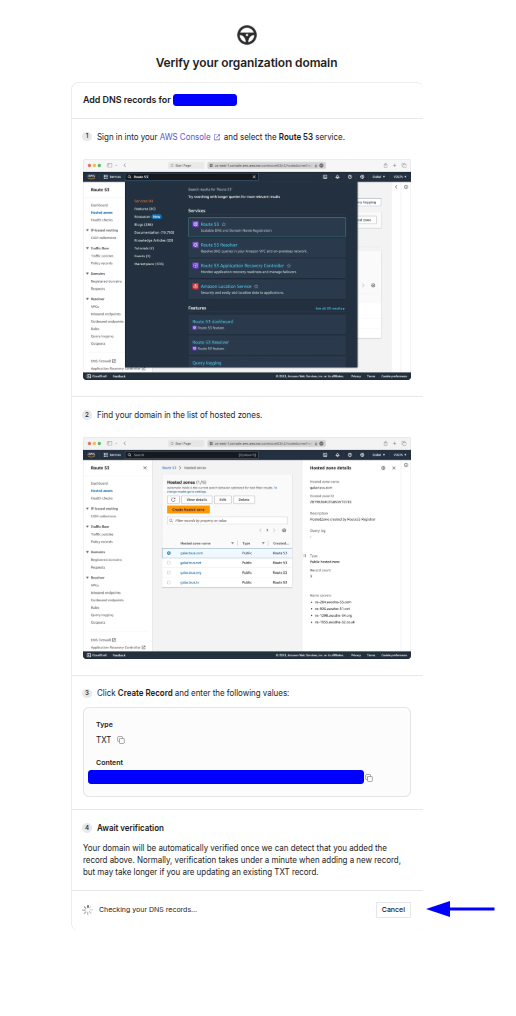
Once your domain is verified, you’ll be able to select your Identity Provider
(IdP).
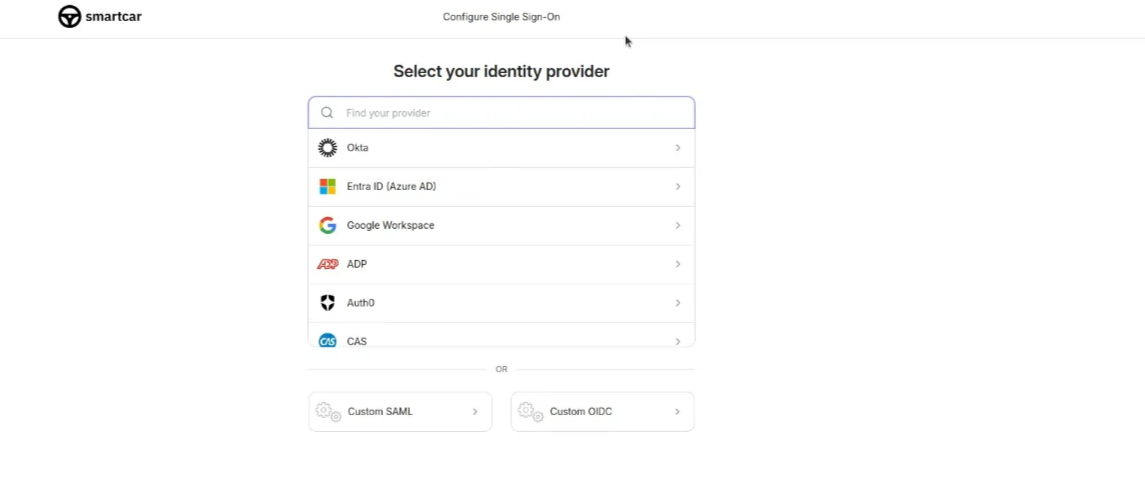
You'll be provided a guide on how to get set up based on your IdP.
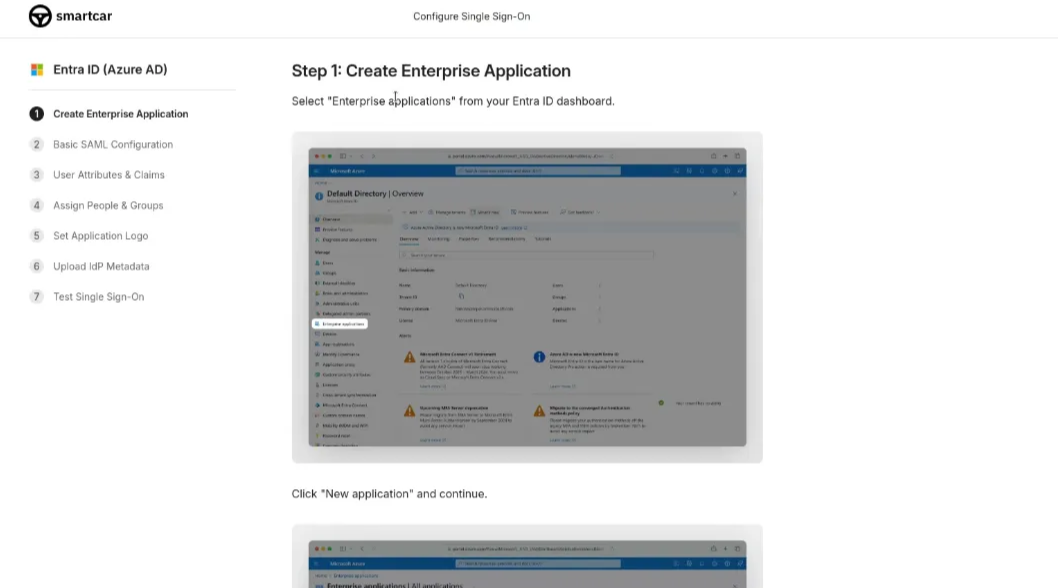
Once you’ve got everything configured, you’ll be prompted to test SSO.
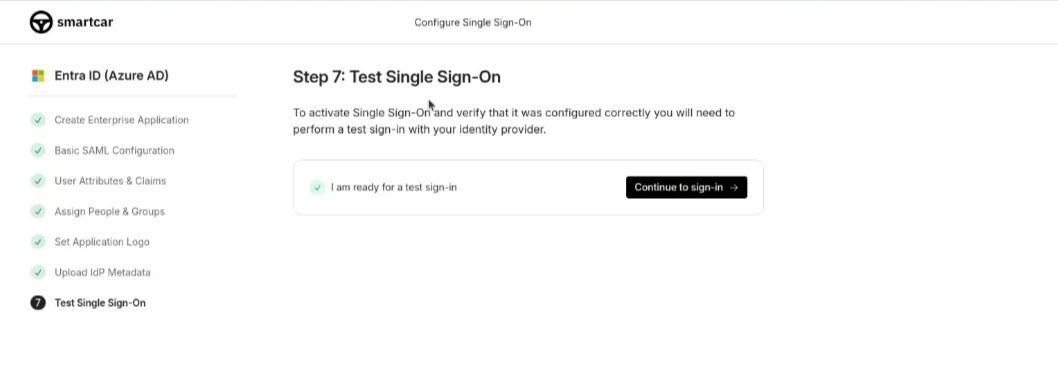
If you exit the flow at any time before finalizing your configuration, you
can jump back in from Dashboard.
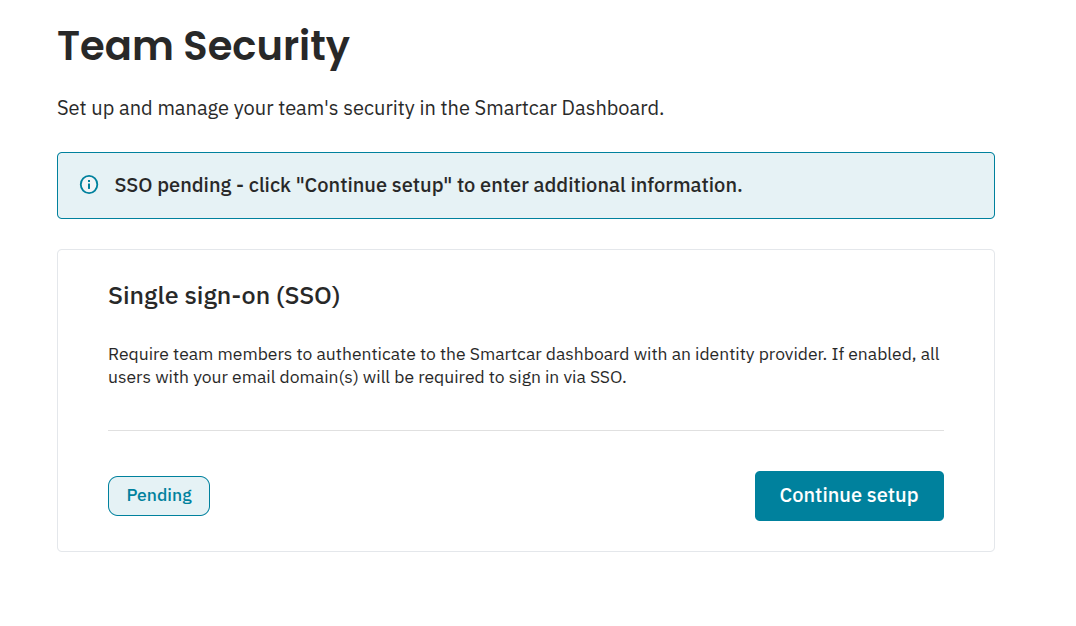
## Disabling SSO
If you have SSO configured, the Team Owner can disable it from **Team
settings > Security**.
Disabling SSO will require you to go through the whole setup process
again.
## Inviting team members with SSO enabled
Once enabled, you’ll need to add members to your team through your identity
provider. Invites via the Dashboard will be disabled while SSO is enabled.
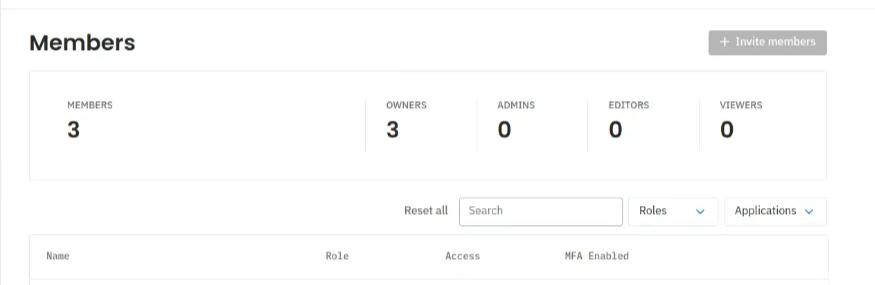
When a newly added member has signed in via SSO, they will have the Viewer role
by default. Other team members with the appropriate role can promote these
members as needed.
If a user creates a Dashboard account with their email prior to being added
to your IdP, they will be added to their own Team initially. When they are
added to your IdP they will be able to switch Teams in Dashboard.
## A note on aliased emails
If members of your team have Dashboard accounts using email aliases prior to
enabling SSO e.g. [rover+thebest@dog.com](mailto:rover+thebest@dog.com), once SSO is enabled for your Team and
they’re added to your IdP, their login will be tied to their non-aliased email
address e.g. [rover@dog.com](mailto:rover@dog.com).
SSO is only available on the **Enterprise** plan, please reach out to your Account Manager for more details.
# Team Settings
Source: https://smartcar.com/docs/getting-started/dashboard/teams
Your Smartcar Team is the central hub for managing your applications, vehicles, members, security settings, and billing information.
Your Smartcar Team allows you to collaborate with other members and manage your applications in one place.
## Members
You can add team members to your Smartcar account on any plan for free.
Team members can be added to your Smartcar account by navigating to the **Settings** tab in the Dashboard and selecting **Members** under the **Team** section.
## Roles and Permissions
Team members can be added on any plan. Specific roles are available on our [Enterprise plan](https://smartcar.com/pricing).
| | Owner | Admin | Editor | Viewer |
| ----------------------------- | ----------------------------------- | ----------------------------------- | ------------------------------------ | ------------------------------------ |
| Manage billing | ✅ | | | |
| View billing | ✅ | | | |
| Delete applications | ✅ | | | |
| Modify team settings | ✅ | | | |
| Remove team members | ✅ | ✅ | | |
| Modify team members | ✅ | ✅ | | |
| Create applications | ✅ | ✅ | | |
| Modify applications | ✅ | ✅ | ✅ | |
| View applications | ✅ | ✅ | ✅ | ✅ |
| View team members | ✅ | ✅ | ✅ | ✅ |
Editors and Viewers can be limited to specific applications.
## Roles
### Owner
Owners have full control and access to the team. They're able to add and remove members, create and modify applications, edit billing information and more.
A team **can** have multiple Owners, but **must** have at least one.
### Admin
Admins have similar access to Owners but can't edit or view billing information.
### Editor
Editors have similar access to Admins but are limited to specific applications. They're unable to manage team members, view the team's billing information, create applications, or delete applications they're allowed to access.
### Viewer
Viewers have similar permissions to Editors but only have read-only access to applications they've been invited to.
# Charging station utilization
Source: https://smartcar.com/docs/getting-started/guides/charging-station-utilization
This guide explains how to use the Smartcar API to enhance charging station discoverability by leveraging vehicle data such as state of charge, battery level, range, and location. These capabilities allow developers to help EV drivers find nearby charging stations based on real-time vehicle data.
## Prerequisites: Creating your application
Before implementing the Smartcar API for charging station discoverability,
ensure you have:
* Created a Smartcar Account and registered your application to obtain your
`client_id` and `client_secret`.
* Set up Smartcar Connect Flow to authenticate and authorize users.
For more details, refer to the [Getting Started
Guide](/getting-started/introduction).
## Smartcar API Endpoints for charging station discoverability
Use the following Smartcar API endpoints to help drivers locate nearby charging
stations based on their vehicle’s current range and location.
### 1. Determine EV Range
Once a vehicle is authorized via Smartcar’s Connect flow, you can use the
vehicle’s access token to retrieve the current battery level and range through
the [GET /battery](/api-reference/evs/get-battery-level) endpoint.
```curl Range Remaining and SoC
GET /vehicles/{vehicle_id}/battery
```
```json Example Response
{
"percentRemaining": 0.3,
"range": 40.5
}
```
### 2. Retrieve Vehicle Location
To locate nearby charging stations based on the vehicle’s current location, use
the [GET /location](/api-reference/get-location) endpoint.
```curl Location
GET /vehicles/{vehicle_id}/location
```
```json Example Response
{
"latitude": 37.4292,
"longitude": -122.1381
}
```
### 3. Calculate Distance to Charging Stations
Once you have the vehicle's location, compare it with nearby charging stations’
coordinates. Charging station locations can be sourced from a Charge Point
Operator (CPO) or through third-party services such as Google’s Places API.
Calculate the distance and estimated time to reach these stations using Google’s
Distance Matrix API or a similar service.
* Sourcing Charging Station Data: While Smartcar provides vehicle location data,
you’ll need to source station coordinates from a CPO database or third-party API
like Google’s Places API.
* Distance Calculation: Use Google’s Distance Matrix API to compute the travel
distance and time, factoring in real-time traffic conditions and travel modes.
This process helps drivers locate the nearest available charging stations in
real time, offering a smooth and integrated experience.
This process helps drivers locate the nearest available charging stations in
real time, offering a smooth and integrated experience.
### 4. Send Destination to Vehicle Navigation (Optional)
Once you’ve identified the nearest charging station, guide the driver to the
location. Depending on the vehicle’s capabilities, there are two options for
navigation:
1. **Mobile Navigation Apps**: For vehicles that don’t support in-dash
navigation, dispatch the destination coordinates to a mobile app (e.g., Google
Maps, Apple Maps). This ensures drivers can still navigate using their
smartphone..
2. **Send to in-dash navigation**: For vehicles that support in-dash
navigation, Smartcar allows you to send destination coordinates directly to the
onboard system. This allows for seamless, in-vehicle routing. You can check
which brands support this feature by visiting the Supported Brands section.
Use [Send Destination](/api-reference/send-destination-to-vehicle) for supported
vehicles to send coordinates to the vehicle's navigation.
```curl Send Destination
POST /vehicles/navigation/destination
{
"latitude": 37.4292,
"longitude": -122.1381
}
```
## FAQs
Scheduled polling is recommended for passive monitoring of vehicle data. For
real-time use cases, such as finding the nearest charging station based on
current range and location, sending requests on-demand is more efficient.
To locate nearby charging stations, you will need external data sources to
locate nearby charging stations. This data can be sourced from Charge Point
Operators (CPOs) or third-party services like Google’s Places API. You may
also use public databases such as the National Renewable Energy Laboratory
(NREL) API for detailed information on charging station availability and
locations.
You may also wish to use Google’s Distance Matrix API to help
calculate drive distance and time based on real-time traffic conditions.
Yes, Smartcar’s API enables integration with any charging station network by
providing vehicle data through our platform.
# Preventive maintenance scheduling
Source: https://smartcar.com/docs/getting-started/guides/preventive-maintenance-scheduling
This guide explains how to use the Smartcar API to proactively automate preventive maintenance scheduling. By leveraging data such as odometer readings, engine oil life, Diagnostic Trouble Codes (DTCs), and system status, you can reduce vehicle downtime, improve reliability, and enhance customer satisfaction.
## Prerequisites: Creating Your Application
Before implementing preventive maintenance capabilities ensure you have:
* Created a Smartcar account and registered your application to obtain your
`client_id` and `client_secret`.
* Set up the Smartcar Connect flow to authenticate and authorize users.
For more details, refer to the [Getting Started Guide](https://smartcar.com/docs/getting-started/).
## Smartcar API Endpoints for Preventive Maintenance
Use the following endpoints to build your preventive maintenance solution:
### 1. Track Odometer Readings
Retrieve odometer data to schedule maintenance services such as tire rotations or oil changes based on mileage.
```curl Odometer
curl "https://api.smartcar.com/v2.0/vehicles/{id}/odometer" \
-H "Authorization: Bearer {token}" \
-X "GET"
```
```json Example Response
{
"distance": 104.32
}
```
**Use Case**
Send a notification when a vehicle’s odometer reading reaches maintenance intervals, such as every 5,000 miles.
### 2. Monitor Engine Oil Life
Retrieve oil life data to prompt users when an oil change is due.
```curl Engine Oil Life
curl "https://api.smartcar.com/v2.0/vehicles/{id}/engine/oil" \
-H "Authorization: Bearer {token}" \
-X "GET"
```
```json Example Response
{
"lifeRemaining": 0.35
}
```
**Use Case**
Trigger a service reminder when oil life falls below 20% to prevent engine damage.
### 3. Retrieve Diagnostic Trouble Codes (DTCs)
DTCs help you identify and diagnose specific vehicle issues, allowing for quick and accurate repairs.
```curl DTCs
curl "https://api.smartcar.com/v2.0/vehicles/{vehicleId}/diagnostics/dtcs" \
-H "Authorization: Bearer {token}" \
-X "GET"
```
```json Example Response
{
"activeCodes": [
{
"code": "P302D",
"timestamp": "2024-09-05T14:48:00.000Z"
}
]
}
```
**Use Case**
Notify a driver immediately if a new trouble code is detected, enabling faster
response times.
### 4. Monitor Vehicle System Status
Retrieve a snapshot of key systems like the engine and battery to assess health
and detect anomalies.
```curl System Status
curl "https://api.smartcar.com/v2.0/vehicles/{vehicleId}/system-status" \
-H "Authorization: Bearer {token}" \
-X "GET"
```
```json Example Response
{
"systems": [
{
"systemId": "SYSTEM_TPMS",
"status": "ALERT",
"description": "Left rear tire sensor battery low"
},
{
"systemId": "SYSTEM_AIRBAG",
"status": "OK",
"description": null
},
{
"systemId": "SYSTEM_MIL",
"status": "OK",
"description": null
},
]
}
```
**Use Case**
Notify users or fleet operators if the engine status changes to warning or the battery health declines.
## Webhooks for Preventive Maintenance
Webhooks enable real-time notifications for certain vehicle events, reducing the
need for polling. Examples include:
* **DTC Updates**: Receive a webhook when a new trouble code is logged.
* **System Changes**: Get updates for changes in engine or battery status.
### Setting Up Webhooks
To receive real-time notifications (e.g., when new DTCs appear), consider
setting up webhooks:
1. **Create a Webhook:** Configure a secure endpoint on your server to handle POST
requests from Smartcar.
2. **Register the Webhook:** Specify which events (such as DTC) you want to
subscribe to.
3. **Instant Alerts:** When an event triggers, Smartcar sends a payload to your endpoint, enabling immediate responses (like sending notifications or scheduling service).
See the [Smartcar Webhooks Documentation](/getting-started/tutorials/webhooks-diagnostic) for more information.
### Example Workflow
1. **Authorization**: The user logs in via Smartcar Connect to grant access to
vehicle data.
2. **Monitoring**: Your backend polls GET endpoints (e.g., odometer, oil, system
status) or listens for webhook events.
3. **Threshold Detection**: Evaluate data (e.g., oil life \< 20%, mileage > 5,000
miles) and identify DTCs or system warnings.
4. **Notifications**: Send push notifications, emails, or SMS to users with
maintenance reminders or alerts.
5. **Ongoing Updates**: Combine periodic polling and webhooks to ensure vehicles stay road-ready.
## FAQs
No. Data availability depends on the OEM, model, and year. Check the
[Compatible Vehicles](https://smartcar.com/product/compatible-vehicles)
page for details.
Yes, webhooks can be used for supported events such as DTC updates or
system status changes.
# Drive revenue with real-time diagnostics
Source: https://smartcar.com/docs/getting-started/guides/real-time-vehicle-diagnostics
This guide explains how to use the Smartcar API to provide real-time vehicle diagnostics by leveraging data such as Diagnostic Trouble Codes (DTCs), overall system health, and odometer readings. These capabilities allow developers and service providers to proactively identify issues, schedule service visits, and reduce vehicle downtime.
## Prerequisites: Creating Your Application
Before implementing the Smartcar API for real-time vehicle diagnostics, ensure
you have:
* Created a Smartcar account and registered your application to obtain your
`client_id` and `client_secret`.
* Set up the Smartcar Connect flow to authenticate and authorize users.
For more details, refer to the [Getting Started Guide](https://smartcar.com/docs/getting-started/).
## Smartcar API Endpoints for Vehicle Diagnostics
Use the following Smartcar API endpoints (and optionally, webhooks) to pinpoint
issues, check vehicle health, and keep track of maintenance schedules based on
odometer data.
* [GET DTCs](/api-reference/get-dtcs)
* [GET System Status](/api-reference/get-system-status)
* [GET Odometer](/api-reference/get-odometer)
### 1. Retrieve Diagnostic Trouble Codes (DTCs)
Once a vehicle is authorized via Smartcar’s Connect flow, you can use the
vehicle’s access token to retrieve DTCs from the following endpoint:
```curl DTCs
curl "https://api.smartcar.com/v2.0/vehicles/{vehicleId}/diagnostics/dtcs" \
-H "Authorization: Bearer {token}" \
-X "GET"
```
```json Example Response
{
"activeCodes": [
{
"code": "P302D",
"timestamp": "2024-09-05T14:48:00.000Z"
},
{
"code": "xxxxx",
"timestamp": null
},
...
]
}
```
* **DTCs:** Provide specific error codes and descriptions of active faults for faster troubleshooting.
* **Service Scheduling:** Automate scheduling or send push notifications to alert customers of potential issues.
### 2. Monitor Vehicle Health
Stay informed of the overall vehicle condition by using the system status
endpoint to retrieve real-time data about critical components like the engine
and battery.
```curl System Status
curl "https://api.smartcar.com/v2.0/vehicles/{vehicleId}/system_status" \
-H "Authorization: Bearer {token}" \
-X "GET"
```
```json Example Response
{
"systems": [
{
// System ID from Smartcar unified system definition list
"systemId": "SYSTEM_TPMS",
"status": "ALERT",
"description": "Left rear tire sensor battery low"
},
{
"systemId": "SYSTEM_AIRBAG",
"status": "OK",
"description": null
},
{
"systemId": "SYSTEM_MIL",
"status": "OK",
"description": null
},
...
]
}
```
* **Engine Status:** Detect early signs of malfunction (e.g., overheating, low oil pressure).
* **Battery Health:** Monitor battery voltage, proactively suggesting replacements or checks.
### 3. Track Odometer Readings
Use the odometer endpoint to maintain timely service intervals (oil changes,
inspections, etc.) based on the actual mileage driven.
```curl Odometer
curl "https://api.smartcar.com/v2.0/vehicles/{vehicleId}/odometer" \
-H "Authorization: Bearer {token}" \
-X "GET"
```
```json Example Response
{
"distance": 104.32
}
```
* **Maintenance Scheduling:** Automatically notify drivers or fleet managers when mileage thresholds are reached.
* **Fleet Management:** Consolidate odometer data across multiple vehicles to streamline service for an entire fleet.
## Webhooks (Optional)
To receive real-time notifications (e.g., when new DTCs appear), consider
setting up webhooks:
1. **Create a Webhook:** Configure a secure endpoint on your server to handle POST
requests from Smartcar.
2. **Register the Webhook:** Specify which events (such as DTC) you want to
subscribe to.
3. **Instant Alerts:** When an event triggers, Smartcar sends a payload to your endpoint, enabling immediate responses (like sending notifications or scheduling service).
See the [Smartcar Webhooks Documentation](/getting-started/tutorials/webhooks-diagnostic) for more information.
## Putting It All Together
1. Retrieve DTCs: Quickly detect and diagnose specific issues.
2. Monitor Vehicle Health: Stay current on engine, battery, and other key
systems.
3. Track Odometer: Automate maintenance scheduling based on real-time mileage.
4. Use Webhooks (Optional): Get instant notifications to further reduce
downtime.
By combining these capabilities, you can deliver proactive and data-driven services—leading to fewer unexpected breakdowns, optimized service operations, and higher customer satisfaction.
## FAQs
You can set up periodic polling to check for new DTCs or system
changes—alternatively, leverage webhooks for real-time alerts when new
diagnostic codes appear or mileage passes certain thresholds
Core diagnostic data is available directly from Smartcar. For
comprehensive service management, you may integrate a CRM or maintenance
scheduling system. However, no extra vehicle hardware is needed.
Yes, Smartcar’s API enables integration with any charging station network by
providing vehicle data through our platform.
# Enabling simplified payments
Source: https://smartcar.com/docs/getting-started/guides/simplified-payments
This guide outlines how charge point operators can utilize the Smartcar API to implement plug-and-charge capabilities, enabling automatic billing and session management through vehicle identification and charging data.
## Prerequisites
Before implementing plug-and-charge capabilities, ensure you have:
* Created a Smartcar account and registered your application to obtain your
client\_id and client\_secret.
* Set up the Smartcar Connect flow to authenticate and authorize users.
For more details, refer to the [Getting Started Guide](https://smartcar.com/docs/getting-started/).
## How Plug-and-Charge Works
Plug-and-charge allows drivers to initiate charging sessions and handle payments
automatically without interacting with external apps or card readers. By
integrating with the Smartcar EV Charging API, operators can:
* **Automate billing** using vehicle identification data (VIN).
* **Control charging** sessions remotely to provide a seamless user experience.
This guide details how to use specific Smartcar API endpoints to implement
plug-and-charge functionality for electric vehicles.
## API Endpoints for Plug-and-Charge Integration
### 1. Retrieve VIN
The [GET /vin](/api-reference/evs/get-vin) endpoint helps link a vehicle to the
driver's account for billing by returning the unique vehicle identification
number, used for identifying and authenticating vehicles during plug-and-charge.
```curl VIN
GET /vehicles/{vehicle_id}/vin
```
```json Example Response
{
"vin" : "2SCTESLFHL4SB3S9S"
}
```
### 2. Retrieve Battery State of Charge (SoC)
You can retrieve the current battery level and range through
the [GET /battery](/api-reference/evs/get-battery-level) endpoint. The battery’s
state of charge is essential for calculating energy consumption during charging.
```curl Range Remaining and SoC
GET /vehicles/{vehicle_id}/battery
```
```json Example Response
{
"percentRemaining": 0.3,
"range": 40.5
}
```
### 3. Remote Start and Stop Charging
Use the [POST /charge](/api-reference/evs/control-charge) endpoint to initiate
and end charging sessions remotely.
```curl Start charge
POST /vehicles/{vehicle_id}/charge
{
"action" : "START"
}
```
```json Example Response
{
"message" : "success"
}
```
```curl Stop charge
POST /vehicles/{vehicle_id}/charge
{
"action" : "STOP"
}
```
```json Example Response
{
"message" : "success"
}
```
## Implementation Workflow
### 1.Authorize Vehicle Access:
Ensure the vehicle is authenticated through the Smartcar Connect flow to allow
API access.
### 2.Check Battery State:
Retrieve the state of charge (SoC) using GET /battery to determine the current
battery level. This ensures that charging sessions start when necessary, and it
allows for accurate tracking of energy consumption from the beginning of the
session.
### 3.Identify Vehicle
Retrieve the VIN using GET /vin to identify the vehicle and link it with the
driver’s account for billing.
### 4.Initiate Charging
Start the charging process remotely using POST /charge/start. The vehicle’s VIN
will be used for billing.
### 5.Monitor Charging Session
Retrieve the state of charge with GET /battery to monitor energy consumption
during the session.
### 6.Stop Charging
End the session with POST /charge/stop once the desired energy level is reached
or based on scheduled requirements.
## Best Practices
* **Link VIN to Payment Account:** Always ensure that the VIN is associated with a valid payment account before initiating plug-and-charge.
* **Monitor SoC Continuously:** Regularly monitor the battery’s SOC to determine the energy consumed during charging, ensuring accurate billing.
* **Use Secure API Calls**: Ensure secure authentication for requests to restrict charging control to authorized users only.
## FAQs
The VIN retrieved through the Smartcar API is linked to the driver’s
payment account, enabling automatic billing based on energy consumption
during charging sessions.
Smartcar supports over 200 EV models across North America and Europe. For a complete list, refer to the [Smartcar Compatibility Matrix](https://smartcar.com/product/compatible-vehicles).
Yes, Smartcar’s API enables integration with any charging station network by
providing vehicle data through our platform.
# Plan Your Integration
Source: https://smartcar.com/docs/getting-started/integration-overview
There are 3 main steps to integrate Smartcar into your application to make API requests to vehicles:
1. Launch Connect to get consent from the vehicle owner
2. Fetch an access token
3. Make a request to the vehicle
## The architecture that's right for you
[Smartcar Connect](/connect/what-is-connect) is Smartcar’s [OAuth 2.0](https://oauth.net/2/) authorization flow. It is your users’ first and only touchpoint with Smartcar. After a user enters the Smartcar Connect
flow and authorizes their vehicle(s), your application is able to make requests to the Smartcar API. Those requests allow your application to retrieve information and send commands to the
user’s vehicle(s), such as reading the odometer and unlocking the doors.
Depending on your web or mobile application architecture, you can implement the Smartcar Connect flow in different ways.
Using the Smartcar iOS SDK or the Smartcar Android SDK, your application launches Smartcar Connect in a browser view. The user logs in with the username and password of their vehicle’s connected services account and grants your application access to their vehicle.
The browser view gets redirected to a special custom-scheme `REDIRECT_URI`. For more details on how to configure a custom-scheme `REDIRECT_URI`, see our API reference.
Once the user has completed the Smartcar Connect flow, the completion handler in the iOS or Android SDK is invoked with the authorization code.
Your mobile application sends the authorization `code` to its back-end service.
In order to exchange the authorization `code` for an `ACCESS_TOKEN`, your application makes a request to the Smartcar API. This request must contain the authorization code along with your application’s `CLIENT_ID` and `CLIENT_SECRET`.
In response, Smartcar returns an `ACCESS_TOKEN` and a `REFRESH_TOKEN`. These should be stored in your application’s database.
Using the `ACCESS_TOKEN`, your application can now make API requests to the user’s vehicle.
Using the [Smartcar JavaScript SDK](https://github.com/smartcar/javascript-sdk), your application launches Smartcar Connect in a pop-up window. The user logs in with the username and password of their vehicle’s connected services account and grants your application access to their vehicle. If you are interested in building a web application that uses Node for API access, check out our [starter applications on Github](https://github.com/smartcar/starter-app-react-node).
The user’s browser gets redirected to a special Smartcar-hosted `REDIRECT_URI`. For more details on how to configure a Smartcar-hosted `REDIRECT_URI`, see our [API reference](/api-reference).
Once the user has completed the Smartcar Connect flow, the `onComplete` callback in the JavaScript SDK is invoked with the authorization `code`.
Your application front end sends the authorization `code` to its back-end service.
In order to exchange the authorization `code` for an `ACCESS_TOKEN`, your application makes a request to the Smartcar API. This request must contain the authorization code along with your application’s `CLIENT_ID` and `CLIENT_SECRET`.
In response, Smartcar returns an `ACCESS_TOKEN` and a `REFRESH_TOKEN`. These should be stored in your application’s database.
Using the `ACCESS_TOKEN`, your application can now make API requests to the user’s vehicle.
You can use your preferred language framework for a server-side setup as long as you use [OAuth2 Retrieval protocol](https://oauth.net/2/). In this case:
1. Your application redirects the user’s browser to Smartcar Connect.
2. The user logs in with the username and password of their vehicle’s connected services account.
3. The user grants your application access to their vehicle.
The Smartcar Connect flow is now complete and the user’s browser gets redirected to a specified `REDIRECT_URI`. Your application’s server, which is listening at the `REDIRECT_URI`, retrieves the authorization `code` from query parameters in the `REDIRECT_URI`.
In order to exchange the authorization `code` for an `ACCESS_TOKEN`, your application makes a request to the Smartcar API. This request must contain the authorization `code` along with your application’s `CLIENT_ID` and `CLIENT_SECRET`.
In response, Smartcar returns an `ACCESS_TOKEN` and a `REFRESH_TOKEN`. These should be stored in your application’s database.
Using the `ACCESS_TOKEN`, your application can now make API requests to the user’s vehicle.
## Let's get building
Now you have an idea of how Smartcar works, take a look at one of our Getting Started guides in the tutorials section to get connected to a vehicle.
{/*
*/}
{/* ## 2. Adapt Smartcar Connect to your use case
### Fit Smartcar Connect into the user journey
To increase conversions and maximize your users’ satisfaction, it is crucial to launch Smartcar Connect at the right moment in your product’s user journey.
We recommend prompting the user to connect their car through Smartcar Connect while they are onboarding their vehicle to your application for the first time.
### Standard: Let the user connect one or more vehicles
In the standard Smartcar Connect flow, the user selects their car brand and logs in with the username and password of their vehicle’s connected services account. On the next screen, the user can see all vehicles associated with their account. The user can select one or multiple of these vehicles and grant your application access to them.
We recommend using the standard Smartcar Connect flow whenever you don’t yet know the make or VIN of your user’s vehicle(s). If you know the make or VIN of your user’s vehicle(s), we recommend personalizing the Smartcar Connect flow in the following ways:
### Optional: Skip the car brand selection screen
If you know the make of your user’s vehicle(s) prior to launching Smartcar Connect, we recommend using [Brand Select](https://smartcar.com/docs/api#brand-select). Brand Select causes the user to automatically skip the car brand selection screen. Instead, the user lands directly on their vehicle brand’s login screen. This speeds up the onboarding experience and increases conversions.
### Optional: Check a vehicle’s eligibility before launching Smartcar Connect
If you know the VIN of your user’s vehicle prior to launching Smartcar Connect, we recommend using the [Compatibility API](/api-reference). The Compatibility API lets you verify a vehicle’s eligibility before sending a user through Smartcar Connect. That way, your application will show Smartcar Connect to users with compatible vehicles only.
The Compatibility API is only available for vehicles sold in the United
States.
### Optional: Make sure the user connects the right vehicle
If you know the VIN of your user’s vehicle prior to launching Smartcar Connect, we recommend using [Single Select](https://smartcar.com/docs/api#single-select). Single Select causes the user to skip the car brand selection screen. It also limits them to connecting only the vehicle with that specific VIN, even if the user has registered multiple vehicles under the same connected services account. This simplifies the user experience and guarantees that the user connects the right vehicle to your application.
## 3. Set optional and/or required permissions
When [redirecting a user to Smartcar Connect](https://smartcar.com/docs/api#smartcar-connect), you need to include a `scope` parameter that indicates which permissions your application requests access to. Every vehicle endpoint has a permission name associated with it. For example, the permission name for the [/fuel endpoint](/api-reference/EVs/EV-charging-limit) is `read_fuel`. Please see our [API reference](/api-reference) for a full list of all permission names.
### Optional permissions
Not all vehicles are capable of all permissions. For example, Tesla vehicles aren’t capable of the `read_fuel` permission, because they don’t have fuel tanks. In order to handle this smoothly, all permissions that your application requests access to in Smartcar Connect are optional by default.
For example, let’s assume that your app requests access to the `read_location` and the `read_fuel` permissions. A user connects their Tesla vehicle through Smartcar Connect. The user is able to successfully authorize their vehicle, because both, the `read_location` and the `read_fuel` permission are optional. In the background, your application is granted permission to the `read_location` endpoint only, because the vehicle is incapable of the read_fuel permission.
In order to verify which permissions your application was granted access to, make a request to the [/permissions endpoint](/api-reference/vehicles/revoke-access):
```java Node
const permissions = await vehicle.permissions();
console.log(permissions);
```
```python Python
response = vehicle.permissions()
print(response)
```
```java Java
ApplicationPermissions response = vehicle.permissions();
System.out.println(response);
```
```ruby Ruby
response = vehicle.permissions
puts response.permissions
```
### Required permissions
If certain permissions are essential to your application’s use case, you can make them required by adding the `required`: prefix to each pertaining permission within the `scope` parameter (e.g. `required:read_fuel`). The user is then able to authorize a vehicle only if the vehicle is capable of all required permissions.
For example, let’s assume that you mark both `read_location` and `read_fuel` as required in the scope parameter. A user tries to connect their Tesla vehicle through Smartcar Connect. As Tesla vehicles are incapable of the read_fuel permission, the user isn’t able to successfully authorize their vehicle. Instead, Smartcar sends the vehicle_incompatible error back to your application.
```java Node
const client = new smartcar.AuthClient({
clientId: CLIENT_ID,
clientSecret: CLIENT_SECRET,
redirectUri: REDIRECT_URI,
});
const scope = ["required:read_location", "required:read_fuel"];
const authUrl = client.getAuthUrl(scope);
```
```python Python
client = smartcar.AuthClient(
client_id=CLIENT_ID,
client_secret=CLIENT_SECRET,
redirect_uri=REDIRECT_URI,
)
scope = ['required:read_location', 'required:read_fuel']
auth_url = client.get_auth_url(scope=scope)
```
```java Java
AuthClient authClient = new AuthClient.Builder()
.clientId(CLIENT_ID)
.clientSecret(CLIENT_SECRET)
.redirectUri(REDIRECT_URI)
.build();
String[] scope = new String[]{"required:read_location", "required:read_fuel"};
String authUrl = authClient.authUrlBuilder(scope).build();
```
```ruby Ruby
client = Smartcar::AuthClient.new({
client_id: CLIENT_ID,
client_secret: CLIENT_SECRET,
redirect_uri: REDIRECT_URI,
})
scope = %w(required:read_location required:read_fuel)
auth_url = client.get_auth_url(scope)
```
*/}
# Introduction to Smartcar's API
Source: https://smartcar.com/docs/getting-started/introduction
Learn about how to build applications that connect to millions of vehicles around the world.
Smartcar is the only car API built with the highest standard for privacy and security. We allow your application to communicate with millions of vehicles easily and securely while giving vehicle owners the choice of how they share their data.
Below are a few concepts to help you get familiar with Smartcar's API.
Let’s get building!
## What you need before you get started
Before making requests to a vehicle, the owner must grant your application permission to access their data. To request permission from the vehicle owner, you must present the Smartcar Connect flow to the user. To launch Smartcar Connect, you need to:
Register an application and get your `CLIENT_ID` and `CLIENT_SECRET`. Set a `REDIRECT_URI` to the path where Smartcar will redirect your users after they grant permission to your app. You can get these from the [Smartcar Dashboard](https://dashboard.smartcar.com/).
With your `CLIENT_ID` and `REDIRECT_URI` you can launch [Connect](/connect). This is how you and your users can add vehicles to your application.
Once users authorize your app to access their vehicles, you receive a `code` that you can exchange for an `ACCESS_TOKEN` and `REFRESH_TOKEN`. Make sure to store these tokens in a secure place (like your application's database). You need the access token to make requests to the Smartcar API. More information can be found in our [Token Management Overview](https://smartcar.com/docs/connect/token-management/overview).
# Test Your Integration
Source: https://smartcar.com/docs/getting-started/test-your-integration
Before launching your integration, use Smartcar’s simulated mode to quickly test your application.
If you prefer to control the vehicle type and receive consistent data in API responses, consider using Smartcar’s [Vehicle Simulator](/getting-started/dashboard/simulator)
If you are not testing with a simulated vehicle, Simulated mode will randomized data for every API request.
## Test successful API requests
### 1. Enabling simulated mode
When launching Smartcar Connect, pass in the query parameter `mode=simulated` to enable simulated mode. Smartcar’s SDKs provide a convenient option to facilitate this:
```node Node
const client = new smartcar.AuthClient({
clientId: CLIENT_ID,
clientSecret: CLIENT_SECRET,
redirectUri: REDIRECT_URI,
mode: 'simulated',
});
```
```python Python
client = smartcar.AuthClient(
client_id=CLIENT_ID,
client_secret=CLIENT_SECRET,
redirect_uri=REDIRECT_URI,
mode='simulated'
)
```
```java Java
AuthClient authClient = new AuthClient.Builder()
.clientId(CLIENT_ID)
.clientSecret(CLIENT_SECRET)
.redirectUri(REDIRECT_URI)
.mode("simulated")
.build();
```
```ruby Ruby
client = Smartcar::AuthClient.new({
client_id: CLIENT_ID,
client_secret: CLIENT_SECRET,
redirect_uri: REDIRECT_URI,
mode: 'simulated'
})
```
### 2. Log into a simulated connected services account
User your simulated vehicle credentials to log in, or select any vehicle brand and log in with any email address `(anything@anything.com)` and any password.
### 3. Send an API request to the simulated vehicle
Our API will return simulated data for each endpoint the simulated vehicle is able to provide, or randomized data for each other endpoint.
For example, a simulated Tesla will return battery capacity consistently, but will return randomized data if fuel level is requested.
See our API reference to learn more about the randomized data you should expect. If you use Single Select, use the below VIN and make a request as follows.
| Email | VIN |
| --------------------------------- | ----------------- |
| `smartcar@successful-request.com` | 0SCAUDI037A5ADB1C |
```node Node
const scope = ["read_vehicle_info"]
const options = singleSelect: {vin: '0SCAUDI037A5ADB1C'}
authUrl = client.getAuthUrl(scope, options);
```
```python Python
scope = ['read_vehicle_info']
options = { single_select={'vin': '0SCAUDI037A5ADB1C'} }
auth_url = client.get_auth_url(scope, options)
```
```java Java
String authUrl = authClient.authUrlBuilder()
.singleSelect(true)
.singleSelectVin("0SCAUDI037A5ADB1C")
.build();
```
```ruby Ruby
scope = %w(read_vehicle_info)
options = { "singleSelect": { vin: "0SCAUDI037A5ADB1C" } }
auth_url = client.get_auth_url(scope, options)
```
## Test successful API requests with specific VINs
If you need a test vehicle with a specific VIN, you can still use simulated mode. When logging into a test mode connected services account (step 2 above), follow this format for the email address:
`@.smartcar.com`
**VIN**: This can be any 17-character alphanumeric string. Note: This string does not need to be a real VIN. Smartcar does not perform any VIN-specific validations.
**Compatibility level**: This string determines which permissions the test mode vehicle is capable of. The following table contains all valid compatibility levels and their corresponding permissions:
| Compatibility level | Permissions |
| ------------------- | ---------------------------------------------------- |
| compatible OR phev | All permissions |
| incompatible | No permissions |
| fuel | Permissions that apply to a gasoline vehicle |
| bev | Permissions that apply to a battery-electric vehicle |
To use Single Select in test mode with a specific VIN, follow step 3 above and replace the Smartcar-provided VIN with the VIN of your choice. Then follow the email format `@.smartcar.com` using the same VIN.
## Test with multiple vehicles
In some cases, you may want to test the Connect flow with an account that has more vehicles than our default test accounts to help ensure your application is paginating and fetching data for a large number of vehicles correctly. You can do this with the following email format in Test mode and any password:
`-vehicles@smartcar.com`
Number: Any number from 1-999. Smartcar will show this many vehicles on the permission grant screen and return this many vehicles from the /vehicles endpoint, with pagination.
# Android Tutorial
Source: https://smartcar.com/docs/getting-started/tutorials/android
In this tutorial, we will use the Android SDK to integrate Connect into your application.
Our frontend SDKs handle getting an authorization code representing a vehicle owner's consent for your application to interact with their vehicle
for the requested permissions. In order to make requests to a vehicle, please use one of our [backend SDKs](/api-reference/api-sdks).
For security, token exchanges and requests to vehicles **should not** be made client side.
# Overview
1. The Mobile Application launches a `Chrome Custom Tab` with Smartcar Connect to request access to a user’s vehicle.
On Connect, the user logs in with their vehicle credentials and grants the Application access to their vehicle.
2. The `Chrome Tab` is redirected to a specified `REDIRECT_URI` along with an authorization `code`.
This will be the custom scheme set on the application. The Smartcar Android receives the authorization `code` in a view listening
for the specified custom scheme URI, and passes it to the Mobile Application.
3. The Mobile Application sends the received authorization `code` to the Application’s backend service.
4. The Application sends a request to the Smartcar API. This request contains the authorization code along with the Application’s
`CLIENT_ID` and `CLIENT_SECRET`.
5. In response, Smartcar returns an `ACCESS_TOKEN` and a `REFRESH_TOKEN`.
6. Using the `ACCESS_TOKEN`, the Application can now send requests to the Smartcar API. It can access protected resources and send commands
to and from the user’s vehicle via the backend service.
# Prerequisites
* [Sign up](https://dashboard.smartcar.com/signup) for a Smartcar account.
* Make a note of your `CLIENT_ID` and `CLIENT_SECRET` from the **Configuration** section on the Dashboard.
* Add a custom scheme redirect URI to your application configuration.
* Add the `app_server` redirect URI from [Setup step 2.](/tutorials/android#setup) to your application configuration.
For Android, we require the custom URI scheme to be in the format of `sc` + `clientId` + `://` + `hostname`.
For now, you can just set it to `sc` + `clientId` + `://exchange`.
Please see our [Connect Docs](/connect/dashboard-config#redirect-uris) for more information.
# Setup
1. Clone our repo and install the required dependencies:
```bash
$git clone https://github.com/smartcar/getting-started-android-sdk.git
```
Do not “checkout from version control” with the Getting Started repo in Android Studio, as it will not open the proper module.
2. Open `getting-started-android-sdk/tutorial` in Android Studio as an existing project and build from existing sources.
Android Studio should automatically import the required dependencies and build gradle. We're setting `app_server` to `http://10.0.2.2:8000`
to pass the authorization `code` from the [Handle the Response](/tutorials/android#handle-the-response) step later on in the tutorial to our backend.
```xml strings.xml
sc[yourClientId][yourClientId]http://10.0.2.2:8000
```
# Build your Connect URL
1. Instantiate a `smartcarAuth` object in the `onCreate` function of the `MainActivity`.
```java MainActivity.java
// TODO: Authorization Step 1a: Initialize the Smartcar object
private static String CLIENT_ID;
private static String REDIRECT_URI;
private static String[] SCOPE;
private SmartcarAuth smartcarAuth;
protected void onCreate(Bundle savedInstanceState) {
...
// TODO: Authorization Step 1b: Initialize the Smartcar object
CLIENT_ID = getString(R.string.client_id);
REDIRECT_URI = getString(R.string.smartcar_auth_scheme) + "://" + getString(R.string.smartcar_auth_host);
SCOPE = new String[]{"required:read_vehicle_info"};
smartcarAuth = new SmartcarAuth(
CLIENT_ID,
REDIRECT_URI,
SCOPE,
true,
new SmartcarCallback() {
// TODO: Authorization Step 3b: Receive an authorization code
}
);
}
```
The Android SDK does not support `simulated` mode at this time - only `test` and `live`.
Feel free to set `testMode` to `false` where you instantiate your `SmartcarAuth` object to
connect to a real vehicle.
2. The Android application will launch a Chrome Tab with Smartcar Connect to request access to a user’s vehicle.
On Connect, the user logs in with the username and password for their vehicle’s connected services account and grants the application access to their vehicle.
To launch Connect, we can use the `addClickHandler` function that our `smartcarAuth` object has access to.
```java MainActivity.java
// TODO: Authorization Step 2: Launch Connect
smartcarAuth.addClickHandler(appContext, connectButton);
```
# Registering your Custom Scheme
Once a user has authorized the application to access their vehicle, the user is redirected to the `REDIRECT_URI` with an authorization `code` as a query parameter.
Android applications use custom URI schemes to intercept calls and launch the relevant application. This is defined within the `AndroidManifest`.
```xml AndroidManifest.xml
```
# Handle the response
Using the Android SDK, the application can receive the code in the `SmartcarCallback` object passed into the `SmartcarAuth` object.
```java MainActivity.java
smartcarAuth = new SmartcarAuth(
CLIENT_ID,
REDIRECT_URI,
SCOPE,
true,
new SmartcarCallback() {
// TODO: Authorization Step 3b: Receive an authorization code
@Override
public void handleResponse(final SmartcarResponse smartcarResponse) {
Log.i("MainActivity", smartcarResponse.getCode());
// TODO: Request Step 1: Obtain an access token
//TODO: Request Step 2: Get vehicle information
}
}
);
```
# Launching Connect
Build your application in Android Studio and click on the **Connect your vehicle** button.
This tutorial configures Connect to launch in `test` mode by default.
In `test` mode, any `username` and `password` is valid for each brand.
Smartcar showcases all the permissions your application is asking for - `read_vehicle_info` in this case.
Once you have logged in and accepted the permissions, you should see your authorization `code` printed to your console.
# Getting your first access token
After receiving the authorization `code`, your iOS application must exchange it for an `ACCESS_TOKEN`. To do so, we can send
the code to a backend service. Let’s assume our backend service contains an endpoint `/exchange` that receives an authorization `code` as a query parameter and exchanges it for an `ACCESS_TOKEN`.
```swift ViewController.swift
// TODO: Obtain an access token
public void handleResponse(final SmartcarResponse smartcarResponse) {
Log.i("MainActivity", smartcarResponse.getCode());
final OkHttpClient client = new OkHttpClient();
// TODO: Request Step 1: Obtain and access token
// Request can not run on the Main Thread
// Main Thread is used for UI and therefore can not be blocked
new Thread(new Runnable() {
@Override
public void run() {
// send request to exchange the auth code for the access token
Request exchangeRequest = new Request.Builder()
.url(getString(R.string.app_server) + "/exchange?code=" + smartcarResponse.getCode())
.build();
try {
client.newCall(exchangeRequest).execute();
} catch (IOException e) {
e.printStackTrace();
}
}
}).start();
}
```
Notice that our backend service **does not** return the `ACCESS_TOKEN`.
This is by design. For security, our frontend should never have access
to the `ACCESS_TOKEN` and should always be stored in the backend.
# Getting data from a vehicle
Once the backend has the `ACCESS_TOKEN`, it can send requests to a vehicle using the Smartcar API. The Android app will
have to send a request to the backend service which in turn sends a request to Smartcar. We have to do this because
our frontend **does not** have the `ACCESS_TOKEN`.
Assuming our backend has a `/vehicle` endpoint that returns the information of a user’s vehicle, we can make this query in
our `completion callback` and start another `activity` to show the returned vehicle attributes.
```java MainActivity.java
public void handleResponse(final SmartcarResponse smartcarResponse) {
...
// TODO: Request Step 2: Get vehicle information
// send request to retrieve the vehicle info
Request infoRequest = new Request.Builder()
.url(getString(R.string.app_server) + "/vehicle")
.build();
try {
Response response = client.newCall(infoRequest).execute();
String jsonBody = response.body().string();
JSONObject JObject = new JSONObject(jsonBody);
String make = JObject.getString("make");
String model = JObject.getString("model");
String year = JObject.getString("year");
Intent intent = new Intent(appContext, DisplayInfoActivity.class);
intent.putExtra("INFO", make + " " + model + " " + year);
startActivity(intent);
} catch (IOException e) {
e.printStackTrace();
} catch (JSONException e) {
e.printStackTrace();
}
}
```
# Setting up your backend
Now that our frontend is complete, we will need to create a backend service that contains the logic for the `/exchange` and `/vehicle` endpoints.
You can use any of our backend SDKs below to set up the service starting from the **Obtaining an Access Token** step.
When setting up the environment variables for your backend SDK, make sure to set `REDIRECT_URI` to the custom scheme
used for this tutorial i.e. `sc + "clientId" + ://exchange`.
# Backend SDK Tutorials
Source: https://smartcar.com/docs/getting-started/tutorials/backend
In this tutorial, we will go over how to integrate Connect into your application and make requests to a vehicle using our backend SDKs.
# Overview
1. The Application redirects the user to Smartcar Connect to request access to the user’s vehicle. In Connect,
the user logs in with their vehicle credentials and grants the Application access to their vehicle.
2. The user’s browser is redirected to a specified `REDIRECT_URI`. The Application Server, which is listening
at the `REDIRECT_URI`, will retrieve the authorization code from query parameters sent to the `REDIRECT_URI`.
3. The Application sends a request to the Smartcar API. This request contains the authorization `code` along with
the Application’s `CLIENT_ID` and `CLIENT_SECRET`.
4. In response, Smartcar returns an `ACCESS_TOKEN` and a `REFRESH_TOKEN`.
5. Using the `ACCESS_TOKEN`, the Application can now send requests to the Smartcar API. It can access protected resources and send commands
to and from the user’s vehicle via the backend service.
# Prerequisites
* [Sign up](https://dashboard.smartcar.com/signup) for a Smartcar account.
* Make a note of your `CLIENT_ID` and `CLIENT_SECRET` from the **Configuration** section on the Dashboard.
* Add the following `REDIRECT_URI` to your application configuration: `http://localhost:8000/exchange`
# Setup
1. Clone the repo for the SDK you want to use and install the required dependencies:
```bash Node
$git clone https://github.com/smartcar/getting-started-express.git
$cd getting-started-express/tutorial
$npm install
```
```bash Python
$git clone https://github.com/smartcar/getting-started-python-sdk.git
$cd getting-started-python-sdk/tutorial
$pip install -r requirements.txt
```
```bash Java
$git clone https://github.com/smartcar/getting-started-java-sdk.git
$cd getting-started-java-sdk/tutorial
$gradlew build
```
```bash Ruby
$git clone https://github.com/smartcar/getting-started-ruby-sdk.git
$cd getting-started-ruby-sdk/tutorial
$bundle install
$ruby app.rb
```
2. You will also have to set the following environment variables
If you've used one of our frontend SDKs to integrate connect, you'll want to set the
`SMARTCAR_REDIRECT_URI` environment variable to the URI you used for that application.
```bash
$export SMARTCAR_CLIENT_ID=
$export SMARTCAR_CLIENT_SECRET=
$export SMARTCAR_REDIRECT_URI=http://localhost:8000/exchange
```
If you are using Windows, ensure you are appropriately setting environment variables for your shell.
Please refer to [this post](https://stackoverflow.com/questions/9249830/how-can-i-set-node-env-production-on-windows/9250168#9250168) which details how to set environment variables on Windows.
# Build your Connect URL
1. Instantiate a `Smartcar` object in the constructor of the App component.
```js index.js
const client = new smartcar.AuthClient({
mode: 'simulated',
});
```
```python main.py
client = smartcar.AuthClient(mode='simulated')
```
```java Main.java
String mode = "simulated";
AuthClient client = new AuthClient.Builder()
.mode(mode)
.build();
```
```ruby index.rb
@@client = Smartcar::AuthClient.new({
mode: 'simulated',
})
```
Feel free to set `mode` to `simulated` or `live` where you instantiate your `Smartcar` object to
connect to a simulated or real vehicle.
2. A Server-side rendered application will redirect to Smartcar Connect to request access to a user’s vehicle.
On Connect, the user logs in with the username and password for their vehicle’s connected services account
and grants the application access to their vehicle.
To launch Connect, we need to redirect the user to the appropriate URL. We can make use of the `AUTHORIZATION_URL`
function in our Smartcar object and redirect the user to the URL to launch the Connect flow.
```js index.js
app.get('/login', function(req, res) {
const scope = ['read_vehicle_info'];
const authUrl = client.getAuthUrl(scope);
res.render('home', {
url: authUrl,
});
});
```
```python main.py
@app.route('/login', methods=['GET'])
def login():
scope = ['read_vehicle_info']
auth_url = client.get_auth_url(scope)
return redirect(auth_url)
```
```java Main.java
get("/login", (req, res) -> {
// TODO: Authorization Step 2: Launch Smartcar authentication dialog
String[] scope = {"read_odometer"};
String link = client.authUrlBuilder(scope).build();
res.redirect(link);
return null;
});
```
```ruby index.rb
get "/login" do
redirect @@client.get_auth_url(['required:read_vehicle_info'])
end
```
# Handle the response
Once a user has authorized the application to access their vehicle, the user is redirected to the `REDIRECT_URI`
with an authorization `code` as a query parameter. In the previous section, we had set our `REDIRECT_URI` as `localhost:8000/exchange`.
Now, our server can be set up as follows to receive the authorization `code`.
```js index.js
app.get('/exchange', function(req, res) {
const code = req.query.code;
console.log(code);
res.sendStatus(200);
});
```
```python main.py
@app.route('/exchange', methods=['GET'])
def exchange():
code = request.args.get('code')
print(code)
return '', 200
```
```java Main.java
get("/exchange", (req, res) -> {
String code = req.queryMap("code").value();
System.out.println(code);
return "";
});
```
```ruby index.rb
get "/exchange" do
code = params[:code]
puts code
"OK"
end
```
# Launching Connect
Let’s try authenticating a vehicle! Restart your server, open up your browser and go to `http://localhost:8000/login`
```bash Node
$node index.js
```
```bash Python
$python main.py
```
```bash Java
$./gradlew run
```
```bash Ruby
$bundle exec ruby app.rb
```
This tutorial configures Connect to launch in `test` mode by default.
In `test` mode, any `username` and `password` is valid for each brand.
Smartcar showcases all the permissions your application is asking for - `read_vehicle_info` in this case.
Once you have logged in and accepted the permissions, you should see your authorization `code` printed to your console.
# Getting your first access token
If you've used one of our frontend SDKs to integrate Connect, this is where you'll need to fetch your access token.
In the previous section, we retrieved an authorization `code`. The application must exchange the code for an `ACCESS_TOKEN` to make a request.
After receiving the ACCESS\_TOKEN, the user can be redirected to the `/vehicle` route.
```js index.js
// Global variable to save our access_token
let access;
app.get('/exchange', async function(req, res) {
const code = req.query.code;
// Access our global variable and store our access tokens.
// In a production app you'll want to store this in some
// kind of persistent storage
access = await client.exchangeCode(code);
res.redirect('/vehicle');
});
```
```python main.py
# Global variable to save our access_token
access = None
@app.route('/exchange', methods=['GET'])
def exchange():
code = request.args.get('code')
# Access our global variable and store our access tokens.
# In a production app you'll want to store this in some
# kind of persistent storage
global access
access = client.exchange_code(code)
return redirect('/vehicle')
```
```java Main.java
// Global variable to save our access_token
private static String access;
public static void main(String[] args) {
get("/exchange", (req, res) -> {
String code = req.queryMap("code").value();
Auth auth = client.exchangeCode(code);
// Access our global variable and store our access tokens.
// In a production app you'll want to store this in some
// kind of persistent storage
access = auth.getAccessToken();
res.redirect("/vehicle");
return null;
})
}
```
```ruby index.rb
# Global variable to store the token
@@token = ''
get "/exchange" do
code = params[:code]
# Access our global variable and store our access tokens.
# In a production app you'll want to store this in
# some kind of persistent storage
@@token = @@client.exchange_code(code)[:access_token]
redirect '/vehicle'
end
```
# Getting data from a vehicle
1. Once the backend service or server-side application has the `ACCESS_TOKEN`, it can send requests to a vehicle using the Smartcar API.
First we'll need to fetch the `vehicle_id`s associated with the `ACCESS_TOKEN`, then fetch vehicle attributes for one of them. After
receiving the `vehicle_attributes` object, we can render it in a simple table on the page.
```js index.js
app.get('/vehicle', async function(req, res) {
// Get the smartcar vehicleIds associated with the access_token
const { vehicles } = await smartcar.getVehicles(access.accessToken);
// Instantiate the first vehicle in the vehicle id list
const vehicle = new smartcar.Vehicle(vehicles[0], access.accessToken);
// Make a request to Smartcar API
const attributes = await vehicle.attributes();
res.render('vehicle', {
info: attributes,
});
});
```
```python main.py
@app.route('/vehicle', methods=['GET'])
def get_vehicle():
# Access our global variable to retrieve our access tokens
global access
# Get the smartcar vehicleIds associated with the access_token
vehicles = smartcar.get_vehicles(access.access_token)
vehicle_ids = vehicles.vehicles
# Instantiate the first vehicle in the vehicle id list
vehicle = smartcar.Vehicle(vehicle_ids[0], access.access_token)
# Make a request to Smartcar API
attributes = vehicle.attributes()
return jsonify({
"make": attributes.make,
"model": attributes.model,
"year": attributes.year
})
'''
{
"make": "TESLA",
"model": "Model S",
"year": 2014
}
'''
```
```java Main.java
get("/vehicle", (req, res) -> {
// Get the smartcar vehicleIds associated with the access_token
VehicleIds vehiclesResponse = Smartcar.getVehicles(access);
String[] vehicleIds = vehiclesResponse.getVehicleIds();
// Instantiate the first vehicle in the vehicle id list
Vehicle vehicle = new Vehicle(vehicleIds[0], access);
// Make a request to Smartcar API
VehicleAttributes attributes = vehicle.attributes();
System.out.println(gson.toJson(attributes));
// {
// "id": "36ab27d0-fd9d-4455-823a-ce30af709ffc",
// "make": "TESLA",
// "model": "Model S",
// "year": 2014
// }
res.type("application/json");
return gson.toJson(attributes);
})
```
```ruby index.rb
get "/vehicle" do
code = params[:code]
# Get the smartcar vehicleIds associated with the access_token
vehicles = Smartcar::Vehicle.get_vehicle(token: @@token)
vehicle_ids = vehicles.vehicles
# Instantiate the first vehicle in the vehicle id list
vehicle = Smartcar::Vehicle.new(token: @@token, id: vehicle_ids.first)
# Get the vehicle_attributes object for vehicle
vehicle_attributes = vehicle.attributes
vehicle_attributes.slice(*%I(id make model year)).to_json
end
```
2. Restart your sever and head back to `http://localhost:8000/login` to go through Connect and make your first API request!
```bash Node
$node index.js
```
```bash Python
$python main.py
```
```bash Java
$./gradlew run
```
```bash Ruby
$bundle exec ruby app.rb
```
# iOS Tutorial
Source: https://smartcar.com/docs/getting-started/tutorials/ios
In this tutorial, we will use the iOS SDK to integrate Connect into your application.
Our frontend SDKs handle getting an authorization code representing a vehicle owner's consent for your application to interact with their vehicle
for the requested permissions. In order to make requests to a vehicle, please use one of our [backend SDKs](/api-reference/api-sdks).
For security, token exchanges and requests to vehicles **should not** be made client side.
# Overview
1. The Mobile Application launches a `SafariView` with Smartcar Connect to request access to a user’s vehicle.
On Connect, the user logs in with their vehicle credentials and grants the Application access to their vehicle.
2. The `SafariView` is redirected to a specified `REDIRECT_URI` along with an authorization `code`.
This will be the custom scheme set on the application. The Smartcar iOS SDK receives the authorization `code` in a view listening
for the specified custom scheme URI, and passes it to the Mobile Application.
3. The Mobile Application sends the received authorization `code` to the Application’s back-end service.
4. The Application sends a request to the Smartcar API. This request contains the authorization code along with the Application’s
`CLIENT_ID` and `CLIENT_SECRET`.
5. In response, Smartcar returns an `ACCESS_TOKEN` and a `REFRESH_TOKEN`.
6. Using the `ACCESS_TOKEN`, the Application can now send requests to the Smartcar API. It can access protected resources and send commands
to and from the user’s vehicle via the backend service.
# Prerequisites
* [Sign up](https://dashboard.smartcar.com/signup) for a Smartcar account.
* Make a note of your `CLIENT_ID` and `CLIENT_SECRET` from the **Configuration** section on the Dashboard.
* Add a custom scheme redirect URI to your application configuration.
* Add the `appServer` redirect URI from [Setup step 2](/tutorials/ios#setup) to your application configuration.
For iOS, we require the custom URI scheme to be in the format of `sc` + `clientId` + `://` + `hostname`.
For now, you can just set it to `sc` + `clientId` + `://exchange`.
Please see our [Connect Docs](connect/dashboard-config#redirect-uris) for more information.
# Setup
1. Clone our repo and install the required dependencies:
```bash
$git clone https://github.com/smartcar/getting-started-ios-sdk.git
$cd getting-started-ios-sdk/tutorial
$pod install
$open getting-started-ios-sdk.xcworkspace
```
2. Set the following constants in `Constants.swift`. We're setting `appServer` to `http://localhost:8000` to pass the authorization `code` from
the [Handle the Response](/tutorials/ios#handle-the-response) step later on in the tutorial to our backend.
```swift Constants.swift
struct Constants {
static let clientId = "";
static let appServer = "http://localhost:8000";
}
```
# Build your Connect URL
1. Instantiate a `SmartcarAuth` object in the `viewdidLoad` function of the `ViewController`.
{/* Smartcar TODO: testMode: true --> mode: "test" and remove the Info box when the SDK gets updated!!! - Travis J.*/}
```swift ViewController.swift
{/* TODO: Authorization Step 1: Initialize the SmartcarAuth object */}
appDelegate.smartcar = SmartcarAuth(
clientId: Constants.clientId,
redirectUri: "sc\(Constants.clientId)://exchange",
testMode: true,
scope: ["required:read_vehicle_info"],
completion: completion
)
```
The iOS SDK does not support `simulated` mode at this time - only `test` and `live`.
Feel free to set `testMode: false` where you instantiate your `SmartcarAuth` object to
connect to a real vehicle.
2. The iOS application will launch a `SafariView` with Connect to request access to a user’s vehicle.
On Connect, the user logs in with the username and password for their vehicle’s connected services account
and grants the application access to their vehicle.
To launch Connect, we can use the `launchAuthFlow` function that our `SmartcarAuth` object has access to. We can place
this within the `connectPressed` action function.
```swift ViewController.swift
@IBAction func connectPressed(_ sender: UIButton) {
{/* TODO: Authorization Step 2: Launch Connect */}
let smartcar = appDelegate.smartcar!
smartcar.launchAuthFlow(viewController: self)
}
```
# Registering your Custom Scheme
Once a user has authorized the application to access their vehicle, the user is redirected to the `REDIRECT_URI` with an authorization code as a query parameter.
iOS applications use custom URI schemes to intercept calls and launch the relevant application. This is defined within the `Info.plist`.
1. Open up `Info.plist` and click on the grey arrow next to **URL types**.
2. Next, click on the grey arrow next to the `Item 0` dictionary and `URL Schemes` array.
3. Finally, set your `Item 0` string to the redirect URI you set up in the [Prerequisites](/tutorials/ios#prerequisites) section (i.e. 'sc' + clientId).
# Handle the response
1. The iOS application will now receive the request in the `application:(_:open:options:)` function within the AppDelegate.
```swift AppDelegate.swift
func application(_ application: UIApplication, open url: URL, options: [UIApplicationOpenURLOptionsKey : Any] = [:]) -> Bool {
{/* TODO: Authorization Step 3a: Receive the authorization code */}
window!.rootViewController?.presentedViewController?.dismiss(animated: true , completion: nil)
smartcar!.handleCallback(with: url)
return true
}
```
2. Using the iOS SDK, the application can receive the code in the `completion callback` passed into the `SmartcarAuth` object.
```swift AppDelegate.swift
func completion(err: Error?, code: String?, state: String?) -> Any {
{/* TODO: Authorization Step 3b: Receive the authorization code */}
print(code!);
{/* prints out the authorization code */}
}
```
# Launching Connect
Build your application in XCode and click on the **Connect your vehicle** button.
This tutorial configures Connect to launch in `test` mode by default.
In `test` mode, any `username` and `password` is valid for each brand.
Smartcar showcases all the permissions your application is asking for - `read_vehicle_info` in this case.
Once you have logged in and accepted the permissions, you should see your authorization `code` printed to your console.
# Getting your first Access Token
After receiving the authorization `code`, your iOS application must exchange it for an `ACCESS_TOKEN`. To do so, we can send
the code to a backend service. Let’s assume our backend service contains an endpoint `/exchange` that receives an authorization `code` as a query parameter and exchanges it for an `ACCESS_TOKEN`.
```swift ViewController.swift
// TODO: Obtain an access token
Alamofire.request("\(Constants.appServer)/exchange?code=\(code!)", method: .get)
.responseJSON {_ in}
```
Notice that our backend service **does not** return the `ACCESS_TOKEN`.
This is by design. For security, our frontend should never have access
to the `ACCESS_TOKEN` and should always be stored in the backend.
# Getting data from a vehicle
Once the backend has the `ACCESS_TOKEN`, it can send requests to a vehicle using the Smartcar API. The iOS app will
have to send a request to the backend service which in turn sends a request to Smartcar. We have to do this because
our frontend **does not** have the `ACCESS_TOKEN`.
Assuming our backend has a `/vehicle` endpoint that returns the information of a user’s vehicle, we can make this query in
our `completion callback` and segue into another `view` to show the returned vehicle attributes
```swift ViewController.swift
func completion(err: Error?, code: String?, state: String?) -> Any {
// send request to exchange auth code for access token
Alamofire.request("\(Constants.appServer)/exchange?code=\(code!)", method: .get).responseJSON {_ in
// TODO: Request Step 2: Get vehicle information
// send request to retrieve the vehicle info
Alamofire.request("\(Constants.appServer)/vehicle", method: .get).responseJSON { response in
print(response.result.value!)
if let result = response.result.value {
let JSON = result as! NSDictionary
let make = JSON.object(forKey: "make")! as! String
let model = JSON.object(forKey: "model")! as! String
let year = String(JSON.object(forKey: "year")! as! Int)
let vehicle = "\(year) \(make) \(model)"
self.vehicleText = vehicle
self.performSegue(withIdentifier: "displayVehicleInfo", sender: self)
}
}
}
return ""
}
```
# Setting up your backend
Now that our frontend is complete, we will need to create a backend service that contains the logic for the `/exchange` and `/vehicle` endpoints.
You can use any of our backend SDKs below to set up the service starting from the **Obtaining an Access Token** step.
When setting up the environment variables for your backend SDK, make sure to set `REDIRECT_URI` to the custom scheme
used for this tutorial i.e. `sc + "clientId" + ://exchange`.
# React Tutorial
Source: https://smartcar.com/docs/getting-started/tutorials/react
In this tutorial, we will use the JavaScript SDK to integrate Connect into your application.
Our frontend SDKs handle getting an authorization code representing a vehicle owner's consent for your application to interact with their vehicle
for the requested permissions. In order to make requests to a vehicle, please use one of our [backend SDKs](/api-reference/api-sdks).
For security, token exchanges and requests to vehicles **should not** be made client side.
# Overview
1. The Single-Page Application launches a pop-up window with Smartcar Connect to request access to a user’s vehicle.
It does so by using the Smartcar JavaScript SDK. On Connect, the user logs in with the username and password for
their vehicle’s connected services account and grants the Application access to their vehicle.
2. The user’s browser is redirected to a specified Smartcar-hosted `REDIRECT_URI` - the Smartcar JavaScript SDK redirect page.
3. The Smartcar JavaScript SDK redirect page will receive an authorization `code` as a query parameter. The redirect page will then
send the code to the Single-Page Application’s `onComplete` callback using the `postMessage` web API. **This step is handled entirely
by the JavaScript SDK.**
4. The Single-Page Application sends the received authorization `code` to the Application’s backend service.
5. The Application sends a request to the Smartcar API. This request contains the authorization code along with the Application’s
`CLIENT_ID` and `CLIENT_SECRET`.
6. In response, Smartcar returns an `ACCESS_TOKEN` and a `REFRESH_TOKEN`.
7. Using the `ACCESS_TOKEN`, the Application can now send requests to the Smartcar API. It can access protected resources and send commands
to and from the user’s vehicle via the backend service.
# Prerequisites
* [Sign up](https://dashboard.smartcar.com/signup) for a Smartcar account.
* Make a note of your `CLIENT_ID` and `CLIENT_SECRET` from the **Configuration** section on the Dashboard.
* Add the special JavaScript SDK redirect URI to your application configuration.
* Add the `REACT_APP_SERVER` redirect URI from [Setup step 2.](/tutorials/react#setup) to your application configuration.
To use the JavaScript SDK, we require the special redirect URI to provide a simpler flow to retrieve authorization codes.
For this tutorial you can use the following:
`https://javascript-sdk.smartcar.com/v2/redirect?app_origin=http://localhost:3000`
# Setup
1. Clone our repo and install the required dependencies:
```bash
$git clone https://github.com/smartcar/getting-started-javascript-sdk-react.git
$cd getting-started-javascript-sdk-react/tutorial
$npm install
```
2. Set the following environment variables. We will be setting up a server later for our React front-end to communicate with.
For now, let’s assume our server is listening on `http://localhost:8000`.
```bash
$export REACT_APP_CLIENT_ID=
$export REACT_APP_REDIRECT_URI= https://javascript-sdk.smartcar.com/v2/redirect?app_origin=http://localhost:3000
$export REACT_APP_SERVER=http://localhost:8000
```
Note: If you are using Windows, ensure you are appropriately setting environment variables for your shell.
Please refer to this post which details how to set environment variables on Windows.
# Build your Connect URL
1. Instantiate a `Smartcar` object in the `constructor` of the App component.
```js App.jsx
constructor(props) {
...
// TODO: Authorization Step 1: Initialize the Smartcar object
this.smartcar = new Smartcar({
clientId: process.env.REACT_APP_CLIENT_ID,
redirectUri: process.env.REACT_APP_REDIRECT_URI,
scope: ['required:read_vehicle_info'],
mode: 'test',
onComplete: this.onComplete,
});
}
```
2. A single-page application will launch a pop-up window with Smartcar Connect to request access to a user’s vehicle.
On Connect, the user logs in with the username and password for their vehicle’s connected services account and grants
the application access to their vehicle.
To launch Connect, we can use the `openDialog` function that our `Smartcar` object has access to. We can place this is
an `onClick` handler of an HTML button.
```js App.jsx
authorize() {
// TODO: Authorization Step 2a: Launch Connect
this.smartcar.openDialog({forcePrompt: true});
}
render() {
// TODO: Authorization Step 2b: Render the Connect component
return ;
}
```
# Handle the response
1. Once a user has authorized the application to access their vehicle, the user is redirected to the `redirect_uri` with an
authorization code as a query parameter and the pop-up dialog is closed. Using the JavaScript SDK, the frontend can
receive this `code` in the `onComplete` callback passed into the `Smartcar` object.
```js App.jsx
onComplete(err, code, status) {
//TODO: Authorization Step 3: Receive the authorization code
console.log(code);
//prints out the authorization code
};
```
# Launching Connect
Restart your server, open up your browser and go to [http://localhost:3000/login](http://localhost:3000/login). You should be reciderect to Smartcar Connect.
This tutorial configures Connect to launch in `test` mode by default.
In `test` mode, any `username` and `password` is valid for each brand.
Smartcar showcases all the permissions your application is asking for - `read_vehicle_info` in this case.
Once you have logged in and accepted the permissions, you should see your authorization `code` printed to your console.
# Getting your first access token
After receiving the authorization code, the React application must exchange it for an `ACCESS_TOKEN`. To do so, we can send
the code to the backend service which we will implement in a bit. Until then, let’s assume our backend service contains
an endpoint `/exchange` that receives an authorization code as a query parameter and exchanges it for an `ACCESS_TOKEN`.
We can add this logic in our `onComplete` callback
```js App.jsx
onComplete(err, code, status) {
// TODO: Request Step 1: Obtain an access token
return axios
.get(`${process.env.REACT_APP_SERVER}/exchange?code=${code}`);
}
```
# Getting data from a vehicle
Once the back-end has the `ACCESS_TOKEN`, it can send requests to a vehicle using the Smartcar API. The React app will
have to send a request to the backend service which in turn sends a request to Smartcar. We have to do this because
our frontend does not have the `ACCESS_TOKEN`.
Assuming our backend has a `/vehicle` endpoint that returns the information of a user’s vehicle, we can make this query in
our `completion callback` and and set the state of the React app with the returned vehicle attributes.
```js App.jsx
onComplete(err, code, status) {
// TODO: Request Step 2a: Get vehicle information
return axios
.get(`${process.env.REACT_APP_SERVER}/exchange?code=${code}`)
.then(_ => {
return axios.get(`${process.env.REACT_APP_SERVER}/vehicle`);
})
.then(res => {
this.setState({vehicle: res.data});
});
}
```
Now that we have the vehicle attributes, we can render a simple Vehicle component that shows the vehicle attributes in a table.
```js App.jsx
render() {
// TODO: Request Step 2b: Get vehicle information
return Object.keys(this.state.vehicle).length !== 0 ? (
) : (
);
}
```
# Setting up your backend
Now that our frontend is complete, we will need to create a backend service that contains the logic for the `/exchange` and `/vehicle` endpoints.
You can use any of our backend SDKs below to set up the service starting from the **Obtaining an access token** step.
When setting up the environment variables for your backend SDK, make sure to set `REDIRECT_URI` to the custom scheme
used for this tutorial i.e. `https://javascript-sdk.smartcar.com/v2/redirect?app_origin=http://localhost:3000`.
# Diagnostic Webhooks
Source: https://smartcar.com/docs/getting-started/tutorials/webhooks-diagnostic
Diagnostic webhooks send events whenever a state change occurs within the vehicle's Health Status or a Diagnostic Trouble Code (DTC) is reported
## Beta
Diagnostic webhooks are available in beta throughout December 2024 - January 2025 for organizations with Enterprise plans with Smartcar.
## Brand Support
Diagnostics provide visibility into Diagnostic Trouble Codes (DTCs) for GM vehicles and System Status information for GM and FCA vehicles.
## Supported Events
[Diagnostic Trouble Codes (DTCs)](https://smartcar.com/docs/api-reference/get-dtcs), which are 5-digit codes that indicate the component and fault type. DTC webhooks will send events for each active DTC including a timestamp it became active. A webhook event will also trigger when a DTC is no longer active.
[System Status](https://smartcar.com/docs/api-reference/get-system-status), where an OEM application’s “Vehicle Health” or “System Health” reports provide a list of components and their health status. System Status webhooks will send events whenever a system reports a change from Healthy to Alert and vice-versa. A [list of diagnostic systems](https://smartcar.com/docs/help/diagnostic-systems) is available in our Help Center.
## Prerequisites
For this tutorial it is recommended to have the following in place:
* To receive webhooks you’ll need to set up a callback URI on your server.
* To [**subscribe**](https://smartcar.com/docs/api-reference/webhooks/subscribe-webhook) a vehicle you’ll want to have [**Connect**](https://smartcar.com/docs/connect/dashboard-config) integrated into your application.
## Setting up a Diagnostic Webhook
Navigate to **Webhooks** from the Smartcar Dashboard, select **+ Add webhook** and select **Event-based** from the Webhooks config wizard.
Name your webhook and specify a `callback URI`. Your `callback URI` is where Smartcar will send payloads from vehicles connected to your webhook. Click **Next**.
Select which event types you wish to receive from System Status or Diagnostic Trouble Codes and hit Add. To receive both event types, create a second webhook.
Hit **Add** when ready.
After adding your webhook, you’ll need to verify your callback URI. This is to ensure Smartcar is sending data to the correct place!
Please see our API reference on how to [verify a webhook](/api-reference/webhooks/callback-verification), or **Part II** on [this blog post](https://smartcar.com/blog/how-to-use-scheduled-webhooks#-part-ii-verify-webhook) for a more in depth guide.
## Subscribing vehicles to a webhook
Now you’ve got your webhook set up, you can subscribe vehicles to start getting data. If you haven’t done so already, please set up [Connect](http://localhost:3000/connect/dashboard-config) for your application.
After going through Connect and receiving your initial `access_token`, you’ll first want to hit the [All Vehicles](/api-reference/all-vehicles) endpoint to fetch the Smartcar `vehicle_ids` of the authorized vehicles.
With your `access_token` and `webhook_id` you can hit the [Subscribe](/api-reference/webhooks/subscribe-webhook) endpoint for each `vehicle_id` to start receiving data.
## Example Diagnostic Trouble Code Payload
```json Example Response
{
"version": "3.0",
"webhookId": "abde94ff-d57d-43b9-8d09-6020db2d977a",
"deliveryId": "aadc13aa-c838-41ba-b5c5-0c655ec2234a",
"deliveryTime": "2022-04-06T01:50:00.000Z",
"mode": "live",
"type": "EVENT_DELIVERY",
"data": {
"events": [
{
"eventId": "1713eab1-eebc-4b4e-9433-0f2a488851c2",
"vehicleId": "9af13248-3b73-4c9d-9a4b-d937ce6bc8e2",
"userId": "9a2630ca-9496-4927-b6dc-991f47e5ed14",
"eventTime": "2022-04-06T01:48:22.845Z",
"eventType": "DIAGNOSTIC_TROUBLE_CODES",
"codes": [
{
"code": "P302D",
"timestamp": "2024-09-05T14:48:00.000Z"
},
{
"code": "xxxxx",
"timestamp": null
},
// ...
]
}
]
}
}
```
## Response
The unique ID of the event delivered.
The ID for the vehicle.
The ID for the user impacted by the event.
The date and time the event was reported.
Indicates the event is a DTC.
the Diagnostic Trouble Code.
The date and time the DTC became active. ISO 8601, millisecond precision, UTC time zone.
## Example System Status Payload
```json Example Response
{
"version": "3.0",
"webhookId": "abde94ff-d57d-43b9-8d09-6020db2d977a",
"deliveryId": "aadc13aa-c838-41ba-b5c5-0c655ec2234a",
"deliveryTime": "2022-04-06T01:50:00.000Z",
"mode": "live",
"type": "EVENT_DELIVERY",
"data": {
"events": [
{
"eventId": "1713eab1-eebc-4b4e-9433-0f2a488851c2",
"vehicleId": "9af13248-3b73-4c9d-9a4b-d937ce6bc8e2",
"userId": "9a2630ca-9496-4927-b6dc-991f47e5ed14",
"eventTime": "2022-04-06T01:48:22.845Z",
"eventType": "DIAGNOSTIC_SYSTEM_ALERTS",
"alerts": [
{
"systemId": "SYSTEM_TPMS",
"status": "ALERT",
"description": "Left rear tire sensor battery low"
},
{
"systemId": "SYSTEM_AIRBAG",
"status": "ALERT",
"description": null
}
// ...
]
}
]
}
}
```
## Response
The unique ID of the event delivered.
The ID for the vehicle.
The ID for the user impacted by the event.
The date and time the event was reported.
Indicates the event is a System Alert
the system reporting alert or OK status. See [Smartcar Systems](https://smartcar.com/docs/help/diagnostic-systems).
Either "ALERT" or "OK".
A plaintext, readable description when available.
The date and time the DTC became active. ISO 8601, millisecond precision, UTC time zone.
## FAQs
Smartcar will handle regular polling for diagnostic events to provide them as quickly as possible.
Yes! You can distinguish between webhook types based on the `eventName` field. Dynamic Webhooks will have `eventName` set to `dynamic`.
Smartcar will attempt to resend payloads with an exponential backoff. Once your server is back online you'll continue to receive data from the vehicles.
* Once a webhook is configured and a vehicle is subscrbied, we’ll begin sending data to your Callback URI.
We’ll expect a 2xx response to each. In the event that we don’t receive a 2xx response, we’ll retry 6 times with exponential backoff.
**If we continue not to receive a successful response, we’ll automatically disable your webhook.**
* If you haven't made any major changes to your callback URI, commonly our request payload may have hit the request size limit for you server.
Smartcar will attempt to get data from the vehicle with retries. However if we are unable to we'll return the relevant error response in the payload for that vehicle.
For example, if a vehicle owner changes the credentials to their connected services account we'll return a [CONNECT\_SERVICES\_ACCOUNT:AUTHENTICATION\_FAILED](/errors/api-errors/connected-services-account-errors#authentication-failed) error prompting you to have the vehicle owner go through Connect again to reauthenticate their vehicle.
To unsubscribe a vehicle you can hit the [unsubscribe](/api-reference/webhooks/unsubscribe-webhook) endpoint or [disconnect](/api-reference/management/delete-vehicle-connections) the vehicle from your application.
# Dynamic Webhooks (Beta)
Source: https://smartcar.com/docs/getting-started/tutorials/webhooks-dynamic
Dynamic webhooks make it incredibly easy to receive data from a vehicle at the optimal frequency supported by the OEM. Specifically, once a certain condition is met, you will be able to receive data more frequently from the vehicle to stay up-to-date with the state of the vehicle when it matters most to you.
## Prerequisites
For this tutorial it is recommended to have the following in place:
* To receive webhooks you’ll need to set up a callback URI on your server.
* To [**subscribe**](https://smartcar.com/docs/api-reference/webhooks/subscribe-webhook) a vehicle you’ll want to have [**Connect**](https://smartcar.com/docs/connect/dashboard-config) integrated into your application.
## Setting up a Dynamic Webhook
Navigate to **Webhooks** from the Smartcar Dashboard, select **+ Add webhook** and select **Dynamic** from the Webhooks config wizard.
Name your webhook and specify a `callback URI`. Your `callback URI` is where Smartcar will send payloads from vehicles connected to your webhook. Click **Next**.
Confirm everything looks good and hit **Add**.
Currently Dynamic Webhooks only support returning data from the
[location](/api-reference/get-location),
[charge](/api-reference/evs/get-charge-status), and
[battery](/api-reference/evs/get-battery-level) endpoints. Changes in data frequency
are only supported based on the plugged in state for EVs.
After adding your webhook, you’ll need to verify your callback URI. This is to ensure Smartcar is sending data to the correct place!
Please see our API reference on how to [verify a webhook](/api-reference/webhooks/callback-verification), or **Part II** on [this blog post](https://smartcar.com/blog/how-to-use-scheduled-webhooks#-part-ii-verify-webhook) for a more in depth guide.
## Subscribing vehicles to a webhook
Now you’ve got your webhook set up, you can subscribe vehicles to start getting data. If you haven’t done so already, please set up [Connect](http://localhost:3000/connect/dashboard-config) for your application.
After going through Connect and receiving your initial `access_token`, you’ll first want to hit the [All Vehicles](/api-reference/all-vehicles) endpoint to fetch the Smartcar `vehicle_ids` of the authorized vehicles.
With your `access_token` and `webhook_id` you can hit the [Subscribe](/api-reference/webhooks/subscribe-webhook) endpoint for each `vehicle_id` to start receiving data.
## Example payload
```json dynamicWebhook.json
{
"version": "2.0",
"webhookId": "865ca68d-a9b6-4d74-856e-605db0209eca",
"eventName": "dynamic",
"mode": "live",
"payload": {
"vehicles": [
{
"vehicleId": "00000000-0000-4000-A000-000000000000",
"requestId": "25d743b8-14cf-4744-b067-bd8d7daf3f1a",
"data": [
{
"path": "/battery",
"code": 200,
"body": {
"range": 283.244544,
"percentRemaining": 0.58
},
"headers": {
"sc-data-age": "2024-09-20T21:58:06.000Z"
}
},
{
"path": "/charge",
"code": 200,
"body": {
"isPluggedIn": false,
"state": "NOT_CHARGING"
},
"headers": {
"sc-data-age": "2024-09-20T21:58:06.000Z"
}
},
{
"path": "/location",
"code": 200,
"body": {
"latitude": 37.4292,
"longitude": 122.1381,
},
"headers": {
"sc-data-age": "2024-09-20T21:58:48.000Z"
}
}
]
}
]
}
}
```
## FAQs
By default Smartcar will deliver data every hour from the time a vehicle is subscribed. From that point on, once we detect the relevant state changes we'll increase this to every 30 minutes, dropping back down to hourly once the state returns to what it was.
Where more frequent data delivery is supported by an OEM, Smartcar will deliver data at those speeds. Please reach out to your Solutions Architect for more information.
Yes! You can distinguish between webhook types based on the `eventName` field. Dynamic Webhooks will have `eventName` set to `dynamic`.
Smartcar will attempt to resend payloads with an exponential backoff. Once your server is back online you'll continue to receive data from the vehicles.
* Once a webhook is configured and a vehicle is subscrbied, we’ll begin sending data to your Callback URI.
We’ll expect a 2xx response to each. In the event that we don’t receive a 2xx response, we’ll retry 6 times with exponential backoff.
**If we continue not to receive a successful response, we’ll automatically disable your webhook.**
* If you haven't made any major changes to your callback URI, commonly our request payload may have hit the request size limit for you server.
Smartcar will attempt to get data from the vehicle with retries. However if we are unable to we'll return the relevant error response in the payload for that vehicle.
For example, if a vehicle owner changes the credentials to their connected services account we'll return a [CONNECT\_SERVICES\_ACCOUNT:AUTHENTICATION\_FAILED](/errors/api-errors/connected-services-account-errors#authentication-failed) error prompting you to have the vehicle owner go through Connect again to reauthenticate their vehicle.
To unsubscribe a vehicle you can hit the [unsubscribe](/api-reference/webhooks/unsubscribe-webhook) endpoint or [disconnect](/api-reference/management/delete-vehicle-connections) the vehicle from your application.
# Scheduled Webhooks
Source: https://smartcar.com/docs/getting-started/tutorials/webhooks-scheduled
Scheduled webhooks make it incredibly easy to receive vehicle data on a cadence of your choosing. You can set up a webhook for any Smartcar endpoint that allows you to read data from a vehicle.
This tutorial is split into four steps:
1. Creating your webhook
2. Verifying your callback URI
3. Confirmation with a test payload
4. Subscribing your first vehicle
# Prerequisites
For this tutorial it is recommended to have the following in place:
* To receive webhooks you'll need to set up a callback URI on your server.
* To [subscribe](/api-reference/webhooks/subscribe-webhook) a vehicle you'll want to have [Connect](/connect/dashboard-config) set up.
# Creating your first webhook
1. Navigate to the **Webhooks** section of Dashboard and hit **+ Add Webhook**.
2. Next, name your webhook, enter in your callback URI, and select **Scheduled** as the type.
3. After selecting the type, you'll need to:
* set how often you want receive data
* select which endpoints you want to receive data from
* select the units you want to receive data
4. Once you're happy with the configuration, hit **Add Webhook** and move on to verifying your callback URI.
# Verifying your callback URI
After adding your webhook, you'll be prompted to verify it. After hitting **Verify this webhook** Smartcar will post a challenge request
to your callback URI to ensure we're sending payloads to the correct place.
See the [callback verification](/api-reference/webhooks/callback-verification) section on our API Reference for insructions on how to format your response.
# Confirmation with a test payload
Once verified you can send a test payload to your callback URI. Payloads from vehicles will have `eventName` set to `scheduled`.
The `mode` field will reflect the type of vehicle you've subscribed - either `live`, `test` or `simulated`.
```json Test Request Body
{
"version": "2.0",
"webhookId": "6f9c7e43-2278-4a9b-b273-cdacab9ed83c",
"eventName": "scheduled",
"mode": "test",
"payload": {
"vehicles": [
{
"vehicleId": "1a9fdd59-b65e-4bf1-8264-5448812eac83",
"requestId": "6c22cd5c-34df-48ae-ad90-b324170629be",
"data": [
{
"path": "/",
"code": 200,
"body": {
"id": "1a9fdd59-b65e-4bf1-8264-5448812eac83",
"make": "BMW",
"model": "i3 94 (+ REX)",
"year": 2017
},
"headers": {}
},
{
"path": "/fuel",
"code": 200,
"body": {
"range": 44.12,
"percentRemaining": 0.57,
"amountRemaining": 1.32
},
"headers": {
"sc-data-age": "2020-05-11T23:44:31.000Z",
"sc-unit-system": "imperial"
}
},
{
"path": "/vin",
"code": 200,
"body": {
"vin": "WBY1Z8C54HV550878"
},
"headers": {}
}
],
"timestamp": "2020-05-13T22:00:01.288Z"
}
]
}
}
```
```json Test Request Headers
{
"sc-signature": "61e60e2e149ccc0c63680ca3a9df09e071ea450dffc1f0e844f962f9df019b44",
"content-type": "application/json",
"user-agent": "Smartcar-Webhooks/2.0",
}
```
# Subscribing your first vehicle
Now you've got your webhook set up, you can subscribe vehicles to start getting data at your desired frequency.
If you haven't done so already, please set up the [Connect](connect/dashboard-config) for your application.
After receiving your initial `ACCESS_TOKEN` from the [auth code exchange](connect/auth-code-exchange) step for Connect,
you'll first want to hit the [All Vehicles](/api-reference/management/all-vehicles) endpoint to fetch the Smartcar
`vehicle_id`s of the authorized vehicles.
With your `ACCESS_TOKEN` and `WEBHOOK_ID` you can hit the [Subscribe](/api-reference/webhooks/subscribe-webhook)
endpoint for each `vehicle_id` to start receiving data.
# FAQs
Data will post to the endpoint depending on your selected cadence. For example:
* if a car is subscribed to an hourly interval webhook at 15:45 UTC, you'll get start to get data after 16:00UTC.
* if a car is subscribed to a monthly interval, we'll process the webhooks at 00:00UTC for the selected day.
No, you'll need to set up a webhook for each webhook type.
Yes! You can distinguish between webhook types based on the `eventName` field - `scheduled` or `eventBased`.
* Once a webhook is configured, we’ll begin sending events to your Callback URI on the cadence that you’ve chosen.
We’ll expect a 2xx response to each. In the event that we don’t receive a 2xx response, we’ll retry 5 times over the next 10 minutes.
**If we continue not to receive a successful response, we’ll automatically disable your webhook.**
* Check that your server didn't experience down time for more than 10 minutes recently resulting in 2XX responses not being sent back to Smartcar.
* If you haven't made any major changes to your callback URI, commonly our request payload may have hit the request size limit for you server.
While we aim for 1 to 5 minutes after your selected cadence, the delivery time largely depends on the number of vehicles you have subscribed as
well as the average OEM latency. Smartcar will try to fulfill requests for your webhook within 15 minutes of the scheduled time. If we were unable
to fulfill a request during that time, we'll try again over the course of subsequent 15 minute intervals and post them to your webhook.
# Testing with Postman
Source: https://smartcar.com/docs/getting-started/using-vs-with-postman
In this guide we'll go over how to use Vehicle Simulator to test out Smartcar using Postman.
## What is Postman?
Postman is a collaboration platform for API development. Postman's features simplify each step of building an API and streamline collaboration so you can create better APIs - faster.
You’ll need a few things to start making API calls with Smartcar and Postman:
* [A Postman Account](https://identity.getpostman.com/signup?continue=https%3A%2F%2Fgo.postman.co%2Fbuild)
* [Smartcar’s Postman Collection](https://www.postman.com/smartcar/workspace/smartcar-api/collection/13172949-37efba9a-c68c-4f45-b123-af9154d7a0a6?action=share\&creator=9829117)
* [A Smartcar Developer Account](https://dashboard.smartcar.com/signup)
## Configuring the Connect flow in Postman
1. Install the latest [Postman app](https://www.postman.com/downloads/) and log in
2. Import the [Smartcar collection](https://www.postman.com/smartcar/workspace/smartcar-api/collection/13172949-37efba9a-c68c-4f45-b123-af9154d7a0a6?action=share\&creator=9829117) into your local Postman workspace. Hit Import.
3. Click Link, paste in [the link](https://www.postman.com/smartcar/workspace/smartcar-api/collection/13172949-37efba9a-c68c-4f45-b123-af9154d7a0a6?action=share\&creator=9829117) to the collection and hit Continue.
4. On the next screen, hit Import.
## Smartcar Application Configuration
If you haven’t already signed up for a Smartcar Developer account, you can do so from this [**signup link**](https://dashboard.smartcar.com/signup).
* Grab your **Client ID** and **Client Secret** from the Configuration page after you sign in, and copy them to someplace secure. You’ll need those tokens a little later in this setup.
* Under the Credentials tab for your application, you’ll need to add the following Redirect URIs. This will ensure that Smartcar Connect exits successfully with both Postman’s Native and Web clients.
```
https://oauth.pstmn.io/v1/browser-callback
http://localhost:8000/exchange
```
Through the Connect flow, you’re able to get consent from vehicle owners to connect their vehicle to your application. Postman provides an easy way to manage oAuth 2.0 flows under the Authorization tab of a Collection. Set up the Configuration Options for either a live or simulated vehicle.
**Live Vehicle**
| Parameter | Description |
| ---------------- | ----------------------------------------------------------------------------------------------------------------- |
| Auth URL | `https://connect.smartcar.com/oauth/authorize?approval_prompt=force` |
| Access Token URL | `https://auth.smartcar.com/oauth/token` |
| Client ID | `client_id` from your Dashboard |
| Client Secret | `client_secret` from your Dashboard |
| Scope | A space-separated list of [permissions](/api-reference/permissions) that your application is requesting access to |
**Simulated Vehicle**
| Parameter | Description |
| ---------------- | ----------------------------------------------------------------------------------------------------------------- |
| Auth URL | `https://connect.smartcar.com/oauth/authorize?approval_prompt=force&mode=simulated` |
| Access Token URL | `https://auth.smartcar.com/oauth/token` |
| Client ID | `client_id` from your Dashboard |
| Client Secret | `client_secret` from your Dashboard |
| Scope | A space-separated list of [permissions](/api-reference/permissions) that your application is requesting access to |
## Setting up a Simulated Vehicle
Under the Simulator tab on Dashboard, hit Add Vehicle. Note that you may already have a pre-created simulated vehicle available, if so skip to **Connecting to a vehicle** below.
Select a region and MMY for your vehicle, then hit Search by MMY
After selecting Use Vehicle, you’ll want to select a state for the vehicle. After that, you’ll get a set of credentials to use in the Connect flow.
Before proceeding to the next step, you’ll want to start the simulation in the Smartcar Dashboard.
## Connecting to a vehicle
If configured correctly, when you click **Get New Access Token** in Postman, you should see the first screen of the Connect flow.
Before hitting Continue, make sure to select a country from the dropdown appropriate to the region you selected for your simulated vehicle.
Continue through the Connect flow, select the brand of your simulated vehicle and enter in the credentials you were issued for your simulated vehicle.
After accepting the permissions you’ll be met with the following screen:
Hit Use Token. You should see Access Token under Current Token populate with the access\_token from the popup. Using this access\_token you can make API
requests to Smartcar's API.
Access tokens are only valid for two hours. If you want to make API requests after it has expired, you'll need to generate a
new one by stepping through the Connect Flow again, or through a [token refresh](/api-reference/authorization/token-refresh).
## Making an API request
Thanks to Postman, requests in the collection will inherit the `access_token` from the OAuth 2.0 Authorization flow we went through in the steps above.
In order to make API requests, we’ll need to first get a Smartcar `vehicle Id`. A `vehicle Id` is a unique identifier for a vehicle on Smartcar’s platform.
This can be done with the `All Vehicles` request. Making this request will assign the `vehicle Id` to the `{{vehicle_id}}` variable for other requests in the Collection.
Now that you've got a vehicle Id, you can make a request to other endpoints. Hitting Vehicle Info, we can see the MMY of the vehicle.
Checking `EV Battery Level`, we can see the response matches the vehicle state on the simulator
## Troubleshooting
* Check that you’re launching Connect in simulated mode
* If you’re still running in to an error make sure you’ve selected a country that matches the region you selected for your simulated vehicle.
* Check that the vehicle you’ve created supports the endpoint. Select View Estimated Latencies to see a list of endpoints supported for the vehicle.
* If you’re still running into the error, check that you’ve requested the appropriate permission in the scope parameter of the Connect URL.
You can always check the `/permissions` endpoint to see what endpoints you have access to.
# Accessing the Smartcar Support Center
Source: https://smartcar.com/docs/help/accessing-support-center
The Smartcar Support Center provides helpful resources including a knowledge base, ticket submission form, AI chat assistant, and a ticket center to manage your requests.
This feature is available on paid plans.
You can access the Support Center in two ways:
### Option 1: Visit the Support Center directly
Go to [support.smartcar.com](https://support.smartcar.com). You’ll be redirected to the Smartcar Dashboard to log in with your Dashboard credentials.
### Option 2: From the Smartcar Dashboard
1. Log in at [dashboard.smartcar.com](https://dashboard.smartcar.com).
2. Click the **Help** icon in the bottom-left corner, or the **Help Hub** icon in the bottom-right. 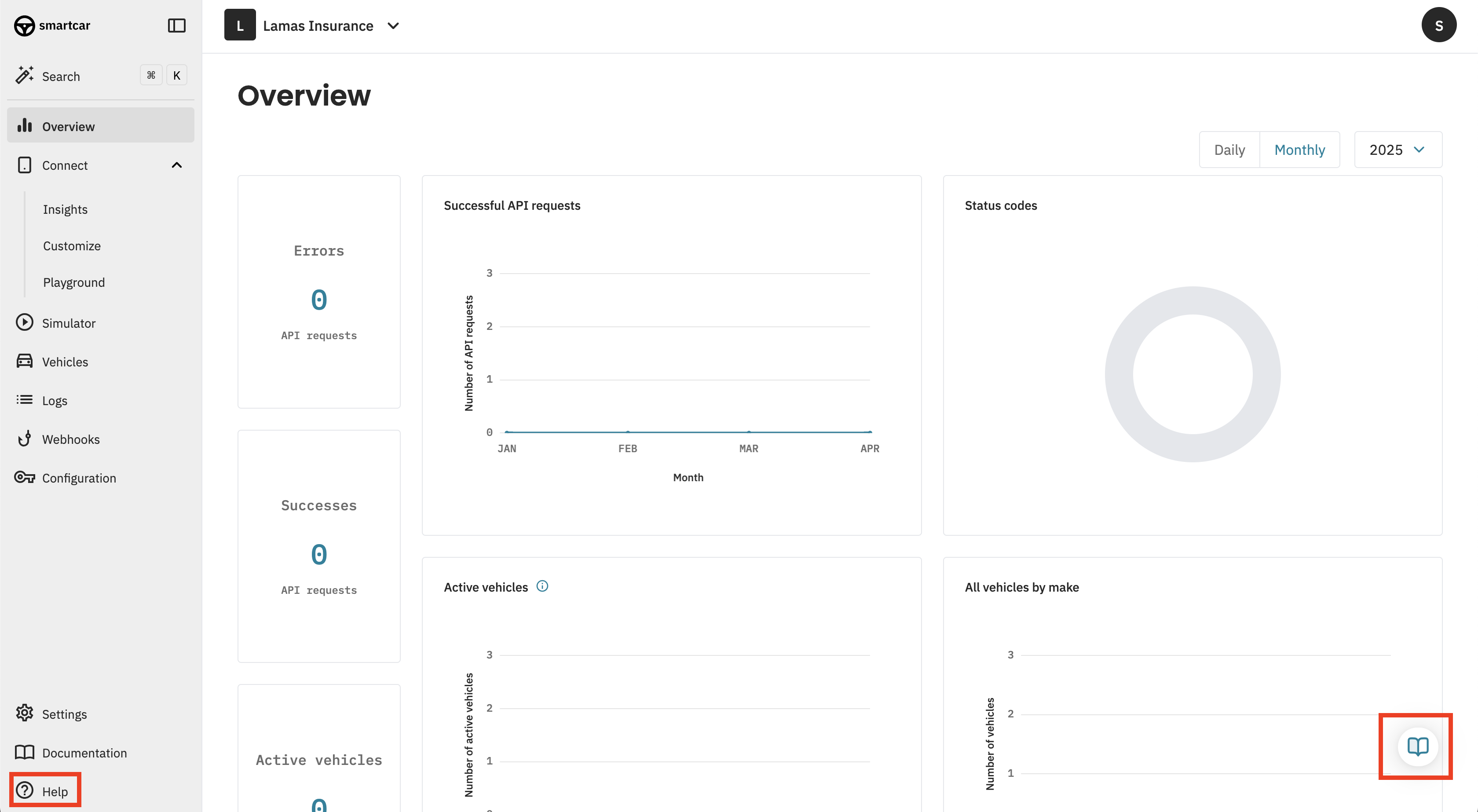
3. Select the **Support Center** option to be redirected to the full Support Center. 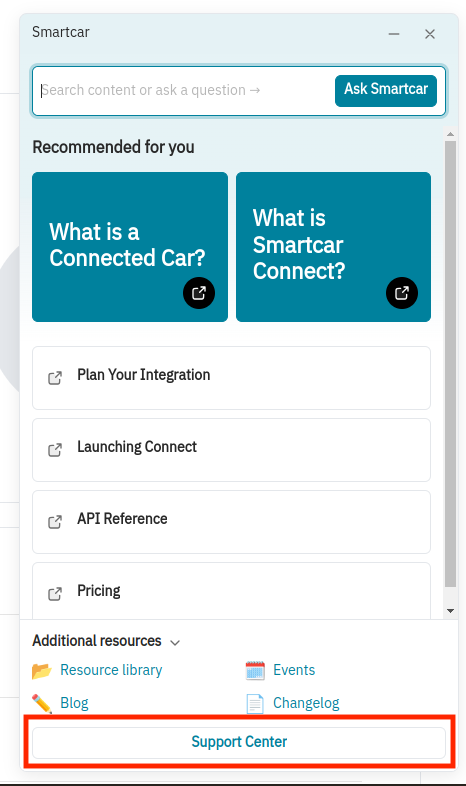
# Smartcar Usage Limits
Source: https://smartcar.com/docs/help/api-limits
Learn about limits you may encounter when using Smartcar.
## Overview
There are several types of limits that govern your use of Smartcar. The nature of telematics requires us to carefully manage vehicle connections and traffic to ensure the stability of our platform and those of our upstream providers.
There are two major types of limits - **Billing Limits** and **Operational Rate Limits**.
# Billing Limits
### [BILLING : VEHICLE\_REQUEST\_LIMIT](/errors/api-errors/billing-errors#vehicle-request-limit)
Your Smartcar plan specifies a maximum number of API calls you are permitted to make to each single vehicle in a given month. If you exceed this limit, you will receive the `VEHICLE_REQUEST_LIMIT` error.
For example: you have been allocated 500 API calls per vehicle per month, but you made the 501st call to a single vehicle during the billing period.
**This limit can be changed.** If you require more calls per vehicle per month, contact [support@smartcar.com](mailto:support@smartcar.com) or your Account Manager and we can work with you to understand your options.
### [BILLING : VEHICLE\_LIMIT](/errors/api-errors/billing-errors#vehicle-request-limit)
Your Smartcar plan specifies a maximum number of vehicles that you may have connected at any one time. This limit applies to vehicle connections, and is separate from the limit regarding the number of API calls to those vehicles. If you receive this error, you have exceeded your maximum number of connected vehicles.
**This limit can be changed.** Reach out to [support@smartcar.com](mailto:support@smartcar.com) or your Account Manager to discuss your options.
# Operational Rate Limits
### [RATE\_LIMIT : SMARTCAR\_API](/errors/api-errors/rate-limit-errors#smartcar-api)
Smartcar limits the total number of requests a single application can make over a given period of time to ensure platform stability for all customers. The Smartcar API Limit is defined as a "bucket" of requests that refills at a constant rate over time.
The default Smartcar API Limit is a **bucket of 120 requests that refills at a rate of 2 requests per minute.**
If you receive a `RATE_LIMIT:SMARTCAR_API` response, your application has exceeded this limit. You should implement an exponential backoff strategy when retrying requests.
**This limit can be changed.** Reach out to [support@smartcar.com](mailto:support@smartcar.com) or your Account Manager to discuss your options.
### [RATE\_LIMIT : VEHICLE](/errors/api-errors/rate-limit-errors#vehicle)
Sending telematics requests to a vehicle causes it to wake up from its sleep state and consume energy and data resources. Excessive requests to a single vehicle can result in:
* EV battery drain
* 12 volt battery drain
* `UPSTREAM:RATE_LIMIT` errors that prevent you from making requests for a longer period of time
Smartcar enforces a per-vehicle rate limit that governs the rate of requests to a single vehicle and mitigates these potential issues. These limits can differ based on the vehicle manufacturer and a number of other factors, and are set by Smartcar to ensure consistent and timely data can be retrieved from vehicles.
**This limit cannot be changed.** If you receive this response, refer to the `retry-after` header (returned as seconds) for when to retry the request.
### [UPSTREAM : RATE\_LIMIT](/errors/api-errors/upstream-errors#rate-limit)
Vehicle manufacturers sometimes impose rate limits on requests to vehicles. The Smartcar Vehicle Rate Limit will generally prevent those limits from being reached, but outside requests from other providers may cause the upstream limit to be exceeded. If the OEM upstream rate limit is exceeded, you will receive `UPSTREAM:RATE_LIMIT` from the Smartcar API for the affected vehicle until the limit is reset.
**This limit cannot be changed.** Smartcar cannot control rate limits imposed by our upstream providers. If you consistently receive this response for a single vehicle, reach out to [support@smartcar.com](mailto:support@smartcar.com) and we can investigate.
# Assist AI features in Slack
Source: https://smartcar.com/docs/help/assist-ai-slack
As part of our Enterprise Slack support offering, Smartcar includes access on select Enterprise plans to an AI-powered assistant that can help you get answers quickly, submit tickets, and navigate support resources—all without leaving your Slack workspace.
## 1. Ask a Question
In your dedicated Slack support channel, send a message with your question. The AI Agent will attempt to answer it based on Smartcar’s support documentation and past support interactions. 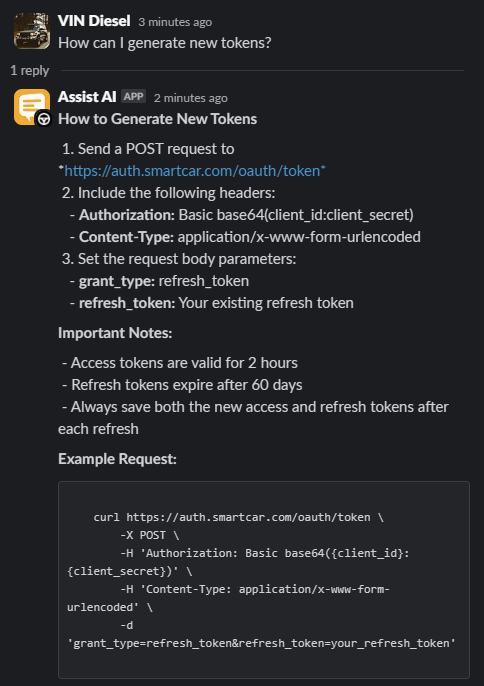
## 2. Submit a Support Ticket
If your question requires deeper support, simply click **"Create a Ticket"** at the bottom of the AI-generated response within the Slack channel. This will open a form to submit a formal, trackable support request. 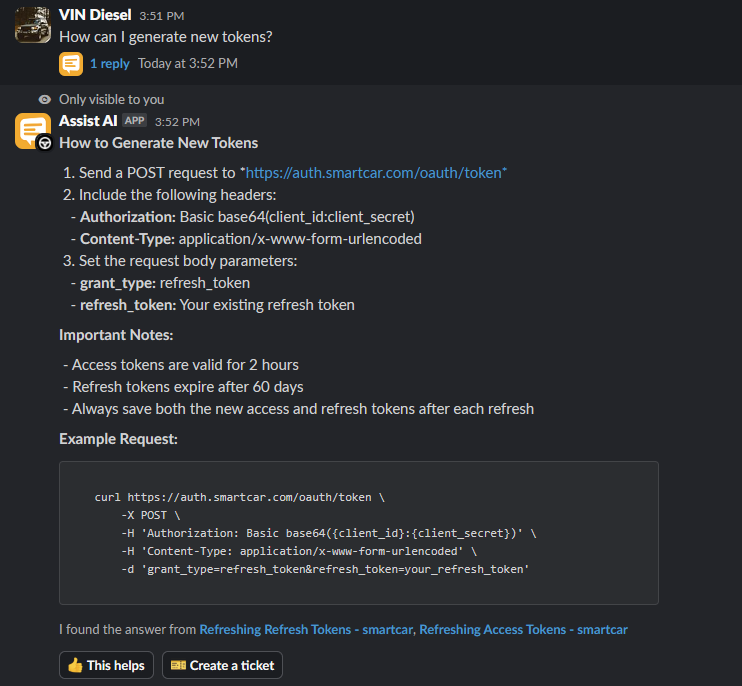 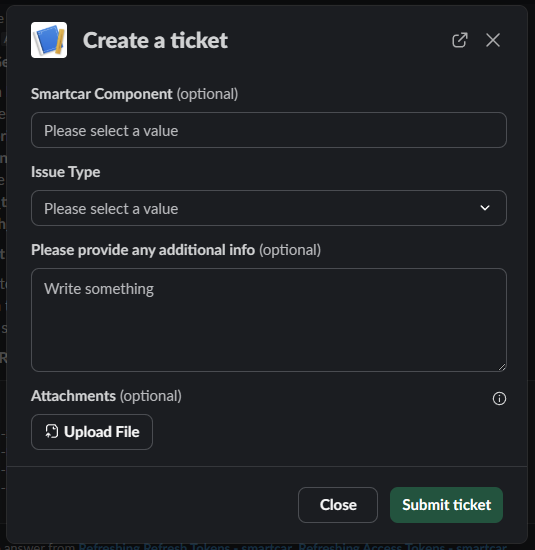
## 3. View Open Tickets
To check the status of an existing ticket, navigate to the Slack thread where the ticket was created and click the **"View Ticket Info"** icon. This will display the current status and details of your support request. 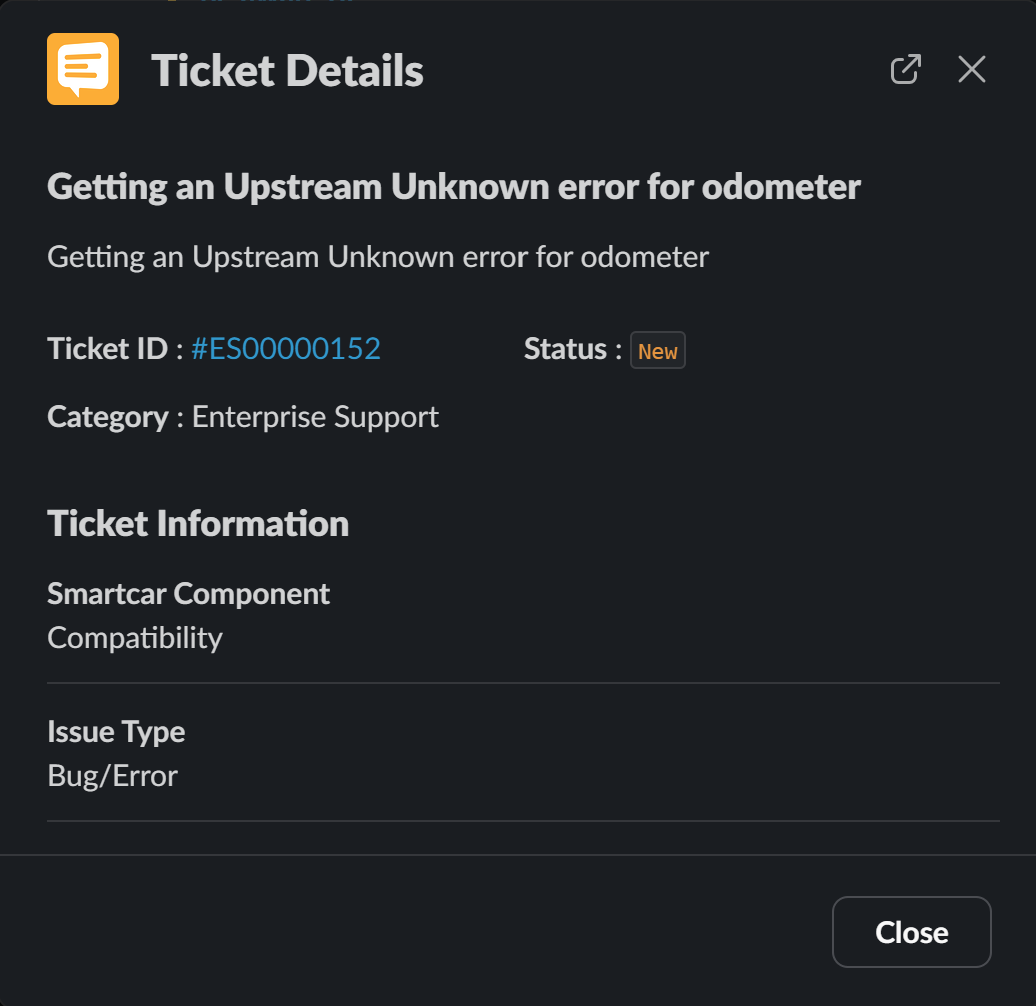
## 4. Navigate to the Support Center
Need more detailed help? You can access your ticket by clicking the **ticket ID** that appears after submitting a request using the **"Create a Ticket"** option. Alternatively, from the **"View Ticket Info"** window, you can click the **ticket number** to be redirected to the Smartcar Developer Dashboard. If you're already logged into an active session, you'll be taken directly to the ticket details within the Support Center. Otherwise, you'll be prompted to log in first.
In the Support Center, you can:
* Browse our documentation
* Submit new support requests
* View and update your existing tickets
For step-by-step instructions on how to log into the Smartcar Support Center, please see the following article: [Accessing the Smartcar Support Center](/help/accessing-support-center)
# Beta
Source: https://smartcar.com/docs/help/beta
Understand Smartcar's approach to features in beta.
Beta is an option that allows developers to test and integrate new Smartcar features before they are fully released. Beta refers to a phase where certain features are made available for testing and feedback prior to general availability. These features are functional but may still be under development or refinement.
## Benefits of Beta Features
Participating in beta allows developers to get a head start on integrating new features, a chance to influence the feature in development through feedback and help Smartcar ensure your app’s needs are met.
## Considerations for features in Beta
When working with beta features, keep in mind that features may change before final release. As such, extra caution is advised when using these features in production environments.
## How to Participate
If you would like to get involved in testing a beta features, contact your Account Manager or our [Support](support@smartcar.com) team for guidance.
# Brand Quirks
Source: https://smartcar.com/docs/help/brand-quirks
Brand specific quirks to keep in mind while building out your application.
## Acura
### Ignition On
Depending on model year, certain Acura vehicles do not return data when the ignition/engine is on.
### Sleep State
Most Acuras will go into a sleep state after about a week of inactivity,
## Audi
### Primary Key User
In order to have access to all vehicle functionality the account that connects to Smartcar will need to be flagged as a Key User by Audi.
## BMW/MINI
### CONNECTED\_SERVICES\_ACCOUNT - SUBSCRIPTION errors
Smartcar API will throw a CONNECTED\_SERVICES\_ACCOUNT - SUBSCRIPTION when the data we receive from the vehicle is older than a month as this normally indicates a subscription issue.
## Ford/Lincoln
### Authentication
Connecting Ford and Lincoln vehicles requires the use of one of our mobile SDKs. We currently offer SDKs for iOS, Android, and Flutter. Smartcar's Connect SDKs will redirect to Ford’s site to handle the authentication process when the user logs in.
**Authentication via non-mobile platforms such as web browsers is not supported at this time**. See [Connect SDKs](/docs/connect/connect-sdks) for our latest SDKs.
## GM (Buick, Chevrolet, Cadillac, GMC)
### VEHICLE\_STATE - ASLEEP errors
After 3-4 days of no activity, GM vehicles will enter a deep sleep state at which point they will no longer respond to API requests to preserve their 12v battery. In order to get data from the vehicle again, the car will need to go through an ignition cycle.
### UPSTREAM - RATE\_LIMIT errors
In order to avoid hitting UPSTREAM - RATE\_LIMIT errors use batch requests and ping no more than once every 30 minutes. Use of the OEM app also counts towards the rate limit.
## Hyundai/Kia
### Polling Limitations
Hyundai/Kia vehicles have a limitation where only 20 requests can be made in a 24 hour period. Smartcar allows one requests every 72 minutes to go to the vehicle directly. All other requests will be sent to the OEM's cloud which may be updated after events such as:
* Every 10% of SoC while charging
* 5 minutes after ignition off
* Vehicle doors are left unlocked for 5 minutes
* After a command successfully completes
* Whenever charging stops
## Nissan
### “Vehicle has been found” MyNISSAN notifications
Vehicle owners may receive a notification from the MyNISSAN app stating “Success! We found the location of your vehicle. Check the map for the location of your YYYY Model.” every time the location endpoint is hit.
The app appears to have an option to edit notifications, but as of Feb 2024 they are not available in the app. Notifications may need to be turned off at the iOS/Android level by the vehicle owner.
## PSA Group (Citroen, DS, Opel, Peugeot, Vauxhall)
### Requesting control\_charge permissions
If you request control\_charge permissions for PSA EVs, upon login owners will be presented with a PIN and MFA screen in Connect upon submitting their credentials. They will need to have set this up on their OEMs application in order to grant your application this permission.
## Rivian
### MFA
If MFA is enabled the vehicle owner will need to re-authorize via Connect about every 2 weeks.
## Tesla
### Remote Access
It may take a few hours or up to a full day for the REMOTE\_ACCESS\_DISABLED errors to subside after remote access is enabled.
### Charge Billing Records
In order to retrieve charge billing records, the main Tesla account must connect the vehicle, as driver profiles do not have access to this data.
### Virtual Key
Tesla now requires virtual keys for 3rd-party applications in order to issue commands for the following models:
* All Cybertrucks, Model 3 and Model Y
* 2021+ Model S and X
## Toyota/Lexus
### Prius Prime - Location
Due to very low reliability/accuracy of the location endpoint for Prius Primes, we currently do not support that endpoint for the Prius Prime vehicles specifically. The regular Prius vehicle **do** support location.
## Volkswagen
### Control charge limits
Volkswagen only allows 15 charge commands (start/stop charge) before the vehicle needs to be driven again in order to start responding to start/stop requests.
### CONNECTED\_SERVICES\_ACCOUNT errors on lock/unlock commands
In addition to verifying the VW account with the activation code, some cars will need to undergo the VW Ident Process before you can access remote lock/unlock functionality. This involves contacting the dealership to verify your ownership of the vehicle.
### Missing Subscription (European VWs)
Upon purchasing (or activating the free trial), VW needs to review the request. You will get an email confirmation once they've cleared everything on their side.
### Primary Driver Status
In order to fully interact with Smartcar, the credentials need to be flagged as the primary driver. You'll need to tap the "become primary driver" flow in the VW app. Depending on the model, this may require you to be in the car to interact with the infotainment system.
## Volvo
### Lock/unlock commands
Volvo is an outlier regarding how unlock requests are processed. After initiating the request, after about 10 seconds you'll need to open the trunk (boot) to successfully complete the request. You'll know the car is ready to unlock the trunk as the hazard lights will flash. We recommend adding a notification for Volvos as part of the request loading animation to inform the user of this process.
### Phone number username
Smartcar Connect currently checks for an email format, as such phone number usernames cannot be used. The owner will need to add their email to their Volvo account via the web portal.
### Compatibility
Some models are not compatible because the device needs to be physically in the vehicle during authentication, which Connect doesn’t support at the moment:
* 2021+: XC40 Recharge
* 2022+: S90, S90 Recharge, V90, V90 Recharge, V90 Cross Country, XC60, XC60 Recharge, C40 Recharge
* 2023+: All models with the exception of the XC40
Even if the vehicle has been connected to and shows up in the Volvo app, if they go through Connect the account will present as if there are no vehicles on the account.
# Brand Subscription Information
Source: https://smartcar.com/docs/help/brand-subscriptions
Below you can find the connected service name for each brand as well as any specific subscription packages needed to connect to the vehicle via Smartcar.
| Make | Region | Connected Service | Package | Subscription Cost |
| --------------------------- | --------------------------- | ------------------------------------------- | ------------------------------------------------- | ------------------------------------------------------------------------------------------------------------- |
| Acura | US, Canada | ACURALINK | Remote | 3 year free trial (2023 and Newer Models), 6 month free trial (2022 and Older Models). \$110/year thereafter. |
| Alfa Romeo | US, Europe | My Alfa Connect | My Car and My Remote | 3 - 5 years included. |
| Audi | US | myAudi | CARE | - |
| | Europe, Canada | Audi Connect | Remote | Free for three years after delivery of a new vehicle. \~£120/year thereafter. |
| BMW | All | BMW Connected | - | Free trial varies with model year. \~\$120/year thereafter. |
| Buick | US, Canada | OnStar | Remote Access | Free trial varies with model year. \$14.99/month for standalone Remote Access package. |
| Cadillac | US, Canada | OnStar | Remote Access | Free trial varies with model year. \$14.99/month for standalone Remote Access package. |
| Chevrolet | US, Canada | OnStar | Remote Access | Free trial varies with model year. \$14.99/month for standalone Remote Access package. |
| Chrysler | US, Canada | Uconnect | Sirius XM Guardian (Remote Package) | 1 year free trial. \$12.99/month for the Remote Package thereafter. |
| Citroën | Europe | My Citroën | ë-remote services needed for remote commands | - |
| CUPRA | Europe | CUPRA CONNECT | Remote Access Services | 3 years free for the Born model, 1 year free for others. Price varies with region. |
| Dacia | Europe | My Dacia | - | |
| Dodge | US, Canada | Uconnect | Sirius XM Guardian (Remote Package) | 1 year free trial. \$12.99/month for the Remote Package thereafter. |
| DS | Europe | MyDS | E-TENSE Remote | - |
| Fiat | Europe | FIAT | My Car and My Remote | 6 month free trial. |
| | US | FIAT Connect | Assistance Package | 3 month free trial with select vehicles. \$14.99/month. |
| Ford | All | FordPass | - | - |
| GMC | US, Canada | OnStar | Remote Access | Free trial varies with model year. \$14.99/month for standalone Remote Access package. |
| Honda | US | HondaLink | EV Access for EV Data. Remote Access for Commands | EV Access 8 year trial. \$14.99/month for standalone Remote Access package. |
| Hyundai | All | Hyundai BlueLink | - | 3 year free trial for new vehicles. \$9.90/month per package thereafter. |
| | US | Hyundai BlueLink (2023 IONIQ 6 & all 2024+) | BlueLink+ | - |
| Infiniti | US | MyINFINITI | - | 1 year free trial. \$12.99/month thereafter. |
| Jaguar | All | Jaguar InControl | - | 3 year free trial. \~\$100/year thereafter. |
| Jeep | US, Canada | Uconnect | Sirius XM Guardian (Remote Package) | 1 year free trial. \$12.99/month for the Remote Package thereafter. |
| | US, Canada | Jeep Connect | Sirius XM Guardian (Remote Package) | 1 year free trial. \$12.99/month for the Remote Package thereafter. |
| Kia | US, Canada | Kia Access | Plus | 1 year free trial. \$149/year thereafter. |
| | Europe | Kia Connect | - | 7 year free trial starting on sale to the first owner. |
| Land Rover | All | Land Rover InControl | - | 3 year free trial. \~\$100/year thereafter. |
| Lexus | US, Canada | Lexus Enform | REMOTE | Free trial up to 3 years, varies with model year. \$80/year thereafter. |
| Lincoln | US | Lincoln Way | - | - |
| Mercedes | All | MeConnect | - | 1 year free trial. \~\$15/year there after. |
| Mini | All | Mini Connected | - | Free trial varies with model year. \~\$120/year thereafter. |
| Mazda | US, Europe | MyMazda | - | 3 year free trial. \$10/month thereafter. |
| Nissan | US | NissanConnect Services | - Convenience - Select (EV data) | Free trial varies with model year. $9.99 - $ 21.98/month thereafter. |
| | Europe | NissanConnect | - | Free trial varies with model year. \~£1.99/month thereafter. |
| Opel | Europe | myOpel | e-Remote services needed for remote commands | - |
| Peugeot | Europe | MYPEUGEOT | E-REMOTE CONTROL needed for remote commands | - |
| Porsche | US, Europe | My Porsche | | 1 year free trial after initial purchase. \$299/year there after. |
| RAM | US, Canada | Uconnect | Sirius XM Guardian (Remote Package) | 1 year free trial. \$12.99/month for the Remote Package thereafter. |
| | US, Canada | Ram Connect | Sirius XM Guardian (Remote Package) | 1 year free trial. \$12.99/month for the Remote Package thereafter. |
| Renault | Europe | My Renault | - | - |
| Rivian | US | Rivian | - | - |
| Skoda | Europe | MySKODA | Care Connect | \~£36/year. |
| Subaru (excluding Solterra) | US | MySubaru | STARLINK Security Plus | 6-month free trial. \$4.95/month thereafter. |
| Subaru Solterra | US | Solterra Connect | Remote Connect | One-year trial included, \$8/month after trial. |
| Tesla | All | Tesla | - | - |
| Toyota | US, Canada | Toyota Connected Services | Remote Connect | 1 year free trial. \$80/year thereafter. |
| Vauxhall | Europe | myVauxhall | e-Remote services needed for remote commands | - |
| Volvo | All | Volvo Cars | - | Free trial varies with model year. \~\$200/year thereafter. |
| Volkswagen | US | Car-Net | Remote Access | - |
| | Europe (non-ID) 2018 - 2022 | Car-Net | - | - |
| | Europe (non-ID) ≤ 2023 | We Connect | - | - |
| | Europe (non-ID) 2024+ | VW Connect | - | - |
| | Europe (ID) Firmware \<3 | We Connect Start | - | - |
| | Europe (ID) Firmware v3+ | We Connect ID | - | - |
## Notes
### Audi
* Owners will need to ensure they're the Primary or Key user for the vehicle connected to their account in order to access all Smartcar functionality.
### Ford and Lincoln
* Owners that use a username to login to their connected services app will need to migrate to using their email address under account settings in their Ford Pass or Lincoln Way portal.
### Onstar
* Chevy Bolts MY 2020+ come with the **EV Mobile Command** service included for 5 years with the purchase of a new vehicle. Location data is not included in the package. Bolt owners will need to also subscribe to **Remote Access** to be able to get access to location data.
* For all other OnStar accounts, we require the modern **Remote Access** plan as our base subscription. This plan comes with the **Vehicle Status** feature set, which is what enables our remote access to data from cars.
More information can be found [in this PDF](https://my.chevrolet.com/content/dam/gmownercenter/gmna/dynamic/onstar/comparePlans/nbm/OC_Compare-Plans-Chevy.pdf).
* There are certain legacy OnStar accounts floating around with similar names. A good check on whether a customer has the correct plan or not is the ability to view vehicle information like fuel level, EV battery level, odometer, or tire pressure from within their vehicle’s mobile app.
### Nissan US
* Convenience features are included as part of the Premium package.
### Toyota
* The Remote Connect subscription is tied to the audio package purchased with the vehicle. Compatible audio package availability is determined based on the trim of the vehicle model and varies model year to model year.
Toyota provides PDFs for Remote Connect availability [here](https://www.toyota.com/connected-services/#:~:text=Learn%20about%20your%20Connected%20Services).
### Uconnect
* For all Uconnect accounts, we require the ability to interact with vehicle information through the UConnect portal
* A Uconnect account may have access to Mopar, but not all Mopar accounts have access to UConnect. If a user can see information in Mopar but not UConnect, they’ll need to reach out to UConnect support to set up the right UConnect plan for their vehicle.
* 2021+ Jeeps have their own connected service - Jeep Connect
* 2022+ Rams have their own connected service - Ram Connect
* 2022+ Jeep Wagoneers have their own connected service - Wagoneer Connect
* Canada has limited support listed [here](https://www.driveuconnect.ca/en/sirius-xm-guardian-vehicles)
### Volkswagen ID Series
* Model year 2021 and certain 2022 Volkswagen ID vehicles require an upgrade to vehicle software version 3 which will allow you to get location and odometer data. This is a requirement for both US and European vehicles.
### Volvo
The following models are not currently compatible with Smartcar:
* 2021+ : XC40 Recharge
* 2022+ : S90, S90 Recharge, V90, V90 Recharge, V90 Cross Country, XC60, XC60 Recharge
* 2023+ : All models with the exception of the XC40
# Compatible Vehicles
Source: https://smartcar.com/docs/help/compatibility
# Connect your car
Source: https://smartcar.com/docs/help/connect-your-car
# Diagnostic Systems
Source: https://smartcar.com/docs/help/diagnostic-systems
To support our System Status endpoint, reference the below list of unified systems.
The [System Status](https://smartcar.com/docs/api-reference/get-system-status) endpoint returns a list of systems reported by any given vehicle, as well as their current state which may be "OK" or "ALERT".
## System IDs
| | |
| --------------------------------- | --------------------------------------------------------- |
| `SYSTEM_ABS` | Antilock Braking Systems |
| `SYSTEM_ACTIVE SAFETY` | Active Safety Systems |
| `SYSTEM_AIRBAG` | Airbag Systems |
| `SYSTEM_BRAKE_FLUID` | Brake Fluid Level |
| `SYSTEM_DRIVER_ASSISTANCE` | Driver Assistance Systems |
| `SYSTEM_EMISSIONS` | Emissions Systems |
| `SYSTEM_ABS` | Antilock Braking Systems |
| `SYSTEM_ENGINE` | Engine Systems |
| `SYSTEM_EV_BATTERY_CONDITIONING` | EV Battery Conditioning Systems |
| `SYSTEM_EV_CHARGING` | EV Charging Systems |
| `SYSTEM_EV_DRIVE_UNIT` | EV Drive Unit Systems |
| `SYSTEM_EV_HV_BATTERY` | EV High-Voltage Battery Systems |
| `SYSTEM_LIGHTING` | Lighting Systems |
| `SYSTEM_MIL` | Malfunction Indiciator Lamp Systems (Check Engine Lights) |
| `SYSTEM_OIL_LIFE` | Oil Life Systems |
| `SYSTEM_OIL_PRESSURE` | Oil Pressure Systems |
| `SYSTEM_OIL_TEMPERATURE` | Oil Temperature Systems |
| `SYSTEM_TELEMATICS` | Telematics Systems |
| `SYSTEM_TIRE_PRESSURE` | Tire Pressure Systems |
| `SYSTEM_TIRE_PRESSURE_MONITORING` | Tire Pressure MonitoringSystems |
| `SYSTEM_TRANSMISSION` | Transmission Systems |
| `SYSTEM_WASHER_FLUID` | Washer Fluid Systems |
| `SYSTEM_WATER_IN_FUEL` | Water in Fuel Detection Systems |
# Frequently Asked Questions (FAQs)
Source: https://smartcar.com/docs/help/faqs
Below you'll find answers to some of the most frequently asked questions about Smartcar.
Smartcar does not build applications ourselves. Rather, we provide the building blocks that our customers need in order to build applications for a variety of use cases.
Yes. For instance, if you own a VW, you will need to have Car-Net activated in the US or WeConnect in Europe. You can read more about connected service subscriptions [here](/help/brand-subscriptions).
Take a look at our [Global Compatibility page](https://smartcar.com/global/) for the latest information on where Smartcar works.
Smartcar is always expanding and working hard to ensure supported countries are stable and reliable. If your country is not currently supported, but you think we'll be a good fit for your use case, [schedule a demo](https://smartcar.com/demo/) and let's see how we can help you!
[Smartcar Connect](/connect/what-is-connect) is available in English, Danish, German, Spanish (Spain), Spanish (Latin America), French, Italian, Dutch, Norwegian, and Swedish providing vehicle owners a native experience as they onboard vehicles to your platform.
Smartcar will always try to pull the latest data point from any given vehicle. Each API response includes an [sc-data-age header](https://smartcar.com/docs/api#response-headers) that will contain the timestamp of when that data was last saved.
Although each OEM saves data at different times, the data age header should not be older than the last time the vehicle was used.
For a list of compatible vehicles, visit [our website](https://smartcar.com/product/compatible-vehicles/).
Smartcar is compatible with **161 million vehicles** across the US, Canada and Europe.
**United States**
Smartcar is compatible with **29 car brands** in the United States.
**Europe**
Smartcar is compatible with **19 car brands** in Europe.
**Canada**
Smartcar is compatible with **21 car brands** in Canada.
**If your make isn't supported...**
We work hard to ensure that supported brands are stable and reliable, which takes time. If you don't see your make on our [compatibility page](https://smartcar.com/product/compatible-vehicles/) but you would like it to be, reach out to [support@smartcar.com](mailto:support@smartcar.com) to see how you can help speed up the process!
**If we support your make, but not your model...**
We might just need to do some additional testing. Sign up to our [research fleet](https://smartcar.com/research-fleet) and we'll reach out to see what we can do for you!
**If we support your make, model, but not your year...**
The majority of automakers only started adding internet connectivity to their cars around 2014. If you're able to access your car through a smartphone application and believe it should be supported, reach out to us and we'll be happy to take a look.
While on-board diagnostics dongles are versatile and useful for tracking and diagnostics of many kinds, they are far from ideal when it comes to mileage verification.
They can be inaccurate, inconvenient, expensive and unreliable.Smartcar is only one example of a pure software alternative that uses API technology to offer the perfect
mileage verification solution to auto insurance companies. Compared with traditional OBD-II devices, our API is accurate, cost efficient and tamper-resistant.
You can read more about why using our API is a great choice on our [blog](https://smartcar.com/blog/obd-mileage-verification/).
In the event that we recieve a response from the OEM after the connection is closed, we will show the error code and error type we would have responded with on the Dashboard if the connection had been kept open.
# Submit a Feature Request
Source: https://smartcar.com/docs/help/feature-request
## Help Us Improve Smartcar!
Your feedback shapes our future. Have an idea or improvement you’d love to see? Submit your suggestions using the form below, and let us know how we can better serve your needs.
If you have trouble seeing the form below, please visit [this link](https://smartcarapi.notion.site/215f1477e9d380538219cb34866b8540?pvs=105) to submit your feature request.
# How to Submit a Ticket in the Support Center
Source: https://smartcar.com/docs/help/how-to-submit-ticket
Learn how to submit a support ticket through the Smartcar Support Center, including step-by-step instructions and eligibility details based on your plan.
For information on how to access the Smartcar Support Center, please visit the following article: [Accessing the Smartcar Support Center](/help/accessing-support-center)
***
### **How to Submit a Support Ticket**
**1. Select "Submit a Ticket"**\
Once in the Support Center, click **"Submit a Ticket"** at the top right corner of the page to begin creating your ticket. 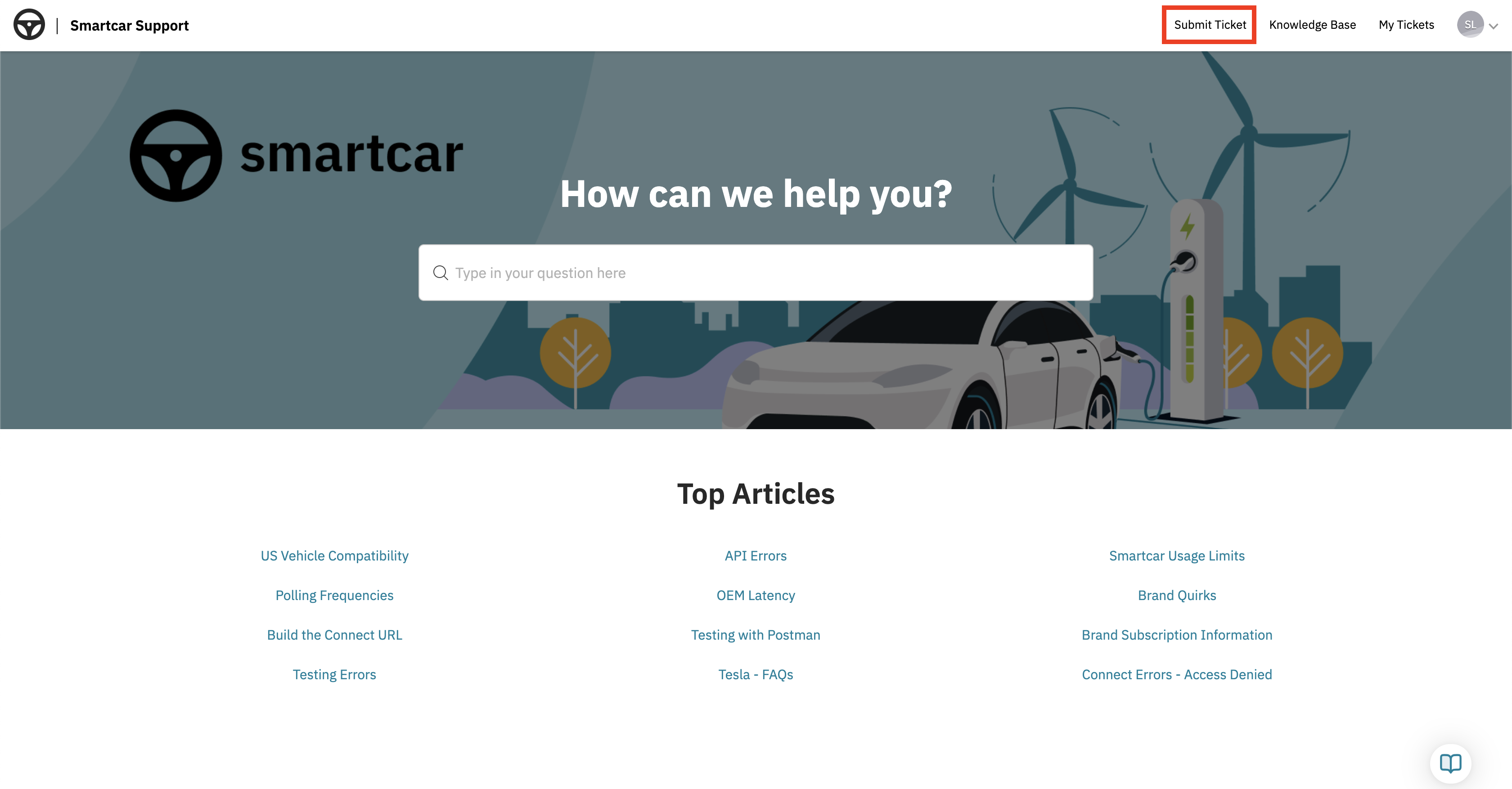
**2. Fill Out the Ticket Form**\
Provide details such as:
* Subject
* Description
* Please include as much relevant context as possible such as Session IDs, Vehicle IDs, screenshots, etc.
* The Smartcar Component affected
* The issue type
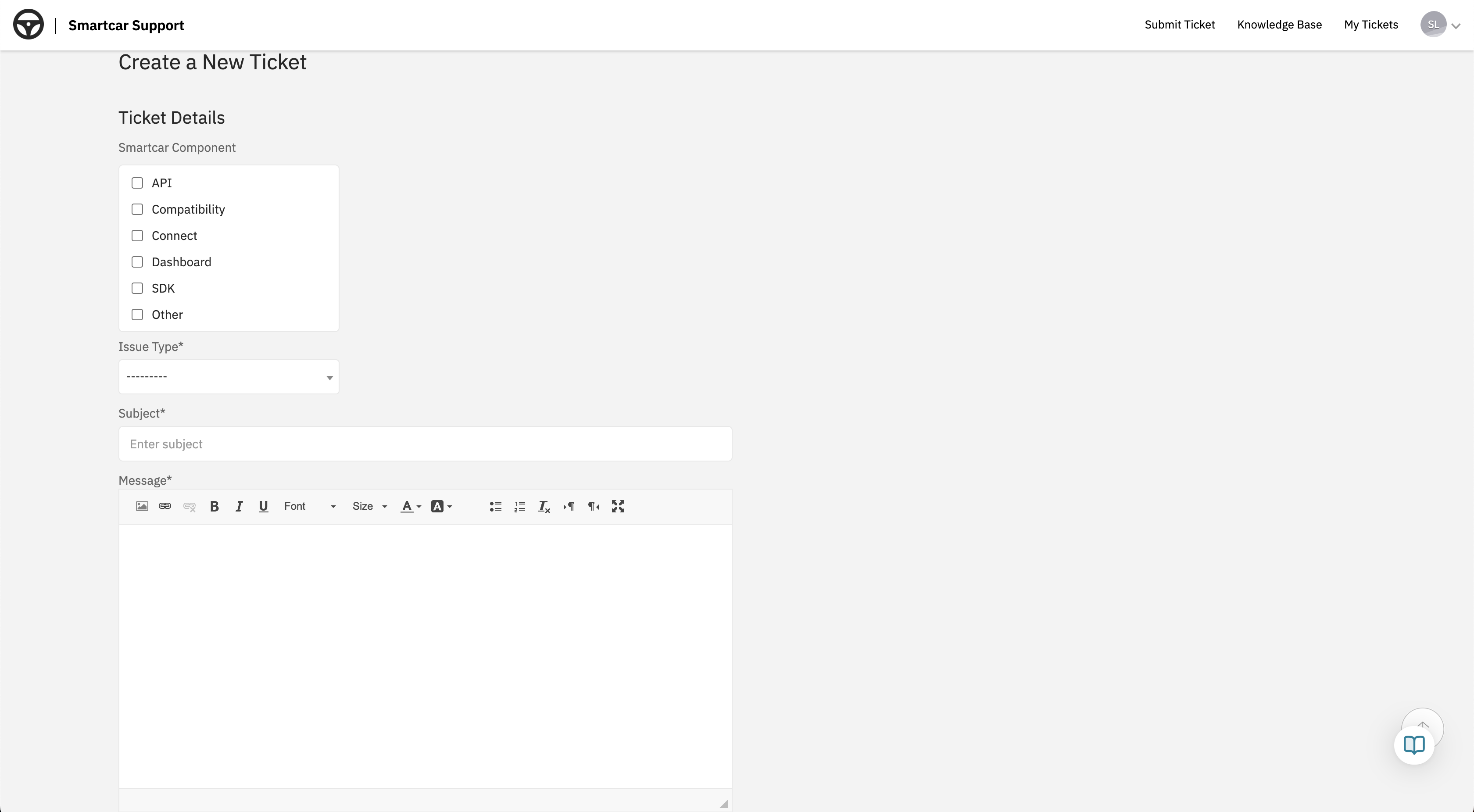
**3. Submit Your Ticket**\
Click **Submit**, and your request will be sent to our Support team.
**4. Track Your Request**\
You can view and track the status of your tickets under **"My Tickets"** in the Support Center. 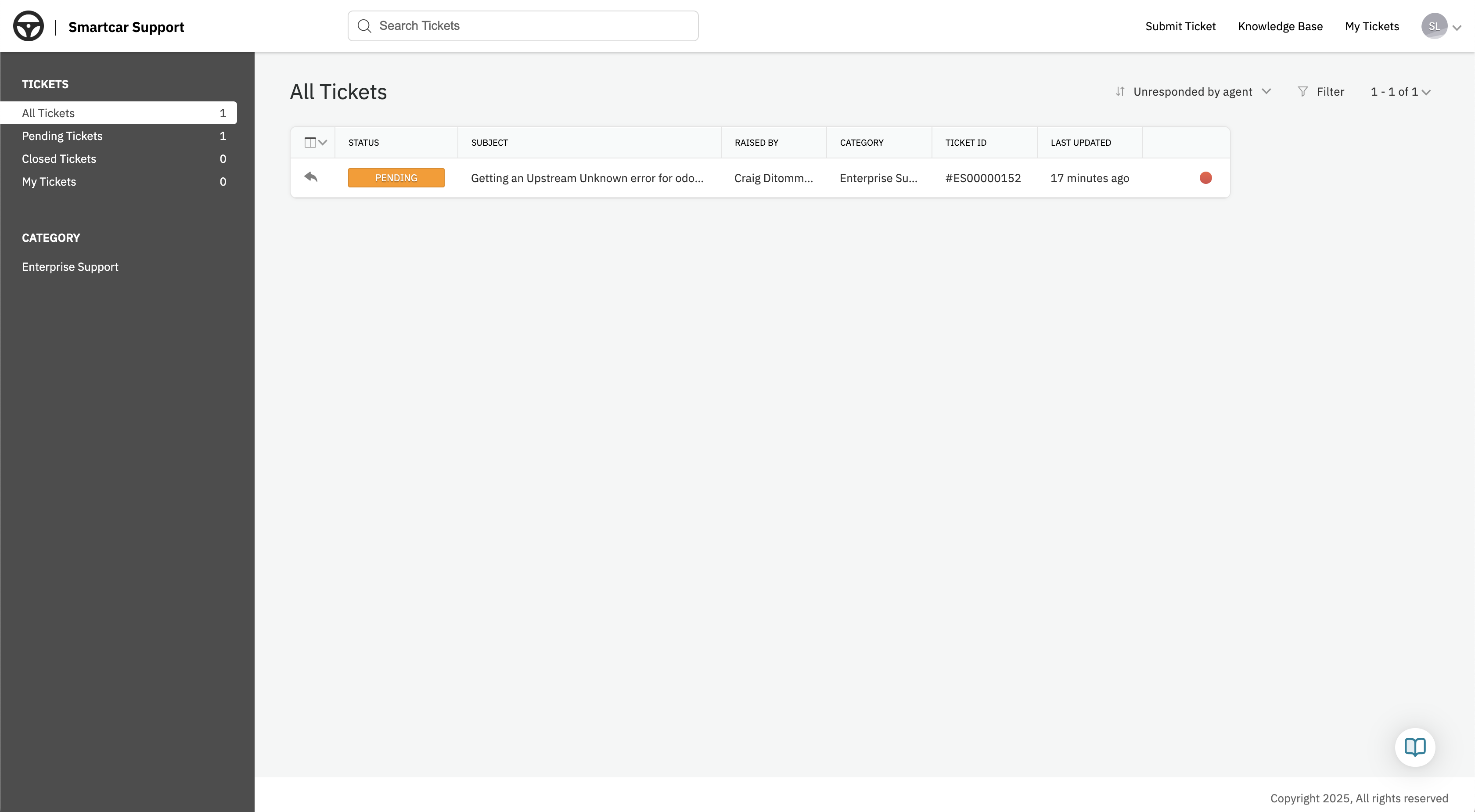
**5. Update or Close a Request**\
Select the ticket you'd like to update or close from the **"My Tickets"** view. Once you're on the ticket page:
* Click **"Reply"** at the bottom left to add more information or request a status update.
* Click **"Close Ticket"** at the bottom right to mark the request as **Closed - Resolved** if you no longer need assistance. 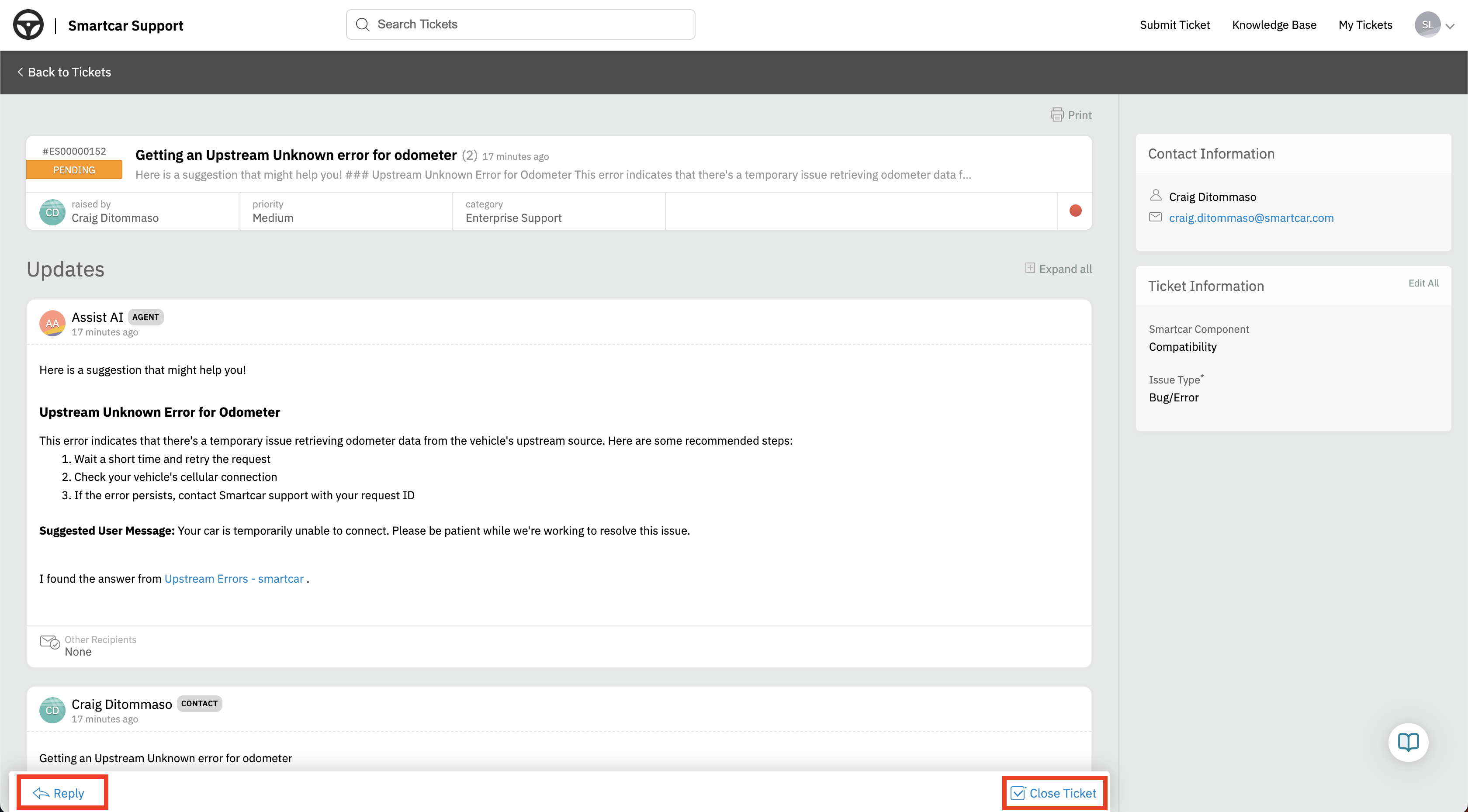
***
### **Who can submit tickets?**
Ticket submission is available to users on **Build** and **Custom** **Enterprise** plans.
**Free plan users** can access the Docs Center and AI Agent within the Dashboard for assistance but do not have access to submit tickets.
# mySmartcar
Source: https://smartcar.com/docs/help/my-smartcar
With [mySmartcar](https://my.smartcar.com/login), you can see which apps are connected to your vehicle and manage their permissions anytime.
## Log in
Log into [mySmartcar](https://my.smartcar.com/login) by providing your preferred email address. A 6-digit code will be sent to you by email, which will grant access to mySmartcar.
## Connect your OEM account
Now press "Connect your Car" and follow the on-screen prompts to pair your Connected Services account with mySmartcar.
## Review and manages connected apps
Once your OEM account is connected, Smartcar will provide a list of each application connected via Smartcar to your Connected Services account. Here you can see when the app was first granted access and the permissions requested.
Should you wish to disconnect an application, click "Revoke Access" and confirm to remove access to your vehicle's data for that application.
# Tesla Permission Info
Source: https://smartcar.com/docs/help/oem-integrations/tesla/developers
This page has information regarding Smartcar's upgraded Tesla integration.
## Updating access with Tesla
You can use either of the following URLs to prompt users for more Tesla
permissions in the event you need additional access from the
vehicle owner due to:
* A [PERMISSION](/errors/api-errors/permission-errors) error from API
* A [CONNECTED\_SERVICES\_ACCOUNT:PERMISSION](/errors/api-errors/connected-services-account-errors#permission) error from API
* Needing access to an endpoint out of scope for your existing permissions
If one or more of the Smartcar scopes you pass map to a new Tesla permission(s)
for the account, the user will be prompted to update access after they log in
with Tesla.
This flow sends a new authorization code to your callback URI in order to fetch a new access and refresh token.
```
https://connect.smartcar.com/oauth/{path}?
response_type=code
&make=TESLA
&client_id=8229df9f-91a0-4ff0-a1ae-a1f38ee24d07
&scope=read_odometer control_security
&redirect_uri=https://example.com
```
| name | type | required | description |
| --------------- | --------------- | -------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| `response_type` | `string` | true | This should be set to `code`. |
| `make` | `string` | true | Specifies the brand to update access to. Currently, the only make available for this flow is `TESLA`. |
| `client_id` | `string` | true | The application’s unique identifier. This is available on the credentials tab of the Smartcar Dashboard. |
| `scope` | `[permissions]` | true | A space-separated list of permissions that your application is requesting access to. The valid permission names can be found in the [permissions](/api-reference/permissions) section. When reauthenticating, the user will be required to grant the corresponding OEM permissions before being able to exit the flow. |
| `redirect_uri` | `string` | true | Required if using the `/authorize` route for Smartcar to return an authorization code. |
This flow **will not** send back an authorization code to fetch a new
refresh and access token, or redirect the user back to your application.
Continue to use the access and refresh tokens you have on file for
associated vehicles.
```
https://connect.smartcar.com/oauth/reauthenticate?
response_type=vehicle_id
&make=TESLA
&client_id=8229df9f-91a0-4ff0-a1ae-a1f38ee24d07
&scope=read_odometer control_security
```
| name | type | required | description |
| --------------- | --------------- | -------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| `response_type` | `string` | true | This should be set to `vehicle_id` . |
| `make` | `string` | true | Specifies the brand to revoke access to. Currently, the only make available for this flow is `TESLA`. |
| `client_id` | `string` | true | The application’s unique identifier. This is available on the credentials tab of the Smartcar Dashboard. |
| `scope` | `[permissions]` | true | A space-separated list of permissions that your application is requesting access to. The valid permission names can be found in the [permissions](/api-reference/permissions) section. When reauthenticating, the user will be required to grant the corresponding OEM permissions before being able to exit the flow. |
## Requiring Tesla Permissions
To ensure users select the necessary permissions with Tesla before
being able to connect their vehicle via Smartcar, please append the `required:`
prefix to Smartcar scopes in your Connect URL e.g. `required:read_location`.
As a result if they do not select the necessary permissions with Tesla, they will see the
following screen and be prompted to return to Tesla to update their permissions.
## Permission Mappings
Tesla provides a limited set of permissions. To ensure vehicle data is shared only with explicit consent, Smartcar has mapped Tesla’s permissions into its existing more granular options. For example, granting access to “vehicle commands” in a Tesla account allows an application to start or stop charging, lock or unlock doors, enable or disable Sentry Mode, and more. Smartcar separates these permissions so that applications must request access to control charging separately from access to vehicle security, control navigation, etc. Smartcar does not use or share vehicle data beyond the permissions listed in this table. An application with the ability to control the charge status of a vehicle, does not have access to control other aspects of the car unless explictly requested and granted by a vehicle owner.
| Smartcar Permission | Tesla Permission |
| ---------------------------- | --------------------------------------------------- |
| `control_charge` | Vehicle Charge Management (vehicle\_charging\_cmds) |
| `control_climate` | Vehicle Commands (vehicle\_cmds) |
| `control_navigation` | Vehicle Commands (vehicle\_cmds) |
| `control_pin` | Vehicle Commands (vehicle\_cmds) |
| `control_security` | Vehicle Commands (vehicle\_cmds) |
| `control_trunk` | Vehicle Commands (vehicle\_cmds) |
| `read_battery` | Vehicle Information (vehicle\_device\_data) |
| `read_charge_records` | Vehicle Charge Management (vehicle\_charging\_cmds) |
| `read_charge` | Vehicle Information (vehicle\_device\_data) |
| `read_climate` | Vehicle Information (vehicle\_device\_data) |
| `read_compass` | Vehicle Information (vehicle\_device\_data) |
| `read_engine_oil` | Vehicle Information (vehicle\_device\_data) |
| `read_extended_vehicle_info` | Vehicle Information (vehicle\_device\_data) |
| `read_fuel` | Vehicle Information (vehicle\_device\_data) |
| `read_location` | Vehicle Location (vehicle\_location) |
| `read_odometer` | Vehicle Information (vehicle\_device\_data) |
| `read_security` | Vehicle Information (vehicle\_device\_data) |
| `read_speedometer` | Vehicle Information (vehicle\_device\_data) |
| `read_thermometer` | Vehicle Information (vehicle\_device\_data) |
| `read_tires` | Vehicle Information (vehicle\_device\_data) |
| `read_user_profile` | Profile Information (user\_data) |
| `read_vehicle_info` | Vehicle Information (vehicle\_device\_data) |
| `read_vin` | Vehicle Information (vehicle\_device\_data) |
# Tesla FAQs
Source: https://smartcar.com/docs/help/oem-integrations/tesla/faqs
FAQs on our new Tesla integration. For an overview of the changes see [this page](https://smartcar.com/docs/help/oem-integrations/tesla/whats-new).
#### What happens if I connect to one of my applications with the legacy flow and another with the new flow?
As long as you have access to the same set of permissions on Tesla’s side and have added our virtual key there will not be any conflicts while the legacy API is active.
#### If my users have more than one Tesla on their account, will they need to add the virtual key to all of their vehicles?
Yes. You will need to add our virtual key to each vehicle on the Tesla account.
#### What happens if I issue a command but Smartcar’s virtual key hasn’t been added to the vehicle?
We will throw a `CONNECTED_SERVICES_ACCOUNT:VIRTUAL_KEY_REQUIRED` error.
## Do all vehicles need a virtual key?
2020 and earlier Model S and X **will not** require a virtual key to keep
working at this time. Smartcar will poll these vehicles in the background every
5 minutes for data. These requests **will not** wake the vehicle.
#### What happens if a user does not add a virtual key for vehicles that support streaming?
If a vehicle is streaming capable and does not yet have a virtual key installed,
requests to the vehicle will be limited to 1 request every 8 hours for data. These requests **will
not** wake the vehicle.
#### What happens if I issue a command but the owner has revoked access through their Tesla portal?
We will throw a `CONNECTED_SERVICES_ACCOUNT:PERMISSION` error.
#### What happens if a user doesn’t select the correct permissions on Tesla’s side?
Connect maps Tesla’s permissions to Smartcar’s and only grants your application access to the ones requested. If we do not get the corresponding permission from Tesla, we will not grant your application access and throw the `PERMISSION:NULL` error as per usual when you make an API request.
Your users will need to [revoke access](\(/help/oem-integrations/tesla/faqs#how-can-my-users-fix-their-permissions-with-tesla\)) through the Tesla portal, then step through the flow again in order to fix this.
#### How can I tell which cars have gone through the new flow?
The [Migration Status](/api-reference/tesla/get-migration-status) make specific endpoint indicates if a vehicle still needs to be migrated to Tesla's new API.
#### Can owner and driver accounts authorize access with Smartcar through Connect?
Yes. Either account type can go through the Connect flow. As long as the Tesla account is attached to the vehicle, you can make requests to it.
#### Does the user need to be next to vehicle when adding a virtual key?
Owner accounts can add a virtual key remotely. Driver accounts need to be in
bluetooth range in order to add a virtual key.
#### Can driver accounts add a virtual key?
Yes. However, driver accounts can only pair virtual keys via bluetooth. They
cannot add a virtual key remotely.
#### Why are my users are seeing a location sharing notification on their navigation unit?
With the [recent changes to data
access](/help/oem-integrations/tesla/whats-new), vehicles will transmit their
live location if your application has requested access to location data. This
notification was put in place by Tesla to ensure transparency for owners about
what data is being shared and with whom.
The domain displayed will match the Virtual Key for your application.
#### How can my users update their permissions with Tesla?
Please [this guide](/help/oem-integrations/tesla/developers#updating-access-with-tesla) for details on how to do facilitate this for your users.
# Virtual Keys
Source: https://smartcar.com/docs/help/oem-integrations/tesla/virtual-key-tesla
This page has information regarding the use of Virtual Keys for Tesla vehicles.
## What is a Virtual Key?
A Virtual Key is a digital access method required by Tesla for third-party
applications to issue commands to Tesla vehicles and the preferred method for accessing data.
Please see [this
section](/help/oem-integrations/tesla/developers#adding-a-virtual-key)
for more information.
### Adding the Virtual Key
Smartcar handles this step on your behalf in the Connect flow. You can get started with the default Smartcar Virtual Key with no configurations required.
If you're on an **Enterprise** plan, please reach out to your Account Manager or
Solutions Architect for information on setting up a custom Virtual Key with your own brand name.
## Where can I find my Virtual Key?
Although Smartcar handles this step on your behalf, the Virtual Key URL for your application is sent back along with the authorization code after a user completes the Connect flow. You can redirect users to this URL if they need to add the Virtual Key again.
### Vehicle Owners adding a Virtual Key
Smartcar Connect will present Tesla vehicle owners a prompt to install the Tesla Virtual Key after granting access and prior to redirecting them back to your application.
The Virtual Key is a URL in the form:
```
https://www.tesla.com/_ak/smartcar.com
```
```
https://www.tesla.com/_ak/{{custom_subdomain}}.app.car
```
After prompting your users to open the link, depending on their device, they will be redirected to the Tesla app or prompted to scan a QR code.
Adding a Virtual Key will need to be done after a user has granted your application access to their Tesla account in the Connect flow.
Please see our [FAQs](/help/oem-integrations/tesla/faqs#can-owner-and-driver-accounts-authorize-access-with-smartcar-through-connect) for details on adding a virtual key for accounts with multiple vehicles and different account types.
On mobile devices, they will be redirected to the Tesla app and prompted to add the Virtual Key
On Desktop, they will be prompted to scan the QR code which in turn opens up the Tesla app and prompts them to add a Virtual Key
Virtual Key status can be checked at any time from the Tesla infotainment screen under Settings, Locks.
## Do all vehicles need a virtual key?
2020 and earlier Model S and X **will not** require a virtual key at this time. Data for these vehicles is refreshed every
5 minutes while the vehicle is awake.
## What if a streaming capable vehicle does not have a virtual key installed?
If a vehicle is streaming capable and does not yet have a virtual key installed,
requests to the vehicle will be limited to 1 request every 8 hours for data. These requests **will
not** wake the vehicle.
# Important Updates
Source: https://smartcar.com/docs/help/oem-integrations/tesla/whats-new
Upcoming changes to data access and permissions in 2025
## May 20, 2025
Smartcar now handles adding Virtual Keys for Tesla vehicles on your behalf in the Connect flow. Virtual Keys are required for third-party applications to issue commands to Tesla vehicles and are the preferred method for accessing data.
For more details, visit the [Virtual Key documentation](/help/oem-integrations/tesla/virtual-key-tesla).
## January 10, 2025
Starting in March 2025, Tesla will require a new permission to access live
location data for its vehicles. Due to this change, you have two options:
**If your application needs live location data:**
Users will need to reauthenticate and grant explicit access to the Vehicle
Location permission to continue receiving location data beyond March 2025, if
they haven’t already done so. This permission was previously included as part of
the Vehicle Information permission.
**If your application only needs to know whether the vehicle is at home:**
A new boolean value will be available soon, and it will not require
reauthentication. Vehicle owners can set their home location in the Tesla
application.
### Why is this change occurring?
Tesla now provides users more granular data access control, and requires vehicle
owners to explicitly consent to sharing location data with 3rd parties like
Smartcar. This ultimately gives drivers more control and awareness of how and
what data they decide to share.
### Next steps if you need live location data
**1. Check existing permissions**
Use the [User Access](/api-reference/tesla/get-user-access) endpoint to verify whether a vehicle has already
been granted the required permission on Tesla's side.
**2. Update Smartcar Connect scopes**
To ensure vehicle owners grant the new permission, append `required:` to your
scopes in the Smartcar Connect URL (for example, `required:read_location`). For
detailed instructions, please refer to [this guide](/help/oem-integrations/tesla/developers#requiring-tesla-permissions).
**3. Prompt users to reauthenticate**
If needed, guide users to reauthenticate their Tesla vehicles using the methods outlined
[here](/help/oem-integrations/tesla/developers#updating-access-with-tesla).
If the Vehicle Location permission isn’t granted by March 2025, you may
encounter a `PERMISSION` error. As a result, your
application will no longer receive live location data from affected Tesla
vehicles until they reauthenticate.
## December 19, 2024
Starting **January 21, 2025**, a Virtual Key will be required to maintain access to
Tesla vehicle data. Failure to make the necessary changes could result in a
disruption to your service after this date. Below, we’ve outlined the steps you
need to take to prepare, depending on your situation.
### For new developers
If you're on an **Enterprise** plan, please reach out to your Account Manager or
Solutions Architect for information on setting up your own Virtual Key.
**Free** and **Build** customers can get started with the default Virtual Key.
### For existing developers
**What’s changing?**
Telsa is changing the way data can be accessed by 3rd party applications.
**What do you need to do?**
To ensure continuous data access, please have your users add your Virtual Key to
their vehicles if they haven’t already. They can follow [this
guide](https://smartcar.com/docs/help/oem-integrations/tesla/developers#adding-a-virtual-key)
for more information. If you already prompt users to add a Virtual Key as your
application issues commands - no additional work is needed, please continue to
do so.
**What is a Virtual Key?**
A virtual key is a digital access method required by Tesla for third-party
applications to receive vehicle data and issue commands to Tesla vehicles.
Please see [this
guide](https://smartcar.com/docs/help/oem-integrations/tesla/developers#adding-a-virtual-key)
for more information.
**Where can I find my Virtual Key?**
The Virtual Key URL for your application will be sent back along with the
authorization code after a user completes the Connect flow.
Alternatively, you can reach out to Support or your Account Manager and they'll be able to provide
it to you.
**What happens if my users do not add my Virtual Key?**
Starting January 21, 2025 you will not be able to get data from Tesla vehicles
you are connected to and will receive a `virtual_key_required` error instead
([docs](https://smartcar.com/docs/errors/api-errors/connected-services-account-errors#virtual-key-required)).
**What about vehicles that do not support Virtual Keys?**
2020 and earlier Model S and X **will not** require a Virtual Key to keep
working at this time. However, you may not always receive the latest data from
the vehicle when you make an API request to Smartcar.
**Will my users need to reauthenticate?**
No. They simply need to add the Virtual Key to their vehicle.
**What’s changing?**
Tesla is changing the way data can be accessed by 3rd party applications.
**What do you need to do?**
To ensure continuous data access, please have your users add your Virtual Key to their vehicles if they haven’t already. They can
follow [this
guide](https://smartcar.com/docs/help/oem-integrations/tesla/developers#adding-a-virtual-key)
for more information. If you already prompt users to add a Virtual Key as your
application issues commands - no additional work is needed, please continue to
do so.
**What is a Virtual Key?**
A virtual key is a digital access method required by Tesla for third-party
applications to receive vehicle data and issue commands to Tesla vehicles.
Please see [this
guide](https://smartcar.com/docs/help/oem-integrations/tesla/developers#adding-a-virtual-key)
for more information.
**Where can I find my Virtual Key?**
The Virtual Key URL for your application will be sent back along with the
authorization code after a user completes the Connect flow. The Virtual Key URL
is:
[`https://www.tesla.com/_ak/smartcar.com`](https://www.tesla.com/_ak/smartcar.com)
**What happens if my users do not add my Virtual Key?**
Starting January 21, 2025 you will not be able to get data from Tesla vehicles
you are connected to and will receive a `virtual_key_required` error instead
([docs](https://smartcar.com/docs/errors/api-errors/connected-services-account-errors#virtual-key-required)).
**What about vehicles that do not support Virtual Keys?**
2020 and earlier Model S and X **will not** require a Virtual Key to keep
working at this time. However, you may not always receive the latest data from
the vehicle when you make an API request to Smartcar.
**Will my users need to reauthenticate?**
No. They simply need to add the Virtual Key to their vehicle.
# Important Updates
Source: https://smartcar.com/docs/help/oem-integrations/vw/whats-new
Updates regarding the Volkswagen Smartcar Integration.
## May 28th, 2025
Smartcar is excited to announce a new and improved integration with Volkswagen Group of America. is a testament to our mission of unlocking seamless mobility experiences for everyone.
Starting today, VW vehicle owners connecting their cars in the US to Smartcar powered applications will be redirected to Volkswagen's login page for authentication, vehicle selection, and acceptance of VW's terms as part of the Smartcar Connect flow. This change does not affect existing VW vehicle connections.
Vehicle owners will receive an email from Volkswagen notifying them of new services being added to their account.
Vehicle owners can revoke access to Smartcar powered applications at any time through the myVW Mobile App or by logginginto their account online.
### Notes
* This change is only applicable to VW vehicles in the US. Volkswagen vehicles in Canada and Europe will continue to use the existing Smartcar integration.
* Car Net does not allow secondary driver accounts to authorize access to any third-party applications including Smartcar. Only the primary account holder can authorize access to any third-party applications.
* For Volkswagen vehicles in the US, 3 minutes is the average of how often the data is refreshed.
# OEM Latency
Source: https://smartcar.com/docs/help/oem-latency
Request latency we've observed by make
## Acura
| HTTP Method | p95 (seconds) | Median (seconds) |
| ----------- | ------------- | ---------------- |
| GET | 22 | 2 |
| POST | 38 | 1 |
## Audi
| HTTP Method | p95 (seconds) | Median (seconds) |
| ----------- | ------------- | ---------------- |
| GET | 9 | 2 |
| POST | 55 | 28 |
## BMW/MINI
| HTTP Method | p95 (seconds) | Median (seconds) |
| ----------- | ------------- | ---------------- |
| GET | 5 | 2 |
| POST | 93 | 17 |
## Cupra
| HTTP Method | p95 (seconds) | Median (seconds) |
| ----------- | ------------- | ---------------- |
| GET | 9 | 4 |
| POST | 170 | 151 |
## Fiat
| HTTP Method | p95 (seconds) | Median (seconds) |
| ----------- | ------------- | ---------------- |
| GET | 9 | 7 |
## Ford
| HTTP Method | p95 (seconds) | Median (seconds) |
| ----------- | ------------- | ---------------- |
| GET | 42 | 11 |
| POST | 90 | 23 |
## GM (Buick, Chevrolet, Cadillac, GMC)
| HTTP Method | p95 (seconds) | Median (seconds) |
| ----------- | ------------- | ---------------- |
| GET | 145 | 33 |
| POST | 70 | 7 |
## Honda
| HTTP Method | p95 (seconds) | Median (seconds) |
| ----------- | ------------- | ---------------- |
| GET | 46 | 34 |
## Hyundai
| HTTP Method | p95 (seconds) | Median (seconds) |
| ----------- | ------------- | ---------------- |
| GET | 41 | 6 |
| POST | 125 | 16 |
## Infiniti
| HTTP Method | p95 (seconds) | Median (seconds) |
| ----------- | ------------- | ---------------- |
| GET | 29 | 18 |
| POST | 20 | 2 |
## Jaguar/Land Rover
| HTTP Method | p95 (seconds) | Median (seconds) |
| ----------- | ------------- | ---------------- |
| GET | 128 | 18 |
| POST | 182 | 66 |
## Kia
| HTTP Method | p95 (seconds) | Median (seconds) |
| ----------- | ------------- | ---------------- |
| GET | 120 | 3 |
| POST | 56 | 14 |
## Mazda
| HTTP Method | p95 (seconds) | Median (seconds) |
| ----------- | ------------- | ---------------- |
| GET | 9 | 5 |
| POST | 58 | 36 |
## Mercedes-Benz
| HTTP Method | p95 (seconds) | Median (seconds) |
| ----------- | ------------- | ---------------- |
| GET | 17 | 2 |
| POST | 271 | 129 |
## MG
| HTTP Method | p95 (seconds) | Median (seconds) |
| ----------- | ------------- | ---------------- |
| GET | 15 | 7 |
| POST | 26 | 8 |
## Nissan
| HTTP Method | p95 (seconds) | Median (seconds) |
| ----------- | ------------- | ---------------- |
| GET | 75 | 2 |
| POST | 50 | 2 |
## Porsche
| HTTP Method | p95 (seconds) | Median (seconds) |
| ----------- | ------------- | ---------------- |
| GET | 8 | 2 |
| POST | 29 | 10 |
## PSA Group (Citroen, DS, Opel, Peugeot, Vauxhall)
| HTTP Method | p95 (seconds) | Median (seconds) |
| ----------- | ------------- | ---------------- |
| GET | 26 | 4 |
| POST | 33 | 31 |
## Stellantis (Chrysler, Dodge, Jeep, RAM)
| HTTP Method | p95 (seconds) | Median (seconds) |
| ----------- | ------------- | ---------------- |
| GET | 6 | 4 |
| POST | 62 | 35 |
## Renault/Dacia
| HTTP Method | p95 (seconds) | Median (seconds) |
| ----------- | ------------- | ---------------- |
| GET | 3 | 2 |
| POST | 10 | 6 |
## Rivian
| HTTP Method | p95 (seconds) | Median (seconds) |
| ----------- | ------------- | ---------------- |
| GET | 3 | 1 |
## Skoda
| HTTP Method | p95 (seconds) | Median (seconds) |
| ----------- | ------------- | ---------------- |
| GET | 49 | 6 |
| POST | 15 | 13 |
## Subaru
| HTTP Method | p95 (seconds) | Median (seconds) |
| ----------- | ------------- | ---------------- |
| GET | 41 | 38 |
| POST | 39 | 21 |
## Tesla
| HTTP Method | p95 (seconds) | Median (seconds) |
| ----------- | ------------- | ---------------- |
| GET | 25 | 2 |
| POST | 15 | 2 |
## Toyota/Lexus
| HTTP Method | p95 (seconds) | Median (seconds) |
| ----------- | ------------- | ---------------- |
| GET | 152 | 25 |
| POST | 126 | 20 |
## Volkswagen
| HTTP Method | p95 (seconds) | Median (seconds) |
| ----------- | ------------- | ---------------- |
| GET | 11 | 4 |
| POST | 290 | 33 |
## Volvo
| HTTP Method | p95 (seconds) | Median (seconds) |
| ----------- | ------------- | ---------------- |
| GET | 144 | 20 |
| POST | 155 | 30 |
# Reliability and Data Age
Source: https://smartcar.com/docs/help/reliability-and-freshness
When making requests to vehicles, there is variation in how often data is updated and how many requests it can process in a given period. The tables below indicate the performance for each of Smartcar supported brands in each region.
**Data Reliability**
This ranking represents how reliably the vehicle or OEM processes data requests. A rank of 1 indicates the highest reliability.
**Command Reliability**
This ranking measures the effectiveness with which the vehicle or OEM handles command requests. A score of 1 reflects the most reliable command execution. N/A means there is not enough data to provide a score.
**Data Freshness**
This ranking indicates the recency of the data provided. In other words, the average time elapsed since the last update from the vehicle as opposed to when it was last fetched. A rank of 1 means the data is the freshest.
***
## United States
|
|
| -------------------------------------- | ------------------------------------------------- | ---------------------------------------------------- | ----------------------------------------------- |
| AUDI | 2 | 3 | 3 |
| BMW | 1 | 3 | 3 |
| CITROEN | 1 | 1 | 2 |
| CUPRA | 1 | 1 | 3 |
| DS | 1 | N/A | 2 |
| FORD | 2 | 3 | 2 |
| JAGUAR | 2 | 3 | 1 |
| KIA | 1 | 1 | 3 |
| LAND ROVER | 2 | 3 | 1 |
| MAZDA | 2 | 2 | 3 |
| MERCEDES-BENZ | 2 | 2 | 3 |
| MINI | 1 | 1 | 3 |
| NISSAN | 3 | 3 | 1 |
| OPEL | 1 | 3 | 3 |
| PEUGEOT | 1 | 1 | 1 |
| PORSCHE | 1 | 3 | 1 |
| RENAULT | 1 | 3 | 1 |
| SKODA | 2 | 1 | 1 |
| TESLA | 1 | 1 | 2 |
| VAUXHALL | 1 | 1 | 1 |
| VOLKSWAGEN | 1 | 2 | 3 |
| VOLVO | 3 | 3 | 1 |
Last Updated: June 2025
# Join the Research Fleet
Source: https://smartcar.com/docs/help/research-fleet
Smartcar is the easiest way to integrate apps with cars, funded by [a16z](https://a16z.com/), [NEA](https://www.nea.com/) and [Energize Capital](https://www.energizecap.com/).\
We enable companies like [Uber](https://smartcar.com/blog/uber), [Lyft](https://smartcar.com/blog/lyft), [Emulate](https://smartcar.com/blog/emulate), [Bluewave](https://smartcar.com/blog/bluwave-ai), [Neowatt](https://smartcar.com/blog/revenue-for-energy-retailers), and [more](https://smartcar.com/customers) to monitor a car's battery state of charge, verify mileage, manage EV charging, and much more.
We are always expanding to new makes and models of cars and we'd love your help!
## What are we asking for?
Building and maintaining our APIs is a complex endeavor. Smartcar operates a research fleet of vehicles across brands, models, and geographies to ensure we can provide the best product possible. **This is where you come in**. We'd like your help with understanding real-world vehicle behaviors with your car and improve the quality of our platform. Understanding things like:
* Does your car’s odometer update in real-time?
* What's the amount of fuel/battery your car has remaining?
* What data and remote actions are available for your specific car's brand, model, year?
## Perks of being part of the Research Fleet
* 💰 You will receive \$250 for the 6 months that your car is part of the fleet
* 🧥 Smartcar swag
* 🙋 Assistance setting up your car's connected services
## Sign up
## FAQs
The Smartcar team will schedule a check to be sent to your preferred address if your car is verified to be eligible for the Smartcar Research Fleet.
Smartcar will use your vehicle to retrieve and verify key data points such as your odometer and fuel tank/battery level. The team will inform you of all data and actions they will retrieve from your vehicle and the schedule at which they will be performing the verification. No action will be perfomed remotely without your permission.
You may opt-out at any point without any explanation. We simply ask that you give us a heads up that you no longer wish to participate by reaching out to [support@smartcar.com](mailto:support@smartcar.com).
# Vehicle Simulator
Source: https://smartcar.com/docs/help/vehicle-simulator
Learn about what to expect from Vehicle Simulator and how it compares to Live mode.
Vehicle Simulator allows you to test your application in realistic scenarios with a simulated Smartcar-compatible vehicle. Simulator responds to API requests just like a real vehicle. You can choose a simulated trip for your vehicle to take, and track it over time.
You can use the simulator for initial testing before you have a real vehicle to connect, or as part of an end-to-end testing suite.
## Creating a Simulated Vehicle
Simulated vehicles are managed in the Smartcar Dashboard.
Select "Add a vehicle" and select a vehicle by Make, Model, and Year, or provide a VIN for a US-based vehicle that you want to simulate. You'll be able to preview the API endpoints that are supported for the vehicle model you select.
Once you create your simulated vehicle, you'll select a simulated trip profile that the vehicle will follow. This profile can run automatically over time, or you can manually select a stage of the trip for the vehicle in the Dashboard.
## Connecting a Simulated Vehicle
Simulated vehicles generate a special set of credentials that mimic what a real-world vehicle owner would have. You'll use these credentials to establish a vehicle connection through the same Smartcar Connect flow that you will use for live vehicles.
In order for Connect to know you're using credentials generated from Vehicle Simulator, you'll need to pass `mode=simulated` in the [Connect URL](/connect/redirect-to-connect).
Please ensure you're passing or changing the country flag for Connect based on the region you've created your vehicle for.
Failure to do so will result in an invalid credential error on login. You can read more about country selection for Connect [here](/connect/advanced-config/country-flag).
You can set `mode` in the auth client builder to `simulated`.
You'll need to pass `mode=simulated` in the Connect URL
## Making requests to a Simulated Vehicle
Once your simulated vehicle is connected, you can make API requests to it just as you would a real vehicle.
Supported endpoints for the simulated vehicle will send responses with simulated data - unsupported endpoints will return errors just as they would with a real vehicle.
You can also send commands to simulated vehicles, like start/stop charge or lock/unlock doors. These requests will send responses that mimic that of a real vehicle, but the vehicle state will continue to reflect the simulated trip that was selected.
## Expected Responses
### Data
| Permission | Request Method / Path | Example Response |
| ------------------- | --------------------- | ------------------------------------------------------------------------------------------- |
| `read_engine_oil` | GET /engine/oil | `{ lifeRemaining: 0.35 }` |
| `read_battery` | GET /battery | `{ percentRemaining: 0.3, range: 40.5 }` |
| `read_battery` | GET /battery/capacity | `{ capacity: 28 }` |
| `read_charge` | GET /charge | `{ isPluggedIn: true, state: 'FULLY_CHARGED' }` |
| `read_charge` | GET /charge/limit | `{ limit: 1 }` |
| `read_fuel` | GET /fuel | `{ amountRemaining: 53.2, percentRemaining: 0.3, range: 40.5 }` |
| `read_location` | GET /location | `{ latitude: 37.4292, longitude: 122.1382 }` |
| `read_odometer` | GET /odometer | `{ distance: 104.32 }` |
| `read_tires` | GET /tires/pressure | `{ backLeft: 219.3, backRight: 219.3, frontLeft: 219.3, frontRight: 219.3 }` |
| `read_vehicle_info` | GET / | `{ id: 36ab27d0-fd9d-4455-823a-ce30af709ffc, make: "TESLA", model: "Model S", year: 2014 }` |
| `read_vin` | GET /vin | `{ vin: 1234A67Q90F2T4567 }` |
### Commands
Below are the various action requests that can be made to simulated vehicles, and what responses to expect based on the simulated vehicle's current state or simulator support.
Not listed here are the standard API errors that will be returned if the vehicle does not support the capability or the application has not been granted permission.
| Permission | Request (method / path) | Action request | Vehicle state | Response |
| ------------------ | ----------------------- | -------------- | --------------------------------- | -------------------------------------------------------------- |
| `control_security` | POST /security | `LOCK` | Parked / Charging | `{ status: "success" }` |
| `control_security` | POST /security | `UNLOCK` | Parked / Charging | `{ status: "success" }` |
| `control_security` | POST /security | `LOCK` | Driving | `{ type: VEHICLE_STATE, code: IN_MOTION }` |
| `control_security` | POST /security | `UNLOCK` | Driving | `{ type: VEHICLE_STATE, code: IN_MOTION }` |
| `control_charge` | POST /charge | `START` | Charging | `{ status: "success" }` |
| `control_charge` | POST /charge | `STOP` | Charging | `{ status: "success" }` |
| `control_charge` | POST /charge | `STOP` | Parked / Driving (not plugged in) | `{ type: VEHICLE_STATE, code: CHARGING_PLUG_NOT_CONNECTED }` |
| `control_charge` | POST /charge | `START` | Parked / Driving (not plugged in) | `{ type: VEHICLE_STATE, code: CHARGING_PLUG_NOT_CONNECTED }` |
| `control_charge` | POST /charge-limit | `` | Charging | `{ "type": "COMPATIBILITY", "code": "SMARTCAR_NOT_CAPABLE", }` |
| `control_charge` | POST /charge-limit | `` | Parked / Driving (not plugged in) | `{ type: VEHICLE_STATE, code: CHARGING_PLUG_NOT_CONNECTED }` |
### Errors
| Event | Error response | Suggested resolution |
| ------------------------------------------------------------------------------------------------------------------- | ---------------------------------------------------------------------------------- | ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| Making an API request to a simulated vehicle before connecting the vehicle to your application via Smartcar Connect | `{ type: "PERMISSION", statusCode: 403, resolution: "REAUTHENTICATE" }` | Locate the 'Connect Credentials' button at the top of the simulation screen in the Smartcar Dashboard for your simulated vehicle. There you will find the credentials necessary to connect the vehicle to your application as well as instructions for doing so through Smartcar Connect. |
| Making an API request to a simulated vehicle before a simulation has been started on Dashboard | `{ type: "CONNECTED_SERVICES_ACCOUNT", code: "ACCOUNT_ISSUE", statusCode: 400 }` | Open the Simulator tab in the Smartcar Dashboard and navigate to your target vehicle. If you haven't yet selected a vehicle state, do so now, and then start the simulation using the 'Play' button on the simulation screen. |
| Making an API request to a simulated vehicle that is not associated with your application | `{ type: "CONNECTED_SERVICES_ACCOUNT", code: "VEHICLE_MISSING", statusCode: 400 }` | Ensure the simulated vehicle you are making requests to has been created in the Simulator within your Smartcar Dashboard. |
## Mode Comparison
| Feature | Simulator | Live | |
| ------------------------------------ | ------------------------------------- | -------------------------------- | - |
| Single Select | ✅ | ✅ | |
| Brand Select | ✅ | ✅ | |
| Compatibility API\* | ✅ | ✅ | |
| `control_security` | ✅ | ✅ | |
| `control_charge` | ✅ | ✅ | |
| Make-Specific Endpoints | ❌ | ✅ | |
| Scheduled Webhooks | ❌ | ✅ | |
| Event Based Webhooks\*\* | ❌ | ✅ | |
\*When a real VIN is used either through `VIN@smartcar` for `test` mode, or generating a simulated vehicle with a real VIN.
0SC and 1SC prefixed VINs will not work.
\*\*For supported brands - Toyota, Ford and Tesla.
## FAQs
Access and refresh tokens behave as they do in `live` mode:
* Access Tokens are valid for 2 hours
* Refresh Tokens are valid for 60 days.
* Using a refresh token to fetch an new access/refresh token pair will invalidate the refresh token that you used.
**Reusing simulated vehicle credentials**
If you use the same simulated credentials for multiple Connect flow sessions, we'll issue a new set of tokens each time.
This will not invalidate any existing tokens from previous logins so you'll be able to keep them refreshed in parallel without impacting the validity of the others.
The number of simulated vehicles that can be created depends on your Smartcar plan tier. Please see [here](https://smartcar.com/pricing#plans) for more information.
* Please check that you have launched Connect with the `mode=simulated` parameter
* Please check that you are passing the country feature flag for the region you've set up your vehicle with.
Please see [Country Selection](/connect/advanced-config/country-flag)for Connect for more information.
# What is a Connected Car?
Source: https://smartcar.com/docs/help/what-is-a-connected-car
Learn about how to build applications that connect to millions of vehicles around the world.
Connected cars are vehicles that have in-built cellular modems. Most vehicles manufactured after 2015 have been shipping with this connectivity, or "embedded telematics." This essentially makes cars "smart" allowing them to communicate with the Internet. Take a look at this blog post for an in depth primer.
# What is Smartcar?
Source: https://smartcar.com/docs/help/what-is-smartcar
Learn about how to build applications that connect to millions of vehicles around the world.
The Smartcar platform allows mobility businesses to easily connect with their customers’ cars. Whether you need to track a vehicle’s location,
verify its mileage, share a virtual key, start charging an EV, monitor a car’s fuel tank level, inspect the tire pressure, or check the engine
oil life, Smartcar lets you do so with a single API request.
Take a look at the video below for a quick overview of how Smartcar works.